Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1305376 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdownWithoutDemand(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdownWithoutDemand(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
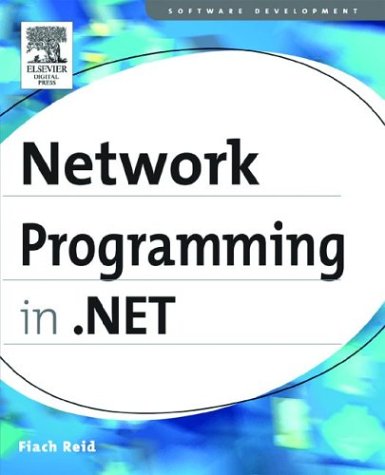
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UpdateException.cs
- DesignerLinkAdapter.cs
- Page.cs
- EmptyEnumerator.cs
- NativeWrapper.cs
- BinaryHeap.cs
- InsufficientExecutionStackException.cs
- Int32CAMarshaler.cs
- SettingsPropertyCollection.cs
- CustomAssemblyResolver.cs
- SocketSettings.cs
- LineMetrics.cs
- CodeSubDirectoriesCollection.cs
- ContentType.cs
- MultiSelector.cs
- FileNotFoundException.cs
- TrustSection.cs
- KeyValueConfigurationElement.cs
- ToolZone.cs
- XmlSchemaSimpleTypeRestriction.cs
- MultiView.cs
- ProxyBuilder.cs
- Int32Animation.cs
- FontUnit.cs
- SolidColorBrush.cs
- SQLDecimal.cs
- TextSegment.cs
- recordstatefactory.cs
- CustomAttributeBuilder.cs
- AuthenticationConfig.cs
- WindowsSidIdentity.cs
- Expression.DebuggerProxy.cs
- MulticastIPAddressInformationCollection.cs
- EncryptedPackage.cs
- WindowsTab.cs
- CFStream.cs
- NodeInfo.cs
- SqlDuplicator.cs
- Signature.cs
- ListBoxItem.cs
- ConfigsHelper.cs
- BooleanFacetDescriptionElement.cs
- ConnectionsZone.cs
- InstanceNameConverter.cs
- ObjectViewEntityCollectionData.cs
- CustomAttributeSerializer.cs
- CaseInsensitiveHashCodeProvider.cs
- RtType.cs
- AvTraceDetails.cs
- DiscoveryOperationContextExtension.cs
- DataGridDetailsPresenter.cs
- BasicCellRelation.cs
- IndependentAnimationStorage.cs
- ReferencedCategoriesDocument.cs
- ToolZone.cs
- OrderPreservingSpoolingTask.cs
- BoolExpression.cs
- MessageFault.cs
- CodeSpit.cs
- SerialReceived.cs
- AutoGeneratedFieldProperties.cs
- XPathChildIterator.cs
- Point4D.cs
- sqlstateclientmanager.cs
- ApplicationTrust.cs
- ProvidePropertyAttribute.cs
- BinaryUtilClasses.cs
- IncrementalHitTester.cs
- VectorAnimationUsingKeyFrames.cs
- OpacityConverter.cs
- HttpPostLocalhostServerProtocol.cs
- ParagraphVisual.cs
- SafeReadContext.cs
- DecoderFallback.cs
- HTMLTextWriter.cs
- LazyInitializer.cs
- SpellerInterop.cs
- ProcessHostServerConfig.cs
- RemotingConfiguration.cs
- JsonReader.cs
- SatelliteContractVersionAttribute.cs
- VariableQuery.cs
- XPathSelectionIterator.cs
- TextInfo.cs
- SecurityUtils.cs
- SrgsDocument.cs
- ContainerSelectorGlyph.cs
- TimeoutValidationAttribute.cs
- MarkupCompilePass1.cs
- XmlProcessingInstruction.cs
- MtomMessageEncodingBindingElement.cs
- TemplateField.cs
- ObjectDataSourceStatusEventArgs.cs
- IRCollection.cs
- AutomationPeer.cs
- GridEntry.cs
- Transform3DCollection.cs
- RpcCryptoRequest.cs
- Transform.cs
- RequestCacheManager.cs