Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / QuadraticBezierSegment.cs / 1305600 / QuadraticBezierSegment.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: QuadraticBezierSegment.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Diagnostics; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// QuadraticBezierSegment /// public sealed partial class QuadraticBezierSegment : PathSegment { #region Constructors ////// /// public QuadraticBezierSegment() { } ////// /// public QuadraticBezierSegment(Point point1, Point point2, bool isStroked) { Point1 = point1; Point2 = point2; IsStroked = isStroked; } ////// /// internal QuadraticBezierSegment(Point point1, Point point2, bool isStroked, bool isSmoothJoin) { Point1 = point1; Point2 = point2; IsStroked = isStroked; IsSmoothJoin = isSmoothJoin; } #endregion #region AddToFigure internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // Out: Segment endpoint, not transformed { current = Point2; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { Point pt1 = Point1; pt1 *= matrix; Point pt2 = current; pt2 *= matrix; figure.Segments.Add(new QuadraticBezierSegment(pt1, pt2, IsStroked, IsSmoothJoin)); } } #endregion #region Resource ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.QuadraticBezierTo(Point1, Point2, IsStroked, IsSmoothJoin); } #endregion internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "Q{1:" + format + "}{0}{2:" + format + "}", separator, Point1, Point2); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
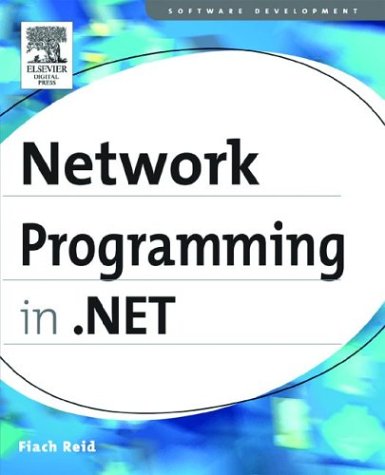
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridRelationshipRow.cs
- QilValidationVisitor.cs
- FrameworkContentElement.cs
- RecognizedAudio.cs
- AppliedDeviceFiltersDialog.cs
- PaginationProgressEventArgs.cs
- ThreadPool.cs
- Lease.cs
- Debug.cs
- TemplateComponentConnector.cs
- ExceptionHandler.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- ReadOnlyActivityGlyph.cs
- AmbiguousMatchException.cs
- XmlReflectionMember.cs
- XmlNavigatorFilter.cs
- DecimalConstantAttribute.cs
- StyleCollection.cs
- RuleSettingsCollection.cs
- PagerSettings.cs
- JoinElimination.cs
- ToolStripItem.cs
- GroupLabel.cs
- ReflectionTypeLoadException.cs
- HighlightVisual.cs
- SiteOfOriginContainer.cs
- CodeRemoveEventStatement.cs
- AuthenticationModulesSection.cs
- ObjectStateFormatter.cs
- CheckedListBox.cs
- ResourceExpressionEditorSheet.cs
- LoginCancelEventArgs.cs
- EditorPartCollection.cs
- TextEditorMouse.cs
- Int16Converter.cs
- TextClipboardData.cs
- BaseTemplateCodeDomTreeGenerator.cs
- EndpointReference.cs
- DataServiceQueryProvider.cs
- SqlCharStream.cs
- ProfileBuildProvider.cs
- XmlBinaryReaderSession.cs
- ContextStaticAttribute.cs
- BrowserCapabilitiesFactoryBase.cs
- ProjectionPlanCompiler.cs
- WebPartConnectionsCancelEventArgs.cs
- SystemResources.cs
- WeakEventTable.cs
- EasingQuaternionKeyFrame.cs
- DataGridViewCellCancelEventArgs.cs
- ButtonBase.cs
- ImageAttributes.cs
- DocobjHost.cs
- Compiler.cs
- XmlSchemaAnnotated.cs
- DetailsViewUpdateEventArgs.cs
- MessageAction.cs
- XsdValidatingReader.cs
- CapiSymmetricAlgorithm.cs
- OdbcConnectionFactory.cs
- TextureBrush.cs
- MappingSource.cs
- TextEditorSpelling.cs
- BasicCellRelation.cs
- SmtpNtlmAuthenticationModule.cs
- VideoDrawing.cs
- SchemaRegistration.cs
- Models.cs
- FormatConvertedBitmap.cs
- httpapplicationstate.cs
- ValidationError.cs
- Rotation3DKeyFrameCollection.cs
- VerificationException.cs
- ActivityExecutorSurrogate.cs
- SHA384Managed.cs
- SSmlParser.cs
- XmlNamespaceMappingCollection.cs
- TextBoxRenderer.cs
- ControlFilterExpression.cs
- TextTreeTextElementNode.cs
- FieldAccessException.cs
- ForeignConstraint.cs
- WindowsSolidBrush.cs
- RelationalExpressions.cs
- DataException.cs
- ElementUtil.cs
- BamlCollectionHolder.cs
- XslVisitor.cs
- DirectoryObjectSecurity.cs
- DataRelationPropertyDescriptor.cs
- WsatAdminException.cs
- TypeElementCollection.cs
- CompilerResults.cs
- WeakReferenceList.cs
- BodyWriter.cs
- EdmScalarPropertyAttribute.cs
- CompiledELinqQueryState.cs
- AttachedAnnotationChangedEventArgs.cs
- DateTimeValueSerializerContext.cs
- documentsequencetextpointer.cs