Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / FontCache / CachedFontFamily.cs / 1 / CachedFontFamily.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The CachedFontFamily class // // History: // 03/04/2004 : mleonov - Cache layout and interface changes for font enumeration. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Markup; // for XmlLanguage using System.Windows.Media; using MS.Win32; using MS.Utility; using MS.Internal; using MS.Internal.FontFace; namespace MS.Internal.FontCache { ////// This structure exists because we need a common wrapper for enumeration, but we can't use original cache structures: /// 1. C# doesn't allow IEnumerable/IEnumerator on pointer. /// 2. The cache structures don't inherit from base class. /// internal struct CachedFontFamily : IEnumerable{ private FamilyCollection _familyCollection; /// /// Critical: This holds reference to a pointer which is not ok to expose /// [SecurityCritical] private unsafe FamilyCollection.CachedFamily * _family; ////// Critical: Determines value of CheckedPointer.Size, which is used for bounds checking. /// [SecurityCritical] private int _sizeInBytes; ////// Critical: This stores a pointer and the class is unsafe because it manipulates the pointer; the sizeInBytes /// parameter is critical because it is used for bounds checking (via CheckedPointer) /// [SecurityCritical] public unsafe CachedFontFamily(FamilyCollection familyCollection, FamilyCollection.CachedFamily* family, int sizeInBytes) { _familyCollection = familyCollection; _family = family; _sizeInBytes = sizeInBytes; } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsNull { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family == null; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsPhysical { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->familyType == FamilyCollection.FamilyType.Physical; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsComposite { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->familyType == FamilyCollection.FamilyType.Composite; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public string OrdinalName { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _familyCollection.GetFamilyName(_family); } } } ////// Critical: This acccesses a pointer and returns a pointer to a structure /// public unsafe FamilyCollection.CachedPhysicalFamily* PhysicalFamily { [SecurityCritical] get { Invariant.Assert(IsPhysical); return (FamilyCollection.CachedPhysicalFamily *)_family; } } ////// Critical: This acccesses a pointer and returns a pointer to a structure /// public unsafe FamilyCollection.CachedCompositeFamily* CompositeFamily { [SecurityCritical] get { Invariant.Assert(IsComposite); return (FamilyCollection.CachedCompositeFamily *)_family; } } ////// Critical: Accesses critical fields and constructs a CheckedPointer which is a critical operation. /// TreatAsSafe: The fields used to construct the CheckedPointer are marked critical and CheckedPointer /// itself is safe to expose. /// public CheckedPointer CheckedPointer { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return new CheckedPointer(_family, _sizeInBytes); } } } public FamilyCollection FamilyCollection { get { return _familyCollection; } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public int NumberOfFaces { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->numberOfTypefaces; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public double Baseline { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->baseline; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public double LineSpacing { [SecurityCritical, SecurityTreatAsSafe] get { unsafe { return _family->lineSpacing; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public IDictionaryNames { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _familyCollection.GetLocalizedNameDictionary(_family); } } } #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private unsafe struct Enumerator : IEnumerator { private int _currentFace; private CachedFontFamily _family; public Enumerator(CachedFontFamily family) { _family = family; _currentFace = -1; } #region IEnumerator Members /// /// Critical: This acccesses a pointer /// TreatAsSafe: This funtions moves to the next /// [SecurityCritical,SecurityTreatAsSafe] public bool MoveNext() { ++_currentFace; if (0 <= _currentFace && _currentFace < _family.NumberOfFaces) return true; _currentFace = _family.NumberOfFaces; return false; } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// CachedFontFace IEnumerator.Current { [SecurityCritical,SecurityTreatAsSafe] get { return _family.FamilyCollection.GetCachedFace(_family, _currentFace); } } #endregion #region IEnumerator Members object IEnumerator.Current { get { return ((IEnumerator )this).Current; } } void IEnumerator.Reset() { _currentFace = -1; } #endregion #region IDisposable Members public void Dispose() {} #endregion } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The CachedFontFamily class // // History: // 03/04/2004 : mleonov - Cache layout and interface changes for font enumeration. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Markup; // for XmlLanguage using System.Windows.Media; using MS.Win32; using MS.Utility; using MS.Internal; using MS.Internal.FontFace; namespace MS.Internal.FontCache { ////// This structure exists because we need a common wrapper for enumeration, but we can't use original cache structures: /// 1. C# doesn't allow IEnumerable/IEnumerator on pointer. /// 2. The cache structures don't inherit from base class. /// internal struct CachedFontFamily : IEnumerable{ private FamilyCollection _familyCollection; /// /// Critical: This holds reference to a pointer which is not ok to expose /// [SecurityCritical] private unsafe FamilyCollection.CachedFamily * _family; ////// Critical: Determines value of CheckedPointer.Size, which is used for bounds checking. /// [SecurityCritical] private int _sizeInBytes; ////// Critical: This stores a pointer and the class is unsafe because it manipulates the pointer; the sizeInBytes /// parameter is critical because it is used for bounds checking (via CheckedPointer) /// [SecurityCritical] public unsafe CachedFontFamily(FamilyCollection familyCollection, FamilyCollection.CachedFamily* family, int sizeInBytes) { _familyCollection = familyCollection; _family = family; _sizeInBytes = sizeInBytes; } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsNull { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family == null; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsPhysical { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->familyType == FamilyCollection.FamilyType.Physical; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public bool IsComposite { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->familyType == FamilyCollection.FamilyType.Composite; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public string OrdinalName { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _familyCollection.GetFamilyName(_family); } } } ////// Critical: This acccesses a pointer and returns a pointer to a structure /// public unsafe FamilyCollection.CachedPhysicalFamily* PhysicalFamily { [SecurityCritical] get { Invariant.Assert(IsPhysical); return (FamilyCollection.CachedPhysicalFamily *)_family; } } ////// Critical: This acccesses a pointer and returns a pointer to a structure /// public unsafe FamilyCollection.CachedCompositeFamily* CompositeFamily { [SecurityCritical] get { Invariant.Assert(IsComposite); return (FamilyCollection.CachedCompositeFamily *)_family; } } ////// Critical: Accesses critical fields and constructs a CheckedPointer which is a critical operation. /// TreatAsSafe: The fields used to construct the CheckedPointer are marked critical and CheckedPointer /// itself is safe to expose. /// public CheckedPointer CheckedPointer { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return new CheckedPointer(_family, _sizeInBytes); } } } public FamilyCollection FamilyCollection { get { return _familyCollection; } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public int NumberOfFaces { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->numberOfTypefaces; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public double Baseline { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _family->baseline; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public double LineSpacing { [SecurityCritical, SecurityTreatAsSafe] get { unsafe { return _family->lineSpacing; } } } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// public IDictionaryNames { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _familyCollection.GetLocalizedNameDictionary(_family); } } } #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private unsafe struct Enumerator : IEnumerator { private int _currentFace; private CachedFontFamily _family; public Enumerator(CachedFontFamily family) { _family = family; _currentFace = -1; } #region IEnumerator Members /// /// Critical: This acccesses a pointer /// TreatAsSafe: This funtions moves to the next /// [SecurityCritical,SecurityTreatAsSafe] public bool MoveNext() { ++_currentFace; if (0 <= _currentFace && _currentFace < _family.NumberOfFaces) return true; _currentFace = _family.NumberOfFaces; return false; } ////// Critical: This acccesses a pointer /// TreatAsSafe: This information is ok to expose /// CachedFontFace IEnumerator.Current { [SecurityCritical,SecurityTreatAsSafe] get { return _family.FamilyCollection.GetCachedFace(_family, _currentFace); } } #endregion #region IEnumerator Members object IEnumerator.Current { get { return ((IEnumerator )this).Current; } } void IEnumerator.Reset() { _currentFace = -1; } #endregion #region IDisposable Members public void Dispose() {} #endregion } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
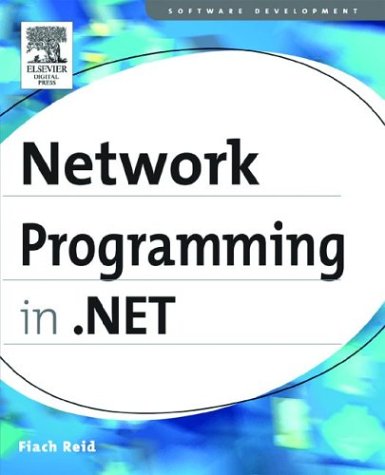
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Setter.cs
- NamespaceCollection.cs
- DmlSqlGenerator.cs
- HyperLinkStyle.cs
- TrackingProfile.cs
- BitmapSourceSafeMILHandle.cs
- AuthenticationConfig.cs
- PointLight.cs
- EmissiveMaterial.cs
- WebConfigurationHostFileChange.cs
- SQLRoleProvider.cs
- WindowsIPAddress.cs
- CodeTypeReference.cs
- FilterUserControlBase.cs
- OutKeywords.cs
- XmlDocumentFragment.cs
- Int32RectConverter.cs
- SparseMemoryStream.cs
- ZipArchive.cs
- EventLogInternal.cs
- PrivilegeNotHeldException.cs
- Path.cs
- DataTableCollection.cs
- ProfileProvider.cs
- PriorityItem.cs
- DrawToolTipEventArgs.cs
- CriticalFinalizerObject.cs
- ToolStripStatusLabel.cs
- ParameterToken.cs
- LinqDataSourceDisposeEventArgs.cs
- PrintDialogDesigner.cs
- ContextMenuAutomationPeer.cs
- ListItemCollection.cs
- Unit.cs
- StandardCommands.cs
- Error.cs
- QueueNameHelper.cs
- ChannelEndpointElementCollection.cs
- HostedNamedPipeTransportManager.cs
- JsonQueryStringConverter.cs
- InputLangChangeRequestEvent.cs
- GeneralTransform2DTo3D.cs
- EntityDataSourceWrapper.cs
- Content.cs
- Odbc32.cs
- DataGridTable.cs
- WebPartVerb.cs
- RawMouseInputReport.cs
- input.cs
- Point4DValueSerializer.cs
- WebPartZoneBase.cs
- DefaultTextStoreTextComposition.cs
- SourceFilter.cs
- RotateTransform.cs
- PerformanceCounterManager.cs
- Brush.cs
- WSMessageEncoding.cs
- BufferBuilder.cs
- TextElementEnumerator.cs
- SpoolingTaskBase.cs
- HttpPostProtocolReflector.cs
- SchemaTableColumn.cs
- Figure.cs
- IResourceProvider.cs
- EventWaitHandleSecurity.cs
- ContentDisposition.cs
- DocumentViewerHelper.cs
- ConfigurationPermission.cs
- ProcessingInstructionAction.cs
- BindingContext.cs
- BufferedGraphicsManager.cs
- FormsAuthenticationUserCollection.cs
- Vars.cs
- SqlDependencyListener.cs
- DeploymentSectionCache.cs
- BamlResourceContent.cs
- SqlClientMetaDataCollectionNames.cs
- ConnectionStringSettingsCollection.cs
- EncryptedData.cs
- ConnectionInterfaceCollection.cs
- BinarySerializer.cs
- SQLString.cs
- SiteOfOriginPart.cs
- ServiceSettingsResponseInfo.cs
- SizeConverter.cs
- CodeAttachEventStatement.cs
- WebServiceFault.cs
- FamilyMap.cs
- TransactionFlowAttribute.cs
- FormViewUpdatedEventArgs.cs
- XmlSerializationWriter.cs
- PointConverter.cs
- ProfilePropertyMetadata.cs
- HorizontalAlignConverter.cs
- OledbConnectionStringbuilder.cs
- Token.cs
- HttpCookieCollection.cs
- ProfileProvider.cs
- PropertyBuilder.cs
- GridViewSelectEventArgs.cs