Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / PlanCompiler / VarInfo.cs / 1305376 / VarInfo.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; //using System.Diagnostics; // Please use PlanCompiler.Assert instead of Debug.Assert in this class... using System.Globalization; using System.Data.Common; using md = System.Data.Metadata.Edm; using System.Data.Query.InternalTrees; using System.Data.Query.PlanCompiler; namespace System.Data.Query.PlanCompiler { ////// Information about a Var and its replacement /// internal abstract class VarInfo { ////// Does this VarInfo represent a collection type /// internal virtual bool IsCollectionType { get { return false; } } ////// Get the list of new Vars introduced by this VarInfo /// internal virtual List NewVars { get { return null; } } } ////// Represents information about a collection typed Var. /// Each such Var is replaced by a Var with a new "mapped" type - the "mapped" type /// is simply a collection type where the element type has been "mapped" /// internal class CollectionVarInfo : VarInfo { private List m_newVars; // always a singleton list ////// Create a CollectionVarInfo /// /// internal CollectionVarInfo(Var newVar) { m_newVars = new List(); m_newVars.Add(newVar); } ////// Get the newVar /// internal Var NewVar { get { return m_newVars[0]; } } ////// This is for a collection-type Var /// internal override bool IsCollectionType { get { return true; } } ////// Get the list of all NewVars - just one really /// internal override List NewVars { get { return m_newVars; } } } ////// The StructuredVarInfo class contains information about a structured type Var /// and how it can be replaced. This is targeted towards Vars of complex/record/ /// entity/ref types, and the goal is to replace all such Vars in this module. /// internal class StructuredVarInfo : VarInfo { private Dictionarym_propertyToVarMap; List m_newVars; bool m_newVarsIncludeNullSentinelVar; List m_newProperties; md.RowType m_newType; md.TypeUsage m_newTypeUsage; /// /// Constructor /// /// new "flat" record type corresponding to the Var's datatype /// List of vars to replace current Var /// List of properties in the "flat" record type /// Do the new vars include a var that represents a null sentinel either for this type or for any nested type internal StructuredVarInfo(md.RowType newType, List newVars, ListnewTypeProperties, bool newVarsIncludeNullSentinelVar) { PlanCompiler.Assert(newVars.Count == newTypeProperties.Count, "count mismatch"); // I see a few places where this is legal // PlanCompiler.Assert(newVars.Count > 0, "0 vars?"); m_newVars = newVars; m_newProperties = newTypeProperties; m_newType = newType; m_newVarsIncludeNullSentinelVar = newVarsIncludeNullSentinelVar; m_newTypeUsage = md.TypeUsage.Create(newType); } /// /// The NewVars property of the VarInfo is a list of the corresponding /// "scalar" Vars that can be used to replace the current Var. This is /// mainly intended for use by other RelOps that maintain lists of Vars /// - for example, the "Vars" property of ProjectOp and other similar /// locations. /// internal override List NewVars { get { return m_newVars; } } ////// The Fields property is matched 1-1 with the NewVars property, and /// specifies the properties of the record type corresponding to the /// original VarType /// internal ListFields { get { return m_newProperties; } } /// /// Indicates whether any of the vars in NewVars 'derives' /// from a null sentinel. For example, for a type that is a Record with two /// nested records, if any has a null sentinel, it would be set to true. /// It is used when expanding sort keys, to be able to indicate that there is a /// sorting operation that includes null sentinels. This indication is later /// used by transformation rules. /// internal bool NewVarsIncludeNullSentinelVar { get { return m_newVarsIncludeNullSentinelVar; } } ////// Get the Var corresponding to a specific property /// /// the requested property /// the corresponding Var ///true, if the Var was found internal bool TryGetVar(md.EdmProperty p, out Var v) { if (m_propertyToVarMap == null) { InitPropertyToVarMap(); } return m_propertyToVarMap.TryGetValue(p, out v); } ////// The NewType property describes the new "flattened" record type /// that is a replacement for the original type of the Var /// internal md.RowType NewType { get { return m_newType; } } ////// Returns the NewType wrapped in a TypeUsage /// internal md.TypeUsage NewTypeUsage { get { return m_newTypeUsage; } } ////// Initialize mapping from properties to the corresponding Var /// private void InitPropertyToVarMap() { if (m_propertyToVarMap == null) { m_propertyToVarMap = new Dictionary(); IEnumerator newVarEnumerator = m_newVars.GetEnumerator(); foreach (md.EdmProperty prop in m_newProperties) { newVarEnumerator.MoveNext(); m_propertyToVarMap.Add(prop, newVarEnumerator.Current); } newVarEnumerator.Dispose(); } } } /// /// The VarInfo map maintains a mapping from Vars to their corresponding VarInfo /// It is logically a Dictionary /// internal class VarInfoMap { private Dictionary m_map; ////// Default constructor /// internal VarInfoMap() { m_map = new Dictionary(); } ////// Create a new VarInfo for a structured type Var /// /// The structured type Var /// "Mapped" type for v /// List of vars corresponding to v /// Flattened Properties /// Do the new vars include a var that represents a null sentinel either for this type or for any nested type ///the VarInfo internal VarInfo CreateStructuredVarInfo(Var v, md.RowType newType, List newVars, ListnewProperties, bool newVarsIncludeNullSentinelVar) { VarInfo varInfo = new StructuredVarInfo(newType, newVars, newProperties, newVarsIncludeNullSentinelVar); m_map.Add(v, varInfo); return varInfo; } /// /// Create a new VarInfo for a structured type Var where the newVars cannot include a null sentinel /// /// The structured type Var /// "Mapped" type for v /// List of vars corresponding to v /// Flattened Properties internal VarInfo CreateStructuredVarInfo(Var v, md.RowType newType, List newVars, ListnewProperties) { return CreateStructuredVarInfo(v, newType, newVars, newProperties, false); } /// /// Create a VarInfo for a collection typed Var /// /// The collection-typed Var /// the new Var ///the VarInfo internal VarInfo CreateCollectionVarInfo(Var v, Var newVar) { VarInfo varInfo = new CollectionVarInfo(newVar); m_map.Add(v, varInfo); return varInfo; } ////// Return the VarInfo for the specified var (if one exists, of course) /// /// The Var /// the corresponding VarInfo ///internal bool TryGetVarInfo(Var v, out VarInfo varInfo) { return m_map.TryGetValue(v, out varInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
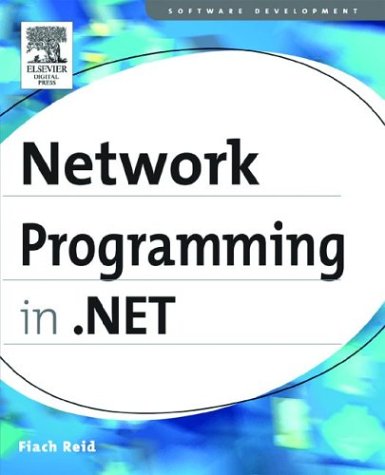
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValueTypeFixupInfo.cs
- DeobfuscatingStream.cs
- OleAutBinder.cs
- InputEventArgs.cs
- IconHelper.cs
- DateTimeOffsetConverter.cs
- _ChunkParse.cs
- PathFigureCollection.cs
- RadioButtonPopupAdapter.cs
- Region.cs
- SizeValueSerializer.cs
- UnmanagedMemoryStream.cs
- PeerContact.cs
- DataListCommandEventArgs.cs
- FullTextState.cs
- ToolStripHighContrastRenderer.cs
- DataGridViewComboBoxColumn.cs
- IDReferencePropertyAttribute.cs
- FrameworkTextComposition.cs
- Normalization.cs
- RequestSecurityToken.cs
- PointCollection.cs
- DataListCommandEventArgs.cs
- TextBounds.cs
- NamedElement.cs
- DataGridCell.cs
- ProtectedConfiguration.cs
- AnnotationDocumentPaginator.cs
- ResourceSetExpression.cs
- X509Certificate2Collection.cs
- SimpleFieldTemplateFactory.cs
- hebrewshape.cs
- BinaryFormatterWriter.cs
- MetadataPropertyAttribute.cs
- ToolStripSeparatorRenderEventArgs.cs
- GridViewRowEventArgs.cs
- DirectoryInfo.cs
- UniqueEventHelper.cs
- CanExecuteRoutedEventArgs.cs
- ProfessionalColors.cs
- DataRelation.cs
- CalendarButton.cs
- XPathSingletonIterator.cs
- ScriptingRoleServiceSection.cs
- Missing.cs
- ExpressionEditorAttribute.cs
- EntitySetRetriever.cs
- TrackingCondition.cs
- PartManifestEntry.cs
- Repeater.cs
- ArgumentNullException.cs
- AndCondition.cs
- DataGridViewLinkCell.cs
- DbProviderFactory.cs
- StateDesigner.CommentLayoutGlyph.cs
- DeviceContext.cs
- PartBasedPackageProperties.cs
- StringCollection.cs
- RegexCode.cs
- updatecommandorderer.cs
- PolygonHotSpot.cs
- FlowPanelDesigner.cs
- IndicCharClassifier.cs
- ToolStripItemRenderEventArgs.cs
- HoistedLocals.cs
- UniqueIdentifierService.cs
- LocalizeDesigner.cs
- _NestedSingleAsyncResult.cs
- LinkConverter.cs
- HtmlControl.cs
- AnnotationDocumentPaginator.cs
- SHA256.cs
- RangeValueProviderWrapper.cs
- __Error.cs
- PrinterUnitConvert.cs
- Int16AnimationUsingKeyFrames.cs
- ControlPropertyNameConverter.cs
- BindingMAnagerBase.cs
- DispatchWrapper.cs
- FontWeight.cs
- GridViewRowPresenterBase.cs
- RectAnimationClockResource.cs
- CompositeFontFamily.cs
- StylusEditingBehavior.cs
- DrawingContextWalker.cs
- CodeGroup.cs
- SafeCryptHandles.cs
- SqlTypeSystemProvider.cs
- ActivityInterfaces.cs
- AbsoluteQuery.cs
- CompiledQuery.cs
- ContainerVisual.cs
- AsyncStreamReader.cs
- Panel.cs
- XmlName.cs
- PointLight.cs
- RectIndependentAnimationStorage.cs
- ChameleonKey.cs
- XmlSchemaAttributeGroupRef.cs
- TextParagraphProperties.cs