Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / Publisher.cs / 1305376 / Publisher.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // Publisher.cs // // Publisher is an IIdentity representing internet sites. // namespace System.Security.Policy { using System.Runtime.Remoting; using System; using System.IO; using System.Security.Util; using System.Collections; using PublisherIdentityPermission = System.Security.Permissions.PublisherIdentityPermission; using System.Security.Cryptography.X509Certificates; using System.Diagnostics.Contracts; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Publisher : EvidenceBase, IIdentityPermissionFactory { private X509Certificate m_cert; public Publisher(X509Certificate cert) { if (cert == null) throw new ArgumentNullException("cert"); Contract.EndContractBlock(); m_cert = cert; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new PublisherIdentityPermission( m_cert ); } // Two Publisher objects are equal if the public keys contained within their certificates // are equal. The certs themselves may be different... public override bool Equals(Object o) { Publisher that = (o as Publisher); return (that != null && PublicKeyEquals( this.m_cert, that.m_cert )); } // Checks if two certificates have the same public key, keyalg, and keyparam. internal static bool PublicKeyEquals( X509Certificate cert1, X509Certificate cert2 ) { if (cert1 == null) { return (cert2 == null); } else if (cert2 == null) { return false; } byte[] publicKey1 = cert1.GetPublicKey(); String keyAlg1 = cert1.GetKeyAlgorithm(); byte[] keyAlgParam1 = cert1.GetKeyAlgorithmParameters(); byte[] publicKey2 = cert2.GetPublicKey(); String keyAlg2 = cert2.GetKeyAlgorithm(); byte[] keyAlgParam2 = cert2.GetKeyAlgorithmParameters(); // Keys are most likely to be different of the three components, // so check them first int len = publicKey1.Length; if (len != publicKey2.Length) return(false); for (int i = 0; i < len; i++) { if (publicKey1[i] != publicKey2[i]) return(false); } if (!(keyAlg1.Equals(keyAlg2))) return(false); len = keyAlgParam1.Length; if (keyAlgParam2.Length != len) return(false); for (int i = 0; i < len; i++) { if (keyAlgParam1[i] != keyAlgParam2[i]) return(false); } return true; } public override int GetHashCode() { return m_cert.GetHashCode(); } public X509Certificate Certificate { get { return new X509Certificate(m_cert); } } public override EvidenceBase Clone() { return new Publisher(m_cert); } public object Copy() { return Clone(); } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Publisher" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Publisher" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); elem.AddChild( new SecurityElement( "X509v3Certificate", m_cert != null ? m_cert.GetRawCertDataString() : "" ) ); return elem; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { MemoryStream ms = new MemoryStream(m_cert.GetRawCertData()); ms.Position = 0; return ms; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // Publisher.cs // // Publisher is an IIdentity representing internet sites. // namespace System.Security.Policy { using System.Runtime.Remoting; using System; using System.IO; using System.Security.Util; using System.Collections; using PublisherIdentityPermission = System.Security.Permissions.PublisherIdentityPermission; using System.Security.Cryptography.X509Certificates; using System.Diagnostics.Contracts; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Publisher : EvidenceBase, IIdentityPermissionFactory { private X509Certificate m_cert; public Publisher(X509Certificate cert) { if (cert == null) throw new ArgumentNullException("cert"); Contract.EndContractBlock(); m_cert = cert; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new PublisherIdentityPermission( m_cert ); } // Two Publisher objects are equal if the public keys contained within their certificates // are equal. The certs themselves may be different... public override bool Equals(Object o) { Publisher that = (o as Publisher); return (that != null && PublicKeyEquals( this.m_cert, that.m_cert )); } // Checks if two certificates have the same public key, keyalg, and keyparam. internal static bool PublicKeyEquals( X509Certificate cert1, X509Certificate cert2 ) { if (cert1 == null) { return (cert2 == null); } else if (cert2 == null) { return false; } byte[] publicKey1 = cert1.GetPublicKey(); String keyAlg1 = cert1.GetKeyAlgorithm(); byte[] keyAlgParam1 = cert1.GetKeyAlgorithmParameters(); byte[] publicKey2 = cert2.GetPublicKey(); String keyAlg2 = cert2.GetKeyAlgorithm(); byte[] keyAlgParam2 = cert2.GetKeyAlgorithmParameters(); // Keys are most likely to be different of the three components, // so check them first int len = publicKey1.Length; if (len != publicKey2.Length) return(false); for (int i = 0; i < len; i++) { if (publicKey1[i] != publicKey2[i]) return(false); } if (!(keyAlg1.Equals(keyAlg2))) return(false); len = keyAlgParam1.Length; if (keyAlgParam2.Length != len) return(false); for (int i = 0; i < len; i++) { if (keyAlgParam1[i] != keyAlgParam2[i]) return(false); } return true; } public override int GetHashCode() { return m_cert.GetHashCode(); } public X509Certificate Certificate { get { return new X509Certificate(m_cert); } } public override EvidenceBase Clone() { return new Publisher(m_cert); } public object Copy() { return Clone(); } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Publisher" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Publisher" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); elem.AddChild( new SecurityElement( "X509v3Certificate", m_cert != null ? m_cert.GetRawCertDataString() : "" ) ); return elem; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { MemoryStream ms = new MemoryStream(m_cert.GetRawCertData()); ms.Position = 0; return ms; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
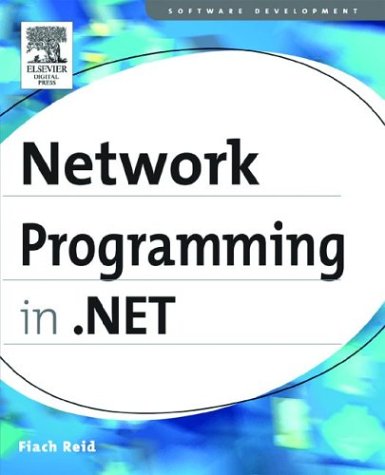
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- precedingquery.cs
- CodeComment.cs
- QualifiedCellIdBoolean.cs
- SchemaImporter.cs
- ContentHostHelper.cs
- AvTrace.cs
- ValidationSettings.cs
- HuffCodec.cs
- Symbol.cs
- DurableInstanceProvider.cs
- CellRelation.cs
- LockedHandleGlyph.cs
- ValidationResult.cs
- Compiler.cs
- XmlSchemaInferenceException.cs
- Sequence.cs
- remotingproxy.cs
- DefaultValueAttribute.cs
- Calendar.cs
- OledbConnectionStringbuilder.cs
- XmlSequenceWriter.cs
- IntSumAggregationOperator.cs
- InputGestureCollection.cs
- PrtTicket_Public.cs
- TreeView.cs
- IssuanceLicense.cs
- MatcherBuilder.cs
- ADMembershipProvider.cs
- HttpCapabilitiesSectionHandler.cs
- ContextMenuStrip.cs
- MessageHeaderAttribute.cs
- MethodImplAttribute.cs
- NamedPermissionSet.cs
- ToolStripDropDownButton.cs
- Deflater.cs
- RpcCryptoRequest.cs
- TreeView.cs
- XamlSerializationHelper.cs
- XmlObjectSerializerReadContextComplexJson.cs
- Stacktrace.cs
- GeneralTransform.cs
- LinqDataSourceEditData.cs
- TextTreeDeleteContentUndoUnit.cs
- ManifestSignedXml.cs
- ConditionCollection.cs
- SmiConnection.cs
- CommonDialog.cs
- PackageRelationshipCollection.cs
- Link.cs
- CompiledScopeCriteria.cs
- Rijndael.cs
- X500Name.cs
- DataControlFieldHeaderCell.cs
- FormViewCommandEventArgs.cs
- ContextBase.cs
- FontStretch.cs
- PrintDialogException.cs
- SqlBulkCopyColumnMapping.cs
- PrintEvent.cs
- X509RecipientCertificateServiceElement.cs
- ErrorHandler.cs
- AsymmetricAlgorithm.cs
- UpdatePanelControlTrigger.cs
- ConfigXmlWhitespace.cs
- DocumentPageViewAutomationPeer.cs
- SessionStateItemCollection.cs
- QueryRewriter.cs
- OleAutBinder.cs
- MarshalByRefObject.cs
- DateBoldEvent.cs
- XmlException.cs
- XmlnsCache.cs
- BrowsableAttribute.cs
- SqlDataSourceCommandEventArgs.cs
- QilDataSource.cs
- DateTimePicker.cs
- EntityDataSourceDataSelection.cs
- HtmlTable.cs
- ToolStripControlHost.cs
- DelayDesigner.cs
- StyleXamlParser.cs
- HostingPreferredMapPath.cs
- BaseCollection.cs
- Oid.cs
- BrowserCapabilitiesCompiler.cs
- Label.cs
- FileDialog.cs
- BitmapCodecInfo.cs
- Scene3D.cs
- SymmetricKeyWrap.cs
- PageAsyncTask.cs
- MethodSignatureGenerator.cs
- PrintEvent.cs
- XmlSerializerVersionAttribute.cs
- Compress.cs
- Constraint.cs
- X509Certificate.cs
- IisTraceListener.cs
- AnimatedTypeHelpers.cs
- OutputCacheProfile.cs