Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / IO / PinnedBufferMemoryStream.cs / 1 / PinnedBufferMemoryStream.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: PinnedBufferMemoryStream ** ** ** Purpose: Pins a byte[], exposing it as an unmanaged memory ** stream. Used in ResourceReader for corner cases. ** ** ===========================================================*/ using System; using System.Runtime.InteropServices; namespace System.IO { internal sealed unsafe class PinnedBufferMemoryStream : UnmanagedMemoryStream { private byte[] _array; private GCHandle _pinningHandle; internal PinnedBufferMemoryStream(byte[] array) { BCLDebug.Assert(array != null, "Array can't be null"); int len = array.Length; // Handle 0 length byte arrays specially. if (len == 0) { array = new byte[1]; len = 0; } _array = array; _pinningHandle = new GCHandle(array, GCHandleType.Pinned); // Now the byte[] is pinned for the lifetime of this instance. // But I also need to get a pointer to that block of memory... fixed(byte* ptr = _array) Initialize(ptr, len, len, FileAccess.Read, true); } ~PinnedBufferMemoryStream() { Dispose(false); } protected override void Dispose(bool disposing) { if (_isOpen) { _pinningHandle.Free(); _isOpen = false; } #if _DEBUG // To help track down lifetime issues on checked builds, force //a full GC here. if (disposing) { GC.Collect(); GC.WaitForPendingFinalizers(); } #endif base.Dispose(disposing); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: PinnedBufferMemoryStream ** ** ** Purpose: Pins a byte[], exposing it as an unmanaged memory ** stream. Used in ResourceReader for corner cases. ** ** ===========================================================*/ using System; using System.Runtime.InteropServices; namespace System.IO { internal sealed unsafe class PinnedBufferMemoryStream : UnmanagedMemoryStream { private byte[] _array; private GCHandle _pinningHandle; internal PinnedBufferMemoryStream(byte[] array) { BCLDebug.Assert(array != null, "Array can't be null"); int len = array.Length; // Handle 0 length byte arrays specially. if (len == 0) { array = new byte[1]; len = 0; } _array = array; _pinningHandle = new GCHandle(array, GCHandleType.Pinned); // Now the byte[] is pinned for the lifetime of this instance. // But I also need to get a pointer to that block of memory... fixed(byte* ptr = _array) Initialize(ptr, len, len, FileAccess.Read, true); } ~PinnedBufferMemoryStream() { Dispose(false); } protected override void Dispose(bool disposing) { if (_isOpen) { _pinningHandle.Free(); _isOpen = false; } #if _DEBUG // To help track down lifetime issues on checked builds, force //a full GC here. if (disposing) { GC.Collect(); GC.WaitForPendingFinalizers(); } #endif base.Dispose(disposing); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
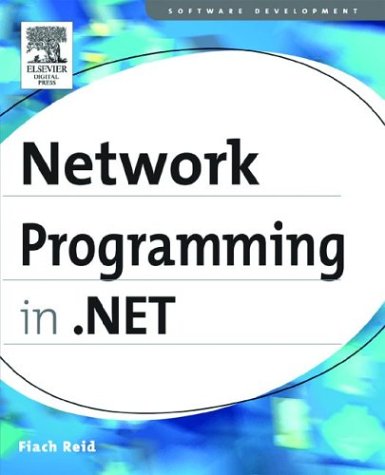
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionViewProxy.cs
- TextContainer.cs
- MetadataProperty.cs
- RequestNavigateEventArgs.cs
- RewritingProcessor.cs
- Input.cs
- ReceiveErrorHandling.cs
- UIAgentAsyncBeginRequest.cs
- ClassGenerator.cs
- PropertyOverridesDialog.cs
- ScrollEventArgs.cs
- EntityParameterCollection.cs
- DataObjectSettingDataEventArgs.cs
- DataPagerField.cs
- ElementInit.cs
- HttpListenerTimeoutManager.cs
- SafeRightsManagementHandle.cs
- PointUtil.cs
- PathFigureCollection.cs
- Propagator.Evaluator.cs
- StateWorkerRequest.cs
- FilterException.cs
- HostedNamedPipeTransportManager.cs
- AttachmentCollection.cs
- LinqTreeNodeEvaluator.cs
- StateRuntime.cs
- OutputCacheProfile.cs
- EntityDataSourceSelectedEventArgs.cs
- AbstractExpressions.cs
- Flattener.cs
- MinimizableAttributeTypeConverter.cs
- isolationinterop.cs
- DataGrid.cs
- LoginDesigner.cs
- xmlfixedPageInfo.cs
- RequestTimeoutManager.cs
- RuleValidation.cs
- FrameworkElement.cs
- DurableErrorHandler.cs
- DataGridViewCell.cs
- ThumbAutomationPeer.cs
- LinkButton.cs
- TemplateDefinition.cs
- EmptyEnumerable.cs
- XhtmlTextWriter.cs
- XslTransform.cs
- TextBoxAutoCompleteSourceConverter.cs
- SplitterCancelEvent.cs
- Wildcard.cs
- SHA512.cs
- CompiledQuery.cs
- DirectionalLight.cs
- BitmapEncoder.cs
- RuleInfoComparer.cs
- GiveFeedbackEventArgs.cs
- ProcessHostMapPath.cs
- BooleanProjectedSlot.cs
- SafeCloseHandleCritical.cs
- TreeNodeStyleCollectionEditor.cs
- DataGridViewCellValueEventArgs.cs
- SmtpNetworkElement.cs
- DictionaryCustomTypeDescriptor.cs
- EntityKey.cs
- DataGridViewLayoutData.cs
- DataViewManagerListItemTypeDescriptor.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- CommentEmitter.cs
- IntranetCredentialPolicy.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- PublisherIdentityPermission.cs
- ObjectParameter.cs
- CodeSnippetCompileUnit.cs
- BitmapEncoder.cs
- WebPartEventArgs.cs
- XmlReflectionImporter.cs
- XmlAttributeOverrides.cs
- TransactionException.cs
- PenLineJoinValidation.cs
- ContainerControl.cs
- FrameworkTextComposition.cs
- RtfFormatStack.cs
- OperandQuery.cs
- EventLogHandle.cs
- DynamicExpression.cs
- VariantWrapper.cs
- FileVersionInfo.cs
- StoreUtilities.cs
- XmlText.cs
- ObjectReaderCompiler.cs
- SqlPersonalizationProvider.cs
- CroppedBitmap.cs
- BreakRecordTable.cs
- ConfigurationProperty.cs
- MergeExecutor.cs
- SystemIPv4InterfaceProperties.cs
- ContourSegment.cs
- ControlIdConverter.cs
- RemotingServices.cs
- Merger.cs
- XamlPointCollectionSerializer.cs