Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ReplyChannelBinder.cs / 1 / ReplyChannelBinder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Diagnostics; class ReplyChannelBinder : IChannelBinder { IReplyChannel channel; Uri listenUri; internal ReplyChannelBinder(IReplyChannel channel, Uri listenUri) { if (!((channel != null))) { DiagnosticUtility.DebugAssert("ReplyChannelBinder.ReplyChannelBinder: (channel != null)"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("channel"); } this.channel = channel; this.listenUri = listenUri; } public IChannel Channel { get { return this.channel; } } public bool HasSession { get { return this.channel is ISessionChannel; } } public Uri ListenUri { get { return this.listenUri; } } public EndpointAddress LocalAddress { get { return this.channel.LocalAddress; } } public EndpointAddress RemoteAddress { get { #pragma warning suppress 56503 // [....], the property is really not implemented, cannot lie, API not public throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } public void Abort() { this.channel.Abort(); } public void CloseAfterFault(TimeSpan timeout) { this.channel.Close(timeout); } public IAsyncResult BeginTryReceive(TimeSpan timeout, AsyncCallback callback, object state) { return this.channel.BeginTryReceiveRequest(timeout, callback, state); } public bool EndTryReceive(IAsyncResult result, out RequestContext requestContext) { return this.channel.EndTryReceiveRequest(result, out requestContext); } public IAsyncResult BeginSend(Message message, TimeSpan timeout, AsyncCallback callback, object state) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public void EndSend(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public void Send(Message message, TimeSpan timeout) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public IAsyncResult BeginRequest(Message message, TimeSpan timeout, AsyncCallback callback, object state) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public Message EndRequest(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool TryReceive(TimeSpan timeout, out RequestContext requestContext) { return this.channel.TryReceiveRequest(timeout, out requestContext); } public Message Request(Message message, TimeSpan timeout) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public bool WaitForMessage(TimeSpan timeout) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool EndWaitForMessage(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
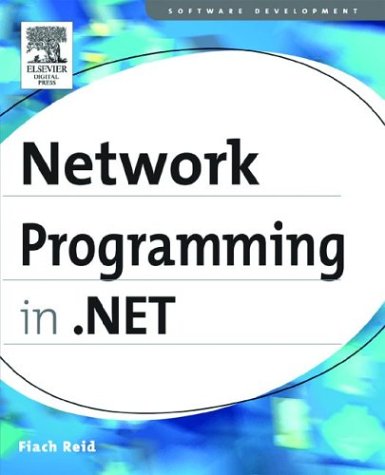
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemParameters.cs
- DbUpdateCommandTree.cs
- ReadonlyMessageFilter.cs
- DbException.cs
- DataStorage.cs
- TypeReference.cs
- DrawingContextWalker.cs
- CodeSnippetExpression.cs
- LogLogRecordHeader.cs
- XmlSchemaGroup.cs
- ExceptionHandler.cs
- HttpApplicationFactory.cs
- WebPartExportVerb.cs
- TextEditorTyping.cs
- TemplateAction.cs
- TimeoutConverter.cs
- WeakHashtable.cs
- RelatedCurrencyManager.cs
- EdmConstants.cs
- WindowsScroll.cs
- PropertyDescriptorComparer.cs
- DrawingAttributesDefaultValueFactory.cs
- PropertyGridView.cs
- CursorInteropHelper.cs
- TextBoxView.cs
- SafeBitVector32.cs
- SoapIgnoreAttribute.cs
- RectKeyFrameCollection.cs
- ToolStripOverflow.cs
- LiteralTextParser.cs
- ObjectStateFormatter.cs
- RowCache.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- TextDecorationCollection.cs
- DeploymentExceptionMapper.cs
- XhtmlConformanceSection.cs
- ParserStreamGeometryContext.cs
- WebBrowserDesigner.cs
- COM2ComponentEditor.cs
- BridgeDataReader.cs
- SecureUICommand.cs
- AutomationPropertyInfo.cs
- Function.cs
- AuthenticationService.cs
- CorrelationManager.cs
- CachedTypeface.cs
- ExpressionVisitor.cs
- SoapExtensionTypeElementCollection.cs
- ListCollectionView.cs
- EdmProperty.cs
- ObjectReferenceStack.cs
- UnsafeNativeMethods.cs
- TraceHandlerErrorFormatter.cs
- CroppedBitmap.cs
- OrderedDictionaryStateHelper.cs
- DataBindingCollection.cs
- Keyboard.cs
- WebPartsPersonalizationAuthorization.cs
- StrokeCollectionConverter.cs
- TypeCodeDomSerializer.cs
- ComboBoxRenderer.cs
- SizeFConverter.cs
- HttpResponseWrapper.cs
- DoubleLinkList.cs
- WebPartZoneBaseDesigner.cs
- CultureSpecificStringDictionary.cs
- FontDialog.cs
- HttpDictionary.cs
- SafeCryptContextHandle.cs
- NameNode.cs
- DefaultBindingPropertyAttribute.cs
- BooleanAnimationBase.cs
- SafeNativeMethods.cs
- ExpandedWrapper.cs
- GZipUtils.cs
- SystemWebCachingSectionGroup.cs
- JavaScriptString.cs
- cookiecontainer.cs
- WindowsFormsSynchronizationContext.cs
- MailSettingsSection.cs
- DataFormats.cs
- FillRuleValidation.cs
- CallTemplateAction.cs
- CommandDevice.cs
- OrderByQueryOptionExpression.cs
- VerificationAttribute.cs
- StartUpEventArgs.cs
- WebPartTransformerCollection.cs
- KeyGestureValueSerializer.cs
- ExceptQueryOperator.cs
- SettingsContext.cs
- Literal.cs
- AbstractSvcMapFileLoader.cs
- ZoneIdentityPermission.cs
- HtmlSelect.cs
- WebHttpBehavior.cs
- SpeechEvent.cs
- LogicalExpressionEditor.cs
- MenuItem.cs
- CatalogZoneDesigner.cs