Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceResponse.cs / 1305376 / DataServiceResponse.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// data service response to ExecuteBatch & SaveChanges // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; ////// Data service response to ExecuteBatch & SaveChanges /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1010", Justification = "required for this feature")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710", Justification = "required for this feature")] public sealed class DataServiceResponse : IEnumerable{ /// Http headers of the response. private Dictionaryheaders; /// Http status code of the response. private int statusCode; ///responses private IEnumerableresponse; /// true if this is a batch response, otherwise false. private bool batchResponse; ////// constructor /// /// HTTP headers /// HTTP status code /// list of responses /// true if this represents a batch response, otherwise false. internal DataServiceResponse(Dictionaryheaders, int statusCode, IEnumerable response, bool batchResponse) { this.headers = headers ?? new Dictionary (EqualityComparer .Default); this.statusCode = statusCode; this.batchResponse = batchResponse; this.response = response; } /// Http headers of the response. public IDictionaryBatchHeaders { get { return this.headers; } } /// Http status code of the response. public int BatchStatusCode { get { return this.statusCode; } } ///Returns true if this is a batch response. Otherwise returns false. public bool IsBatchResponse { get { return this.batchResponse; } } ///Responses of a batch query operation. ///The sequence of respones to operation public IEnumeratorGetEnumerator() { return this.response.GetEnumerator(); } /// Get an enumerator for the OperationResponse. ///an enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// data service response to ExecuteBatch & SaveChanges // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; ////// Data service response to ExecuteBatch & SaveChanges /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1010", Justification = "required for this feature")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710", Justification = "required for this feature")] public sealed class DataServiceResponse : IEnumerable{ /// Http headers of the response. private Dictionaryheaders; /// Http status code of the response. private int statusCode; ///responses private IEnumerableresponse; /// true if this is a batch response, otherwise false. private bool batchResponse; ////// constructor /// /// HTTP headers /// HTTP status code /// list of responses /// true if this represents a batch response, otherwise false. internal DataServiceResponse(Dictionaryheaders, int statusCode, IEnumerable response, bool batchResponse) { this.headers = headers ?? new Dictionary (EqualityComparer .Default); this.statusCode = statusCode; this.batchResponse = batchResponse; this.response = response; } /// Http headers of the response. public IDictionaryBatchHeaders { get { return this.headers; } } /// Http status code of the response. public int BatchStatusCode { get { return this.statusCode; } } ///Returns true if this is a batch response. Otherwise returns false. public bool IsBatchResponse { get { return this.batchResponse; } } ///Responses of a batch query operation. ///The sequence of respones to operation public IEnumeratorGetEnumerator() { return this.response.GetEnumerator(); } /// Get an enumerator for the OperationResponse. ///an enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return this.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
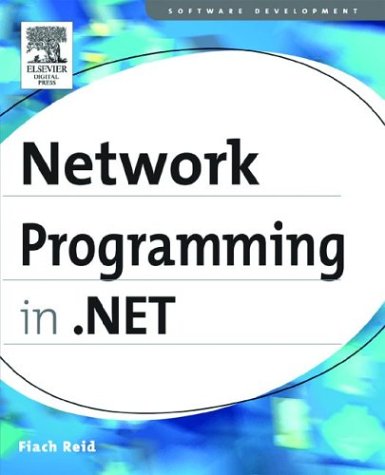
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericRootAutomationPeer.cs
- OracleColumn.cs
- CodeTypeReferenceCollection.cs
- XmlQueryStaticData.cs
- datacache.cs
- LateBoundChannelParameterCollection.cs
- SwitchElementsCollection.cs
- MasterPageBuildProvider.cs
- XmlSchemaChoice.cs
- TraceContext.cs
- Domain.cs
- PreviewKeyDownEventArgs.cs
- RC2CryptoServiceProvider.cs
- ApplicationInfo.cs
- XmlAttributeProperties.cs
- RegexGroupCollection.cs
- Privilege.cs
- MSAAWinEventWrap.cs
- PrintPreviewDialog.cs
- CapabilitiesPattern.cs
- MultipleViewPattern.cs
- Collection.cs
- ButtonRenderer.cs
- ImplicitInputBrush.cs
- MessageContractExporter.cs
- BitmapFrameEncode.cs
- GeometryGroup.cs
- PropertyKey.cs
- LinearGradientBrush.cs
- WebPartConnectionsConnectVerb.cs
- SerialErrors.cs
- DispatchWrapper.cs
- PagerSettings.cs
- XmlNavigatorStack.cs
- RuntimeResourceSet.cs
- BufferedWebEventProvider.cs
- MethodImplAttribute.cs
- PrintDialog.cs
- DrawingCollection.cs
- Int32Collection.cs
- TemplatePartAttribute.cs
- PositiveTimeSpanValidator.cs
- FrameworkName.cs
- ServiceDescriptionSerializer.cs
- HttpModuleAction.cs
- EventData.cs
- NativeMethodsOther.cs
- FormattedTextSymbols.cs
- XmlText.cs
- DoubleCollection.cs
- CollectionConverter.cs
- Misc.cs
- AsyncInvokeContext.cs
- SharedUtils.cs
- JsonQNameDataContract.cs
- SQLGuid.cs
- Opcode.cs
- MethodCallExpression.cs
- EventData.cs
- PreviewKeyDownEventArgs.cs
- TextHidden.cs
- ReadOnlyPropertyMetadata.cs
- GradientBrush.cs
- SHA512Cng.cs
- CodeEventReferenceExpression.cs
- UnicodeEncoding.cs
- ChtmlTextWriter.cs
- MetadataPropertyCollection.cs
- ToolStripSeparatorRenderEventArgs.cs
- WmlSelectionListAdapter.cs
- ProtocolsConfiguration.cs
- DataGridViewComboBoxCell.cs
- DbException.cs
- Mutex.cs
- ObfuscateAssemblyAttribute.cs
- StylusOverProperty.cs
- Span.cs
- Parser.cs
- infer.cs
- DuplicateWaitObjectException.cs
- MDIClient.cs
- ActiveDocumentEvent.cs
- HostingEnvironmentSection.cs
- ListContractAdapter.cs
- RowBinding.cs
- _NetRes.cs
- SqlResolver.cs
- OverflowException.cs
- EarlyBoundInfo.cs
- TextRangeProviderWrapper.cs
- CompletionBookmark.cs
- ScrollBarAutomationPeer.cs
- ContainerAction.cs
- TreeNodeStyleCollection.cs
- TreeNodeStyleCollection.cs
- ContextProperty.cs
- InkCollectionBehavior.cs
- XsdBuilder.cs
- WebServiceReceiveDesigner.cs
- WebEventCodes.cs