Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / SymbolTable.cs / 2 / SymbolTable.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// The symbol table is quite primitive - it is a stack with a new entry for /// each scope. Lookups search from the top of the stack to the bottom, until /// an entry is found. /// /// The symbols are of the following kinds /// internal sealed class SymbolTable { private List///
/// /// Symbols represent names- ///
represents tables (extents/nested selects/unnests) - ///
represents Join nodes - ///
columns. to be resolved, /// or things to be renamed. /// > symbols = new List >(); internal void EnterScope() { symbols.Add(new Dictionary (StringComparer.OrdinalIgnoreCase)); } internal void ExitScope() { symbols.RemoveAt(symbols.Count - 1); } internal void Add(string name, Symbol value) { symbols[symbols.Count - 1][name] = value; } internal Symbol Lookup(string name) { for (int i = symbols.Count - 1; i >= 0; --i) { if (symbols[i].ContainsKey(name)) { return symbols[i][name]; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// The symbol table is quite primitive - it is a stack with a new entry for /// each scope. Lookups search from the top of the stack to the bottom, until /// an entry is found. /// /// The symbols are of the following kinds /// internal sealed class SymbolTable { private List///
/// /// Symbols represent names- ///
represents tables (extents/nested selects/unnests) - ///
represents Join nodes - ///
columns. to be resolved, /// or things to be renamed. /// > symbols = new List >(); internal void EnterScope() { symbols.Add(new Dictionary (StringComparer.OrdinalIgnoreCase)); } internal void ExitScope() { symbols.RemoveAt(symbols.Count - 1); } internal void Add(string name, Symbol value) { symbols[symbols.Count - 1][name] = value; } internal Symbol Lookup(string name) { for (int i = symbols.Count - 1; i >= 0; --i) { if (symbols[i].ContainsKey(name)) { return symbols[i][name]; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
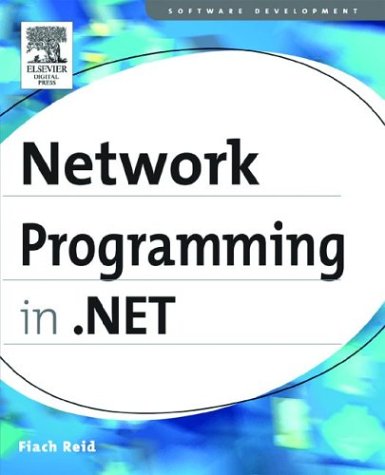
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMLineCollection.cs
- EdmType.cs
- GridViewCommandEventArgs.cs
- SqlCacheDependencyDatabase.cs
- DefaultValueAttribute.cs
- EntityCommandCompilationException.cs
- RequestChannelBinder.cs
- MaterialCollection.cs
- GacUtil.cs
- ResolvedKeyFrameEntry.cs
- DecimalAnimationBase.cs
- JsonFormatReaderGenerator.cs
- Codec.cs
- ManagementEventArgs.cs
- ProfileProvider.cs
- GridViewColumnHeaderAutomationPeer.cs
- BuilderElements.cs
- PersonalizationProviderHelper.cs
- OdbcConnectionHandle.cs
- AutoGeneratedField.cs
- WorkflowApplicationIdleEventArgs.cs
- ListBox.cs
- FileIOPermission.cs
- HttpServerVarsCollection.cs
- NullRuntimeConfig.cs
- XmlSchemaProviderAttribute.cs
- UniqueID.cs
- FormClosingEvent.cs
- GrammarBuilderWildcard.cs
- X509SubjectKeyIdentifierClause.cs
- ClientRoleProvider.cs
- PropertyReference.cs
- BitmapEffectRenderDataResource.cs
- ObjectCacheHost.cs
- CompositeControl.cs
- EventLog.cs
- LocalizedNameDescriptionPair.cs
- ManifestBasedResourceGroveler.cs
- TextParagraphCache.cs
- basecomparevalidator.cs
- ISSmlParser.cs
- RichTextBoxConstants.cs
- ConfigXmlText.cs
- ExecutionEngineException.cs
- VectorValueSerializer.cs
- ScriptDescriptor.cs
- XmlSchemaImporter.cs
- SessionIDManager.cs
- Interlocked.cs
- Color.cs
- Point3DCollectionValueSerializer.cs
- MenuBase.cs
- HealthMonitoringSection.cs
- MarshalDirectiveException.cs
- ExpressionVisitorHelpers.cs
- RadioButtonAutomationPeer.cs
- KeyTime.cs
- ListViewTableCell.cs
- RecognizedPhrase.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- StrokeIntersection.cs
- FullTextBreakpoint.cs
- DictionaryTraceRecord.cs
- InvalidAsynchronousStateException.cs
- ExtensionFile.cs
- HostExecutionContextManager.cs
- KeyMatchBuilder.cs
- CallbackTimeoutsElement.cs
- OptimizedTemplateContent.cs
- DataList.cs
- OperationParameterInfo.cs
- ConstantCheck.cs
- NullRuntimeConfig.cs
- Parameter.cs
- SafeTimerHandle.cs
- ExpressionNode.cs
- WebBrowserNavigatedEventHandler.cs
- WebControlsSection.cs
- RankException.cs
- CodePageEncoding.cs
- AspCompat.cs
- CfgParser.cs
- OleCmdHelper.cs
- StateItem.cs
- TemplateBindingExpressionConverter.cs
- DataGridViewAccessibleObject.cs
- CheckBoxStandardAdapter.cs
- HttpContextServiceHost.cs
- PersistenceException.cs
- DataColumnMappingCollection.cs
- XsltInput.cs
- TemplateField.cs
- ClientTargetSection.cs
- CustomAssemblyResolver.cs
- DefaultAssemblyResolver.cs
- EmptyEnumerator.cs
- StackOverflowException.cs
- XmlSchemaInclude.cs
- TypeUtil.cs
- PenLineCapValidation.cs