Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Compilation / WCFModel / SvcMapFile.cs / 1 / SvcMapFile.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// The SvcMapFile is responsible for serializing/deserializing the information in a .svcmap file. /// [System.Xml.Serialization.XmlRootAttribute(Namespace = SvcMapFile.NamespaceUri, ElementName = "ReferenceGroup")] #if WEB_EXTENSIONS_CODE internal class SvcMapFile #else [CLSCompliant(true)] public class SvcMapFile #endif { ////// Namespace for the svcmap file schema /// public const string NamespaceUri = "urn:schemas-microsoft-com:xml-wcfservicemap"; // GUID string, to track the reference group when the name is changed private string m_ID; // Metadata Source List private Listm_MetadataSourceList; // Metadata Item list private List m_MetadataList; // Extension File List private List m_ExtensionFileList; // Generator options private ClientOptions m_ClientOptions; // Errors encountered while loading this file private IEnumerable loadErrors; /// /// Constructor /// public SvcMapFile() { m_ID = Guid.NewGuid().ToString(); } ////// Proxy options /// ////// [System.Xml.Serialization.XmlElement(Order = 0)] public ClientOptions ClientOptions { get { if (m_ClientOptions == null) { m_ClientOptions = new ClientOptions(); } return m_ClientOptions; } set { if (value == null) { throw new ArgumentNullException("value"); } m_ClientOptions = value; } } /// /// Extension item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "Extensions", Order = 3)] [System.Xml.Serialization.XmlArrayItem("ExtensionFile", typeof(ExtensionFile))] public List Extensions { get { if (m_ExtensionFileList == null) { m_ExtensionFileList = new List (); } return m_ExtensionFileList; } } /// /// Unique ID of the reference group. It is a GUID string. /// ////// [System.Xml.Serialization.XmlAttribute()] public string ID { get { return m_ID; } set { m_ID = value; } } /// /// Metadata item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "Metadata", Order = 2)] [System.Xml.Serialization.XmlArrayItem("MetadataFile", typeof(MetadataFile))] public List MetadataList { get { if (m_MetadataList == null) { m_MetadataList = new List (); } return m_MetadataList; } } /// /// Metadata source item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "MetadataSources", Order = 1)] [System.Xml.Serialization.XmlArrayItem("MetadataSource", typeof(MetadataSource))] public List MetadataSourceList { get { if (m_MetadataSourceList == null) { m_MetadataSourceList = new List (); } return m_MetadataSourceList; } } /// /// Errors encountered during load /// [System.Xml.Serialization.XmlIgnore()] public IEnumerableLoadErrors { get { List errors = new List (); if (loadErrors != null) { errors.AddRange(loadErrors); } return errors; } } internal void SetLoadErrors(IEnumerable loadErrors) { this.loadErrors = loadErrors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// The SvcMapFile is responsible for serializing/deserializing the information in a .svcmap file. /// [System.Xml.Serialization.XmlRootAttribute(Namespace = SvcMapFile.NamespaceUri, ElementName = "ReferenceGroup")] #if WEB_EXTENSIONS_CODE internal class SvcMapFile #else [CLSCompliant(true)] public class SvcMapFile #endif { ////// Namespace for the svcmap file schema /// public const string NamespaceUri = "urn:schemas-microsoft-com:xml-wcfservicemap"; // GUID string, to track the reference group when the name is changed private string m_ID; // Metadata Source List private Listm_MetadataSourceList; // Metadata Item list private List m_MetadataList; // Extension File List private List m_ExtensionFileList; // Generator options private ClientOptions m_ClientOptions; // Errors encountered while loading this file private IEnumerable loadErrors; /// /// Constructor /// public SvcMapFile() { m_ID = Guid.NewGuid().ToString(); } ////// Proxy options /// ////// [System.Xml.Serialization.XmlElement(Order = 0)] public ClientOptions ClientOptions { get { if (m_ClientOptions == null) { m_ClientOptions = new ClientOptions(); } return m_ClientOptions; } set { if (value == null) { throw new ArgumentNullException("value"); } m_ClientOptions = value; } } /// /// Extension item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "Extensions", Order = 3)] [System.Xml.Serialization.XmlArrayItem("ExtensionFile", typeof(ExtensionFile))] public List Extensions { get { if (m_ExtensionFileList == null) { m_ExtensionFileList = new List (); } return m_ExtensionFileList; } } /// /// Unique ID of the reference group. It is a GUID string. /// ////// [System.Xml.Serialization.XmlAttribute()] public string ID { get { return m_ID; } set { m_ID = value; } } /// /// Metadata item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "Metadata", Order = 2)] [System.Xml.Serialization.XmlArrayItem("MetadataFile", typeof(MetadataFile))] public List MetadataList { get { if (m_MetadataList == null) { m_MetadataList = new List (); } return m_MetadataList; } } /// /// Metadata source item list /// ////// [System.Xml.Serialization.XmlArray(ElementName = "MetadataSources", Order = 1)] [System.Xml.Serialization.XmlArrayItem("MetadataSource", typeof(MetadataSource))] public List MetadataSourceList { get { if (m_MetadataSourceList == null) { m_MetadataSourceList = new List (); } return m_MetadataSourceList; } } /// /// Errors encountered during load /// [System.Xml.Serialization.XmlIgnore()] public IEnumerableLoadErrors { get { List errors = new List (); if (loadErrors != null) { errors.AddRange(loadErrors); } return errors; } } internal void SetLoadErrors(IEnumerable loadErrors) { this.loadErrors = loadErrors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
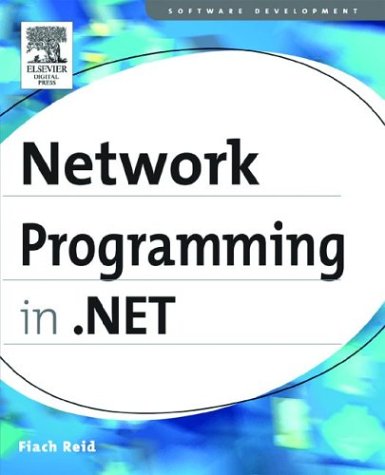
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Misc.cs
- IISMapPath.cs
- EmbossBitmapEffect.cs
- TdsParserSafeHandles.cs
- DocumentGridPage.cs
- AlignmentYValidation.cs
- DynamicValidator.cs
- ClientBuildManagerCallback.cs
- WindowsGraphicsWrapper.cs
- XhtmlBasicSelectionListAdapter.cs
- SafeEventLogReadHandle.cs
- SqlSupersetValidator.cs
- ImageListStreamer.cs
- HtmlGenericControl.cs
- NavigationExpr.cs
- _AutoWebProxyScriptEngine.cs
- PersonalizablePropertyEntry.cs
- SecurityVerifiedMessage.cs
- SqlServices.cs
- EmptyEnumerable.cs
- DefaultBinder.cs
- DeviceContext.cs
- WaitForChangedResult.cs
- ArrayTypeMismatchException.cs
- RelatedEnd.cs
- GenericRootAutomationPeer.cs
- webclient.cs
- HtmlFormAdapter.cs
- SemanticBasicElement.cs
- WorkflowExecutor.cs
- TableRowCollection.cs
- HttpRawResponse.cs
- TimeoutValidationAttribute.cs
- IntSecurity.cs
- ItemsControlAutomationPeer.cs
- PolyLineSegmentFigureLogic.cs
- FromRequest.cs
- CodeIndexerExpression.cs
- Tracking.cs
- SQLInt64.cs
- StylusPointPropertyId.cs
- ResourceCategoryAttribute.cs
- Soap12ProtocolReflector.cs
- MetadataWorkspace.cs
- DataViewListener.cs
- NonClientArea.cs
- HostingEnvironmentException.cs
- Matrix.cs
- HijriCalendar.cs
- SecurityRuntime.cs
- ComboBoxItem.cs
- PrivilegedConfigurationManager.cs
- GridEntryCollection.cs
- GridViewDeletedEventArgs.cs
- BuildTopDownAttribute.cs
- AliasGenerator.cs
- RSAPKCS1SignatureFormatter.cs
- HttpModulesSection.cs
- UInt64Converter.cs
- TextMessageEncodingBindingElement.cs
- ExtensionQuery.cs
- String.cs
- DeadCharTextComposition.cs
- BindingList.cs
- XmlSerializerAssemblyAttribute.cs
- SpellerError.cs
- ApplicationDirectoryMembershipCondition.cs
- LinearGradientBrush.cs
- ReachUIElementCollectionSerializerAsync.cs
- CompleteWizardStep.cs
- exports.cs
- MimeBasePart.cs
- _NtlmClient.cs
- WebServiceAttribute.cs
- TextLine.cs
- ListItemConverter.cs
- InputScopeNameConverter.cs
- TransformPattern.cs
- BamlBinaryReader.cs
- Int32CollectionConverter.cs
- DataGridViewCellPaintingEventArgs.cs
- DtrList.cs
- latinshape.cs
- ForwardPositionQuery.cs
- CodeBlockBuilder.cs
- sqlmetadatafactory.cs
- BufferBuilder.cs
- RelationshipWrapper.cs
- PerformanceCounter.cs
- StructuralObject.cs
- HashCodeCombiner.cs
- ReaderWriterLock.cs
- ConnectionPoint.cs
- WorkflowViewManager.cs
- LocalizableAttribute.cs
- Helpers.cs
- XmlReader.cs
- MsmqHostedTransportManager.cs
- ShellProvider.cs
- ProviderConnectionPointCollection.cs