Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Shaping / Item.cs / 1 / Item.cs
//+------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: Item.cs // // Contents: Avalon item description & script system // // Created: 12-17-2001 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Globalization; namespace MS.Internal.Shaping { ////// Run sharing the same character properties and glyph variation. /// ////// Generated by itemizer and consumed by shaping engine /// internal struct Item { internal Item( ScriptID script, ItemFlags flags ) { _script = script; _flags = flags; _digitCulture = null; } public static bool operator ==( Item left, Item right ) { return left._script == right._script && left._flags == right._flags; } public static bool operator !=( Item left, Item right ) { return !(left == right); } public override bool Equals(object o) { if (o == null) return false; if (o is Item) return this == (Item)o; return false; } public override int GetHashCode() { return (int)_script ^ (int)Flags; } ////// Shaping engine script identifier /// internal ScriptID Script { get { return _script; } set { _script = value; } } ////// Character-based flags for the item /// internal ItemFlags Flags { get { return _flags; } set { _flags = value; } } ////// Digit culture if it's a ScriptID.Digit item and we're doing number /// substitution. Otherwise, null. /// internal CultureInfo DigitCulture { get { return _digitCulture; } set { _digitCulture = value; } } private ScriptID _script; private ItemFlags _flags; private CultureInfo _digitCulture; } [Flags] internal enum ItemFlags : ushort { Default = 0, ////// Display glyph using vertical glyph form /// VerticalForm = 0x0001, ////// Display glyph using glyph of the mirrored code point form /// PairMirrorForm = 0x0002, ////// Display glyph using mirrored glyph form /// SingleMirrorForm = 0x0004, ////// The OR of both variants /// GlyphVariant = VerticalForm, ////// Item displays sideway to the baseline /// DisplaySideway = 0x0008, ////// Item containing combining marks that need precomposition /// HasCombiningMark = 0x0010, ////// Item containing extended character /// HasExtendedCharacter = 0x0020, ////// Set if the previous item ended with ZWJ /// LeadingJoin = 0x0040, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
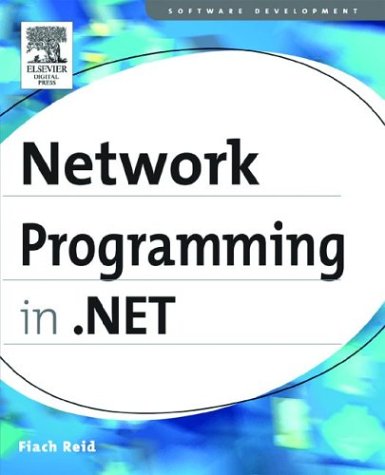
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SynchronizedPool.cs
- BinHexEncoder.cs
- smtpconnection.cs
- SqlParameter.cs
- TextBox.cs
- DataGridViewRowHeaderCell.cs
- SafeReversePInvokeHandle.cs
- AQNBuilder.cs
- ToolstripProfessionalRenderer.cs
- BlurBitmapEffect.cs
- SvcMapFileSerializer.cs
- ProviderUtil.cs
- HttpServerProtocol.cs
- TrackingServices.cs
- MappingSource.cs
- TypeBinaryExpression.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- TextRunProperties.cs
- StringArrayConverter.cs
- Translator.cs
- RefreshPropertiesAttribute.cs
- EndpointAddressProcessor.cs
- CacheAxisQuery.cs
- DiscriminatorMap.cs
- EditorZoneDesigner.cs
- PropertyIDSet.cs
- GridViewUpdatedEventArgs.cs
- ViewStateModeByIdAttribute.cs
- LicFileLicenseProvider.cs
- XpsFilter.cs
- GenericWebPart.cs
- CultureInfo.cs
- RowUpdatedEventArgs.cs
- ParameterBuilder.cs
- DataObjectMethodAttribute.cs
- DesignTimeData.cs
- DataChangedEventManager.cs
- PrivilegedConfigurationManager.cs
- ToolStripDesigner.cs
- StyleSelector.cs
- CodeEventReferenceExpression.cs
- Button.cs
- XmlParserContext.cs
- SchemaImporterExtensionElement.cs
- BoundingRectTracker.cs
- DictionaryEditChange.cs
- KeyedByTypeCollection.cs
- CommandValueSerializer.cs
- LexicalChunk.cs
- WmlCommandAdapter.cs
- CheckBoxAutomationPeer.cs
- TagMapCollection.cs
- XpsDocument.cs
- TraceInternal.cs
- TraceData.cs
- ellipse.cs
- ObjectDataSourceMethodEventArgs.cs
- ObjectDataSource.cs
- ConfigurationStrings.cs
- SrgsRule.cs
- ErrorHandler.cs
- TextRange.cs
- ContentType.cs
- CodeAttachEventStatement.cs
- BitmapPalettes.cs
- AnnotationResourceCollection.cs
- SwitchAttribute.cs
- StaticResourceExtension.cs
- TaiwanLunisolarCalendar.cs
- CacheAxisQuery.cs
- ProtectedConfiguration.cs
- LogPolicy.cs
- ObjectDataSourceDisposingEventArgs.cs
- TimersDescriptionAttribute.cs
- FileVersion.cs
- GradientBrush.cs
- WeakKeyDictionary.cs
- DataGridRow.cs
- AddingNewEventArgs.cs
- ToolZone.cs
- WindowsFormsHost.cs
- PhonemeConverter.cs
- ConnectorEditor.cs
- MouseOverProperty.cs
- ControlBuilderAttribute.cs
- ListBindingHelper.cs
- StrokeNode.cs
- WmpBitmapEncoder.cs
- MessageBox.cs
- Memoizer.cs
- ResizeBehavior.cs
- Geometry.cs
- DropShadowEffect.cs
- DesignObjectWrapper.cs
- SqlNodeAnnotation.cs
- SpecialNameAttribute.cs
- Splitter.cs
- Journal.cs
- SQLBinary.cs
- DirectoryNotFoundException.cs