Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ReferenceEqualityComparer.cs / 1305376 / ReferenceEqualityComparer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class that can compare objects for reference equality. // //--------------------------------------------------------------------- //// #define NON_GENERIC_AVAILABLE #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { #region Namespaces. using System.Collections.Generic; using System.Diagnostics; using System.Linq.Expressions; using System.Text; using System.Collections; #endregion Namespaces. ///Equality comparer implementation that uses reference equality. internal class ReferenceEqualityComparer : IEqualityComparer { #region Private fields. #if NON_GENERIC_AVAILABLE ///Singleton instance (non-generic, as opposed to the one in ReferenceEqualityComparer<T>. private static ReferenceEqualityComparer nonGenericInstance; #endif #endregion Private fields. #region Constructors. ///Initializes a new protected ReferenceEqualityComparer() { } #endregion Constructors. #region Properties. ///instance. Determines whether two objects are the same. /// First object to compare. /// Second object to compare. ///true if both are the same; false otherwise. bool IEqualityComparer.Equals(object x, object y) { return object.ReferenceEquals(x, y); } ///Serves as hashing function for collections. /// Object to hash. ////// Hash code for the object; shouldn't change through the lifetime /// of int IEqualityComparer.GetHashCode(object obj) { if (obj == null) { return 0; } return obj.GetHashCode(); } #if NON_GENERIC_AVAILABLE ///. /// Singleton instance (non-generic, as opposed to the one in ReferenceEqualityComparer<T>. internal ReferenceEqualityComparer NonGenericInstance { get { if (nonGenericInstance == null) { ReferenceEqualityComparer comparer = new ReferenceEqualityComparer(); System.Threading.Interlocked.CompareExchange(ref nonGenericInstance, comparer, null); } return nonGenericInstance; } } #endif #endregion Properties. } ////// Use this class to compare objects by reference in collections such as /// dictionary or hashsets. /// ///Type of objects to compare. ////// Typically accesses statically as eg /// ReferenceEqualityComparer<Expression>.Instance. /// internal sealed class ReferenceEqualityComparer: ReferenceEqualityComparer, IEqualityComparer { #region Private fields. /// Single instance per 'T' for comparison. private static ReferenceEqualityComparerinstance; #endregion Private fields. #region Constructors. /// Initializes a new private ReferenceEqualityComparer() : base() { } #endregion Constructors. #region Properties. ///instance. Returns a singleton instance for this comparer type. internal static ReferenceEqualityComparerInstance { get { if (instance == null) { Debug.Assert(!typeof(T).IsValueType, "!typeof(T).IsValueType -- can't use reference equality in a meaningful way with value types"); ReferenceEqualityComparer newInstance = new ReferenceEqualityComparer (); System.Threading.Interlocked.CompareExchange(ref instance, newInstance, null); } return instance; } } #endregion Properties. #region Methods. /// Determines whether two objects are the same. /// First object to compare. /// Second object to compare. ///true if both are the same; false otherwise. public bool Equals(T x, T y) { return object.ReferenceEquals(x, y); } ///Serves as hashing function for collections. /// Object to hash. ////// Hash code for the object; shouldn't change through the lifetime /// of public int GetHashCode(T obj) { if (obj == null) { return 0; } return obj.GetHashCode(); } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.. ///
Link Menu
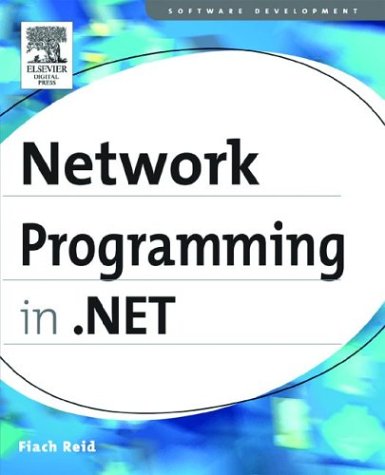
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OperationDescription.cs
- SvcMapFile.cs
- RegexCharClass.cs
- TrackingValidationObjectDictionary.cs
- TcpSocketManager.cs
- WmlPageAdapter.cs
- SerializationObjectManager.cs
- Baml6Assembly.cs
- HelpInfo.cs
- PageHandlerFactory.cs
- MaskedTextBox.cs
- BuildResult.cs
- NativeCppClassAttribute.cs
- RoleGroup.cs
- ClientBuildManager.cs
- PropertyGroupDescription.cs
- FormatConvertedBitmap.cs
- DesignOnlyAttribute.cs
- MenuRendererStandards.cs
- Query.cs
- CodeAttributeDeclarationCollection.cs
- TypeExtension.cs
- HtmlWindowCollection.cs
- MessageBox.cs
- UnsafeNativeMethodsCLR.cs
- OpenTypeLayout.cs
- XmlSerializerAssemblyAttribute.cs
- SourceLineInfo.cs
- PolyBezierSegmentFigureLogic.cs
- InProcStateClientManager.cs
- SiteMapDataSource.cs
- RecordBuilder.cs
- KnownTypesHelper.cs
- ProtocolsSection.cs
- ZoneLinkButton.cs
- XmlSchemaSimpleContentRestriction.cs
- Enlistment.cs
- MouseEventArgs.cs
- ImpersonationOption.cs
- XmlSchemaAll.cs
- ColumnCollection.cs
- SQLByteStorage.cs
- EntitySqlQueryState.cs
- ServiceOperationListItem.cs
- XmlValidatingReaderImpl.cs
- TextEditorLists.cs
- OperatingSystem.cs
- BindingCollection.cs
- HealthMonitoringSectionHelper.cs
- AssertSection.cs
- ApplicationBuildProvider.cs
- WebPartsSection.cs
- LostFocusEventManager.cs
- MailAddressCollection.cs
- ItemsPanelTemplate.cs
- EventHandlersStore.cs
- DbFunctionCommandTree.cs
- WebDisplayNameAttribute.cs
- XDeferredAxisSource.cs
- DurationConverter.cs
- DataIdProcessor.cs
- ContractType.cs
- ServicePrincipalNameElement.cs
- MenuRendererStandards.cs
- ForeignKeyConstraint.cs
- WindowsRichEditRange.cs
- FixedBufferAttribute.cs
- SizeChangedInfo.cs
- XmlHierarchicalDataSourceView.cs
- HotSpotCollection.cs
- CodeDirectionExpression.cs
- EdmComplexPropertyAttribute.cs
- NavigationFailedEventArgs.cs
- SettingsProperty.cs
- MarkupExtensionReturnTypeAttribute.cs
- DeploymentSection.cs
- SchemaExporter.cs
- DataGridViewColumn.cs
- srgsitem.cs
- XsdCachingReader.cs
- XomlCompilerHelpers.cs
- ByteStorage.cs
- OdbcEnvironment.cs
- FileUtil.cs
- ListViewEditEventArgs.cs
- LogReservationCollection.cs
- ThreadStateException.cs
- AsymmetricKeyExchangeFormatter.cs
- TableAdapterManagerMethodGenerator.cs
- Int16Animation.cs
- CounterSample.cs
- IntranetCredentialPolicy.cs
- ParallelTimeline.cs
- FolderBrowserDialog.cs
- CalendarButton.cs
- QueryOutputWriter.cs
- UTF8Encoding.cs
- SingleKeyFrameCollection.cs
- ReadOnlyNameValueCollection.cs
- Vector.cs