Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / Binding.cs / 1 / Binding.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the bindings used for WS-Coor / WS-AT / .NET-AT port types using System; using System.Collections.Generic; using System.Net; using System.Net.Security; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Security; using System.Text; using Microsoft.Transactions.Wsat.Protocol; using Microsoft.Transactions.Bridge; namespace Microsoft.Transactions.Wsat.Messaging { abstract class CoordinationBinding : Binding { ProtocolVersion protocolVersion; // Calling CreateFault on an incoming message can expose some DoS-related security // vulnerabilities when a service is in streaming mode. See MB 47592 for more details. // The WS-AT protocol service does not use streaming mode on any of its bindings, so the // message we have in hand has already passed the binding�s MaxReceivedMessageSize check. // int.MaxValue may be a safe value, but it will require a formal review. internal const int MaxFaultSize = (int)TransportDefaults.MaxReceivedMessageSize; protected CoordinationBinding(string name, string ns, ProtocolVersion protocolVersion) : base(name, ns) { this.protocolVersion = protocolVersion; } public override string Scheme { get { return Uri.UriSchemeHttps; } } protected void AddTransactionFlowBindingElement(BindingElementCollection bindingElements) { // The TransactionFlowBindingElement is only used for IssuedTokens and IssuedTokens // require a TransactionProtocol in order to function correctly... TransactionProtocol protocol = (protocolVersion == ProtocolVersion.Version10) ? TransactionProtocol.WSAtomicTransactionOctober2004 : TransactionProtocol.WSAtomicTransaction11; TransactionFlowBindingElement txFlowBE = new TransactionFlowBindingElement(protocol); txFlowBE.Transactions = false; txFlowBE.IssuedTokens = TransactionFlowOption.Allowed; bindingElements.Add(txFlowBE); } protected void AddTransportSecurityBindingElement(BindingElementCollection bindingElements) { TransportSecurityBindingElement transportSecurity = new TransportSecurityBindingElement(); transportSecurity.MessageSecurityVersion = MessagingVersionHelper.SecurityVersion(this.protocolVersion); bindingElements.Add(transportSecurity); } protected void AddTextEncodingBindingElement(BindingElementCollection bindingElements) { TextMessageEncodingBindingElement encoding = new TextMessageEncodingBindingElement(); encoding.WriteEncoding = Encoding.UTF8; encoding.MessageVersion = MessagingVersionHelper.MessageVersion(this.protocolVersion); bindingElements.Add(encoding); } protected void AddCompositeDuplexBindingElement(BindingElementCollection bindingElements, Uri clientBaseAddress) { bindingElements.Add(new DuplexCorrelationBindingElement()); InternalDuplexBindingElement internalBE = new InternalDuplexBindingElement(true); bindingElements.Add(internalBE); CompositeDuplexBindingElement duplexBE = new CompositeDuplexBindingElement(); duplexBE.ClientBaseAddress = clientBaseAddress; bindingElements.Add(duplexBE); } protected void AddOneWayBindingElement(BindingElementCollection bindingElements) { bindingElements.Add(new OneWayBindingElement()); } protected void AddInteropHttpsTransportBindingElement(BindingElementCollection bindingElements) { HttpsTransportBindingElement httpsBE = new HttpsTransportBindingElement(); httpsBE.RequireClientCertificate = true; httpsBE.UseDefaultWebProxy = false; bindingElements.Add(httpsBE); } protected void AddNamedPipeBindingElement(BindingElementCollection bindingElements) { NamedPipeTransportBindingElement pipeTransportBE = new NamedPipeTransportBindingElement(); // See MB 32913 for details on how these numbers were chosen pipeTransportBE.MaxPendingConnections = 50; pipeTransportBE.ConnectionPoolSettings.MaxOutboundConnectionsPerEndpoint = 25; pipeTransportBE.MaxPendingAccepts = 25; bindingElements.Add(pipeTransportBE); } protected void AddWindowsStreamSecurityBindingElement(BindingElementCollection bindingElements) { WindowsStreamSecurityBindingElement windowsSecurityBE = new WindowsStreamSecurityBindingElement(); windowsSecurityBE.ProtectionLevel = ProtectionLevel.EncryptAndSign; bindingElements.Add(windowsSecurityBE); } public override BindingElementCollection CreateBindingElements() { BindingElementCollection bindingElements = new BindingElementCollection(); AddBindingElements(bindingElements); return bindingElements; } protected abstract void AddBindingElements(BindingElementCollection bindingElements); // class DuplexCorrelationBindingElement : BindingElement { public override BindingElement Clone() { return this; } public override T GetProperty(BindingContext context) { if (typeof(T) == typeof(ISecurityCapabilities)) { ISecurityCapabilities lowerCapabilities = context.GetInnerProperty (); if (lowerCapabilities != null) { // this binding can do server auth even over composite duplex return (T)(object)(new SecurityCapabilities(lowerCapabilities.SupportsClientAuthentication, true, lowerCapabilities.SupportsClientWindowsIdentity, lowerCapabilities.SupportedRequestProtectionLevel, lowerCapabilities.SupportedRequestProtectionLevel)); } else { return null; } } else { return context.GetInnerProperty (); } } } } class InteropRequestReplyBinding : CoordinationBinding { Uri clientBaseAddress; public InteropRequestReplyBinding(Uri clientBaseAddress, ProtocolVersion protocolVersion) : base(BindingStrings.InteropBindingName, AtomicTransactionStrings.Version(protocolVersion).Namespace, protocolVersion) { this.clientBaseAddress = clientBaseAddress; } protected override void AddBindingElements(BindingElementCollection bindingElements) { AddCompositeDuplexBindingElement(bindingElements, this.clientBaseAddress); AddOneWayBindingElement(bindingElements); AddTextEncodingBindingElement(bindingElements); AddInteropHttpsTransportBindingElement(bindingElements); } } class InteropRegistrationBinding : InteropRequestReplyBinding { SupportingTokenBindingElement supportingTokenBE; public InteropRegistrationBinding(Uri clientBaseAddress, bool acceptSupportingTokens, ProtocolVersion protocolVersion) : base(clientBaseAddress, protocolVersion) { if (acceptSupportingTokens) { this.supportingTokenBE = new SupportingTokenBindingElement(protocolVersion); } } public SupportingTokenBindingElement SupportingTokenBindingElement { get { return this.supportingTokenBE; } } protected override void AddBindingElements(BindingElementCollection bindingElements) { if (this.supportingTokenBE != null) { AddTransportSecurityBindingElement(bindingElements); bindingElements.Add(this.supportingTokenBE); } base.AddBindingElements(bindingElements); } } class InteropActivationBinding: InteropRequestReplyBinding { public InteropActivationBinding(Uri clientBaseAddress, ProtocolVersion protocolVersion) : base(clientBaseAddress, protocolVersion) { } protected override void AddBindingElements(BindingElementCollection bindingElements) { AddTransactionFlowBindingElement(bindingElements); base.AddBindingElements(bindingElements); } } class InteropDatagramBinding : CoordinationBinding { public InteropDatagramBinding(ProtocolVersion protocolVersion) : base(BindingStrings.InteropBindingName, AtomicTransactionStrings.Version(protocolVersion).Namespace, protocolVersion) { } protected override void AddBindingElements(BindingElementCollection bindingElements) { AddTextEncodingBindingElement(bindingElements); AddInteropHttpsTransportBindingElement(bindingElements); } } class NamedPipeBinding : CoordinationBinding { public NamedPipeBinding(ProtocolVersion protocolVersion) : base(BindingStrings.NamedPipeBindingName, DotNetAtomicTransactionExternalStrings.Namespace, protocolVersion) { } public override string Scheme { get { return Uri.UriSchemeNetPipe; } } protected override void AddBindingElements(BindingElementCollection bindingElements) { bindingElements.Add(new BinaryMessageEncodingBindingElement()); AddTransactionFlowBindingElement(bindingElements); AddWindowsStreamSecurityBindingElement(bindingElements); AddNamedPipeBindingElement(bindingElements); } } class WindowsRequestReplyBinding : CoordinationBinding { public WindowsRequestReplyBinding(ProtocolVersion protocolVersion) : base(BindingStrings.WindowsBindingName, DotNetAtomicTransactionExternalStrings.Namespace, protocolVersion) { } void AddWindowsHttpsTransportBindingElement(BindingElementCollection bindingElements) { HttpsTransportBindingElement httpsBE = new HttpsTransportBindingElement(); httpsBE.RequireClientCertificate = false; httpsBE.UseDefaultWebProxy = false; httpsBE.AuthenticationScheme = AuthenticationSchemes.Negotiate; bindingElements.Add(httpsBE); } protected override void AddBindingElements(BindingElementCollection bindingElements) { AddTransactionFlowBindingElement(bindingElements); AddTextEncodingBindingElement(bindingElements); AddWindowsHttpsTransportBindingElement(bindingElements); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
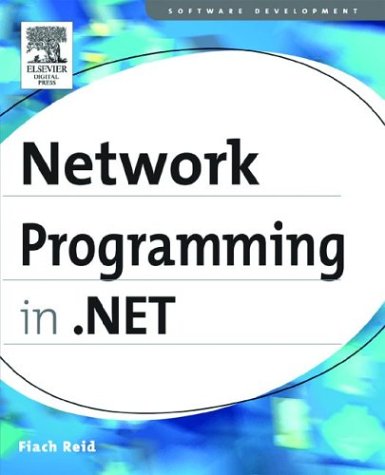
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeDirectiveCollection.cs
- TrackingLocation.cs
- TextRange.cs
- PageParserFilter.cs
- DesignerToolboxInfo.cs
- OperationCanceledException.cs
- EventHandlersStore.cs
- SimpleType.cs
- LogEntryUtils.cs
- UserPreferenceChangingEventArgs.cs
- ReceiveActivityValidator.cs
- WebPartConnection.cs
- ColorAnimation.cs
- Processor.cs
- SafeHandles.cs
- XomlCompilerParameters.cs
- TypeConstant.cs
- RepeaterCommandEventArgs.cs
- EntityClassGenerator.cs
- BitmapSizeOptions.cs
- BasePattern.cs
- XmlDataImplementation.cs
- HttpStreamXmlDictionaryWriter.cs
- DataGridViewHitTestInfo.cs
- ParseChildrenAsPropertiesAttribute.cs
- ServiceEndpointElementCollection.cs
- SafeNativeMethods.cs
- SettingsSection.cs
- Geometry.cs
- SafeEventHandle.cs
- SqlCommandBuilder.cs
- TreeViewDesigner.cs
- CallbackValidator.cs
- FileDetails.cs
- CookieProtection.cs
- ImageSourceConverter.cs
- SpanIndex.cs
- SystemFonts.cs
- MbpInfo.cs
- CodeArrayIndexerExpression.cs
- SocketException.cs
- TCPListener.cs
- webeventbuffer.cs
- SerializationHelper.cs
- QilReplaceVisitor.cs
- SqlDataSource.cs
- MemberCollection.cs
- DataTemplate.cs
- CachedBitmap.cs
- ClientTargetCollection.cs
- UpdatePanel.cs
- Imaging.cs
- ProcessHostConfigUtils.cs
- TextBoxRenderer.cs
- sqlstateclientmanager.cs
- RequestCachingSection.cs
- SqlWorkflowPersistenceService.cs
- CharKeyFrameCollection.cs
- IpcClientManager.cs
- MemoryFailPoint.cs
- NativeMethods.cs
- fixedPageContentExtractor.cs
- PopupRoot.cs
- BaseHashHelper.cs
- HttpRequest.cs
- DesignBinding.cs
- GC.cs
- BrowserCapabilitiesCompiler.cs
- ObjectStateFormatter.cs
- PointF.cs
- SchemaImporterExtensionElement.cs
- SmtpSection.cs
- ConfigsHelper.cs
- EventEntry.cs
- METAHEADER.cs
- HttpWebRequest.cs
- ConfigPathUtility.cs
- Events.cs
- SQLSingle.cs
- Constraint.cs
- figurelength.cs
- XmlSecureResolver.cs
- AudioFormatConverter.cs
- SoapAttributeOverrides.cs
- DateTime.cs
- DescendantOverDescendantQuery.cs
- MDIClient.cs
- IIS7WorkerRequest.cs
- ExtentKey.cs
- XPathSelectionIterator.cs
- DataGridViewSortCompareEventArgs.cs
- GrammarBuilderDictation.cs
- XmlDocument.cs
- DataSourceControlBuilder.cs
- BrowserInteropHelper.cs
- LogicalExpressionEditor.cs
- WebPartDisplayMode.cs
- InputDevice.cs
- TextCharacters.cs
- _ConnectStream.cs