Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / DesignParameter.cs / 1 / DesignParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System.Diagnostics; using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Text; using System.Globalization; [ DataSourceXmlClass(SchemaName.Parameter) ] internal class DesignParameter: DataSourceComponent, IDbDataParameter, IDataSourceNamedObject, ICloneable { private string parameterName; private string columnName; private string autogeneratedName; // Used while substituting VSDB-generated parameter names (ParamXx) with our, "pretty" names. private DbType dbType; private byte precision; private byte scale; private int size; private string dataTypeServer; private ParameterDirection direction; private string sourceColumn; private DataRowVersion sourceVersion; private string dataSourceName; private bool sourceColumnNullMapping; private bool allowDbNull; private string providerType = null; private static int[] adoDataType2DbType = new int[] { (int) ADODataType.adBSTR, (int) DbType.AnsiString, (int) ADODataType.adBigInt, (int) DbType.Int64, (int) ADODataType.adBinary, (int) DbType.Binary, (int) ADODataType.adBoolean, (int) DbType.Boolean, (int) ADODataType.adChar, (int) DbType.AnsiString, (int) ADODataType.adCurrency, (int) DbType.Currency, (int) ADODataType.adDate, (int) DbType.Date, (int) ADODataType.adDBDate, (int) DbType.Date, (int) ADODataType.adDBTime, (int) DbType.DateTime, (int) ADODataType.adDBTimeStamp, (int) DbType.DateTime, (int) ADODataType.adDecimal, (int) DbType.Decimal, (int) ADODataType.adDouble, (int) DbType.Double, (int) ADODataType.adEmpty, (int) DbType.Object, (int) ADODataType.adError, (int) DbType.Object, (int) ADODataType.adFileTime, (int) DbType.DateTime, (int) ADODataType.adGUID, (int) DbType.Guid, (int) ADODataType.adIDispatch, (int) DbType.Object, (int) ADODataType.adIUnknown, (int) DbType.Object, (int) ADODataType.adInteger, (int) DbType.Int32, (int) ADODataType.adLongVarBinary, (int) DbType.Binary, (int) ADODataType.adLongVarChar, (int) DbType.AnsiString, (int) ADODataType.adLongVarWChar, (int) DbType.String, (int) ADODataType.adNumeric, (int) DbType.Decimal, (int) ADODataType.adPropVariant, (int) DbType.Object, (int) ADODataType.adSingle, (int) DbType.Single, (int) ADODataType.adSmallInt, (int) DbType.Int16, (int) ADODataType.adTinyInt, (int) DbType.SByte, (int) ADODataType.adUnsignedBigInt, (int) DbType.UInt64, (int) ADODataType.adUnsignedInt, (int) DbType.UInt32, (int) ADODataType.adUnsignedSmallInt, (int) DbType.UInt16, (int) ADODataType.adUnsignedTinyInt, (int) DbType.Byte, (int) ADODataType.adVarBinary, (int) DbType.Binary, (int) ADODataType.adVarChar, (int) DbType.AnsiString, (int) ADODataType.adVarNumeric, (int) DbType.VarNumeric, (int) ADODataType.adVarWChar, (int) DbType.String, (int) ADODataType.adVariant, (int) DbType.Object, (int) ADODataType.adWChar, (int) DbType.String }; [ DataSourceXmlAttribute(), DefaultValue(false) ] public bool AllowDbNull { get { return this.allowDbNull; } set { this.allowDbNull = value; } } [ DataSourceXmlAttribute(), MergableProperty(false), DefaultValue(""), ] public string ParameterName { get { return this.parameterName; } set { if (!StringUtil.EqualValue(this.ParameterName, value)) { if (this.CollectionParent != null) { CollectionParent.ValidateUniqueName(this, value); } this.parameterName = value; } } } [ DataSourceXmlAttribute() ] public string ColumnName { get { return this.columnName; } set { this.columnName = value; } } [ DataSourceXmlAttribute(), Browsable(false) ] public string AutogeneratedName { get { return this.autogeneratedName; } set { this.autogeneratedName = value; } } [ DataSourceXmlAttribute() ] public DbType DbType { get { return this.dbType; } set { this.dbType = value; } } [ DataSourceXmlAttribute() ] public string ProviderType { get { return this.providerType; } set { this.providerType = value; } } [ DataSourceXmlAttribute(), DefaultValueAttribute((byte)0) ] public byte Precision { get { return this.precision; } set { this.precision = value; } } [ DataSourceXmlAttribute(), DefaultValueAttribute((byte)0) ] public byte Scale { get { return this.scale; } set { this.scale = value; } } [ DataSourceXmlAttribute(), DefaultValueAttribute((int)0) ] public int Size { get { return this.size; } set { this.size = value; // For BLOBs VSDB will return 0xffffffff; if( this.size < 0 ) { this.size = int.MaxValue; } } } [ DataSourceXmlAttribute(), Browsable(false) ] public string DataTypeServer { get { return this.dataTypeServer; } set { this.dataTypeServer = value; } } [ DataSourceXmlAttribute(), DefaultValue(ParameterDirection.Input) ] public ParameterDirection Direction { get { return this.direction; } set { this.direction = value; } } [ DefaultValue(""), DataSourceXmlAttribute() ] public string SourceColumn { get { return this.sourceColumn; } set { this.sourceColumn = value; } } [ DefaultValue(DataRowVersion.Current), DataSourceXmlAttribute() ] public DataRowVersion SourceVersion { get { return this.sourceVersion; } set { this.sourceVersion = value; } } [ DataSourceXmlAttribute(), Browsable(false) ] public string DataSourceName { get { return this.dataSourceName; } set { this.dataSourceName = value; } } string INamedObject.Name { get { return ParameterName; } set { ParameterName = value; } } string IDataSourceNamedObject.PublicTypeName { get { return "Parameter"; } } [ Browsable(false), DefaultValue(false), DesignOnly(true), EditorBrowsableAttribute(EditorBrowsableState.Never) ] public bool IsNullable { get { return true; } } [Browsable(false), DefaultValue(null)] public object Value { get { return null; } set { // noop } } ////// This is for the object collection editor. Overriding ToString() is not enough in case of DesignParameter because /// it is a component. Because of that TypeConverter will try to use Site.Name when converting to string, this will /// fail and they will fall back to class name. /// If that is not acceptable we should implement a custom type converter for DesignParameter. /// [Browsable(false)] public string Name { get { return this.ParameterName; } } [ DefaultValue(false), DataSourceXmlAttribute() ] public bool SourceColumnNullMapping { get { return this.sourceColumnNullMapping; } set { this.sourceColumnNullMapping = value; } } public override string ToString() { return this.ParameterName; } public const string DEFAULT_RETVAL_NAME = "RETURN_VALUE"; // this constructor is only used by serializor.. public DesignParameter() : this(String.Empty) { } public DesignParameter (string name) { this.parameterName = name; this.autogeneratedName = string.Empty; this.direction = ParameterDirection.Input; this.sourceVersion = DataRowVersion.Current; this.dataSourceName = string.Empty; } public override bool Equals( object o ) { DesignParameter p = o as DesignParameter; if( p == null ) return false; return p.ParameterName.Equals( this.ParameterName ); } // This is just to make compiler happy (because we're overriding Equals( object ) ) public override int GetHashCode() { return this.ParameterName.GetHashCode(); } public object Clone() { DesignParameter p = new DesignParameter( this.ParameterName ); p.ColumnName = ColumnName; p.AutogeneratedName = AutogeneratedName; p.DbType = DbType; p.Precision = Precision; p.Scale = Scale; p.Size = Size; p.DataTypeServer = DataTypeServer; p.Direction = Direction; p.SourceColumn = SourceColumn; p.SourceVersion = SourceVersion; p.SourceColumnNullMapping = SourceColumnNullMapping; return p; } public static DesignParameter GetDefaultRetvalParam() { DesignParameter p = new DesignParameter( DEFAULT_RETVAL_NAME ); p.DbType = DbType.Int32; p.Precision = p.Scale = 0; p.Size = 4; p.Direction = ParameterDirection.ReturnValue; return p; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
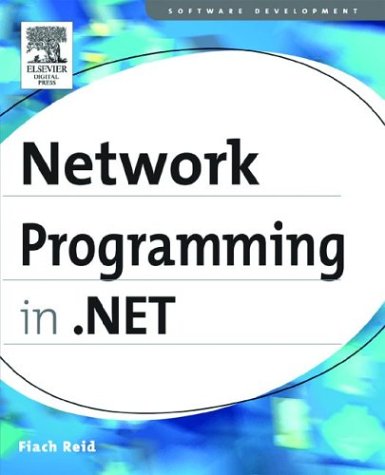
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMElement.cs
- AtlasWeb.Designer.cs
- InputMethodStateTypeInfo.cs
- CngProperty.cs
- ScalarConstant.cs
- DictionaryBase.cs
- ConnectionManagementElement.cs
- IntPtr.cs
- AuthenticationServiceManager.cs
- Rss20FeedFormatter.cs
- indexingfiltermarshaler.cs
- DbConnectionClosed.cs
- HtmlLabelAdapter.cs
- SvcMapFile.cs
- SR.Designer.cs
- TextReader.cs
- NotSupportedException.cs
- DescendantOverDescendantQuery.cs
- TextSpan.cs
- ClientFormsIdentity.cs
- BitmapEffectInput.cs
- AutomationPropertyInfo.cs
- TabControlDesigner.cs
- StorageInfo.cs
- EntityDataSourceSelectedEventArgs.cs
- GregorianCalendarHelper.cs
- ErrorHandlerModule.cs
- ClientApiGenerator.cs
- RangeValuePattern.cs
- BaseAsyncResult.cs
- EpmSourcePathSegment.cs
- EntityReference.cs
- mediapermission.cs
- KeyGestureValueSerializer.cs
- TdsEnums.cs
- Int32AnimationBase.cs
- CodeCatchClauseCollection.cs
- Condition.cs
- DoubleAnimationBase.cs
- ContextMenu.cs
- ObjectTag.cs
- ApplicationFileCodeDomTreeGenerator.cs
- CreateParams.cs
- DefaultBindingPropertyAttribute.cs
- ListBoxItem.cs
- SubMenuStyleCollection.cs
- Dynamic.cs
- ProfileElement.cs
- HtmlEncodedRawTextWriter.cs
- ContentTextAutomationPeer.cs
- LinearKeyFrames.cs
- HostingPreferredMapPath.cs
- Html32TextWriter.cs
- OdbcRowUpdatingEvent.cs
- AbandonedMutexException.cs
- ConstraintCollection.cs
- SerialPort.cs
- CompiledELinqQueryState.cs
- PersonalizationAdministration.cs
- FileIOPermission.cs
- Utils.cs
- DesignerLoader.cs
- ScrollChrome.cs
- CqlLexer.cs
- DataObjectSettingDataEventArgs.cs
- WebPartVerbCollection.cs
- PlainXmlDeserializer.cs
- Converter.cs
- Misc.cs
- WindowClosedEventArgs.cs
- GeneralTransform3DGroup.cs
- DiscreteKeyFrames.cs
- AmbientLight.cs
- QueryResponse.cs
- DateTime.cs
- TemplateBuilder.cs
- ImageField.cs
- Int64AnimationUsingKeyFrames.cs
- ToolStripManager.cs
- TransactionsSectionGroup.cs
- SemaphoreFullException.cs
- ClientConfigPaths.cs
- CapabilitiesSection.cs
- HtmlTable.cs
- SqlDelegatedTransaction.cs
- ChangeNode.cs
- BitmapDownload.cs
- TableCellAutomationPeer.cs
- SqlFacetAttribute.cs
- TTSEngineProxy.cs
- BaseTemplateBuildProvider.cs
- PostBackOptions.cs
- HttpHeaderCollection.cs
- FactoryMaker.cs
- OutOfProcStateClientManager.cs
- SrgsElementFactoryCompiler.cs
- Point3D.cs
- StructuredType.cs
- DependencyObject.cs
- PropertyGeneratedEventArgs.cs