Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / System.Runtime.DurableInstancing / System / Runtime / SignalGate.cs / 1305376 / SignalGate.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime { using System; using System.Threading; [Fx.Tag.SynchronizationPrimitive(Fx.Tag.BlocksUsing.NonBlocking)] class SignalGate { [Fx.Tag.SynchronizationObject(Blocking=false, Kind=Fx.Tag.SynchronizationKind.InterlockedNoSpin)] int state; public SignalGate() { } internal bool IsLocked { get { return this.state == GateState.Locked; } } internal bool IsSignalled { get { return this.state == GateState.Signalled; } } // Returns true if this brings the gate to the Signalled state. // Transitions - Locked -> SignalPending | Completed before it was unlocked // Unlocked -> Signaled public bool Signal() { int lastState = this.state; if (lastState == GateState.Locked) { lastState = Interlocked.CompareExchange(ref this.state, GateState.SignalPending, GateState.Locked); } if (lastState == GateState.Unlocked) { this.state = GateState.Signalled; return true; } if (lastState != GateState.Locked) { ThrowInvalidSignalGateState(); } return false; } // Returns true if this brings the gate to the Signalled state. // Transitions - SignalPending -> Signaled | return the AsyncResult since the callback already // | completed and provided the result on its thread // Locked -> Unlocked public bool Unlock() { int lastState = this.state; if (lastState == GateState.Locked) { lastState = Interlocked.CompareExchange(ref this.state, GateState.Unlocked, GateState.Locked); } if (lastState == GateState.SignalPending) { this.state = GateState.Signalled; return true; } if (lastState != GateState.Locked) { ThrowInvalidSignalGateState(); } return false; } // This is factored out to allow Signal and Unlock to be inlined. void ThrowInvalidSignalGateState() { throw Fx.Exception.AsError(new InvalidOperationException(SRCore.InvalidSemaphoreExit)); } static class GateState { public const int Locked = 0; public const int SignalPending = 1; public const int Unlocked = 2; public const int Signalled = 3; } } [Fx.Tag.SynchronizationPrimitive(Fx.Tag.BlocksUsing.NonBlocking)] class SignalGate: SignalGate { T result; public SignalGate() : base() { } public bool Signal(T result) { this.result = result; return Signal(); } public bool Unlock(out T result) { if (Unlock()) { result = this.result; return true; } result = default(T); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime { using System; using System.Threading; [Fx.Tag.SynchronizationPrimitive(Fx.Tag.BlocksUsing.NonBlocking)] class SignalGate { [Fx.Tag.SynchronizationObject(Blocking=false, Kind=Fx.Tag.SynchronizationKind.InterlockedNoSpin)] int state; public SignalGate() { } internal bool IsLocked { get { return this.state == GateState.Locked; } } internal bool IsSignalled { get { return this.state == GateState.Signalled; } } // Returns true if this brings the gate to the Signalled state. // Transitions - Locked -> SignalPending | Completed before it was unlocked // Unlocked -> Signaled public bool Signal() { int lastState = this.state; if (lastState == GateState.Locked) { lastState = Interlocked.CompareExchange(ref this.state, GateState.SignalPending, GateState.Locked); } if (lastState == GateState.Unlocked) { this.state = GateState.Signalled; return true; } if (lastState != GateState.Locked) { ThrowInvalidSignalGateState(); } return false; } // Returns true if this brings the gate to the Signalled state. // Transitions - SignalPending -> Signaled | return the AsyncResult since the callback already // | completed and provided the result on its thread // Locked -> Unlocked public bool Unlock() { int lastState = this.state; if (lastState == GateState.Locked) { lastState = Interlocked.CompareExchange(ref this.state, GateState.Unlocked, GateState.Locked); } if (lastState == GateState.SignalPending) { this.state = GateState.Signalled; return true; } if (lastState != GateState.Locked) { ThrowInvalidSignalGateState(); } return false; } // This is factored out to allow Signal and Unlock to be inlined. void ThrowInvalidSignalGateState() { throw Fx.Exception.AsError(new InvalidOperationException(SRCore.InvalidSemaphoreExit)); } static class GateState { public const int Locked = 0; public const int SignalPending = 1; public const int Unlocked = 2; public const int Signalled = 3; } } [Fx.Tag.SynchronizationPrimitive(Fx.Tag.BlocksUsing.NonBlocking)] class SignalGate : SignalGate { T result; public SignalGate() : base() { } public bool Signal(T result) { this.result = result; return Signal(); } public bool Unlock(out T result) { if (Unlock()) { result = this.result; return true; } result = default(T); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
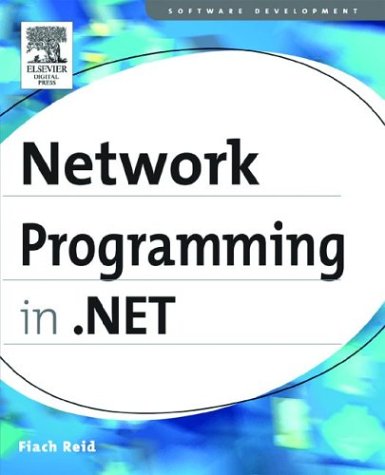
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HexParser.cs
- Interfaces.cs
- PageRouteHandler.cs
- ReflectionServiceProvider.cs
- ContentWrapperAttribute.cs
- FaultDescription.cs
- XmlDataSourceNodeDescriptor.cs
- ConsumerConnectionPoint.cs
- __ConsoleStream.cs
- sqlcontext.cs
- BindToObject.cs
- XamlRtfConverter.cs
- ResourceCategoryAttribute.cs
- RuntimeEnvironment.cs
- AsnEncodedData.cs
- AspProxy.cs
- DesignerActionHeaderItem.cs
- RichTextBox.cs
- StreamGeometry.cs
- DrawToolTipEventArgs.cs
- DataShape.cs
- OutputCacheModule.cs
- RewritingSimplifier.cs
- TimeEnumHelper.cs
- FlowPosition.cs
- ErrorHandlingReceiver.cs
- ScriptMethodAttribute.cs
- ItemsControlAutomationPeer.cs
- EpmSyndicationContentSerializer.cs
- Version.cs
- TemplateNodeContextMenu.cs
- ListenerAdaptersInstallComponent.cs
- SemanticTag.cs
- BitmapEffectGeneralTransform.cs
- ExceptionAggregator.cs
- Version.cs
- ActiveXHelper.cs
- GenerateTemporaryAssemblyTask.cs
- SafeLibraryHandle.cs
- StructuralCache.cs
- EdmComplexPropertyAttribute.cs
- GotoExpression.cs
- MeshGeometry3D.cs
- ValidatorUtils.cs
- CommentEmitter.cs
- Vector3D.cs
- LambdaCompiler.Address.cs
- ArgumentException.cs
- TypeUtil.cs
- ViewBox.cs
- WindowsStartMenu.cs
- DataTablePropertyDescriptor.cs
- RuntimeConfig.cs
- HashAlgorithm.cs
- Convert.cs
- NumberFormatInfo.cs
- DataGridTextBox.cs
- TextEditorThreadLocalStore.cs
- HttpHeaderCollection.cs
- VirtualPathUtility.cs
- CollectionType.cs
- SqlMetaData.cs
- TableItemPattern.cs
- ProviderCommandInfoUtils.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- DecoderNLS.cs
- ActivityCodeDomSerializationManager.cs
- PageThemeCodeDomTreeGenerator.cs
- ActionFrame.cs
- Behavior.cs
- RegionIterator.cs
- Hashtable.cs
- LocalizableResourceBuilder.cs
- IdentifierCreationService.cs
- ActivityWithResultConverter.cs
- ServiceSettingsResponseInfo.cs
- DataGridViewComboBoxEditingControl.cs
- PortCache.cs
- DataGrid.cs
- DiagnosticSection.cs
- SignedInfo.cs
- CompensationExtension.cs
- InstanceNormalEvent.cs
- EntityProviderFactory.cs
- XmlCodeExporter.cs
- And.cs
- MultiplexingFormatMapping.cs
- Delegate.cs
- SelectionRangeConverter.cs
- Pool.cs
- ToolStripItemImageRenderEventArgs.cs
- DataMisalignedException.cs
- TimerElapsedEvenArgs.cs
- CodeTypeMember.cs
- SchemaImporter.cs
- CacheSection.cs
- PartBasedPackageProperties.cs
- RSAPKCS1SignatureDeformatter.cs
- counter.cs
- ProviderConnectionPoint.cs