Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeIntersection.cs / 1 / StrokeIntersection.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
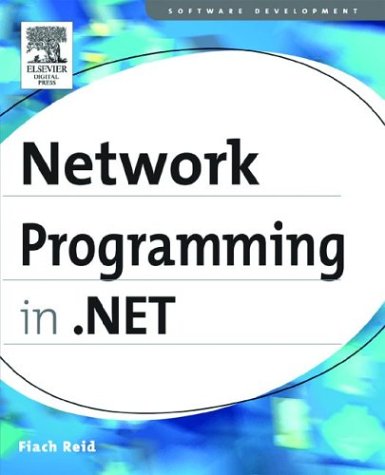
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RsaSecurityToken.cs
- FloaterParaClient.cs
- BufferedReadStream.cs
- ToolBar.cs
- UnaryNode.cs
- MouseBinding.cs
- ImageAttributes.cs
- X509SecurityTokenProvider.cs
- MailWriter.cs
- HwndSource.cs
- ContractNamespaceAttribute.cs
- BaseParagraph.cs
- InfoCardAsymmetricCrypto.cs
- ToolStripPanelRow.cs
- ChannelSinkStacks.cs
- UTF32Encoding.cs
- MatrixIndependentAnimationStorage.cs
- AuthenticationConfig.cs
- ServiceEndpointElement.cs
- DuplexChannel.cs
- HtmlInputImage.cs
- DocComment.cs
- ContentElement.cs
- DbDataSourceEnumerator.cs
- TemplateColumn.cs
- ImageKeyConverter.cs
- SQLDateTime.cs
- Guid.cs
- RestHandlerFactory.cs
- CultureTableRecord.cs
- ContextStaticAttribute.cs
- FontInfo.cs
- TemplateControlCodeDomTreeGenerator.cs
- LazyTextWriterCreator.cs
- DataGridViewCellValidatingEventArgs.cs
- TagMapCollection.cs
- HandlerWithFactory.cs
- TableAdapterManagerMethodGenerator.cs
- IndexOutOfRangeException.cs
- SmtpReplyReader.cs
- Fonts.cs
- dsa.cs
- SrgsDocument.cs
- StructuredTypeEmitter.cs
- TransactionChannelListener.cs
- WebPermission.cs
- IListConverters.cs
- DBDataPermission.cs
- MSG.cs
- AnimatedTypeHelpers.cs
- Listbox.cs
- ExtensibleClassFactory.cs
- EventLogPermissionAttribute.cs
- ISCIIEncoding.cs
- Operand.cs
- ContentFilePart.cs
- SrgsGrammar.cs
- IPAddressCollection.cs
- XmlDocumentType.cs
- FormatConvertedBitmap.cs
- HashRepartitionStream.cs
- ConfigXmlAttribute.cs
- TraceHwndHost.cs
- DataObject.cs
- SrgsNameValueTag.cs
- IconConverter.cs
- HttpRequest.cs
- ColorContextHelper.cs
- StateItem.cs
- ConfigurationElementCollection.cs
- HtmlInputImage.cs
- RuntimeResourceSet.cs
- ProxyGenerator.cs
- Geometry.cs
- Int32KeyFrameCollection.cs
- TextTreeUndoUnit.cs
- XmlSchemaAttributeGroup.cs
- CharKeyFrameCollection.cs
- ProxyAttribute.cs
- Int32Storage.cs
- ExpressionEvaluator.cs
- UnsupportedPolicyOptionsException.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- SoapEnumAttribute.cs
- ScriptRegistrationManager.cs
- SmiConnection.cs
- TreeViewImageIndexConverter.cs
- WebPartUserCapability.cs
- SoapHeaderAttribute.cs
- FileStream.cs
- XmlCDATASection.cs
- AnonymousIdentificationSection.cs
- MemberAssignmentAnalysis.cs
- ObsoleteAttribute.cs
- EndpointConfigContainer.cs
- DefaultPropertyAttribute.cs
- ValidatedControlConverter.cs
- sqlmetadatafactory.cs
- ExpressionWriter.cs
- UnsafeNativeMethods.cs