Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / XslException.cs / 1 / XslException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.CodeDom.Compiler; using System.Diagnostics; using System.Globalization; using System.Resources; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; namespace System.Xml.Xsl { using Res = System.Xml.Utils.Res; [Serializable] internal class XslTransformException : XsltException { protected XslTransformException(SerializationInfo info, StreamingContext context) : base(info, context) {} public XslTransformException(Exception inner, string res, params string[] args) : base(CreateMessage(res, args), inner) {} public XslTransformException(string message) : base(CreateMessage(message, null), null) {} internal XslTransformException(string res, params string[] args) : this(null, res, args) {} internal static string CreateMessage(string res, params string[] args) { string message = null; try { message = Res.GetString(res, args); } catch (MissingManifestResourceException) { } if (message != null) { return message; } StringBuilder sb = new StringBuilder(res); if (args != null && args.Length > 0) { Debug.Fail("Resource string '" + res + "' was not found"); sb.Append('('); sb.Append(args[0]); for (int idx = 1; idx < args.Length; idx++) { sb.Append(", "); sb.Append(args[idx]); } sb.Append(')'); } return sb.ToString(); } internal virtual string FormatDetailedMessage() { return Message; } public override string ToString() { string result = this.GetType().FullName; string info = FormatDetailedMessage(); if (info != null && info.Length > 0) { result += ": " + info; } if (InnerException != null) { result += " ---> " + InnerException.ToString() + Environment.NewLine + " " + CreateMessage(Res.Xml_EndOfInnerExceptionStack); } if (StackTrace != null) { result += Environment.NewLine + StackTrace; } return result; } } [Serializable] internal class XslLoadException : XslTransformException { ISourceLineInfo lineInfo; protected XslLoadException (SerializationInfo info, StreamingContext context) : base(info, context) { bool hasLineInfo = (bool) info.GetValue("hasLineInfo", typeof(bool)); if (hasLineInfo) { string uriString; int startLine, startPos, endLine, endPos; uriString = (string) info.GetValue("Uri" , typeof(string )); startLine = (int) info.GetValue("StartLine" , typeof(int )); startPos = (int) info.GetValue("StartPos" , typeof(int )); endLine = (int) info.GetValue("EndLine" , typeof(int )); endPos = (int) info.GetValue("EndPos" , typeof(int )); lineInfo = new SourceLineInfo(uriString, startLine, startPos, endLine, endPos); } } [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); info.AddValue("hasLineInfo" , lineInfo != null); if (lineInfo != null) { info.AddValue("Uri" , lineInfo.Uri); info.AddValue("StartLine", lineInfo.StartLine); info.AddValue("StartPos" , lineInfo.StartPos ); info.AddValue("EndLine" , lineInfo.EndLine); info.AddValue("EndPos" , lineInfo.EndPos ); } } internal XslLoadException(string res, params string[] args) : base(null, res, args) {} internal XslLoadException(Exception inner, ISourceLineInfo lineInfo) : base(inner, Res.Xslt_CompileError2, null) { this.lineInfo = lineInfo; } internal XslLoadException(CompilerError error) : base(Res.Xml_UserException, new string[] { error.ErrorText }) { SetSourceLineInfo(new SourceLineInfo(error.FileName, error.Line, error.Column, error.Line, error.Column)); } internal void SetSourceLineInfo(ISourceLineInfo lineInfo) { this.lineInfo = lineInfo; } public override string SourceUri { get { return lineInfo != null ? lineInfo.Uri : null; } } public override int LineNumber { get { return lineInfo != null ? lineInfo.StartLine : 0; } } public override int LinePosition { get { return lineInfo != null ? lineInfo.StartPos : 0; } } private static string AppendLineInfoMessage(string message, ISourceLineInfo lineInfo) { if (lineInfo != null) { string fileName = SourceLineInfo.GetFileName(lineInfo.Uri); string lineInfoMessage = CreateMessage(Res.Xml_ErrorFilePosition, fileName, lineInfo.StartLine.ToString(CultureInfo.InvariantCulture), lineInfo.StartPos.ToString(CultureInfo.InvariantCulture)); if (lineInfoMessage != null && lineInfoMessage.Length > 0) { if (message.Length > 0 && !XmlCharType.Instance.IsWhiteSpace(message[message.Length - 1])) { message += " "; } message += lineInfoMessage; } } return message; } internal static string CreateMessage(ISourceLineInfo lineInfo, string res, params string[] args) { return AppendLineInfoMessage(CreateMessage(res, args), lineInfo); } internal override string FormatDetailedMessage() { return AppendLineInfoMessage(Message, lineInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.CodeDom.Compiler; using System.Diagnostics; using System.Globalization; using System.Resources; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; namespace System.Xml.Xsl { using Res = System.Xml.Utils.Res; [Serializable] internal class XslTransformException : XsltException { protected XslTransformException(SerializationInfo info, StreamingContext context) : base(info, context) {} public XslTransformException(Exception inner, string res, params string[] args) : base(CreateMessage(res, args), inner) {} public XslTransformException(string message) : base(CreateMessage(message, null), null) {} internal XslTransformException(string res, params string[] args) : this(null, res, args) {} internal static string CreateMessage(string res, params string[] args) { string message = null; try { message = Res.GetString(res, args); } catch (MissingManifestResourceException) { } if (message != null) { return message; } StringBuilder sb = new StringBuilder(res); if (args != null && args.Length > 0) { Debug.Fail("Resource string '" + res + "' was not found"); sb.Append('('); sb.Append(args[0]); for (int idx = 1; idx < args.Length; idx++) { sb.Append(", "); sb.Append(args[idx]); } sb.Append(')'); } return sb.ToString(); } internal virtual string FormatDetailedMessage() { return Message; } public override string ToString() { string result = this.GetType().FullName; string info = FormatDetailedMessage(); if (info != null && info.Length > 0) { result += ": " + info; } if (InnerException != null) { result += " ---> " + InnerException.ToString() + Environment.NewLine + " " + CreateMessage(Res.Xml_EndOfInnerExceptionStack); } if (StackTrace != null) { result += Environment.NewLine + StackTrace; } return result; } } [Serializable] internal class XslLoadException : XslTransformException { ISourceLineInfo lineInfo; protected XslLoadException (SerializationInfo info, StreamingContext context) : base(info, context) { bool hasLineInfo = (bool) info.GetValue("hasLineInfo", typeof(bool)); if (hasLineInfo) { string uriString; int startLine, startPos, endLine, endPos; uriString = (string) info.GetValue("Uri" , typeof(string )); startLine = (int) info.GetValue("StartLine" , typeof(int )); startPos = (int) info.GetValue("StartPos" , typeof(int )); endLine = (int) info.GetValue("EndLine" , typeof(int )); endPos = (int) info.GetValue("EndPos" , typeof(int )); lineInfo = new SourceLineInfo(uriString, startLine, startPos, endLine, endPos); } } [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter=true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); info.AddValue("hasLineInfo" , lineInfo != null); if (lineInfo != null) { info.AddValue("Uri" , lineInfo.Uri); info.AddValue("StartLine", lineInfo.StartLine); info.AddValue("StartPos" , lineInfo.StartPos ); info.AddValue("EndLine" , lineInfo.EndLine); info.AddValue("EndPos" , lineInfo.EndPos ); } } internal XslLoadException(string res, params string[] args) : base(null, res, args) {} internal XslLoadException(Exception inner, ISourceLineInfo lineInfo) : base(inner, Res.Xslt_CompileError2, null) { this.lineInfo = lineInfo; } internal XslLoadException(CompilerError error) : base(Res.Xml_UserException, new string[] { error.ErrorText }) { SetSourceLineInfo(new SourceLineInfo(error.FileName, error.Line, error.Column, error.Line, error.Column)); } internal void SetSourceLineInfo(ISourceLineInfo lineInfo) { this.lineInfo = lineInfo; } public override string SourceUri { get { return lineInfo != null ? lineInfo.Uri : null; } } public override int LineNumber { get { return lineInfo != null ? lineInfo.StartLine : 0; } } public override int LinePosition { get { return lineInfo != null ? lineInfo.StartPos : 0; } } private static string AppendLineInfoMessage(string message, ISourceLineInfo lineInfo) { if (lineInfo != null) { string fileName = SourceLineInfo.GetFileName(lineInfo.Uri); string lineInfoMessage = CreateMessage(Res.Xml_ErrorFilePosition, fileName, lineInfo.StartLine.ToString(CultureInfo.InvariantCulture), lineInfo.StartPos.ToString(CultureInfo.InvariantCulture)); if (lineInfoMessage != null && lineInfoMessage.Length > 0) { if (message.Length > 0 && !XmlCharType.Instance.IsWhiteSpace(message[message.Length - 1])) { message += " "; } message += lineInfoMessage; } } return message; } internal static string CreateMessage(ISourceLineInfo lineInfo, string res, params string[] args) { return AppendLineInfoMessage(CreateMessage(res, args), lineInfo); } internal override string FormatDetailedMessage() { return AppendLineInfoMessage(Message, lineInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
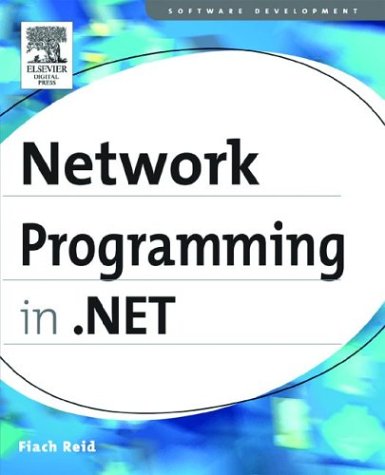
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColumnTypeConverter.cs
- IDReferencePropertyAttribute.cs
- QueueProcessor.cs
- ResolveResponse.cs
- GeometryConverter.cs
- WebPartCatalogAddVerb.cs
- EncoderBestFitFallback.cs
- StringAnimationUsingKeyFrames.cs
- SelectionRangeConverter.cs
- WindowsMenu.cs
- SizeIndependentAnimationStorage.cs
- SurrogateEncoder.cs
- DataSetUtil.cs
- SrgsNameValueTag.cs
- Brush.cs
- FormsAuthentication.cs
- OleDbPermission.cs
- DataListItemCollection.cs
- MetadataCollection.cs
- WorkflowDurableInstance.cs
- __ComObject.cs
- Privilege.cs
- ContextMarshalException.cs
- AsymmetricSignatureFormatter.cs
- RawAppCommandInputReport.cs
- XmlComplianceUtil.cs
- Win32Native.cs
- UshortList2.cs
- ResourceKey.cs
- IDictionary.cs
- DatePickerDateValidationErrorEventArgs.cs
- WmlImageAdapter.cs
- WeakReferenceList.cs
- IIS7UserPrincipal.cs
- File.cs
- WSFederationHttpBindingElement.cs
- FontNamesConverter.cs
- SafeSecurityHandles.cs
- WpfPayload.cs
- PropertyInfoSet.cs
- SocketInformation.cs
- XmlAttributeOverrides.cs
- ObjectConverter.cs
- COM2IProvidePropertyBuilderHandler.cs
- TagNameToTypeMapper.cs
- XPathScanner.cs
- ExpressionBuilderContext.cs
- ScriptIgnoreAttribute.cs
- AnnotationObservableCollection.cs
- RectConverter.cs
- Crypto.cs
- RotateTransform3D.cs
- NavigationCommands.cs
- SessionSwitchEventArgs.cs
- SymbolDocumentGenerator.cs
- GraphicsPath.cs
- SessionPageStateSection.cs
- WindowHelperService.cs
- StringHandle.cs
- BitmapEffectInput.cs
- Directory.cs
- StackSpiller.cs
- ValidationPropertyAttribute.cs
- SiteMapDataSourceView.cs
- translator.cs
- LineVisual.cs
- HtmlControlPersistable.cs
- BindingValueChangedEventArgs.cs
- StackBuilderSink.cs
- DetailsViewPageEventArgs.cs
- ImageMapEventArgs.cs
- ResourceSet.cs
- WebBrowsableAttribute.cs
- GenericUriParser.cs
- GridViewColumnHeader.cs
- TextSelectionHelper.cs
- TextEditorSpelling.cs
- DataGridViewCellStyleEditor.cs
- OleDbPropertySetGuid.cs
- CharacterHit.cs
- ConstraintConverter.cs
- EncoderFallback.cs
- ComponentResourceManager.cs
- CaseKeyBox.xaml.cs
- ToolboxDataAttribute.cs
- ClientSettings.cs
- BlockingCollection.cs
- CodeTypeReferenceCollection.cs
- Pens.cs
- SQLMembershipProvider.cs
- TitleStyle.cs
- SqlCommand.cs
- XmlLanguage.cs
- RelationalExpressions.cs
- ResourceAttributes.cs
- DataServiceQueryProvider.cs
- Configuration.cs
- AuthenticationService.cs
- CellConstant.cs
- CompilerWrapper.cs