Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PrtCap_Public.cs / 1 / PrtCap_Public.cs
/*++ Copyright (C) 2003-2005 Microsoft Corporation All rights reserved. Module Name: PrtCap_Public.cs Abstract: Definition and implementation of public PrintCapabilities class. Author: [....] ([....]) 06/22/2003 --*/ using System; using System.IO; using System.Xml; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using System.Diagnostics; using System.Globalization; using System.Printing; using MS.Internal.Printing.Configuration; namespace MS.Internal.Printing.Configuration { ////// Represents a device's printing capabilities. /// internal class InternalPrintCapabilities { #region Constructors ////// Constructs a new instance of the InternalPrintCapabilities class with capabilities based on the XML form of PrintCapabilities. /// /// Stream object containing the XML form of PrintCapabilities. ////// The ///parameter is null. /// /// The stream object specified by public InternalPrintCapabilities(Stream xmlStream) { // Verify input parameter if (xmlStream == null) { throw new ArgumentNullException("xmlStream"); } #if _DEBUG // Direct Trace output to console Trace.Listeners.Clear(); Trace.Listeners.Add(new TextWriterTraceListener(Console.Out)); #endif // Calculate the read-only counter values (this rely on the internal rules listed at // PrintSchema.Features type) _countRootFeatures = Enum.GetNames(typeof(CapabilityName)).Length; _countLocalParamDefs = Enum.GetNames(typeof(PrintSchemaLocalParameterDefs)).Length; try { // Construct a builder instance _builder = new PrintCapBuilder(xmlStream); // Construct the feature and local-parameter arrays. // (all elements should be null at this stage) _pcRootFeatures = new object[_countRootFeatures]; _pcLocalParamDefs = new ParameterDefinition[_countLocalParamDefs]; _baLocalParamRequired = new bool[_countLocalParamDefs]; for (int i=0; i<_countLocalParamDefs; i++) { _baLocalParamRequired[i] = false; } // Ask the builder to populate the PrintCapabilities object states _builder.Build(this); // Populate aggregated states based on builder's result PostBuildProcessing(); } catch (XmlException e) { // Translate XMLException into FormatException so client only needs // to catch FormatException for non-well-formed XML PrintCapabilities (the non-well-formness // could be either non-well-formed raw XML or non-well-formed PrintCapabilities content). throw NewPrintCapFormatException(e.Message, e); } // Notice we are not catching FormatException here, so it will surface to the client. // (Only the PrintCapBuilder constructor should throw FormatException for invalid PrintCapabilities // root element) } #endregion Constructors #region Public Methods ///parameter doesn't contain a well-formed XML PrintCapabilities. /// The exception object's property describes why the XML is not well-formed. And if not /// null, the exception object's property provides more details. /// /// Gets a Boolean value indicating whether the device supports a specific capability. /// /// Capability feature to check for device support. ///True if the capability is supported. False otherwise. ////// The public bool SupportsCapability(CapabilityName feature) { // Verify input parameter if (feature < PrintSchema.CapabilityNameEnumMin || feature > PrintSchema.CapabilityNameEnumMax) { throw new ArgumentOutOfRangeException("feature"); } return (_pcRootFeatures[(int)feature] != null); } #endregion Public Methods #region Public Properties ///parameter is not a standard feature defined in . /// /// Gets a ///object that specifies the device's document collate capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public DocumentCollateCapability DocumentCollateCapability { get { return (DocumentCollateCapability)_pcRootFeatures[(int)CapabilityName.DocumentCollate]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job duplex capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobDuplexCapability JobDuplexCapability { get { return (JobDuplexCapability)_pcRootFeatures[(int)CapabilityName.JobDuplex]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job NUp capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobNUpCapability JobNUpCapability { get { return (JobNUpCapability)_pcRootFeatures[(int)CapabilityName.JobNUp]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job output stapling capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobStapleCapability JobStapleCapability { get { return (JobStapleCapability)_pcRootFeatures[(int)CapabilityName.JobStaple]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page device font substitution capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageDeviceFontSubstitutionCapability PageDeviceFontSubstitutionCapability { get { return (PageDeviceFontSubstitutionCapability)_pcRootFeatures[(int)CapabilityName.PageDeviceFontSubstitution]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page media size capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageMediaSizeCapability PageMediaSizeCapability { get { return (PageMediaSizeCapability)_pcRootFeatures[(int)CapabilityName.PageMediaSize]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page media type capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageMediaTypeCapability PageMediaTypeCapability { get { return (PageMediaTypeCapability)_pcRootFeatures[(int)CapabilityName.PageMediaType]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page orientation capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageOrientationCapability PageOrientationCapability { get { return (PageOrientationCapability)_pcRootFeatures[(int)CapabilityName.PageOrientation]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page output color capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageOutputColorCapability PageOutputColorCapability { get { return (PageOutputColorCapability)_pcRootFeatures[(int)CapabilityName.PageOutputColor]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page resolution capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageResolutionCapability PageResolutionCapability { get { return (PageResolutionCapability)_pcRootFeatures[(int)CapabilityName.PageResolution]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page scaling capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageScalingCapability PageScalingCapability { get { return (PageScalingCapability)_pcRootFeatures[(int)CapabilityName.PageScaling]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page TrueType font handling mode capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageTrueTypeFontModeCapability PageTrueTypeFontModeCapability { get { return (PageTrueTypeFontModeCapability)_pcRootFeatures[(int)CapabilityName.PageTrueTypeFontMode]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job page ordering capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobPageOrderCapability JobPageOrderCapability { get { return (JobPageOrderCapability)_pcRootFeatures[(int)CapabilityName.JobPageOrder]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page photo printing intent capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PagePhotoPrintingIntentCapability PagePhotoPrintingIntentCapability { get { return (PagePhotoPrintingIntentCapability)_pcRootFeatures[(int)CapabilityName.PagePhotoPrintingIntent]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page borderless capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageBorderlessCapability PageBorderlessCapability { get { return (PageBorderlessCapability)_pcRootFeatures[(int)CapabilityName.PageBorderless]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page output quality capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageOutputQualityCapability PageOutputQualityCapability { get { return (PageOutputQualityCapability)_pcRootFeatures[(int)CapabilityName.PageOutputQuality]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job input bins capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobInputBinCapability JobInputBinCapability { get { return (JobInputBinCapability)_pcRootFeatures[(int)CapabilityName.JobInputBin]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's document input bins capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public DocumentInputBinCapability DocumentInputBinCapability { get { return (DocumentInputBinCapability)_pcRootFeatures[(int)CapabilityName.DocumentInputBin]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's page input bins capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public PageInputBinCapability PageInputBinCapability { get { return (PageInputBinCapability)_pcRootFeatures[(int)CapabilityName.PageInputBin]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that specifies the device's job copy count capability. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public JobCopyCountCapability JobCopyCountCapability { get { return (JobCopyCountCapability)_pcRootFeatures[(int)CapabilityName.JobCopyCount]; } } ///to check the capability is supported before accessing the returned reference. /// /// Gets a ///object that describes the imaged canvas for layout and rendering. /// /// If the device doesn't support this capability, null reference will be returned. /// You should use public ImageableSizeCapability PageImageableSizeCapability { get { return (ImageableSizeCapability)_pcRootFeatures[(int)CapabilityName.PageImageableSize]; } } #endregion Public Properties #region Internal Methods ///to check the capability is supported before accessing the returned reference. /// /// Returns a new FormatException instance for not-well-formed PrintCapabilities XML. /// /// detailed message about the violation of well-formness ///the new FormatException instance internal static FormatException NewPrintCapFormatException(string detailMsg) { return NewPrintCapFormatException(detailMsg, null); } ////// Returns a new FormatException instance for not-well-formed PrintCapabilities XML. /// /// detailed message about the violation of well-formness /// the exception that causes the violation of well-formness ///the new FormatException instance internal static FormatException NewPrintCapFormatException(string detailMsg, Exception innerException) { return new FormatException(String.Format(CultureInfo.CurrentCulture, "{0} {1} {2}", PrintSchemaTags.Framework.PrintCapRoot, PTUtility.GetTextFromResource("FormatException.XMLNotWellFormed"), detailMsg), innerException); } internal void SetLocalParameterDefAsRequired(int paramDefIndex, bool isRequired) { _baLocalParamRequired[paramDefIndex] = isRequired; } #endregion internal Methods #region Internal Fields // array of Print Capabilities features (feature or global-parameter-def or root-level property) internal object[] _pcRootFeatures; // array of Print Capabilities local parameter definitions internal ParameterDefinition[] _pcLocalParamDefs; #endregion Internal Fields #region Private Methods ////// Post-process states populated by the builder and populate aggregates states /// ///thrown if XML PrintCapabilities is not well-formed private void PostBuildProcessing() { for (int i=0; i<_countLocalParamDefs; i++) { if (_baLocalParamRequired[i]) { // If a parameter definition has be referenced, then the parameter definition must be present in the XML. if (_pcLocalParamDefs[i] == null) { throw NewPrintCapFormatException(String.Format(CultureInfo.CurrentCulture, PTUtility.GetTextFromResource("FormatException.ParameterDefMissOrInvalid"), PrintSchemaTags.Framework.ParameterDef, (PrintSchemaLocalParameterDefs)i)); } } } } #endregion Private Methods #region Private Fields // number of root features private readonly int _countRootFeatures; // number of local parameter definitions private readonly int _countLocalParamDefs; // array of Boolean values to indicate whether a local parameter-def is required or not private bool[] _baLocalParamRequired; private PrintCapBuilder _builder; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
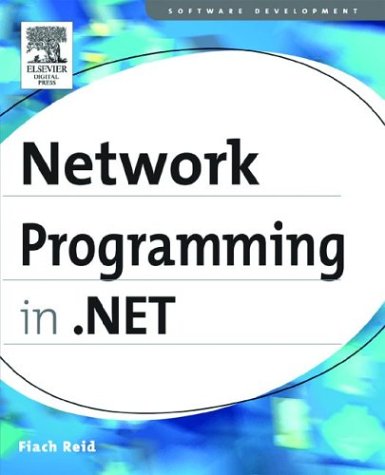
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Function.cs
- ProgressPage.cs
- ErrorFormatterPage.cs
- InertiaTranslationBehavior.cs
- HttpHandlerActionCollection.cs
- _Connection.cs
- ReadOnlyAttribute.cs
- LocatorManager.cs
- TextEditorSpelling.cs
- TreeView.cs
- Preprocessor.cs
- ConsumerConnectionPoint.cs
- TouchPoint.cs
- HostVisual.cs
- DesignerActionList.cs
- EmptyStringExpandableObjectConverter.cs
- WebRequestModuleElement.cs
- DeploymentSectionCache.cs
- DbProviderFactory.cs
- UnknownWrapper.cs
- RecognitionEventArgs.cs
- HttpCachePolicy.cs
- VisualBrush.cs
- _UriSyntax.cs
- PointLight.cs
- DataGridViewHeaderCell.cs
- ReadOnlyDataSourceView.cs
- TargetException.cs
- DocumentAutomationPeer.cs
- FilterElement.cs
- PolyBezierSegment.cs
- UnionCqlBlock.cs
- Guid.cs
- DataPagerFieldCollection.cs
- OleDbErrorCollection.cs
- RoutedEventConverter.cs
- ClientTargetSection.cs
- _StreamFramer.cs
- CursorConverter.cs
- NegationPusher.cs
- HtmlLink.cs
- ComponentChangingEvent.cs
- SettingsBindableAttribute.cs
- EdmItemCollection.cs
- StringStorage.cs
- SqlMethodAttribute.cs
- KeyboardNavigation.cs
- HtmlPageAdapter.cs
- MergeLocalizationDirectives.cs
- XamlGridLengthSerializer.cs
- CompoundFileStreamReference.cs
- CompositeCollectionView.cs
- OrderingInfo.cs
- AlignmentYValidation.cs
- MenuItemStyleCollection.cs
- RangeBaseAutomationPeer.cs
- StorageMappingItemLoader.cs
- WebAdminConfigurationHelper.cs
- EntityKey.cs
- AppSecurityManager.cs
- SelectedGridItemChangedEvent.cs
- MsmqChannelFactory.cs
- StringAnimationUsingKeyFrames.cs
- ScrollBar.cs
- LOSFormatter.cs
- AnimatedTypeHelpers.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- DataTableMappingCollection.cs
- Flowchart.cs
- Funcletizer.cs
- Message.cs
- DataPagerCommandEventArgs.cs
- _RequestLifetimeSetter.cs
- CookieProtection.cs
- UnsignedPublishLicense.cs
- RequestQueue.cs
- NativeMethods.cs
- ToolboxItemCollection.cs
- WindowsEditBoxRange.cs
- CheckPair.cs
- ArrayWithOffset.cs
- FontInfo.cs
- StrokeIntersection.cs
- SQLMembershipProvider.cs
- EditingCommands.cs
- BitmapEffectInput.cs
- FormattedTextSymbols.cs
- ModelTreeEnumerator.cs
- DataChangedEventManager.cs
- FontWeights.cs
- CustomErrorCollection.cs
- HitTestFilterBehavior.cs
- _Rfc2616CacheValidators.cs
- ColorBuilder.cs
- GroupQuery.cs
- SynchronizationLockException.cs
- GiveFeedbackEventArgs.cs
- XmlLanguage.cs
- DocumentReference.cs
- BinaryObjectReader.cs