Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / CroppedBitmap.cs / 1 / CroppedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: CroppedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region CroppedBitmap ////// CroppedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class CroppedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public CroppedBitmap() : base(true) { } ////// Construct a CroppedBitmap /// /// BitmapSource to apply to the crop to /// Source rect of the bitmap to use public CroppedBitmap(BitmapSource source, Int32Rect sourceRect) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } _bitmapInit.BeginInit(); Source = source; SourceRect = sourceRect; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } FinalizeCreation(); } private void ClonePrequel(CroppedBitmap otherCroppedBitmap) { BeginInit(); } private void ClonePostscript(CroppedBitmap otherCroppedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicClipper = null; Int32Rect rect = SourceRect; BitmapSource source = Source; if (rect.IsEmpty) { rect.Width = source.PixelWidth; rect.Height = source.PixelHeight; } using (FactoryMaker factoryMaker = new FactoryMaker()) { try { IntPtr wicFactory = factoryMaker.ImagingFactoryPtr; HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapClipper( wicFactory, out wicClipper)); lock (_syncObject) { HRESULT.Check(UnsafeNativeMethods.WICBitmapClipper.Initialize( wicClipper, source.WicSourceHandle, ref rect)); } WicSourceHandle = wicClipper; _isSourceCached = source.IsSourceCached; wicClipper = null; } catch { _bitmapInit.Reset(); throw; } finally { if (wicClipper != null) wicClipper.Close(); } } CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } ////// Notification on source rect changing. /// private void SourceRectPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceRect = (Int32Rect)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceRect /// private static object CoerceSourceRect(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceRect; } else { return value; } } #region Data members private BitmapSource _source; private Int32Rect _sourceRect; #endregion } #endregion // CroppedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: CroppedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region CroppedBitmap ////// CroppedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class CroppedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public CroppedBitmap() : base(true) { } ////// Construct a CroppedBitmap /// /// BitmapSource to apply to the crop to /// Source rect of the bitmap to use public CroppedBitmap(BitmapSource source, Int32Rect sourceRect) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } _bitmapInit.BeginInit(); Source = source; SourceRect = sourceRect; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } FinalizeCreation(); } private void ClonePrequel(CroppedBitmap otherCroppedBitmap) { BeginInit(); } private void ClonePostscript(CroppedBitmap otherCroppedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicClipper = null; Int32Rect rect = SourceRect; BitmapSource source = Source; if (rect.IsEmpty) { rect.Width = source.PixelWidth; rect.Height = source.PixelHeight; } using (FactoryMaker factoryMaker = new FactoryMaker()) { try { IntPtr wicFactory = factoryMaker.ImagingFactoryPtr; HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapClipper( wicFactory, out wicClipper)); lock (_syncObject) { HRESULT.Check(UnsafeNativeMethods.WICBitmapClipper.Initialize( wicClipper, source.WicSourceHandle, ref rect)); } WicSourceHandle = wicClipper; _isSourceCached = source.IsSourceCached; wicClipper = null; } catch { _bitmapInit.Reset(); throw; } finally { if (wicClipper != null) wicClipper.Close(); } } CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } ////// Notification on source rect changing. /// private void SourceRectPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceRect = (Int32Rect)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceRect /// private static object CoerceSourceRect(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceRect; } else { return value; } } #region Data members private BitmapSource _source; private Int32Rect _sourceRect; #endregion } #endregion // CroppedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
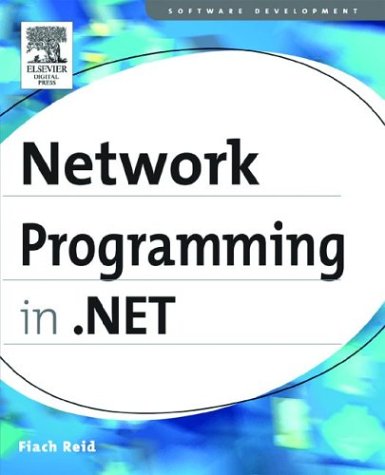
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImageFormatConverter.cs
- HTTPNotFoundHandler.cs
- BridgeDataReader.cs
- QueryOpeningEnumerator.cs
- ConnectionManagementSection.cs
- ProfilePropertySettingsCollection.cs
- TimeoutHelper.cs
- XPathDocumentIterator.cs
- ReliableSession.cs
- ListViewSortEventArgs.cs
- ResXResourceReader.cs
- DataGridViewSelectedRowCollection.cs
- PassportAuthenticationModule.cs
- SqlTriggerContext.cs
- TypeConverter.cs
- Operator.cs
- SerialPort.cs
- QilName.cs
- ManagementObjectCollection.cs
- KeyEventArgs.cs
- UnitySerializationHolder.cs
- InputManager.cs
- TemplatePropertyEntry.cs
- AndCondition.cs
- LinqToSqlWrapper.cs
- TypeHelpers.cs
- KeyValueInternalCollection.cs
- sqlcontext.cs
- AutomationProperty.cs
- DiscoveryDocumentSearchPattern.cs
- GlobalItem.cs
- ServiceDeploymentInfo.cs
- PropertyExpression.cs
- RuntimeConfigLKG.cs
- CapabilitiesPattern.cs
- BaseAddressPrefixFilterElement.cs
- WindowsListViewItemStartMenu.cs
- UniqueCodeIdentifierScope.cs
- SchemaElementLookUpTableEnumerator.cs
- Point.cs
- HttpModulesSection.cs
- ToolStripItemRenderEventArgs.cs
- InfoCardTraceRecord.cs
- JsonReader.cs
- HtmlInputReset.cs
- StatusBarItem.cs
- TextSpan.cs
- GeneralTransform3DGroup.cs
- HtmlProps.cs
- AggregateNode.cs
- MediaContextNotificationWindow.cs
- StreamSecurityUpgradeInitiatorBase.cs
- _LocalDataStoreMgr.cs
- XmlWriterTraceListener.cs
- BrushMappingModeValidation.cs
- bindurihelper.cs
- XmlSchemaRedefine.cs
- TreeNodeConverter.cs
- TaiwanLunisolarCalendar.cs
- DesignTimeTemplateParser.cs
- TextServicesDisplayAttribute.cs
- Thread.cs
- DbFunctionCommandTree.cs
- ToolStripMenuItem.cs
- CancellationState.cs
- IntegerValidator.cs
- StringUtil.cs
- SqlFileStream.cs
- DesignerActionUI.cs
- OperatingSystem.cs
- AsyncPostBackErrorEventArgs.cs
- Color.cs
- DefaultExpression.cs
- SafeSerializationManager.cs
- PersistenceException.cs
- TrackingMemoryStream.cs
- TaskExceptionHolder.cs
- XmlChoiceIdentifierAttribute.cs
- SqlMethodTransformer.cs
- WriteFileContext.cs
- DataGridViewCheckBoxColumn.cs
- BevelBitmapEffect.cs
- MouseBinding.cs
- PropertyMetadata.cs
- MetadataUtil.cs
- OutOfProcStateClientManager.cs
- ScalarOps.cs
- DataGridComboBoxColumn.cs
- DataMisalignedException.cs
- MissingFieldException.cs
- LicenseContext.cs
- QueryContinueDragEvent.cs
- DataStorage.cs
- XmlDictionaryString.cs
- PinnedBufferMemoryStream.cs
- SafeSystemMetrics.cs
- SplitterCancelEvent.cs
- TemplatePartAttribute.cs
- ProxyWebPartConnectionCollection.cs
- XmlNavigatorStack.cs