Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Dispatcher / WorkflowDurableInstance.cs / 1305376 / WorkflowDurableInstance.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.Workflow.Runtime; using System.Runtime.Diagnostics; using System.ServiceModel.Diagnostics; using System.Diagnostics; class WorkflowDurableInstance : DurableInstance { WorkflowOperationAsyncResult currentOperationInvocation; WorkflowInstanceContextProvider instanceContextProvider; bool shouldCreateNew = false; object thisLock = new object(); WorkflowDefinitionContext workflowDefinition; WorkflowInstance workflowInstance = null; public WorkflowDurableInstance(WorkflowInstanceContextProvider instanceContextProvider, Guid instanceId, WorkflowDefinitionContext workflowDefinition, bool createNew) : base(instanceContextProvider, instanceId) { if (workflowDefinition == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("workflowDefinition"); } this.workflowDefinition = workflowDefinition; this.shouldCreateNew = createNew; this.instanceContextProvider = instanceContextProvider; } public WorkflowOperationAsyncResult CurrentOperationInvocation { get { return this.currentOperationInvocation; } set { this.currentOperationInvocation = value; } } public WorkflowInstance GetWorkflowInstance(bool canCreateInstance) { if (this.workflowInstance == null) { lock (thisLock) { if (shouldCreateNew) { if (canCreateInstance) { this.workflowInstance = this.workflowDefinition.CreateWorkflow(this.InstanceId); shouldCreateNew = false; if (DiagnosticUtility.ShouldTraceInformation) { string traceText = SR.GetString(SR.TraceCodeWorkflowDurableInstanceActivated, InstanceId); TraceUtility.TraceEvent(TraceEventType.Information, TraceCode.WorkflowDurableInstanceActivated, traceText, new StringTraceRecord("DurableInstanceDetail", traceText), this, null); } using (new WorkflowDispatchContext(true, true)) { this.workflowInstance.Start(); } } else { //Make sure we clean up this InstanceContext; DurableErrorHandler.CleanUpInstanceContextAtOperationCompletion(); //Inform InstanceLifeTimeManager to clean up record for InstanceId; if (this.instanceContextProvider.InstanceLifeTimeManager != null) { this.instanceContextProvider.InstanceLifeTimeManager.CleanUp(this.InstanceId); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new FaultException(new DurableDispatcherAddressingFault())); } } else { this.workflowInstance = this.workflowDefinition.WorkflowRuntime.GetWorkflow(InstanceId); if (DiagnosticUtility.ShouldTraceInformation) { string traceText = SR.GetString(SR.TraceCodeWorkflowDurableInstanceLoaded, InstanceId); TraceUtility.TraceEvent(TraceEventType.Information, TraceCode.WorkflowDurableInstanceLoaded, traceText, new StringTraceRecord("DurableInstanceDetail", traceText), this, null); } } } } return workflowInstance; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
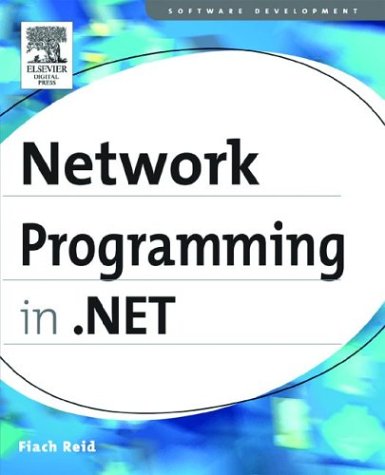
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryMethodMessage.cs
- SamlSubject.cs
- ZipIOExtraFieldZip64Element.cs
- StreamResourceInfo.cs
- PipelineModuleStepContainer.cs
- SapiGrammar.cs
- Win32KeyboardDevice.cs
- DataControlCommands.cs
- DirectoryObjectSecurity.cs
- CodeNamespaceImportCollection.cs
- ModelItemImpl.cs
- OpenTypeLayout.cs
- BrushValueSerializer.cs
- TypeConverterHelper.cs
- ToolStripCustomTypeDescriptor.cs
- ConfigXmlWhitespace.cs
- InputGestureCollection.cs
- JsonStringDataContract.cs
- EditCommandColumn.cs
- AspNetHostingPermission.cs
- MultiBindingExpression.cs
- _DomainName.cs
- ContextMarshalException.cs
- CommandValueSerializer.cs
- FixedSOMSemanticBox.cs
- ServiceHostFactory.cs
- StrongNameIdentityPermission.cs
- SqlFunctionAttribute.cs
- WinEventTracker.cs
- IsolationInterop.cs
- TypeDescriptor.cs
- filewebrequest.cs
- SynchronousChannel.cs
- UnauthorizedWebPart.cs
- Rotation3DAnimation.cs
- XmlHierarchyData.cs
- AssertFilter.cs
- CodePageEncoding.cs
- DataIdProcessor.cs
- TextRangeEdit.cs
- GroupBoxAutomationPeer.cs
- DataGridCellClipboardEventArgs.cs
- CapabilitiesState.cs
- IisTraceListener.cs
- XmlStreamStore.cs
- OrderedHashRepartitionEnumerator.cs
- HtmlInputRadioButton.cs
- WebSysDefaultValueAttribute.cs
- SqlBulkCopyColumnMapping.cs
- FilteredAttributeCollection.cs
- MasterPageCodeDomTreeGenerator.cs
- peersecurityelement.cs
- DispatcherTimer.cs
- DataColumnMapping.cs
- Activator.cs
- DataViewSetting.cs
- XhtmlMobileTextWriter.cs
- Renderer.cs
- Config.cs
- Pkcs7Recipient.cs
- HostVisual.cs
- FontDialog.cs
- UIElementParagraph.cs
- XmlSchemaAny.cs
- control.ime.cs
- BamlBinaryWriter.cs
- ComplexTypeEmitter.cs
- AssociationSetEnd.cs
- MouseEventArgs.cs
- ExecutionScope.cs
- UserNameSecurityTokenAuthenticator.cs
- InputLanguageManager.cs
- Util.cs
- TimeZone.cs
- SqlDependencyUtils.cs
- Cloud.cs
- Types.cs
- MailDefinitionBodyFileNameEditor.cs
- TokenFactoryBase.cs
- TdsParserStaticMethods.cs
- DataGridRelationshipRow.cs
- Hex.cs
- ViewStateException.cs
- AppDomainGrammarProxy.cs
- SqlFactory.cs
- WindowsListViewSubItem.cs
- SettingsSavedEventArgs.cs
- RoutedPropertyChangedEventArgs.cs
- DataKeyCollection.cs
- ImplicitInputBrush.cs
- ActivityCodeDomReferenceService.cs
- PerformanceCounterNameAttribute.cs
- JoinCqlBlock.cs
- ListParagraph.cs
- ObjectManager.cs
- ListViewDesigner.cs
- HistoryEventArgs.cs
- DataErrorValidationRule.cs
- BinarySecretKeyIdentifierClause.cs
- Pair.cs