Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Routing / System / ServiceModel / Routing / SessionChannels.cs / 1305376 / SessionChannels.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Routing { using System; using System.Diagnostics.CodeAnalysis; using System.Collections.Generic; using System.Runtime; using System.ServiceModel.Description; using System.ServiceModel.Channels; using System.ComponentModel; using System.Threading; class SessionChannels { Guid activityID; [Fx.Tag.SynchronizationObject] Dictionarysessions; List sessionList; [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.AvoidUncalledPrivateCode, Justification = "gets called in RoutingService.ctor()")] public SessionChannels(Guid activityID) { this.activityID = activityID; this.sessions = new Dictionary (); this.sessionList = new List (); } void ChannelFaulted(object sender, EventArgs args) { FxTrace.Trace.SetAndTraceTransfer(this.activityID, true); IRoutingClient client = (IRoutingClient)sender; if (TD.RoutingServiceChannelFaultedIsEnabled()) { TD.RoutingServiceChannelFaulted(client.Key.ToString()); } this.AbortChannel(client.Key); } [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.AvoidUncalledPrivateCode, Justification = "BeginClose is called by RoutingChannelExtension.AttachService")] public IAsyncResult BeginClose(TimeSpan timeout, AsyncCallback callback, object state) { List localClients = null; lock(this.sessions) { if (this.sessions.Count > 0) { localClients = this.sessionList.ConvertAll ((client) => (ICommunicationObject)client); this.sessionList.Clear(); this.sessions.Clear(); } } if (localClients != null && localClients.Count > 0) { localClients.ForEach((client) => { if (TD.RoutingServiceClosingClientIsEnabled()) { TD.RoutingServiceClosingClient(((IRoutingClient)client).Key.ToString()); } }); return new CloseCollectionAsyncResult(timeout, callback, state, localClients); } else { return new CompletedAsyncResult(callback, state); } } public void EndClose(IAsyncResult asyncResult) { if (asyncResult is CloseCollectionAsyncResult) { CloseCollectionAsyncResult.End(asyncResult); } else { CompletedAsyncResult.End(asyncResult); } } internal IRoutingClient GetOrCreateClient (RoutingEndpointTrait key, RoutingService service, bool impersonating) { IRoutingClient value; lock (this.sessions) { if (!this.sessions.TryGetValue(key, out value)) { //Create the client here value = ClientFactory.Create(key, service, impersonating); value.Faulted += ChannelFaulted; this.sessions[key] = value; sessionList.Add(value); } } return value; } public void AbortAll() { List clients = new List (); lock (this.sessions) { foreach (IRoutingClient client in this.sessions.Values) { clients.Add(client); } this.sessions.Clear(); this.sessionList.Clear(); } foreach (IRoutingClient client in clients) { RoutingUtilities.Abort((ICommunicationObject)client, client.Key); } } public void AbortChannel(RoutingEndpointTrait key) { IRoutingClient client; lock (this.sessions) { if (this.sessions.TryGetValue(key, out client)) { this.sessions.Remove(key); this.sessionList.Remove(client); } } if (client != null) { RoutingUtilities.Abort((ICommunicationObject)client, client.Key); } } public IRoutingClient ReleaseChannel() { IRoutingClient client = null; lock (this.sessions) { int count = this.sessionList.Count; if (count > 0) { client = this.sessionList[count - 1]; this.sessionList.RemoveAt(count - 1); this.sessions.Remove(client.Key); } } return client; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
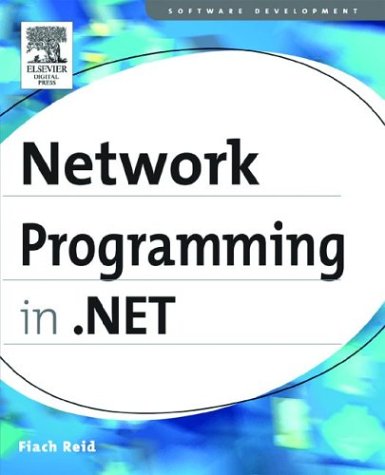
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerCustomResolverBindingElement.cs
- DataSourceHelper.cs
- Binding.cs
- ToolStripRendererSwitcher.cs
- DBBindings.cs
- ValidationSummary.cs
- FixedSchema.cs
- TableLayoutPanel.cs
- LayoutEvent.cs
- ResourceAttributes.cs
- EventMetadata.cs
- UIElementAutomationPeer.cs
- GridViewCommandEventArgs.cs
- dtdvalidator.cs
- _LazyAsyncResult.cs
- TemplateManager.cs
- ToolStripContainer.cs
- ComPlusSynchronizationContext.cs
- OciHandle.cs
- AstTree.cs
- Timer.cs
- CachedFontFamily.cs
- VideoDrawing.cs
- StackSpiller.Bindings.cs
- MenuStrip.cs
- TransformerConfigurationWizardBase.cs
- DoubleAnimationClockResource.cs
- DataGridTextColumn.cs
- GridView.cs
- EdmFunctionAttribute.cs
- MetadataArtifactLoaderComposite.cs
- ElementProxy.cs
- FilteredDataSetHelper.cs
- ResourceFallbackManager.cs
- ErrorWebPart.cs
- UTF7Encoding.cs
- InternalDispatchObject.cs
- StrokeFIndices.cs
- EventMemberCodeDomSerializer.cs
- XPathChildIterator.cs
- Accessible.cs
- InsufficientMemoryException.cs
- Size.cs
- Site.cs
- Scene3D.cs
- GlobalProxySelection.cs
- FieldBuilder.cs
- XmlSignatureManifest.cs
- XmlDataLoader.cs
- ZipIOLocalFileHeader.cs
- CellParagraph.cs
- MenuItemBinding.cs
- TypeUtils.cs
- IODescriptionAttribute.cs
- AndCondition.cs
- MultiBinding.cs
- DbFunctionCommandTree.cs
- TraceListeners.cs
- FormatterServices.cs
- DefaultMemberAttribute.cs
- TextStore.cs
- ComponentResourceKeyConverter.cs
- XmlSchemaCollection.cs
- ConnectionStringSettings.cs
- ActiveXHost.cs
- entityreference_tresulttype.cs
- FramingDecoders.cs
- FieldToken.cs
- Module.cs
- ActivityValidator.cs
- DataGridViewCellEventArgs.cs
- HybridDictionary.cs
- CultureInfo.cs
- XmlSchemas.cs
- Schema.cs
- CharUnicodeInfo.cs
- BinaryCommonClasses.cs
- QueuePropertyVariants.cs
- Bidi.cs
- UniqueEventHelper.cs
- DesignerAutoFormat.cs
- XmlMtomWriter.cs
- Literal.cs
- CroppedBitmap.cs
- FrameworkElementFactoryMarkupObject.cs
- AttributedMetaModel.cs
- PathFigureCollectionValueSerializer.cs
- KerberosSecurityTokenAuthenticator.cs
- StatusBarPanel.cs
- XmlIgnoreAttribute.cs
- TableHeaderCell.cs
- SortedDictionary.cs
- PartialList.cs
- EntryPointNotFoundException.cs
- UiaCoreApi.cs
- CodeDOMUtility.cs
- PasswordRecoveryDesigner.cs
- TypeToken.cs
- SymbolTable.cs
- FormsAuthentication.cs