Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / NamedPipeWorkerProcess.cs / 1 / NamedPipeWorkerProcess.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; using System.ServiceModel.Activation.Diagnostics; using EventLogCategory = System.ServiceModel.Diagnostics.EventLogCategory; using EventLogEventId = System.ServiceModel.Diagnostics.EventLogEventId; class NamedPipeWorkerProcess : WorkerProcess { protected override DuplicateContext DuplicateConnection(ListenerSessionConnection session) { bool success = false; IntPtr dupedPipe = IntPtr.Zero; try { dupedPipe = (IntPtr)session.Connection.DuplicateAndClose(this.ProcessId); success = true; } #pragma warning suppress 56500 // covered by FxCOP catch (Exception exception) { if (DiagnosticUtility.IsFatal(exception)) { throw; } // this normally happens if: // A) we don't have rights to duplicate handles to the WorkerProcess NativeErrorCode == 87 // B) we fail to duplicate handle because the WorkerProcess is exiting/exited NativeErrorCode == ??? // - in the self hosted case: report error to the client // - in the web hosted case: roundrobin to the next available WorkerProcess (if this WorkerProcess is down?) #if DEBUG if (exception is CommunicationException) { int errorCode = ((System.IO.PipeException)exception.InnerException).ErrorCode; Debug.Print("NamedPipeWorkerProcess.DuplicateConnection() failed duplicating pipe for processId: " + this.ProcessId + " errorCode:" + errorCode + " exception:" + exception.Message); } #endif if (DiagnosticUtility.ShouldTraceError) { ListenerTraceUtility.TraceEvent(TraceEventType.Error, TraceCode.MessageQueueDuplicatedPipe, this, exception); } } if (success) { if (DiagnosticUtility.ShouldTraceInformation) { ListenerTraceUtility.TraceEvent(TraceEventType.Information, TraceCode.MessageQueueDuplicatedPipe, this); } return new NamedPipeDuplicateContext(dupedPipe, session.Via, session.Data); } return null; } protected override void OnDispatchSuccess() { ListenerPerfCounters.IncrementConnectionsDispatchedNamedPipe(); } protected override TransportType TransportType { get { return TransportType.NamedPipe; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
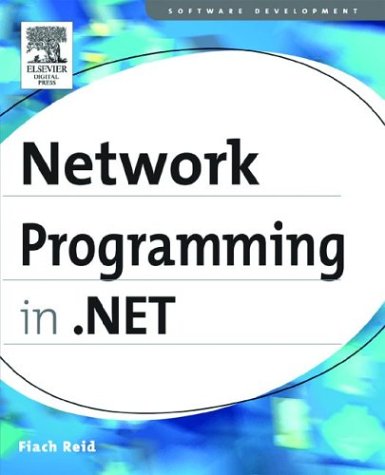
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsSysHeader.cs
- CodeExporter.cs
- login.cs
- DllNotFoundException.cs
- HttpDebugHandler.cs
- WebPartZoneBase.cs
- DiagnosticsConfigurationHandler.cs
- ProcessThread.cs
- RouteParametersHelper.cs
- Window.cs
- CodeStatement.cs
- OutgoingWebRequestContext.cs
- JsonStringDataContract.cs
- OwnerDrawPropertyBag.cs
- CollectionCodeDomSerializer.cs
- VoiceObjectToken.cs
- ToolBarButton.cs
- XmlSchemaAttributeGroup.cs
- HttpWebRequest.cs
- ClientType.cs
- DataGridViewToolTip.cs
- UnsafeNativeMethods.cs
- Rule.cs
- ProxyWebPart.cs
- InteropTrackingRecord.cs
- DocumentGrid.cs
- CallSiteOps.cs
- GlyphCache.cs
- dbenumerator.cs
- EnumMemberAttribute.cs
- CollectionExtensions.cs
- ToolStripRenderer.cs
- HtmlButton.cs
- SqlClientFactory.cs
- ConfigPathUtility.cs
- DictionaryBase.cs
- rsa.cs
- CodeExpressionStatement.cs
- ErrorWrapper.cs
- InstanceHandleReference.cs
- CngAlgorithmGroup.cs
- MonitoringDescriptionAttribute.cs
- FixedMaxHeap.cs
- TemplateBamlTreeBuilder.cs
- FragmentNavigationEventArgs.cs
- FilteredXmlReader.cs
- Label.cs
- ProcessHostFactoryHelper.cs
- MemberPath.cs
- XomlDesignerLoader.cs
- System.Data.OracleClient_BID.cs
- SourceFileBuildProvider.cs
- AccessDataSource.cs
- ProgressChangedEventArgs.cs
- SystemResourceKey.cs
- CollectionViewGroupRoot.cs
- LockRenewalTask.cs
- DeclarativeCatalogPart.cs
- SecurityUniqueId.cs
- ImageListImageEditor.cs
- Listbox.cs
- __Error.cs
- FontWeights.cs
- IncrementalReadDecoders.cs
- ShortcutKeysEditor.cs
- EncoderParameters.cs
- SecurityIdentifierElementCollection.cs
- SQLDoubleStorage.cs
- StrokeIntersection.cs
- HitTestResult.cs
- ArrayWithOffset.cs
- GeometryDrawing.cs
- Style.cs
- GPPOINT.cs
- PointUtil.cs
- SelectionProviderWrapper.cs
- CursorInteropHelper.cs
- XslException.cs
- ConnectionProviderAttribute.cs
- HotCommands.cs
- MobileListItem.cs
- DbUpdateCommandTree.cs
- AppDomainFactory.cs
- FontDialog.cs
- WaitForChangedResult.cs
- CodeMemberMethod.cs
- IteratorDescriptor.cs
- XhtmlBasicListAdapter.cs
- ReferenceEqualityComparer.cs
- PhysicalAddress.cs
- DivideByZeroException.cs
- SurrogateEncoder.cs
- ContractListAdapter.cs
- PropertyIDSet.cs
- MatrixKeyFrameCollection.cs
- Privilege.cs
- XmlDomTextWriter.cs
- XmlSchemaAny.cs
- DesignOnlyAttribute.cs
- TransformValueSerializer.cs