Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / Validation / ValidationErrorCollection.cs / 1305376 / ValidationErrorCollection.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections.ObjectModel; using System.Collections.Generic; #region ValidationErrorCollection [Serializable()] public sealed class ValidationErrorCollection : Collection{ public ValidationErrorCollection() { } public ValidationErrorCollection(ValidationErrorCollection value) { this.AddRange(value); } public ValidationErrorCollection(IEnumerable value) { if (value == null) throw new ArgumentNullException("value"); this.AddRange(value); } protected override void InsertItem(int index, ValidationError item) { if (item == null) throw new ArgumentNullException("item"); base.InsertItem(index, item); } protected override void SetItem(int index, ValidationError item) { if (item == null) throw new ArgumentNullException("item"); base.SetItem(index, item); } public void AddRange(IEnumerable value) { if (value == null) throw new ArgumentNullException("value"); foreach (ValidationError error in value) this.Add(error); } public bool HasErrors { get { if (Count > 0) { foreach (ValidationError e in this) { if (e != null && !e.IsWarning) return true; } } return false; } } public bool HasWarnings { get { if (Count > 0) { foreach (ValidationError e in this) { if (e != null && e.IsWarning) return true; } } return false; } } public ValidationError[] ToArray() { ValidationError[] errorsArray = new ValidationError[this.Count]; this.CopyTo(errorsArray, 0); return errorsArray; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections.ObjectModel; using System.Collections.Generic; #region ValidationErrorCollection [Serializable()] public sealed class ValidationErrorCollection : Collection { public ValidationErrorCollection() { } public ValidationErrorCollection(ValidationErrorCollection value) { this.AddRange(value); } public ValidationErrorCollection(IEnumerable value) { if (value == null) throw new ArgumentNullException("value"); this.AddRange(value); } protected override void InsertItem(int index, ValidationError item) { if (item == null) throw new ArgumentNullException("item"); base.InsertItem(index, item); } protected override void SetItem(int index, ValidationError item) { if (item == null) throw new ArgumentNullException("item"); base.SetItem(index, item); } public void AddRange(IEnumerable value) { if (value == null) throw new ArgumentNullException("value"); foreach (ValidationError error in value) this.Add(error); } public bool HasErrors { get { if (Count > 0) { foreach (ValidationError e in this) { if (e != null && !e.IsWarning) return true; } } return false; } } public bool HasWarnings { get { if (Count > 0) { foreach (ValidationError e in this) { if (e != null && e.IsWarning) return true; } } return false; } } public ValidationError[] ToArray() { ValidationError[] errorsArray = new ValidationError[this.Count]; this.CopyTo(errorsArray, 0); return errorsArray; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
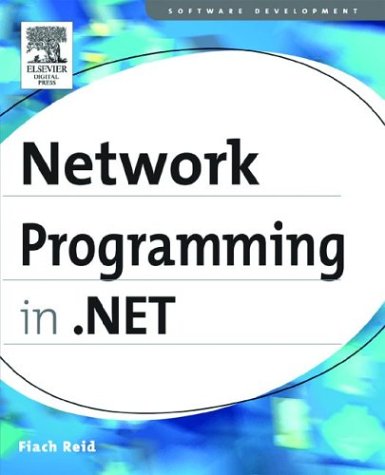
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringValidatorAttribute.cs
- HTMLTagNameToTypeMapper.cs
- SHA256Cng.cs
- DisplayMemberTemplateSelector.cs
- EntityKeyElement.cs
- PackUriHelper.cs
- DynamicQueryStringParameter.cs
- GlobalizationSection.cs
- CompositeControl.cs
- TypeFieldSchema.cs
- ToolStripDropDownButton.cs
- ToolboxBitmapAttribute.cs
- PagePropertiesChangingEventArgs.cs
- ColorContext.cs
- FacetDescriptionElement.cs
- EncryptedData.cs
- HttpCookie.cs
- SetIterators.cs
- XmlTextReaderImplHelpers.cs
- SqlConnectionFactory.cs
- UnSafeCharBuffer.cs
- PropertyMappingExceptionEventArgs.cs
- BinaryParser.cs
- PassportPrincipal.cs
- AuthenticationModuleElementCollection.cs
- RegularExpressionValidator.cs
- DeobfuscatingStream.cs
- FuncCompletionCallbackWrapper.cs
- NavigationCommands.cs
- DebugTrace.cs
- QilInvoke.cs
- FileRecordSequenceHelper.cs
- DataRelationCollection.cs
- PrintDocument.cs
- StylusPlugInCollection.cs
- DataColumnMappingCollection.cs
- SqlDataSourceConnectionPanel.cs
- EditBehavior.cs
- NamespaceTable.cs
- ToolStrip.cs
- RadialGradientBrush.cs
- AppDomainCompilerProxy.cs
- WebServiceParameterData.cs
- HttpWriter.cs
- EventMappingSettings.cs
- Message.cs
- QueryCreatedEventArgs.cs
- RightsManagementInformation.cs
- FixedPageStructure.cs
- CachedResourceDictionaryExtension.cs
- PanelDesigner.cs
- SMSvcHost.cs
- ManipulationInertiaStartingEventArgs.cs
- Size3DConverter.cs
- CmsInterop.cs
- ImportedPolicyConversionContext.cs
- UnsafeNativeMethods.cs
- WebPartEditorApplyVerb.cs
- ComponentEditorForm.cs
- QilPatternFactory.cs
- TransformerTypeCollection.cs
- ValuePatternIdentifiers.cs
- ProjectionPruner.cs
- UniqueConstraint.cs
- ListControlConvertEventArgs.cs
- FileSystemWatcher.cs
- DataBinding.cs
- MenuTracker.cs
- XmlAttributeProperties.cs
- Header.cs
- PersistencePipeline.cs
- IPCCacheManager.cs
- IsolatedStorageFilePermission.cs
- EncryptedPackageFilter.cs
- DependencyObjectCodeDomSerializer.cs
- DataColumn.cs
- EastAsianLunisolarCalendar.cs
- StubHelpers.cs
- Debugger.cs
- ZipQueryOperator.cs
- PerformanceCounterPermissionEntryCollection.cs
- ToolStripArrowRenderEventArgs.cs
- Triplet.cs
- SoapSchemaImporter.cs
- Site.cs
- NestedContainer.cs
- TreeNodeEventArgs.cs
- Base64Encoding.cs
- AddressHeaderCollection.cs
- SimpleMailWebEventProvider.cs
- SingletonInstanceContextProvider.cs
- ProfileGroupSettingsCollection.cs
- ReadOnlyCollection.cs
- NumberFormatInfo.cs
- Literal.cs
- XmlDeclaration.cs
- WebEvents.cs
- ReaderOutput.cs
- NamedPipeTransportBindingElement.cs
- NativeObjectSecurity.cs