Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / HttpProxyTransportBindingElement.cs / 2 / HttpProxyTransportBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.InfoCards { using System.Collections.Generic; using System.ServiceModel.Description; using System.ServiceModel; using System.Net; using System.ServiceModel.Channels; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class is used to replace the TransportBindingElement to a binding element which uses the user's proxy // class HttpProxyTransportBindingElement :TransportBindingElement { HttpTransportBindingElement innerHttpTransport; IWebProxy proxy; // // Summary: // Replaces the HttpTransportBindingElement from the collection with HttpProxyTransportBindingElement // // Arguments: // bindingElements: The bindingElements collection to update // proxy: The proxy to be used for HttpTransport // turnOffClientAuthOnTransport: When set to true, the AuthenticationScheme for the transport // binding will be set to ANonymous // // Returns: // Returns the updated bindingElements collection. // public static BindingElementCollection ReplaceHttpTransportWithProxy( BindingElementCollection bindingElements, IWebProxy proxy, bool turnOffClientAuthOnTransport ) { int httpIndex = -1; for( int i = 0; i < bindingElements.Count; i++ ) { if( bindingElements[ i ] is HttpTransportBindingElement ) { httpIndex = i; break; } } if( httpIndex == -1 ) { // no HttpTransport to wrap, just return original binding return bindingElements; } IDT.Assert( httpIndex == bindingElements.Count - 1, "Transport should be last in the Binding Element list" ); HttpTransportBindingElement httpTransport = ( HttpTransportBindingElement )bindingElements[ httpIndex ]; if( turnOffClientAuthOnTransport ) { httpTransport.AuthenticationScheme = AuthenticationSchemes.Anonymous; } HttpProxyTransportBindingElement proxyTransport = new HttpProxyTransportBindingElement( proxy, httpTransport ); bindingElements[ httpIndex ] = proxyTransport; return bindingElements; } HttpProxyTransportBindingElement( IWebProxy proxy, HttpTransportBindingElement innerHttpTransport ) : base() { this.innerHttpTransport = innerHttpTransport; this.proxy = proxy; } HttpProxyTransportBindingElement( HttpProxyTransportBindingElement elementToBeCloned ) : base( elementToBeCloned ) { this.innerHttpTransport = elementToBeCloned.innerHttpTransport; this.proxy = elementToBeCloned.proxy; } public override long MaxBufferPoolSize { get { return this.innerHttpTransport.MaxBufferPoolSize; } set { this.innerHttpTransport.MaxBufferPoolSize = value; } } public override long MaxReceivedMessageSize { get { return this.innerHttpTransport.MaxReceivedMessageSize; } set { this.innerHttpTransport.MaxReceivedMessageSize = value; } } public override string Scheme { get { return this.innerHttpTransport.Scheme; } } public override IChannelFactoryBuildChannelFactory ( BindingContext context ) { this.innerHttpTransport.Proxy = this.proxy; HttpChannelFactory factory = (HttpChannelFactory)this.innerHttpTransport.BuildChannelFactory (context); return (IChannelFactory )(object)factory; } public override IChannelListener BuildChannelListener ( BindingContext context ) { return this.innerHttpTransport.BuildChannelListener ( context ); } public override bool CanBuildChannelFactory ( BindingContext context ) { return this.innerHttpTransport.CanBuildChannelFactory ( context ); } public override bool CanBuildChannelListener ( BindingContext context ) { return this.innerHttpTransport.CanBuildChannelListener ( context ); } public override BindingElement Clone() { return new HttpProxyTransportBindingElement( this ); } public override T GetProperty ( BindingContext context ) { return this.innerHttpTransport.GetProperty ( context ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
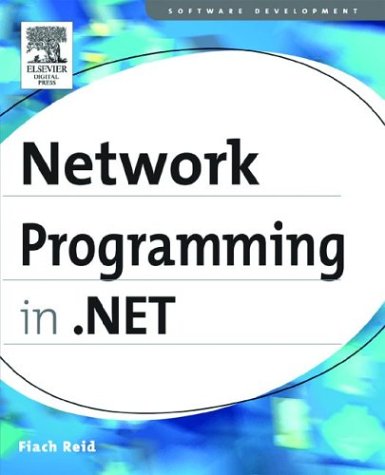
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Adorner.cs
- ShapingWorkspace.cs
- SqlCacheDependency.cs
- FontSource.cs
- XmlHelper.cs
- ClientConfigurationHost.cs
- UriParserTemplates.cs
- XPathBuilder.cs
- DataMisalignedException.cs
- XmlSchemaCollection.cs
- RuleInfoComparer.cs
- ApplicationProxyInternal.cs
- ChangeTracker.cs
- DesignerVerbCollection.cs
- AutomationPattern.cs
- UpDownBase.cs
- HttpConfigurationSystem.cs
- BaseDataListComponentEditor.cs
- DataComponentGenerator.cs
- SpellerStatusTable.cs
- WebPartMinimizeVerb.cs
- IResourceProvider.cs
- IFlowDocumentViewer.cs
- DesignTimeTemplateParser.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- CheckBox.cs
- NullableIntAverageAggregationOperator.cs
- EditorPartChrome.cs
- MouseActionConverter.cs
- BlobPersonalizationState.cs
- XmlTypeMapping.cs
- ViewGenResults.cs
- SqlGenerator.cs
- FrameworkContentElement.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- CngKeyBlobFormat.cs
- ProcessInfo.cs
- MsmqIntegrationProcessProtocolHandler.cs
- GridViewSelectEventArgs.cs
- GACMembershipCondition.cs
- PreloadedPackages.cs
- ShaderEffect.cs
- SoapTypeAttribute.cs
- Clause.cs
- RuleConditionDialog.Designer.cs
- SymLanguageType.cs
- SoapExtensionReflector.cs
- EntityDataSourceQueryBuilder.cs
- SourceFilter.cs
- DataGridViewComboBoxCell.cs
- fixedPageContentExtractor.cs
- CharKeyFrameCollection.cs
- XsltLibrary.cs
- InstanceKeyCollisionException.cs
- BufferedOutputStream.cs
- CharacterString.cs
- WithParamAction.cs
- FileDataSourceCache.cs
- EventKeyword.cs
- SineEase.cs
- ImpersonateTokenRef.cs
- JournalEntry.cs
- SubMenuStyleCollection.cs
- ListManagerBindingsCollection.cs
- FileDataSourceCache.cs
- EntityDataSourceChangedEventArgs.cs
- GridViewRowCollection.cs
- Listbox.cs
- SchemaNamespaceManager.cs
- SocketAddress.cs
- DataGridCommandEventArgs.cs
- UriExt.cs
- IisTraceWebEventProvider.cs
- WindowHideOrCloseTracker.cs
- MessageContractMemberAttribute.cs
- SpStreamWrapper.cs
- Utils.cs
- XPathMultyIterator.cs
- FixedTextPointer.cs
- CompositeFontParser.cs
- Config.cs
- SqlDataSource.cs
- UnicastIPAddressInformationCollection.cs
- RuleProcessor.cs
- ViewBox.cs
- SafeNativeMethods.cs
- XmlSchemaSimpleTypeUnion.cs
- NetSectionGroup.cs
- SubtreeProcessor.cs
- TailCallAnalyzer.cs
- VarInfo.cs
- IPipelineRuntime.cs
- AdapterUtil.cs
- SubstitutionList.cs
- EventLogger.cs
- ItemDragEvent.cs
- TypedServiceOperationListItem.cs
- _TransmitFileOverlappedAsyncResult.cs
- ExportOptions.cs
- UriSection.cs