Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / Nodes.cs / 2 / Nodes.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// A Node describes a node in a query tree. Each node has an operator, and /// a list of zero or more children of that operator. /// internal class Node { #region private state private int m_id; private Listm_children; private Op m_op; private NodeInfo m_nodeInfo; #endregion #region constructors /// /// Basic constructor. /// /// NEVER call this routine directly - you should always use the Command.CreateNode /// factory methods. /// /// id for the node /// The operator /// List of child nodes internal Node(int nodeId, Op op, Listchildren) { m_id = nodeId; m_op = op; m_children = children; } /// /// This routine is only used for building up rule patterns. /// NEVER use this routine for building up nodes in a user command tree. /// /// /// internal Node(Op op, params Node[] children) : this(-1, op, new List(children)) { } #endregion #region public properties and methods #if DEBUG internal int Id { get { return m_id; } } #endif /// /// Get the list of children /// internal ListChildren { get { return m_children; } } /// /// Gets or sets the node's operator /// internal Op Op { get { return m_op; } set { m_op = value; } } ////// Simpler (?) getter/setter routines /// internal Node Child0 { get { return m_children[0]; } set { m_children[0] = value; } } ////// Do I have a zeroth child? /// internal bool HasChild0 { get { return m_children.Count > 0; } } ////// Get/set first child /// internal Node Child1 { get { return m_children[1]; } set { m_children[1] = value; } } ////// Do I have a child1? /// internal bool HasChild1 { get { return m_children.Count > 1; } } ////// get/set second child /// internal Node Child2 { get { return m_children[2]; } set { m_children[2] = value; } } ////// Do I have a child2 (third child really) /// internal bool HasChild2 { get { return m_children.Count > 2; } } #region equivalence functions ////// Is this subtree equivalent to another subtree /// /// ///internal bool IsEquivalent(Node other) { if (this.Children.Count != other.Children.Count) { return false; } bool? opEquivalent = this.Op.IsEquivalent(other.Op); if (opEquivalent != true) { return false; } for (int i = 0; i < this.Children.Count; i++) { if (!this.Children[i].IsEquivalent(other.Children[i])) { return false; } } return true; } #endregion #region NodeInfo functions /// /// Get the nodeInfo for a node. Initializes it, if it has not yet been initialized /// /// Current command object ///NodeInfo for this node internal NodeInfo GetNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } return m_nodeInfo; } ////// Gets the "extended" nodeinfo for a node; if it has not yet been initialized, then it will be /// /// current command object ///extended nodeinfo for this node internal ExtendedNodeInfo GetExtendedNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } ExtendedNodeInfo extendedNodeInfo = m_nodeInfo as ExtendedNodeInfo; Debug.Assert(extendedNodeInfo != null); return extendedNodeInfo; } private void InitializeNodeInfo(Command command) { if (this.Op.IsRelOp || this.Op.IsPhysicalOp) { m_nodeInfo = new ExtendedNodeInfo(command); } else { m_nodeInfo = new NodeInfo(command); } command.RecomputeNodeInfo(this); } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// A Node describes a node in a query tree. Each node has an operator, and /// a list of zero or more children of that operator. /// internal class Node { #region private state private int m_id; private Listm_children; private Op m_op; private NodeInfo m_nodeInfo; #endregion #region constructors /// /// Basic constructor. /// /// NEVER call this routine directly - you should always use the Command.CreateNode /// factory methods. /// /// id for the node /// The operator /// List of child nodes internal Node(int nodeId, Op op, Listchildren) { m_id = nodeId; m_op = op; m_children = children; } /// /// This routine is only used for building up rule patterns. /// NEVER use this routine for building up nodes in a user command tree. /// /// /// internal Node(Op op, params Node[] children) : this(-1, op, new List(children)) { } #endregion #region public properties and methods #if DEBUG internal int Id { get { return m_id; } } #endif /// /// Get the list of children /// internal ListChildren { get { return m_children; } } /// /// Gets or sets the node's operator /// internal Op Op { get { return m_op; } set { m_op = value; } } ////// Simpler (?) getter/setter routines /// internal Node Child0 { get { return m_children[0]; } set { m_children[0] = value; } } ////// Do I have a zeroth child? /// internal bool HasChild0 { get { return m_children.Count > 0; } } ////// Get/set first child /// internal Node Child1 { get { return m_children[1]; } set { m_children[1] = value; } } ////// Do I have a child1? /// internal bool HasChild1 { get { return m_children.Count > 1; } } ////// get/set second child /// internal Node Child2 { get { return m_children[2]; } set { m_children[2] = value; } } ////// Do I have a child2 (third child really) /// internal bool HasChild2 { get { return m_children.Count > 2; } } #region equivalence functions ////// Is this subtree equivalent to another subtree /// /// ///internal bool IsEquivalent(Node other) { if (this.Children.Count != other.Children.Count) { return false; } bool? opEquivalent = this.Op.IsEquivalent(other.Op); if (opEquivalent != true) { return false; } for (int i = 0; i < this.Children.Count; i++) { if (!this.Children[i].IsEquivalent(other.Children[i])) { return false; } } return true; } #endregion #region NodeInfo functions /// /// Get the nodeInfo for a node. Initializes it, if it has not yet been initialized /// /// Current command object ///NodeInfo for this node internal NodeInfo GetNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } return m_nodeInfo; } ////// Gets the "extended" nodeinfo for a node; if it has not yet been initialized, then it will be /// /// current command object ///extended nodeinfo for this node internal ExtendedNodeInfo GetExtendedNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } ExtendedNodeInfo extendedNodeInfo = m_nodeInfo as ExtendedNodeInfo; Debug.Assert(extendedNodeInfo != null); return extendedNodeInfo; } private void InitializeNodeInfo(Command command) { if (this.Op.IsRelOp || this.Op.IsPhysicalOp) { m_nodeInfo = new ExtendedNodeInfo(command); } else { m_nodeInfo = new NodeInfo(command); } command.RecomputeNodeInfo(this); } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
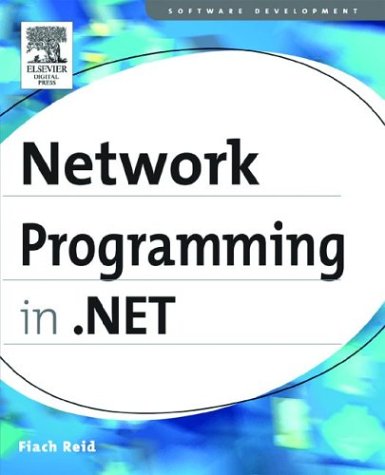
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeSnippetExpression.cs
- DataGridViewCheckBoxColumn.cs
- RelationshipEnd.cs
- WorkflowInstanceContextProvider.cs
- FrameworkRichTextComposition.cs
- ConnectionStringsSection.cs
- Constraint.cs
- DefaultCommandConverter.cs
- SpellerStatusTable.cs
- Helper.cs
- ObjectAnimationUsingKeyFrames.cs
- DataServiceResponse.cs
- Tuple.cs
- ColumnMapCopier.cs
- EntityCommand.cs
- TemplateDefinition.cs
- RtfFormatStack.cs
- SqlCommandBuilder.cs
- CalendarButton.cs
- DataGridViewCellParsingEventArgs.cs
- SpanIndex.cs
- StyleModeStack.cs
- OuterGlowBitmapEffect.cs
- VirtualizedItemPattern.cs
- EnterpriseServicesHelper.cs
- ReadOnlyObservableCollection.cs
- ImplicitInputBrush.cs
- SpeechSynthesizer.cs
- DataGridViewCellCollection.cs
- Html32TextWriter.cs
- EventLogPermissionAttribute.cs
- PropertyCollection.cs
- DeflateEmulationStream.cs
- StatusBar.cs
- HttpHandlerAction.cs
- ChannelBuilder.cs
- RuntimeCompatibilityAttribute.cs
- RadialGradientBrush.cs
- XmlStreamStore.cs
- RNGCryptoServiceProvider.cs
- XmlSchemaInfo.cs
- Polygon.cs
- HierarchicalDataSourceConverter.cs
- LinkLabelLinkClickedEvent.cs
- HtmlImage.cs
- HashAlgorithm.cs
- SingleSelectRootGridEntry.cs
- PipelineModuleStepContainer.cs
- Pair.cs
- InitializationEventAttribute.cs
- CodeSubDirectory.cs
- ObjectAssociationEndMapping.cs
- InitializingNewItemEventArgs.cs
- TransformationRules.cs
- FormsAuthenticationModule.cs
- MemoryFailPoint.cs
- ResXBuildProvider.cs
- NameValueCollection.cs
- SmtpFailedRecipientException.cs
- SqlDataReader.cs
- TraceSource.cs
- RadioButtonList.cs
- StringSorter.cs
- BaseResourcesBuildProvider.cs
- ContainerActivationHelper.cs
- ControlValuePropertyAttribute.cs
- SqlDataSourceSelectingEventArgs.cs
- AuthenticationServiceManager.cs
- ReverseInheritProperty.cs
- CapiSafeHandles.cs
- NonSerializedAttribute.cs
- HttpValueCollection.cs
- CreationContext.cs
- RequestResizeEvent.cs
- PerformanceCounter.cs
- NamespaceMapping.cs
- PriorityRange.cs
- CompensationDesigner.cs
- FileUtil.cs
- Point3DCollectionConverter.cs
- CommandField.cs
- MsmqAuthenticationMode.cs
- GenericsInstances.cs
- TrackBar.cs
- TranslateTransform3D.cs
- ClientTarget.cs
- ExtendedProperty.cs
- XmlReaderDelegator.cs
- NavigationPropertySingletonExpression.cs
- CanonicalFontFamilyReference.cs
- PublisherMembershipCondition.cs
- DataRowView.cs
- Encoder.cs
- DoubleLink.cs
- StringAnimationBase.cs
- TypeExtensionConverter.cs
- SoapMessage.cs
- LayoutUtils.cs
- SuppressMergeCheckAttribute.cs
- LeftCellWrapper.cs