Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Mail / SmtpNegotiateAuthenticationModule.cs / 2 / SmtpNegotiateAuthenticationModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mail { using System; using System.Collections; using System.IO; using System.Net; using System.Security.Permissions; using System.ComponentModel; internal class SmtpNegotiateAuthenticationModule : ISmtpAuthenticationModule { Hashtable sessions = new Hashtable(); internal SmtpNegotiateAuthenticationModule() { } #region ISmtpAuthenticationModule Members // Security this method will access NetworkCredential properties that demand UnmanagedCode and Environment Permission [EnvironmentPermission(SecurityAction.Assert, Unrestricted=true)] [SecurityPermission(SecurityAction.Assert, Flags=SecurityPermissionFlag.UnmanagedCode)] public Authorization Authenticate(string challenge, NetworkCredential credential, object sessionCookie) { if(Logging.On)Logging.Enter(Logging.Web, this, "Authenticate", null); try { lock (this.sessions) { NTAuthentication clientContext = this.sessions[sessionCookie] as NTAuthentication; if (clientContext == null) { if(credential == null){ return null; } this.sessions[sessionCookie] = clientContext = new NTAuthentication(false,"Negotiate",credential,null,ContextFlags.Connection | ContextFlags.InitIntegrity); } byte[] byteResp; string resp = null; if (!clientContext.IsCompleted) { // If auth is not yet completed keep producing // challenge responses with GetOutgoingBlob SecurityStatus statusCode; byte[] decodedChallenge = null; if (challenge != null) { decodedChallenge = Convert.FromBase64String(challenge); } byteResp = clientContext.GetOutgoingBlob( decodedChallenge, false, out statusCode); // Note sure why this is here...keeping it. if (clientContext.IsCompleted && byteResp == null) { resp = "\r\n"; } if (byteResp != null) { resp = Convert.ToBase64String(byteResp); } } else { // If auth completed and still have a challenge then // server may be doing "correct" form of GSSAPI SASL. // Validate incoming and produce outgoing SASL security // layer negotiate message. resp = GetSecurityLayerOutgoingBlob( challenge, clientContext); } return new Authorization(resp, clientContext.IsCompleted); } } finally { if(Logging.On)Logging.Exit(Logging.Web, this, "Authenticate", null); } } public string AuthenticationType { get { return "gssapi"; } } public void CloseContext(object sessionCookie) { NTAuthentication clientContext = null; lock (sessions) { clientContext = sessions[sessionCookie] as NTAuthentication; if (clientContext != null) { sessions.Remove(sessionCookie); } } if (clientContext != null) { clientContext.CloseContext(); } } #endregion // Function for SASL security layer negotiation after // authorization completes. // // Returns null for failure, Base64 encoded string on // success. private string GetSecurityLayerOutgoingBlob( string challenge, NTAuthentication clientContext) { // must have a security layer challenge if (challenge == null) return null; // "unwrap" challenge byte[] input = Convert.FromBase64String(challenge); int len; try { len = clientContext.VerifySignature(input, 0, input.Length); } catch (Win32Exception) { // any decrypt failure is an auth failure return null; } // Per RFC 2222 Section 7.2.2: // the client should then expect the server to issue a // token in a subsequent challenge. The client passes // this token to GSS_Unwrap and interprets the first // octet of cleartext as a bit-mask specifying the // security layers supported by the server and the // second through fourth octets as the maximum size // output_message to send to the server. // Section 7.2.3 // The security layer and their corresponding bit-masks // are as follows: // 1 No security layer // 2 Integrity protection // Sender calls GSS_Wrap with conf_flag set to FALSE // 4 Privacy protection // Sender calls GSS_Wrap with conf_flag set to TRUE // // Exchange 2007 and our client only support // "No security layer". Therefore verify first byte is value 1 // and the 2nd-4th bytes are value zero since token size is not // applicable when there is no security layer. if (len < 4 || // expect 4 bytes input[0] != 1 || // first value 1 input[1] != 0 || // rest value 0 input[2] != 0 || input[3] != 0) { return null; } // Continuing with RFC 2222 section 7.2.2: // The client then constructs data, with the first octet // containing the bit-mask specifying the selected security // layer, the second through fourth octets containing in // network byte order the maximum size output_message the client // is able to receive, and the remaining octets containing the // authorization identity. // // So now this contructs the "wrapped" response. The response is // payload is identical to the received server payload and the // "authorization identity" is not supplied as it is unnecessary. // let MakeSignature figure out length of output byte[] output = null; try { len = clientContext.MakeSignature(input, 0, 4, ref output); } catch (Win32Exception) { // any decrypt failure is an auth failure return null; } // return Base64 encoded string of signed payload return Convert.ToBase64String(output, 0, len); } } }
Link Menu
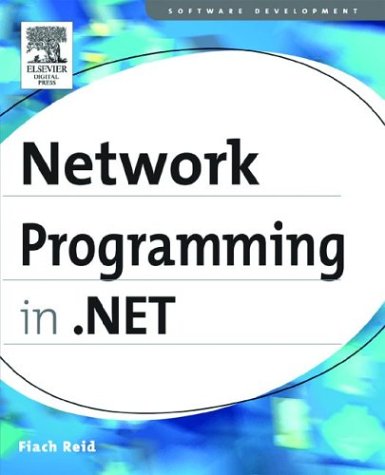
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeSpanMinutesOrInfiniteConverter.cs
- ValidationPropertyAttribute.cs
- XmlNamedNodeMap.cs
- ClientCultureInfo.cs
- EntityDataSourceSelectingEventArgs.cs
- StylusOverProperty.cs
- XmlSchemaSubstitutionGroup.cs
- QilStrConcat.cs
- Subtree.cs
- RequestQueue.cs
- WorkflowDesignerMessageFilter.cs
- filewebresponse.cs
- ObjectDataSourceEventArgs.cs
- BaseResourcesBuildProvider.cs
- BindingListCollectionView.cs
- Rect.cs
- FactoryGenerator.cs
- ModuleElement.cs
- DataGridViewTextBoxColumn.cs
- WindowsAltTab.cs
- EdmToObjectNamespaceMap.cs
- PolicyManager.cs
- PerfCounterSection.cs
- ThousandthOfEmRealPoints.cs
- TypedDataSourceCodeGenerator.cs
- DataSvcMapFile.cs
- ProcessModuleDesigner.cs
- AuthenticationSchemesHelper.cs
- DesignerObjectListAdapter.cs
- RoleService.cs
- ErrorHandler.cs
- TcpClientSocketManager.cs
- RegexInterpreter.cs
- IgnoreFlushAndCloseStream.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- WindowsFont.cs
- FormsAuthenticationUser.cs
- HtmlGenericControl.cs
- BindingExpressionBase.cs
- SchemaTypeEmitter.cs
- StoreItemCollection.cs
- SqlConnection.cs
- CaseCqlBlock.cs
- AttachedPropertyMethodSelector.cs
- DisplayInformation.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- XamlReaderHelper.cs
- Misc.cs
- RuntimeVariableList.cs
- RoutedEvent.cs
- DiagnosticsConfigurationHandler.cs
- AccessedThroughPropertyAttribute.cs
- PersonalizationProvider.cs
- SessionSwitchEventArgs.cs
- SlipBehavior.cs
- SQLRoleProvider.cs
- TypeUtils.cs
- HeaderUtility.cs
- CancellationHandler.cs
- XmlQueryCardinality.cs
- NamespaceEmitter.cs
- SettingsPropertyCollection.cs
- EngineSiteSapi.cs
- DBNull.cs
- GenerateTemporaryTargetAssembly.cs
- InputScopeManager.cs
- SafeSecurityHelper.cs
- ContactManager.cs
- MouseEvent.cs
- DeviceContext.cs
- DataControlFieldCell.cs
- MemoryFailPoint.cs
- GridViewColumnCollection.cs
- BreakRecordTable.cs
- WebRequestModulesSection.cs
- XamlVector3DCollectionSerializer.cs
- WebUtil.cs
- BuildManager.cs
- ScrollChrome.cs
- SqlDependencyListener.cs
- RecipientInfo.cs
- NonClientArea.cs
- WebPartZoneBase.cs
- RNGCryptoServiceProvider.cs
- CodePropertyReferenceExpression.cs
- VisualStyleInformation.cs
- HttpWrapper.cs
- PackagePartCollection.cs
- MembershipUser.cs
- DefinitionBase.cs
- ReflectionUtil.cs
- Expr.cs
- DocumentSequenceHighlightLayer.cs
- CaseInsensitiveHashCodeProvider.cs
- WebPartEditVerb.cs
- RowCache.cs
- DataColumnCollection.cs
- StoryFragments.cs
- TreeNodeSelectionProcessor.cs
- ProfileSettingsCollection.cs