Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Shapes / Polygon.cs / 1 / Polygon.cs
//---------------------------------------------------------------------------- // File: Polygon.cs // // Description: // Implementation of Polygon shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The polygon shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// Since a Polygon is really a polyline which closes its path /// public sealed class Polygon : Shape { #region Constructors ////// Instantiates a new instance of a polygon. /// public Polygon() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polygon), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polygon), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and properties ////// Get the polygon that defines this shape /// protected override Geometry DefiningGeometry { get { return _polygonGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polygonGeometry = Geometry.Empty; return; } // Create the polygon PathGeometry // if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } pathFigure.IsClosed = true; } PathGeometry polygonGeometry = new PathGeometry(); polygonGeometry.Figures.Add(pathFigure); // Set FillRule polygonGeometry.FillRule = FillRule; _polygonGeometry = polygonGeometry; } #endregion Internal Methods #region Private Methods and Members private Geometry _polygonGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
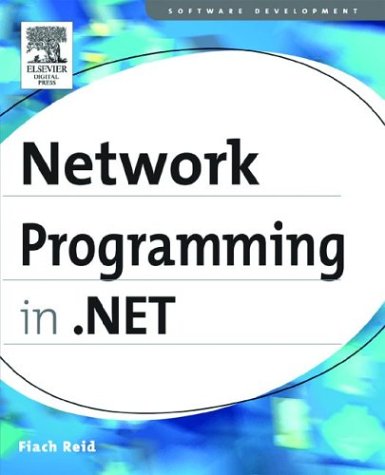
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParameterExpression.cs
- StrokeNode.cs
- XmlCodeExporter.cs
- SelectionItemPattern.cs
- ElementFactory.cs
- BamlRecordReader.cs
- Vector.cs
- DecimalAnimationBase.cs
- HotSpotCollection.cs
- MenuStrip.cs
- DbConnectionPoolOptions.cs
- ImageAnimator.cs
- ReadOnlyPropertyMetadata.cs
- InputBinding.cs
- NonBatchDirectoryCompiler.cs
- Debugger.cs
- StorageMappingItemLoader.cs
- BitmapSizeOptions.cs
- ContentElement.cs
- Policy.cs
- ExpressionNode.cs
- SelectionProcessor.cs
- StreamGeometryContext.cs
- SymbolEqualComparer.cs
- Header.cs
- Crypto.cs
- _ScatterGatherBuffers.cs
- SectionXmlInfo.cs
- DocumentReference.cs
- MediaPlayerState.cs
- ExecutionContext.cs
- keycontainerpermission.cs
- AsyncResult.cs
- ContentValidator.cs
- InstallerTypeAttribute.cs
- WindowsGraphics2.cs
- ControlHelper.cs
- ProcessInputEventArgs.cs
- SupportingTokenParameters.cs
- ImageAnimator.cs
- WindowsAltTab.cs
- SqlParameter.cs
- XmlQueryContext.cs
- DataGridCheckBoxColumn.cs
- Expressions.cs
- ComPlusInstanceProvider.cs
- FileUtil.cs
- Privilege.cs
- UrlAuthFailedErrorFormatter.cs
- CommandBindingCollection.cs
- CompilerScopeManager.cs
- ReadOnlyDataSourceView.cs
- PresentationAppDomainManager.cs
- EntityExpressionVisitor.cs
- CompareValidator.cs
- SQLInt16.cs
- WindowsListViewSubItem.cs
- EncoderParameters.cs
- UndoManager.cs
- SqlRemoveConstantOrderBy.cs
- PageTheme.cs
- Interlocked.cs
- XPathDocument.cs
- Visual3DCollection.cs
- Semaphore.cs
- ScriptRegistrationManager.cs
- SelfIssuedAuthProofToken.cs
- UrlPath.cs
- FontTypeConverter.cs
- WebUtility.cs
- Certificate.cs
- XmlSchemaSimpleContent.cs
- WebPartsPersonalizationAuthorization.cs
- ColumnBinding.cs
- AnnotationHighlightLayer.cs
- XmlAnyElementAttribute.cs
- PackageRelationship.cs
- DrawingContextWalker.cs
- ListViewContainer.cs
- documentsequencetextcontainer.cs
- MarshalByRefObject.cs
- SystemColors.cs
- WebPartChrome.cs
- HyperLinkField.cs
- ImmutableObjectAttribute.cs
- QilTypeChecker.cs
- PolicyChain.cs
- CommonDialog.cs
- ObjectManager.cs
- WebPartRestoreVerb.cs
- UserNamePasswordServiceCredential.cs
- AppDomainUnloadedException.cs
- FileClassifier.cs
- ConvertersCollection.cs
- QilGenerator.cs
- Control.cs
- AxHost.cs
- FieldNameLookup.cs
- Blend.cs
- QilLiteral.cs