Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / MD5CryptoServiceProvider.cs / 1305376 / MD5CryptoServiceProvider.cs
using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // MD5CryptoServiceProvider.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class MD5CryptoServiceProvider : MD5 { [System.Security.SecurityCritical /*auto-generated*/] private SafeHashHandle _safeHashHandle = null; // // public constructors // [System.Security.SecuritySafeCritical] // auto-generated public MD5CryptoServiceProvider() { if (CryptoConfig.AllowOnlyFipsAlgorithms) throw new InvalidOperationException(Environment.GetResourceString("Cryptography_NonCompliantFIPSAlgorithm")); Contract.EndContractBlock(); // _CreateHash will check for failures and throw the appropriate exception _safeHashHandle = Utils.CreateHash(Utils.StaticProvHandle, Constants.CALG_MD5); } [System.Security.SecuritySafeCritical] // overrides public transparent member protected override void Dispose(bool disposing) { if (_safeHashHandle != null && !_safeHashHandle.IsClosed) _safeHashHandle.Dispose(); base.Dispose(disposing); } // // public methods // [System.Security.SecuritySafeCritical] // auto-generated public override void Initialize() { if (_safeHashHandle != null && !_safeHashHandle.IsClosed) _safeHashHandle.Dispose(); // _CreateHash will check for failures and throw the appropriate exception _safeHashHandle = Utils.CreateHash(Utils.StaticProvHandle, Constants.CALG_MD5); } [System.Security.SecuritySafeCritical] // overrides protected transparent member protected override void HashCore(byte[] rgb, int ibStart, int cbSize) { Utils.HashData(_safeHashHandle, rgb, ibStart, cbSize); } [System.Security.SecuritySafeCritical] // overrides protected transparent member protected override byte[] HashFinal() { return Utils.EndHash(_safeHashHandle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
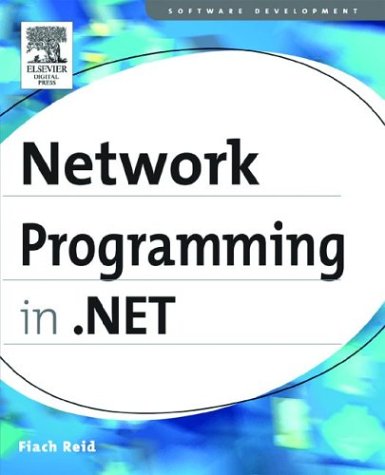
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTimeValueSerializer.cs
- AppSettingsSection.cs
- AnnotationStore.cs
- ContextInformation.cs
- TypographyProperties.cs
- ScrollProperties.cs
- ClientFormsAuthenticationMembershipProvider.cs
- SchemaType.cs
- WithParamAction.cs
- WebRequestModuleElementCollection.cs
- StringResourceManager.cs
- ControlEvent.cs
- PrintDialogException.cs
- Validator.cs
- ChannelManager.cs
- UserControlAutomationPeer.cs
- ScalarConstant.cs
- ExpressionBindingCollection.cs
- DataSourceSelectArguments.cs
- CodeAttributeDeclaration.cs
- Stylesheet.cs
- FormattedText.cs
- RandomNumberGenerator.cs
- DrawingAttributesDefaultValueFactory.cs
- DataPager.cs
- SqlProviderUtilities.cs
- Helper.cs
- AbsoluteQuery.cs
- EventHandlerList.cs
- XmlJsonReader.cs
- ProviderConnectionPoint.cs
- sqlcontext.cs
- PackWebRequestFactory.cs
- RequestUriProcessor.cs
- RuntimeConfigurationRecord.cs
- DeclaredTypeValidator.cs
- SignalGate.cs
- DeriveBytes.cs
- CatalogPartDesigner.cs
- XmlWriter.cs
- StatusBarPanelClickEvent.cs
- UnrecognizedPolicyAssertionElement.cs
- ResourceManager.cs
- Visual.cs
- DataProviderNameConverter.cs
- DbConnectionHelper.cs
- UpdatePanel.cs
- BitmapPalette.cs
- MemoryFailPoint.cs
- QueryCacheKey.cs
- NameObjectCollectionBase.cs
- QuadraticBezierSegment.cs
- XmlValidatingReaderImpl.cs
- ProcessHostMapPath.cs
- RefreshPropertiesAttribute.cs
- ClientSideProviderDescription.cs
- Msmq4SubqueuePoisonHandler.cs
- CanonicalFormWriter.cs
- X509Chain.cs
- EntityContainerEmitter.cs
- EntityCommandCompilationException.cs
- ListViewAutomationPeer.cs
- BasicExpandProvider.cs
- SortExpressionBuilder.cs
- DayRenderEvent.cs
- VSDExceptions.cs
- LogPolicy.cs
- LateBoundBitmapDecoder.cs
- MouseBinding.cs
- Size.cs
- HttpAsyncResult.cs
- __Error.cs
- RoleExceptions.cs
- TemplateXamlParser.cs
- SelectedGridItemChangedEvent.cs
- WebResponse.cs
- _FixedSizeReader.cs
- StringComparer.cs
- AddInAdapter.cs
- KoreanLunisolarCalendar.cs
- XPathPatternBuilder.cs
- BuilderPropertyEntry.cs
- MembershipValidatePasswordEventArgs.cs
- XmlLinkedNode.cs
- MetadataPropertyCollection.cs
- StylusCaptureWithinProperty.cs
- ReferenceEqualityComparer.cs
- SignedInfo.cs
- BamlTreeMap.cs
- MultipleViewProviderWrapper.cs
- LoginView.cs
- HandleValueEditor.cs
- SystemInformation.cs
- httpapplicationstate.cs
- SspiWrapper.cs
- TableAdapterManagerHelper.cs
- HyperlinkAutomationPeer.cs
- TreeNodeConverter.cs
- BinarySecretKeyIdentifierClause.cs
- MetadataProperty.cs