Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / Configuration / ListenerAdaptersInstallComponent.cs / 1 / ListenerAdaptersInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install.Configuration { using WebAdmin = Microsoft.Web.Administration; using System; using System.ServiceModel; using System.Collections.Generic; using System.Configuration; using System.Globalization; using System.Reflection; using System.Security.Principal; using System.Text; using System.Web.Configuration; using System.Runtime.InteropServices; using System.ComponentModel; internal class ListenerAdaptersInstallComponent : ServiceModelInstallComponent { IIS7ConfigurationLoader configLoader; string displayString; string localServiceAccount; string name; ListenerAdaptersInstallComponent(string name, IIS7ConfigurationLoader configLoader) { if (!IisHelper.ShouldInstallWas || !IisHelper.ShouldInstallApplicationHost) { throw new WasNotInstalledException(SR.GetString(SR.WasNotInstalled, SR.GetString(SR.ListenerAdaptersName, name))); } this.name = name; // This account must match the same one in InstallServiceModelOperations.InstallOperations() if (!OSEnvironmentHelper.IsVistaOrGreater) { // this is not localized this.localServiceAccount = ServiceModelInstallStrings.LocalServiceAccountName; } else { // We use the hard-coded service SIDs because we cannot tell when the service is installed here. if (name.Equals(ServiceModelInstallStrings.NetTcp, StringComparison.OrdinalIgnoreCase)) { this.localServiceAccount = ServiceModelInstallStrings.Sid_NetTcpActivator; } else if (name.Equals(ServiceModelInstallStrings.NetPipe, StringComparison.OrdinalIgnoreCase)) { this.localServiceAccount = ServiceModelInstallStrings.Sid_NetPipeActivator; } else // if (name.Equals(ServiceModelInstallStrings.NetMsmq, StringComparison.OrdinalIgnoreCase) || name.Equals(ServiceModelInstallStrings.MsmqFormatName, StringComparison.OrdinalIgnoreCase)) { this.localServiceAccount = ServiceModelInstallStrings.Sid_NetMsmqActivator; } } this.configLoader = configLoader; } internal override string DisplayName { get {return this.displayString; } } protected override string InstallActionMessage { get {return SR.GetString(SR.ListenerAdaptersInstall, this.name); } } internal override string[] InstalledVersions { get { ListinstalledVersions = new List (); WebAdmin.ConfigurationElement listenerAdapter = this.GetListenerAdapterFromCollection(); if (null != listenerAdapter) { string identity = String.Empty; if (null != listenerAdapter.GetAttribute(ServiceModelInstallStrings.Identity)) { identity = (string)listenerAdapter.GetAttribute(ServiceModelInstallStrings.Identity).Value; } installedVersions.Add(SR.GetString(SR.ListenerAdaptersInstalledVersion, identity)); } return installedVersions.ToArray(); } } internal override bool IsInstalled { get { return (null != this.GetListenerAdapterFromCollection()); } } protected override string ReinstallActionMessage { get {return SR.GetString(SR.ListenerAdaptersReinstall, this.name); } } protected override string UninstallActionMessage { get {return SR.GetString(SR.ListenerAdaptersUninstall, this.name); } } internal static ListenerAdaptersInstallComponent CreateNativeListenerAdaptersInstallComponent(string name) { ListenerAdaptersInstallComponent listenerAdapters = new ListenerAdaptersInstallComponent(name, new IIS7ConfigurationLoader(new NativeConfigurationLoader())); listenerAdapters.displayString = SR.GetString(SR.ListenerAdaptersName, name); return listenerAdapters; } WebAdmin.ConfigurationElement GetListenerAdapterFromCollection() { WebAdmin.ConfigurationElement listenerAdapter = null; if (null != this.configLoader.ListenerAdaptersSection) { WebAdmin.ConfigurationElementCollection listenerAdaptersCollection = this.configLoader.ListenerAdaptersSection.GetCollection(); // ListenerAdaptersCollection does not have a unique key, thus need to enumerate collection rather than check for key foreach (WebAdmin.ConfigurationElement element in listenerAdaptersCollection) { if (((string)element.GetAttribute(ServiceModelInstallStrings.Name).Value).Equals(this.name, StringComparison.OrdinalIgnoreCase)) { listenerAdapter = element; break; } } } return listenerAdapter; } internal override void Install(OutputLevel outputLevel) { if (!this.IsInstalled) { if (null != this.configLoader.ListenerAdaptersSection) { WebAdmin.ConfigurationElementCollection listenerAdaptersCollection = this.configLoader.ListenerAdaptersSection.GetCollection(); WebAdmin.ConfigurationElement element = listenerAdaptersCollection.CreateElement(); element.GetAttribute(ServiceModelInstallStrings.Name).Value = this.name; element.GetAttribute(ServiceModelInstallStrings.Identity).Value = this.localServiceAccount; listenerAdaptersCollection.Add(element); configLoader.Save(); } else { throw new InvalidOperationException(SR.GetString(SR.IIS7ConfigurationSectionNotFound, this.configLoader.ListenerAdaptersSectionPath)); } } else { EventLogger.LogWarning(SR.GetString(SR.ListenerAdaptersAlreadyExists, name), OutputLevel.Verbose == outputLevel); } } internal override void Uninstall(OutputLevel outputLevel) { if (this.IsInstalled) { if (null != this.configLoader.ListenerAdaptersSection) { WebAdmin.ConfigurationElementCollection listenerAdaptersCollection = this.configLoader.ListenerAdaptersSection.GetCollection(); // ListenerAdaptersCollection does not have a unique key, thus need to enumerate collection rather than check for key // Also, Microsoft.Web.Administration.ConfigurationElementCollection.Remove(element) does not work here. It // translates (bug?) to RemoveAt(-1) which fails. Instead, get index manually and call RemoveAt(int) directly. for(int i = 0; i < listenerAdaptersCollection.Count; i++) { WebAdmin.ConfigurationElement element = listenerAdaptersCollection[i]; if (((string)element.GetAttribute(ServiceModelInstallStrings.Name).Value).Equals(this.name, StringComparison.OrdinalIgnoreCase)) { listenerAdaptersCollection.RemoveAt(i); configLoader.Save(); break; } } } } else { EventLogger.LogWarning(SR.GetString(SR.ListenerAdaptersNotInstalled, name), OutputLevel.Verbose == outputLevel); } } internal override InstallationState VerifyInstall() { InstallationState installState = InstallationState.Unknown; if (this.IsInstalled) { WebAdmin.ConfigurationElement listenerAdapter = this.GetListenerAdapterFromCollection(); if (null != listenerAdapter.GetAttribute(ServiceModelInstallStrings.Identity) && ((string)listenerAdapter.GetAttribute(ServiceModelInstallStrings.Identity).Value).Equals(this.localServiceAccount, StringComparison.OrdinalIgnoreCase)) { installState = InstallationState.InstalledDefaults; } else { installState = InstallationState.InstalledCustom; } } else { installState = InstallationState.NotInstalled; } return installState; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
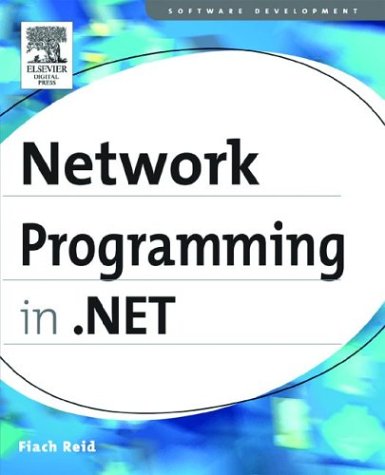
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TCEAdapterGenerator.cs
- ConnectionManagementElementCollection.cs
- ListItemConverter.cs
- ResourceDescriptionAttribute.cs
- DbConnectionStringBuilder.cs
- CodeDelegateInvokeExpression.cs
- WindowsPrincipal.cs
- DataPagerFieldItem.cs
- ProxyAttribute.cs
- NetworkInformationPermission.cs
- KeyboardDevice.cs
- Properties.cs
- WinEventQueueItem.cs
- HyperLinkColumn.cs
- CollectionChangedEventManager.cs
- AnnotationObservableCollection.cs
- WrappedIUnknown.cs
- ExpandCollapseProviderWrapper.cs
- HorizontalAlignConverter.cs
- XMLSchema.cs
- HebrewNumber.cs
- TypeSystem.cs
- LifetimeManager.cs
- DataBindingExpressionBuilder.cs
- ConfigurationFileMap.cs
- TypeExtension.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- Identifier.cs
- ExtentCqlBlock.cs
- Stylesheet.cs
- GridViewRow.cs
- PixelFormatConverter.cs
- RecognizerBase.cs
- PersonalizationProvider.cs
- SQLMoneyStorage.cs
- WithParamAction.cs
- FileReservationCollection.cs
- ExpressionEditorAttribute.cs
- SignatureToken.cs
- TextParagraphView.cs
- SqlConnectionPoolProviderInfo.cs
- DeferredSelectedIndexReference.cs
- ImageAnimator.cs
- XmlResolver.cs
- BrowserCapabilitiesCodeGenerator.cs
- DataGridCell.cs
- BamlLocalizableResourceKey.cs
- QueryInterceptorAttribute.cs
- TypeGeneratedEventArgs.cs
- ValueTypeFieldReference.cs
- StylusPointPropertyInfoDefaults.cs
- RegisteredDisposeScript.cs
- ControlIdConverter.cs
- DelegatingConfigHost.cs
- FileSystemInfo.cs
- PopupRoot.cs
- ObjectSet.cs
- RangeValidator.cs
- UpdateException.cs
- GeneratedCodeAttribute.cs
- AuthenticationSection.cs
- TimeZone.cs
- Formatter.cs
- DataRowChangeEvent.cs
- SqlConnectionFactory.cs
- IndependentlyAnimatedPropertyMetadata.cs
- WizardPanel.cs
- UnsafeNativeMethods.cs
- NotificationContext.cs
- TextEndOfSegment.cs
- TimelineGroup.cs
- errorpatternmatcher.cs
- ProviderCollection.cs
- CaseInsensitiveHashCodeProvider.cs
- WindowsListViewItemStartMenu.cs
- KnownTypesProvider.cs
- TagPrefixCollection.cs
- LinkButton.cs
- ExpressionBinding.cs
- IResourceProvider.cs
- GlyphRunDrawing.cs
- XmlNamedNodeMap.cs
- InfoCardTraceRecord.cs
- MulticastOption.cs
- IgnoreDeviceFilterElementCollection.cs
- ArrayConverter.cs
- Object.cs
- AdornerLayer.cs
- ApplicationDirectory.cs
- CompoundFileDeflateTransform.cs
- PropertySourceInfo.cs
- RoutedPropertyChangedEventArgs.cs
- IQueryable.cs
- ImageMetadata.cs
- TextBlock.cs
- AvTraceFormat.cs
- XmlQualifiedName.cs
- PassportAuthenticationModule.cs
- DnsPermission.cs
- Utils.cs