Code:
/ DotNET / DotNET / 8.0 / untmp / Orcas / RTM / ndp / fx / src / xsp / System / Web / Extensions / Script / Serialization / JavaScriptString.cs / 1 / JavaScriptString.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Serialization { using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Text; internal class JavaScriptString { private string _s; private int _index; internal JavaScriptString(string s) { _s = s; } internal NullableGetNextNonEmptyChar() { while (_s.Length > _index) { char c = _s[_index++]; if (!Char.IsWhiteSpace(c)) { return c; } } return null; } internal Nullable MoveNext() { if (_s.Length > _index) { return _s[_index++]; } return null; } internal string MoveNext(int count) { if (_s.Length >= _index + count) { string result = _s.Substring(_index, count); _index += count; return result; } return null; } internal void MovePrev() { if (_index > 0) { _index--; } } internal void MovePrev(int count) { while (_index > 0 && count > 0) { _index--; count--; } } private static void AppendCharAsUnicode(StringBuilder builder, char c) { builder.Append("\\u"); builder.AppendFormat(CultureInfo.InvariantCulture, "{0:x4}", (int)c); } [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="Method would be more complex if split into separate pieces.")] internal static string QuoteString(string value) { StringBuilder b = null; if (String.IsNullOrEmpty(value)) { return String.Empty; } int startIndex = 0; int count = 0; for (int i = 0; i < value.Length; i++) { char c = value[i]; // Append the unhandled characters (that do not require special treament) // to the string builder when special characters are detected. if (c == '\r' || c == '\t' || c == '\"' || c == '\'' || c == '<' || c == '>' || c == '\\' || c == '\n' || c == '\b' || c == '\f' || c < ' ') { if (b == null) { b = new StringBuilder(value.Length + 5); } if (count > 0) { b.Append(value, startIndex, count); } startIndex = i+1; count = 0; } switch (c) { case '\r': b.Append("\\r"); break; case '\t': b.Append("\\t"); break; case '\"': b.Append("\\\""); break; case '\\': b.Append("\\\\"); break; case '\n': b.Append("\\n"); break; case '\b': b.Append("\\b"); break; case '\f': b.Append("\\f"); break; case '\'': case '>': case '<': AppendCharAsUnicode(b, c); break; default: if (c < ' ') { AppendCharAsUnicode(b, c); } else { count++; } break; } } if (b == null) { return value; } if (count > 0) { b.Append(value, startIndex, count); } return b.ToString(); } internal static string QuoteString(string value, bool addQuotes) { string s = QuoteString(value); if (addQuotes) { s = "\"" + s + "\""; } return s; } public override string ToString() { if (_s.Length > _index) { return _s.Substring(_index); } return String.Empty; } internal string GetDebugString(string message) { return message + " (" + _index + "): " + _s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
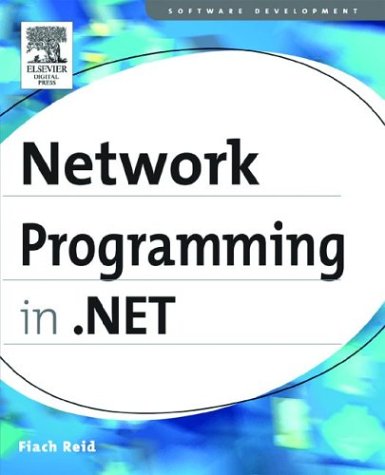
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateMachineSubscriptionManager.cs
- prefixendpointaddressmessagefiltertable.cs
- MessageContractExporter.cs
- ProfileSection.cs
- InvalidCastException.cs
- WebReferencesBuildProvider.cs
- NativeMethods.cs
- UrlMappingsSection.cs
- ExecutionContext.cs
- TextContainerHelper.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- SafeFileHandle.cs
- ConfigurationCollectionAttribute.cs
- ConstraintEnumerator.cs
- TriggerAction.cs
- KerberosSecurityTokenAuthenticator.cs
- OrderedDictionary.cs
- UIElement3D.cs
- OdbcException.cs
- Certificate.cs
- RepeatBehaviorConverter.cs
- ParenExpr.cs
- ActivityWithResultValueSerializer.cs
- PagesSection.cs
- WizardPanel.cs
- TextViewSelectionProcessor.cs
- StreamMarshaler.cs
- ConnectionStringsExpressionEditor.cs
- WindowsFormsHelpers.cs
- MaskInputRejectedEventArgs.cs
- wgx_exports.cs
- Binding.cs
- ColumnMapCopier.cs
- StringValidatorAttribute.cs
- ScrollItemProviderWrapper.cs
- SqlNotificationRequest.cs
- RtfToken.cs
- KnownIds.cs
- UpdateCommand.cs
- ObjectConverter.cs
- Int16Storage.cs
- TextFormatterHost.cs
- CachedFontFamily.cs
- TextParaClient.cs
- Asn1IntegerConverter.cs
- Int32Storage.cs
- CollectionViewGroup.cs
- SelectionPattern.cs
- DmlSqlGenerator.cs
- WriteFileContext.cs
- SpanIndex.cs
- RootProfilePropertySettingsCollection.cs
- EnumerableWrapperWeakToStrong.cs
- DataGridViewColumnStateChangedEventArgs.cs
- TextStore.cs
- DynamicDataResources.Designer.cs
- RemoteWebConfigurationHostStream.cs
- CompletionCallbackWrapper.cs
- VectorKeyFrameCollection.cs
- DateTimeConverter.cs
- MdImport.cs
- DrawingDrawingContext.cs
- ConstraintManager.cs
- Encoding.cs
- ProcessHostMapPath.cs
- VectorAnimationBase.cs
- BitmapCodecInfoInternal.cs
- ProviderConnectionPointCollection.cs
- ImageInfo.cs
- EntitySqlQueryCacheKey.cs
- InnerItemCollectionView.cs
- SqlInternalConnectionSmi.cs
- ClearTypeHintValidation.cs
- ListViewGroupConverter.cs
- FixedBufferAttribute.cs
- ThreadExceptionEvent.cs
- InvalidComObjectException.cs
- MessageBodyMemberAttribute.cs
- ClientBuildManagerCallback.cs
- DiscardableAttribute.cs
- StackSpiller.cs
- ReaderContextStackData.cs
- WrappedIUnknown.cs
- XmlSchemaType.cs
- ApplicationSecurityInfo.cs
- PropertyStore.cs
- BitmapSizeOptions.cs
- FormViewModeEventArgs.cs
- MatrixTransform3D.cs
- ScriptServiceAttribute.cs
- DataGridToolTip.cs
- EventHandlersStore.cs
- CompiledELinqQueryState.cs
- GPStream.cs
- AssemblyBuilder.cs
- ConnectionManagementElementCollection.cs
- TransactionContext.cs
- GreenMethods.cs
- exports.cs
- StdValidatorsAndConverters.cs