Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / ComponentModel / PropertyFilterAttribute.cs / 1305600 / PropertyFilterAttribute.cs
namespace System.ComponentModel { using System; using System.Diagnostics.CodeAnalysis; ////// This attribute is a "query" attribute. It is /// an attribute that causes the type description provider /// to narrow the scope of returned properties. It differs /// from normal attributes in that it cannot actually be /// placed on a class as metadata and that the filter mechanism /// is code rather than static metadata. /// [AttributeUsage(AttributeTargets.Property | AttributeTargets.Method)] public sealed class PropertyFilterAttribute : Attribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new attribute. /// public PropertyFilterAttribute(PropertyFilterOptions filter) { _filter = filter; } #endregion Constructors //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Override of Object.Equals that returns true if the filters /// contained in both attributes match. /// public override bool Equals(object value) { PropertyFilterAttribute a = value as PropertyFilterAttribute; if (a != null && a._filter.Equals(_filter)) { return true; } return false; } ////// Override of Object.GetHashCode. /// public override int GetHashCode() { return _filter.GetHashCode(); } ////// Match determines if one attribute "matches" another. For /// attributes that store flags, a match may be different from /// an equals. For example, a filter of SetValid matches a /// filter of All, because All is a merge of all filter values. /// public override bool Match(object value) { PropertyFilterAttribute a = value as PropertyFilterAttribute; if (a == null) return false; return ((_filter & a._filter) == _filter); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// The filter value passed into the constructor. /// public PropertyFilterOptions Filter { get { return _filter; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Fields // //----------------------------------------------------- #region Public Fields ////// Attributes may declare a Default field that indicates /// what should be done if the attribute is not defined. /// Our default is to return all properties. /// [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] public static readonly PropertyFilterAttribute Default = new PropertyFilterAttribute(PropertyFilterOptions.All); #endregion Public Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private PropertyFilterOptions _filter; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.ComponentModel { using System; using System.Diagnostics.CodeAnalysis; ////// This attribute is a "query" attribute. It is /// an attribute that causes the type description provider /// to narrow the scope of returned properties. It differs /// from normal attributes in that it cannot actually be /// placed on a class as metadata and that the filter mechanism /// is code rather than static metadata. /// [AttributeUsage(AttributeTargets.Property | AttributeTargets.Method)] public sealed class PropertyFilterAttribute : Attribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new attribute. /// public PropertyFilterAttribute(PropertyFilterOptions filter) { _filter = filter; } #endregion Constructors //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Override of Object.Equals that returns true if the filters /// contained in both attributes match. /// public override bool Equals(object value) { PropertyFilterAttribute a = value as PropertyFilterAttribute; if (a != null && a._filter.Equals(_filter)) { return true; } return false; } ////// Override of Object.GetHashCode. /// public override int GetHashCode() { return _filter.GetHashCode(); } ////// Match determines if one attribute "matches" another. For /// attributes that store flags, a match may be different from /// an equals. For example, a filter of SetValid matches a /// filter of All, because All is a merge of all filter values. /// public override bool Match(object value) { PropertyFilterAttribute a = value as PropertyFilterAttribute; if (a == null) return false; return ((_filter & a._filter) == _filter); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// The filter value passed into the constructor. /// public PropertyFilterOptions Filter { get { return _filter; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Fields // //----------------------------------------------------- #region Public Fields ////// Attributes may declare a Default field that indicates /// what should be done if the attribute is not defined. /// Our default is to return all properties. /// [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] public static readonly PropertyFilterAttribute Default = new PropertyFilterAttribute(PropertyFilterOptions.All); #endregion Public Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private PropertyFilterOptions _filter; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
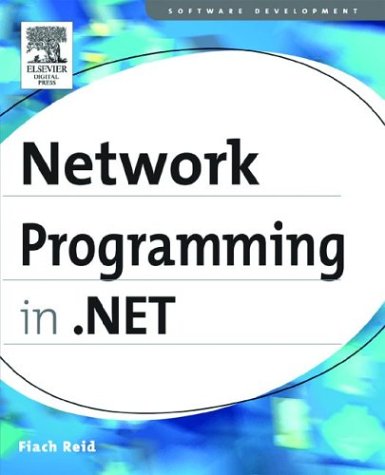
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostedAspNetEnvironment.cs
- NonParentingControl.cs
- ColorMap.cs
- WebSysDefaultValueAttribute.cs
- DocumentPageTextView.cs
- FrameworkObject.cs
- OperationAbortedException.cs
- RequestCacheEntry.cs
- AbstractSvcMapFileLoader.cs
- CheckBoxPopupAdapter.cs
- Command.cs
- NoPersistHandle.cs
- MethodMessage.cs
- FilterQueryOptionExpression.cs
- ModuleBuilder.cs
- IndentedTextWriter.cs
- SelectionRange.cs
- DocumentReferenceCollection.cs
- Icon.cs
- PipeConnection.cs
- GroupStyle.cs
- MenuCommands.cs
- SpeechSeg.cs
- XmlSiteMapProvider.cs
- Vector.cs
- XmlSchemaAnnotation.cs
- Odbc32.cs
- SlipBehavior.cs
- VersionUtil.cs
- ZipIOExtraField.cs
- ConnectionModeReader.cs
- ReachSerializationCacheItems.cs
- TTSEngineTypes.cs
- BufferAllocator.cs
- XMLSchema.cs
- MetadataFile.cs
- DataGridViewRowsAddedEventArgs.cs
- Int16AnimationBase.cs
- HttpProfileBase.cs
- CategoriesDocumentFormatter.cs
- PageEventArgs.cs
- SortQuery.cs
- ProviderUtil.cs
- FrameworkObject.cs
- AutomationPatternInfo.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- RuleSetBrowserDialog.cs
- Form.cs
- TemplateNodeContextMenu.cs
- FlowDocumentView.cs
- BamlBinaryReader.cs
- RequestCache.cs
- SecurityAlgorithmSuite.cs
- ValidationErrorCollection.cs
- SafeReversePInvokeHandle.cs
- ProtocolsConfiguration.cs
- SiteMapHierarchicalDataSourceView.cs
- GridErrorDlg.cs
- sqlmetadatafactory.cs
- BaseTemplatedMobileComponentEditor.cs
- HashSetDebugView.cs
- ContextMenuStrip.cs
- MemberRelationshipService.cs
- RepeaterItemEventArgs.cs
- TextBox.cs
- TypedElement.cs
- HorizontalAlignConverter.cs
- PageStatePersister.cs
- CompatibleComparer.cs
- StateManagedCollection.cs
- TreeViewItemAutomationPeer.cs
- HttpModulesSection.cs
- Vector3DCollectionConverter.cs
- ContractAdapter.cs
- SynthesizerStateChangedEventArgs.cs
- DataGridCellAutomationPeer.cs
- XmlTextAttribute.cs
- ExpressionPrinter.cs
- FieldAccessException.cs
- assertwrapper.cs
- ResourceWriter.cs
- Soap12ProtocolReflector.cs
- ScrollItemPatternIdentifiers.cs
- ToolBarTray.cs
- CompilationLock.cs
- ColumnWidthChangedEvent.cs
- StylusPointPropertyInfo.cs
- SqlInternalConnectionSmi.cs
- Region.cs
- LineServicesCallbacks.cs
- AssemblyBuilder.cs
- DataGridCaption.cs
- ThreadStateException.cs
- MetadataArtifactLoaderCompositeResource.cs
- IIS7UserPrincipal.cs
- ECDiffieHellman.cs
- ChangePasswordDesigner.cs
- SemanticAnalyzer.cs
- FixedSOMImage.cs
- DataGridViewCellValueEventArgs.cs