Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / FlowPanelDesigner.cs / 1 / FlowPanelDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Windows.Forms.Design.Behavior; ////// /// Base class to our flow control designers: TableLayoutPanel and FlowLayoutPanel. /// This class shares common operations for these designers including: /// Stripping all padding SnapLines, adding children, and refreshing selection /// after a drag-drop operation - since these types of controls will always /// reposition children. /// internal class FlowPanelDesigner : PanelDesigner { ////// /// Overridden to disallow SnapLines during drag operations if the primary drag control /// is over the FlowPanelDesigner. /// public override bool ParticipatesWithSnapLines { get { return false; } } ////// /// Get the standard SnapLines from our ParentControlDesigner then /// strips all the padding lines - since we don't want these guys /// for flow designers. public override IList SnapLines { get { ArrayList snapLines = (ArrayList)base.SnapLines; //identify all the paddings to remove ArrayList paddingsToRemove = new ArrayList(4); foreach (SnapLine line in snapLines) { if (line.Filter != null && line.Filter.Contains(SnapLine.Padding)) { paddingsToRemove.Add(line); } } //remove all padding foreach (SnapLine line in paddingsToRemove) { snapLines.Remove(line); } return snapLines; } } /// /// /// Overrides the base and skips the adjustment of the child position /// since the runtime control will re-position this for us. /// internal override void AddChildControl(Control newChild) { // Skip location adjustment because FlowPanel is going to position this control. // Also, Skip z-order adjustment because SendToFront will put the new control at the // beginning of the flow instead of the end, plus FlowLayout is already preventing // overlap. this.Control.Controls.Add(newChild); } ////// /// We override this, call base, then attempt to re-sync the selection /// since the control will most likely re-position a child for us. /// protected override void OnDragDrop(DragEventArgs de) { base.OnDragDrop(de); SelectionManager sm = GetService(typeof(SelectionManager)) as SelectionManager; if (sm != null) { sm.Refresh(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
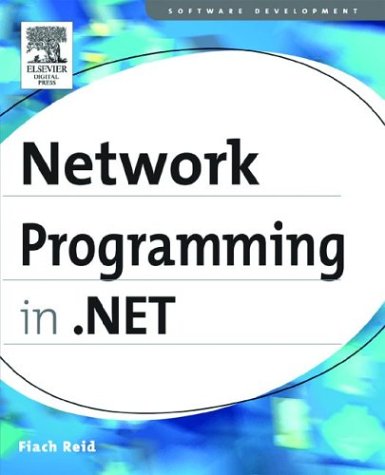
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SizeF.cs
- CSharpCodeProvider.cs
- ProcessInfo.cs
- _CookieModule.cs
- DialogResultConverter.cs
- ChangeInterceptorAttribute.cs
- GenericFlowSwitchHelper.cs
- DateTimeUtil.cs
- AppDomain.cs
- RangeValueProviderWrapper.cs
- DocumentViewerConstants.cs
- DoubleAnimationBase.cs
- ShaderEffect.cs
- ACE.cs
- RuleElement.cs
- IteratorFilter.cs
- SafeNativeMethods.cs
- XmlAnyElementAttribute.cs
- FixedElement.cs
- SolidBrush.cs
- HostProtectionException.cs
- RSAOAEPKeyExchangeDeformatter.cs
- ObjectSecurity.cs
- DataGridViewComboBoxColumn.cs
- CultureTable.cs
- StreamWriter.cs
- COM2AboutBoxPropertyDescriptor.cs
- AsyncResult.cs
- ClientRoleProvider.cs
- CoTaskMemHandle.cs
- MessagingDescriptionAttribute.cs
- QuaternionRotation3D.cs
- SQLBytes.cs
- FontCacheUtil.cs
- DispatcherEventArgs.cs
- KeyBinding.cs
- SchemaElement.cs
- GeometryConverter.cs
- ConstructorNeedsTagAttribute.cs
- AsyncCompletedEventArgs.cs
- BitmapEffectGroup.cs
- HeaderedContentControl.cs
- ToolStripDropDownItem.cs
- HtmlInputText.cs
- DbException.cs
- PathData.cs
- XsltFunctions.cs
- ToggleButton.cs
- TextEncodedRawTextWriter.cs
- NumberSubstitution.cs
- DataGridItem.cs
- TextEffect.cs
- ToolStripLabel.cs
- OAVariantLib.cs
- UnmanagedMemoryStream.cs
- X509ThumbprintKeyIdentifierClause.cs
- ProcessManager.cs
- FormatterServices.cs
- PointAnimationUsingKeyFrames.cs
- RuleSettingsCollection.cs
- RoleService.cs
- CompilerLocalReference.cs
- HMACRIPEMD160.cs
- DataRelation.cs
- DataSourceXmlSubItemAttribute.cs
- WebServiceEnumData.cs
- SkipQueryOptionExpression.cs
- MenuItemAutomationPeer.cs
- TableProviderWrapper.cs
- HelpProvider.cs
- IdentifierService.cs
- EntityModelBuildProvider.cs
- WindowsFormsSynchronizationContext.cs
- HostedElements.cs
- SamlNameIdentifierClaimResource.cs
- BuildTopDownAttribute.cs
- SQLBoolean.cs
- ComponentRenameEvent.cs
- PeerNearMe.cs
- StyleConverter.cs
- ConfigUtil.cs
- WindowsStartMenu.cs
- DefaultExpressionVisitor.cs
- WindowsAuthenticationEventArgs.cs
- Quad.cs
- DelegateOutArgument.cs
- Drawing.cs
- FamilyMap.cs
- Style.cs
- AssemblyUtil.cs
- IItemProperties.cs
- WhitespaceReader.cs
- EntityException.cs
- CompletedAsyncResult.cs
- DecoratedNameAttribute.cs
- AttributeQuery.cs
- SoapIgnoreAttribute.cs
- InstanceDataCollection.cs
- FormViewDeleteEventArgs.cs
- DataGridCellsPresenter.cs