Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / srgsitem.cs / 1 / srgsitem.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item"]/*' /> [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] [DebuggerTypeProxy (typeof (SrgsItemDebugDisplay))] public class SrgsItem : SrgsElement, IItem { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item1"]/*' /> public SrgsItem () { _elements = new SrgsElementList (); } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item2"]/*' /> public SrgsItem (string text) : this () { Helpers.ThrowIfEmptyOrNull (text, "text"); _elements.Add (new SrgsText (text)); } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item8"]/*' /> public SrgsItem (params SrgsElement [] elements) : this () { Helpers.ThrowIfNull (elements, "elements"); for (int iElement = 0; iElement < elements.Length; iElement++) { if (elements [iElement] == null) { throw new ArgumentNullException ("elements", SR.Get (SRID.ParamsEntryNullIllegal)); } _elements.Add (elements [iElement]); } } ////// TODOC /// /// public SrgsItem (int repeatCount) : this () { SetRepeat (repeatCount); } ////// TODOC /// /// /// public SrgsItem (int min, int max) : this () { SetRepeat (min, max); } //overloads with setting the repeat. ////// TODOC /// /// /// /// public SrgsItem (int min, int max, string text) : this (text) { SetRepeat (min, max); } ////// TODOC /// /// /// /// public SrgsItem (int min, int max, params SrgsElement [] elements) : this (elements) { SetRepeat (min, max); } #endregion //******************************************************************** // // Public Method // //******************************************************************* #region Public Method ////// TODOC /// /// public void SetRepeat (int count) { // Negative values are not allowed if (count < 0 || count > 255) { throw new ArgumentOutOfRangeException ("count"); } _minRepeat = _maxRepeat = count; } ////// TODOC /// /// /// public void SetRepeat (int minRepeat, int maxRepeat) { // Negative values are not allowed if (minRepeat < 0 || minRepeat > 255) { throw new ArgumentOutOfRangeException ("minRepeat", SR.Get (SRID.InvalidMinRepeat, minRepeat)); } if (maxRepeat != int.MaxValue && (maxRepeat < 0 || maxRepeat > 255)) { throw new ArgumentOutOfRangeException ("maxRepeat", SR.Get (SRID.InvalidMinRepeat, maxRepeat)); } // Max be greater or equal to min if (minRepeat > maxRepeat) { throw new ArgumentException (SR.Get (SRID.MinGreaterThanMax)); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } ////// TODOC /// /// public void Add (SrgsElement element) { Helpers.ThrowIfNull (element, "element"); Elements.Add (element); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region Public Properties /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Elements"]/*' /> public CollectionElements { get { return _elements; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.RepeatProbability"]/*' /> // The probability that this item will be repeated. public float RepeatProbability { get { return _repeatProbability; } set { if (value < 0.0f || value > 1.0f) { throw new ArgumentOutOfRangeException ("value", SR.Get (SRID.InvalidRepeatProbability, value)); } _repeatProbability = value; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.MinRepeat"]/*' /> // The minimum number of occurrences this item can/must be repeated. public int MinRepeat { get { return _minRepeat == NotSet ? 1 : _minRepeat; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.MaxRepeat"]/*' /> // The maximum number of occurrences this item can/must be repeated. public int MaxRepeat { get { return _maxRepeat == NotSet ? 1 : _maxRepeat; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Weight"]/*' /> public float Weight { get { return _weight; } set { if (value <= 0.0f) { throw new ArgumentOutOfRangeException ("value", SR.Get (SRID.InvalidWeight, value)); } _weight = value; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods /// /// Write the XML fragment describing the object. /// /// internal override void WriteSrgs (XmlWriter writer) { // Write- writer.WriteStartElement ("item"); if (!_weight.Equals (1.0f)) { writer.WriteAttributeString ("weight", _weight.ToString ("0.########", CultureInfo.InvariantCulture)); } if (!_repeatProbability.Equals (0.5f)) { writer.WriteAttributeString ("repeat-prob", _repeatProbability.ToString ("0.########", CultureInfo.InvariantCulture)); } if (_minRepeat == _maxRepeat) { // could be because both value are NotSet of equal if (_minRepeat != NotSet) { writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}", _minRepeat)); } } else if (_maxRepeat == int.MaxValue || _maxRepeat == NotSet) { // MinValue Set but not Max Value writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}-", _minRepeat)); } else { // Max Value Set and maybe MinValue int minRepeat = _minRepeat == NotSet ? 1 : _minRepeat; writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}-{1}", minRepeat, _maxRepeat)); } // Write
- body and footer. Type previousElementType = null; foreach (SrgsElement element in _elements) { // Insert space between consecutive SrgsText _elements. Type elementType = element.GetType (); if ((elementType == typeof (SrgsText)) && (elementType == previousElementType)) { writer.WriteString (" "); } previousElementType = elementType; element.WriteSrgs (writer); } writer.WriteEndElement (); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder (); if (_elements.Count > 7) { sb.Append ("SrgsItem Count = "); sb.Append (_elements.Count.ToString (CultureInfo.InstalledUICulture)); } else { if (_minRepeat != _maxRepeat || _maxRepeat != NotSet) { sb.Append ("["); if (_minRepeat == _maxRepeat) { sb.Append (_minRepeat.ToString (CultureInfo.InvariantCulture)); } else if (_maxRepeat == int.MaxValue || _maxRepeat == NotSet) { // MinValue Set but not Max Value sb.Append (string.Format (CultureInfo.InvariantCulture, "{0},-", _minRepeat)); } else { // Max Value Set and maybe MinValue int minRepeat = _minRepeat == NotSet ? 1 : _minRepeat; sb.Append (string.Format (CultureInfo.InvariantCulture, "{0},{1}", minRepeat, _maxRepeat)); } sb.Append ("] "); } bool first = true; foreach (SrgsElement element in _elements) { if (!first) { sb.Append (" "); } sb.Append ("{"); sb.Append (element.DebuggerDisplayString ()); sb.Append ("}"); first = false; } } return sb.ToString (); } #endregion //******************************************************************* // // Protected Properties // //******************************************************************* #region Protected Properties ///
/// Allows the Srgs Element base class to implement /// features requiring recursion in the elements tree. /// ///internal override SrgsElement [] Children { get { SrgsElement [] elements = new SrgsElement [_elements.Count]; int i = 0; foreach (SrgsElement element in _elements) { elements [i++] = element; } return elements; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************** #region Private Methods #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private float _weight = 1.0f; private float _repeatProbability = 0.5f; private int _minRepeat = NotSet; private int _maxRepeat = NotSet; private SrgsElementList _elements; private const int NotSet = -1; #endregion //******************************************************************** // // Private Types // //******************************************************************* #region Private Types // Used by the debbugger display attribute internal class SrgsItemDebugDisplay { public SrgsItemDebugDisplay (SrgsItem item) { _weight = item._weight; _repeatProbability = item._repeatProbability; _minRepeat = item._minRepeat; _maxRepeat = item._maxRepeat; _elements = item._elements; } public object Weigth { get { return _weight; } } public object MinRepeat { get { return _minRepeat; } } public object MaxRepeat { get { return _maxRepeat; } } public object RepeatProbability { get { return _repeatProbability; } } public object Count { get { return _elements.Count; } } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsElement [] AKeys { get { SrgsElement [] elements = new SrgsElement [_elements.Count]; for (int i = 0; i < _elements.Count; i++) { elements [i] = _elements [i]; } return elements; } } private float _weight = 1.0f; private float _repeatProbability = 0.5f; private int _minRepeat = NotSet; private int _maxRepeat = NotSet; private SrgsElementList _elements; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; using System.Speech.Internal.SrgsParser; using System.Text; using System.Xml; namespace System.Speech.Recognition.SrgsGrammar { /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item"]/*' /> [Serializable] [DebuggerDisplay ("{DebuggerDisplayString ()}")] [DebuggerTypeProxy (typeof (SrgsItemDebugDisplay))] public class SrgsItem : SrgsElement, IItem { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item1"]/*' /> public SrgsItem () { _elements = new SrgsElementList (); } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item2"]/*' /> public SrgsItem (string text) : this () { Helpers.ThrowIfEmptyOrNull (text, "text"); _elements.Add (new SrgsText (text)); } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Item8"]/*' /> public SrgsItem (params SrgsElement [] elements) : this () { Helpers.ThrowIfNull (elements, "elements"); for (int iElement = 0; iElement < elements.Length; iElement++) { if (elements [iElement] == null) { throw new ArgumentNullException ("elements", SR.Get (SRID.ParamsEntryNullIllegal)); } _elements.Add (elements [iElement]); } } ////// TODOC /// /// public SrgsItem (int repeatCount) : this () { SetRepeat (repeatCount); } ////// TODOC /// /// /// public SrgsItem (int min, int max) : this () { SetRepeat (min, max); } //overloads with setting the repeat. ////// TODOC /// /// /// /// public SrgsItem (int min, int max, string text) : this (text) { SetRepeat (min, max); } ////// TODOC /// /// /// /// public SrgsItem (int min, int max, params SrgsElement [] elements) : this (elements) { SetRepeat (min, max); } #endregion //******************************************************************** // // Public Method // //******************************************************************* #region Public Method ////// TODOC /// /// public void SetRepeat (int count) { // Negative values are not allowed if (count < 0 || count > 255) { throw new ArgumentOutOfRangeException ("count"); } _minRepeat = _maxRepeat = count; } ////// TODOC /// /// /// public void SetRepeat (int minRepeat, int maxRepeat) { // Negative values are not allowed if (minRepeat < 0 || minRepeat > 255) { throw new ArgumentOutOfRangeException ("minRepeat", SR.Get (SRID.InvalidMinRepeat, minRepeat)); } if (maxRepeat != int.MaxValue && (maxRepeat < 0 || maxRepeat > 255)) { throw new ArgumentOutOfRangeException ("maxRepeat", SR.Get (SRID.InvalidMinRepeat, maxRepeat)); } // Max be greater or equal to min if (minRepeat > maxRepeat) { throw new ArgumentException (SR.Get (SRID.MinGreaterThanMax)); } _minRepeat = minRepeat; _maxRepeat = maxRepeat; } ////// TODOC /// /// public void Add (SrgsElement element) { Helpers.ThrowIfNull (element, "element"); Elements.Add (element); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region Public Properties /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Elements"]/*' /> public CollectionElements { get { return _elements; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.RepeatProbability"]/*' /> // The probability that this item will be repeated. public float RepeatProbability { get { return _repeatProbability; } set { if (value < 0.0f || value > 1.0f) { throw new ArgumentOutOfRangeException ("value", SR.Get (SRID.InvalidRepeatProbability, value)); } _repeatProbability = value; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.MinRepeat"]/*' /> // The minimum number of occurrences this item can/must be repeated. public int MinRepeat { get { return _minRepeat == NotSet ? 1 : _minRepeat; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.MaxRepeat"]/*' /> // The maximum number of occurrences this item can/must be repeated. public int MaxRepeat { get { return _maxRepeat == NotSet ? 1 : _maxRepeat; } } /// TODOC <_include file='doc\Item.uex' path='docs/doc[@for="Item.Weight"]/*' /> public float Weight { get { return _weight; } set { if (value <= 0.0f) { throw new ArgumentOutOfRangeException ("value", SR.Get (SRID.InvalidWeight, value)); } _weight = value; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods /// /// Write the XML fragment describing the object. /// /// internal override void WriteSrgs (XmlWriter writer) { // Write- writer.WriteStartElement ("item"); if (!_weight.Equals (1.0f)) { writer.WriteAttributeString ("weight", _weight.ToString ("0.########", CultureInfo.InvariantCulture)); } if (!_repeatProbability.Equals (0.5f)) { writer.WriteAttributeString ("repeat-prob", _repeatProbability.ToString ("0.########", CultureInfo.InvariantCulture)); } if (_minRepeat == _maxRepeat) { // could be because both value are NotSet of equal if (_minRepeat != NotSet) { writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}", _minRepeat)); } } else if (_maxRepeat == int.MaxValue || _maxRepeat == NotSet) { // MinValue Set but not Max Value writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}-", _minRepeat)); } else { // Max Value Set and maybe MinValue int minRepeat = _minRepeat == NotSet ? 1 : _minRepeat; writer.WriteAttributeString ("repeat", string.Format (CultureInfo.InvariantCulture, "{0}-{1}", minRepeat, _maxRepeat)); } // Write
- body and footer. Type previousElementType = null; foreach (SrgsElement element in _elements) { // Insert space between consecutive SrgsText _elements. Type elementType = element.GetType (); if ((elementType == typeof (SrgsText)) && (elementType == previousElementType)) { writer.WriteString (" "); } previousElementType = elementType; element.WriteSrgs (writer); } writer.WriteEndElement (); } internal override string DebuggerDisplayString () { StringBuilder sb = new StringBuilder (); if (_elements.Count > 7) { sb.Append ("SrgsItem Count = "); sb.Append (_elements.Count.ToString (CultureInfo.InstalledUICulture)); } else { if (_minRepeat != _maxRepeat || _maxRepeat != NotSet) { sb.Append ("["); if (_minRepeat == _maxRepeat) { sb.Append (_minRepeat.ToString (CultureInfo.InvariantCulture)); } else if (_maxRepeat == int.MaxValue || _maxRepeat == NotSet) { // MinValue Set but not Max Value sb.Append (string.Format (CultureInfo.InvariantCulture, "{0},-", _minRepeat)); } else { // Max Value Set and maybe MinValue int minRepeat = _minRepeat == NotSet ? 1 : _minRepeat; sb.Append (string.Format (CultureInfo.InvariantCulture, "{0},{1}", minRepeat, _maxRepeat)); } sb.Append ("] "); } bool first = true; foreach (SrgsElement element in _elements) { if (!first) { sb.Append (" "); } sb.Append ("{"); sb.Append (element.DebuggerDisplayString ()); sb.Append ("}"); first = false; } } return sb.ToString (); } #endregion //******************************************************************* // // Protected Properties // //******************************************************************* #region Protected Properties ///
/// Allows the Srgs Element base class to implement /// features requiring recursion in the elements tree. /// ///internal override SrgsElement [] Children { get { SrgsElement [] elements = new SrgsElement [_elements.Count]; int i = 0; foreach (SrgsElement element in _elements) { elements [i++] = element; } return elements; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************** #region Private Methods #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private float _weight = 1.0f; private float _repeatProbability = 0.5f; private int _minRepeat = NotSet; private int _maxRepeat = NotSet; private SrgsElementList _elements; private const int NotSet = -1; #endregion //******************************************************************** // // Private Types // //******************************************************************* #region Private Types // Used by the debbugger display attribute internal class SrgsItemDebugDisplay { public SrgsItemDebugDisplay (SrgsItem item) { _weight = item._weight; _repeatProbability = item._repeatProbability; _minRepeat = item._minRepeat; _maxRepeat = item._maxRepeat; _elements = item._elements; } public object Weigth { get { return _weight; } } public object MinRepeat { get { return _minRepeat; } } public object MaxRepeat { get { return _maxRepeat; } } public object RepeatProbability { get { return _repeatProbability; } } public object Count { get { return _elements.Count; } } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsElement [] AKeys { get { SrgsElement [] elements = new SrgsElement [_elements.Count]; for (int i = 0; i < _elements.Count; i++) { elements [i] = _elements [i]; } return elements; } } private float _weight = 1.0f; private float _repeatProbability = 0.5f; private int _minRepeat = NotSet; private int _maxRepeat = NotSet; private SrgsElementList _elements; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
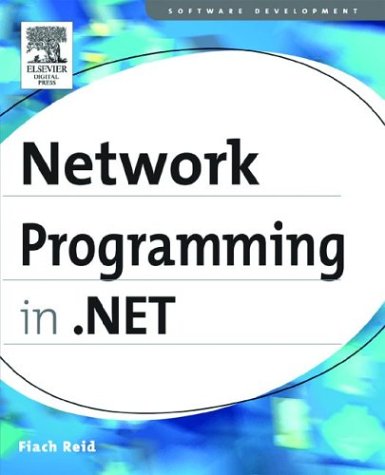
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceLockException.cs
- GatewayDefinition.cs
- VersionConverter.cs
- MD5.cs
- HtmlContainerControl.cs
- ImplicitInputBrush.cs
- CompilerGlobalScopeAttribute.cs
- MsmqHostedTransportConfiguration.cs
- DBConnection.cs
- DefinitionBase.cs
- CultureNotFoundException.cs
- BrowsableAttribute.cs
- WhitespaceRuleLookup.cs
- Material.cs
- WebPartChrome.cs
- XmlSchemaDatatype.cs
- SHA256Managed.cs
- TextContainer.cs
- ResourceAttributes.cs
- StrokeCollection.cs
- CngProvider.cs
- ADMembershipUser.cs
- Nodes.cs
- Separator.cs
- RecognizedWordUnit.cs
- ReadOnlyHierarchicalDataSourceView.cs
- TypeGeneratedEventArgs.cs
- SynchronizationContext.cs
- TimelineClockCollection.cs
- PngBitmapDecoder.cs
- XmlAttributes.cs
- AttachedPropertyMethodSelector.cs
- CapabilitiesSection.cs
- HttpListenerContext.cs
- StoragePropertyMapping.cs
- KeyFrames.cs
- CompilerError.cs
- SqlDependencyListener.cs
- ConnectionStringsExpressionBuilder.cs
- Control.cs
- DefaultHttpHandler.cs
- PopupEventArgs.cs
- DataTableTypeConverter.cs
- CursorInteropHelper.cs
- EventLogConfiguration.cs
- GeneralTransformGroup.cs
- propertytag.cs
- WpfSharedBamlSchemaContext.cs
- XPathNodeIterator.cs
- EventLogQuery.cs
- RuntimeIdentifierPropertyAttribute.cs
- complextypematerializer.cs
- __ComObject.cs
- WebServiceData.cs
- DataGridItemCollection.cs
- List.cs
- FollowerQueueCreator.cs
- TabletCollection.cs
- TabControl.cs
- XmlAtomicValue.cs
- HMAC.cs
- CompositeDataBoundControl.cs
- StrongNameIdentityPermission.cs
- DirectoryGroupQuery.cs
- ModuleBuilder.cs
- DependencyPropertyChangedEventArgs.cs
- SerializationAttributes.cs
- ThousandthOfEmRealPoints.cs
- SqlDataSourceQueryEditor.cs
- SwitchLevelAttribute.cs
- Line.cs
- LinqDataSourceUpdateEventArgs.cs
- ObjectQueryState.cs
- DoubleLink.cs
- BitmapCodecInfoInternal.cs
- Model3DGroup.cs
- HMACRIPEMD160.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SemaphoreSecurity.cs
- ChannelServices.cs
- OperationExecutionFault.cs
- NumericExpr.cs
- DesignOnlyAttribute.cs
- SemanticResolver.cs
- OracleParameterBinding.cs
- KerberosTicketHashIdentifierClause.cs
- WorkflowNamespace.cs
- Visual.cs
- LineBreak.cs
- ArglessEventHandlerProxy.cs
- DataSysAttribute.cs
- EncodedStreamFactory.cs
- RawMouseInputReport.cs
- Emitter.cs
- _NestedSingleAsyncResult.cs
- ErrorTableItemStyle.cs
- MsmqActivation.cs
- ParallelTimeline.cs
- DesignRelation.cs
- linebase.cs