Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / MenuTracker.cs / 1 / MenuTracker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track context menus appearing // // History: // 3/23/2004 : preid Created // //--------------------------------------------------------------------------- using System; using System.Text; using System.Windows.Automation; using System.Diagnostics; using MS.Win32; namespace MS.Internal.Automation { internal delegate void MenuHandler( AutomationElement rawEl, bool menuHasOpened ); // MenuOpened - Class used to track context menus appearing internal class MenuTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal MenuTracker(MenuHandler newHandler) : base(new int[] {NativeMethods.EVENT_SYSTEM_MENUPOPUPSTART, NativeMethods.EVENT_SYSTEM_MENUPOPUPEND}) { AddCallback(newHandler); } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { AutomationElement rawEl = null; bool menuHasOpened = eventId == NativeMethods.EVENT_SYSTEM_MENUPOPUPSTART; // Only create a raw element wrapper if the menu has popped up. Send a // null element for menu closed (the element isn't available anymore). // if( menuHasOpened ) { // Ignore if this is a bogus hwnd (shouldn't happen) if( hwnd == IntPtr.Zero ) return; NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast( hwnd ); if( !SafeNativeMethods.IsWindow( nativeHwnd ) ) return; // Filter... send events for visible hwnds only if( !SafeNativeMethods.IsWindowVisible( nativeHwnd ) ) return; rawEl = AutomationElement.FromHandle( hwnd ); } // Do callback. This handler is called due to a WinEvent on the client. The handler // is going to hand off the work of calling out to the client code to another thread // via a queue so it is safe to do this callback w/in the lock. object [] handlers = GetHandlers(); Debug.Assert(handlers.Length <= 1, "handlers.Length"); if( handlers.Length > 0 ) ( ( MenuHandler )handlers[0] )( rawEl, menuHasOpened ); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track context menus appearing // // History: // 3/23/2004 : preid Created // //--------------------------------------------------------------------------- using System; using System.Text; using System.Windows.Automation; using System.Diagnostics; using MS.Win32; namespace MS.Internal.Automation { internal delegate void MenuHandler( AutomationElement rawEl, bool menuHasOpened ); // MenuOpened - Class used to track context menus appearing internal class MenuTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal MenuTracker(MenuHandler newHandler) : base(new int[] {NativeMethods.EVENT_SYSTEM_MENUPOPUPSTART, NativeMethods.EVENT_SYSTEM_MENUPOPUPEND}) { AddCallback(newHandler); } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { AutomationElement rawEl = null; bool menuHasOpened = eventId == NativeMethods.EVENT_SYSTEM_MENUPOPUPSTART; // Only create a raw element wrapper if the menu has popped up. Send a // null element for menu closed (the element isn't available anymore). // if( menuHasOpened ) { // Ignore if this is a bogus hwnd (shouldn't happen) if( hwnd == IntPtr.Zero ) return; NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast( hwnd ); if( !SafeNativeMethods.IsWindow( nativeHwnd ) ) return; // Filter... send events for visible hwnds only if( !SafeNativeMethods.IsWindowVisible( nativeHwnd ) ) return; rawEl = AutomationElement.FromHandle( hwnd ); } // Do callback. This handler is called due to a WinEvent on the client. The handler // is going to hand off the work of calling out to the client code to another thread // via a queue so it is safe to do this callback w/in the lock. object [] handlers = GetHandlers(); Debug.Assert(handlers.Length <= 1, "handlers.Length"); if( handlers.Length > 0 ) ( ( MenuHandler )handlers[0] )( rawEl, menuHasOpened ); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
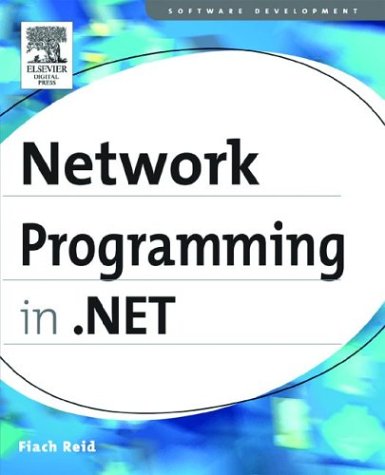
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProvideValueServiceProvider.cs
- PersistenceTypeAttribute.cs
- TransferRequestHandler.cs
- EditorAttributeInfo.cs
- AsyncSerializedWorker.cs
- DataGridViewRowStateChangedEventArgs.cs
- XPathScanner.cs
- TreeViewCancelEvent.cs
- CodeCatchClauseCollection.cs
- XPathPatternParser.cs
- ProcessHostFactoryHelper.cs
- SafeFileHandle.cs
- DataStreams.cs
- BamlCollectionHolder.cs
- Expression.cs
- SystemResourceHost.cs
- TimerEventSubscriptionCollection.cs
- ListMarkerLine.cs
- SEHException.cs
- DesignTimeValidationFeature.cs
- TableRow.cs
- documentsequencetextcontainer.cs
- OdbcReferenceCollection.cs
- ZipArchive.cs
- StackBuilderSink.cs
- HatchBrush.cs
- TracePayload.cs
- XmlText.cs
- GridViewHeaderRowPresenter.cs
- StylusTip.cs
- CellParagraph.cs
- AliasGenerator.cs
- SchemaTableOptionalColumn.cs
- PinnedBufferMemoryStream.cs
- DataGridViewButtonColumn.cs
- HtmlInputText.cs
- TextRunCacheImp.cs
- NameValueSectionHandler.cs
- ThreadStartException.cs
- KeyValuePairs.cs
- Graph.cs
- rsa.cs
- SortExpressionBuilder.cs
- complextypematerializer.cs
- Point4DConverter.cs
- DecimalConstantAttribute.cs
- Bold.cs
- XmlSchemaSet.cs
- VariantWrapper.cs
- PageParserFilter.cs
- CollectionType.cs
- MULTI_QI.cs
- SchemaMapping.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- XPathItem.cs
- TableHeaderCell.cs
- RenderingEventArgs.cs
- AudioSignalProblemOccurredEventArgs.cs
- SymbolTable.cs
- TemplateBindingExpressionConverter.cs
- FunctionNode.cs
- ThicknessAnimation.cs
- ButtonBase.cs
- Slider.cs
- _LocalDataStore.cs
- MarkupCompiler.cs
- ReflectionHelper.cs
- CacheOutputQuery.cs
- Command.cs
- Point3DValueSerializer.cs
- TlsSspiNegotiation.cs
- OdbcEnvironment.cs
- SafeArrayTypeMismatchException.cs
- DragDropHelper.cs
- RefreshEventArgs.cs
- KeyboardEventArgs.cs
- RelationshipConstraintValidator.cs
- InvalidProgramException.cs
- FileEnumerator.cs
- PrintPageEvent.cs
- TextProviderWrapper.cs
- SqlBulkCopyColumnMappingCollection.cs
- CorrelationExtension.cs
- SetterBase.cs
- ContextProperty.cs
- EntityDesignerBuildProvider.cs
- ColorInterpolationModeValidation.cs
- SystemFonts.cs
- HelpEvent.cs
- XmlILConstructAnalyzer.cs
- CalendarTable.cs
- Literal.cs
- Enum.cs
- BeginEvent.cs
- FileNotFoundException.cs
- AddingNewEventArgs.cs
- FileSystemEventArgs.cs
- XPathNodeIterator.cs
- CompilationSection.cs
- HyperLink.cs