Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / SingletonInstanceContextProvider.cs / 1 / SingletonInstanceContextProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel; using System.ServiceModel.Channels; internal class SingletonInstanceContextProvider : InstanceContextProviderBase { InstanceContext singleton; object thisLock; internal SingletonInstanceContextProvider(DispatchRuntime dispatchRuntime) : base(dispatchRuntime) { this.thisLock = new Object(); } internal InstanceContext SingletonInstance { get { if (this.singleton == null) { lock (this.thisLock) { if (this.singleton == null) { InstanceContext instanceContext = this.DispatchRuntime.SingletonInstanceContext; if (instanceContext == null) { instanceContext = new InstanceContext(this.DispatchRuntime.ChannelDispatcher.Host, false); } if (instanceContext.State == CommunicationState.Created) { instanceContext.Open(); } //Set the IsUsercreated flag to false for singleton mode even in cases when users create their own runtime. instanceContext.IsUserCreated = false; //Delay assigning the potentially newly created InstanceContext (till after its opened) to this.Singleton //to ensure that it is opened only once. this.singleton = instanceContext; } } } return this.singleton; } } #region IInstanceContextProvider Members public override InstanceContext GetExistingInstanceContext(Message message, IContextChannel channel) { ServiceChannel serviceChannel = this.GetServiceChannelFromProxy(channel); if (serviceChannel != null && serviceChannel.HasSession) { this.SingletonInstance.BindIncomingChannel(serviceChannel); } return this.SingletonInstance; } public override void InitializeInstanceContext(InstanceContext instanceContext, Message message, IContextChannel channel) { //no-op } public override bool IsIdle(InstanceContext instanceContext) { //By default return false return false; } public override void NotifyIdle(InstanceContextIdleCallback callback, InstanceContext instanceContext) { //no-op } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
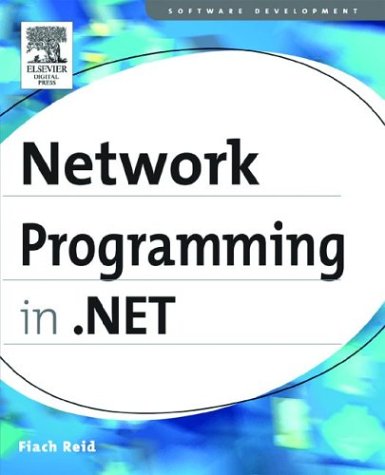
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NullRuntimeConfig.cs
- SystemPens.cs
- WindowsFormsSynchronizationContext.cs
- TypeForwardedToAttribute.cs
- MenuItem.cs
- ValidationRuleCollection.cs
- FileSecurity.cs
- ReliabilityContractAttribute.cs
- _SSPIWrapper.cs
- DynamicRendererThreadManager.cs
- StopStoryboard.cs
- BooleanConverter.cs
- XmlNavigatorStack.cs
- Clock.cs
- ValidationSummary.cs
- ComponentCodeDomSerializer.cs
- WebPartActionVerb.cs
- RuleSetBrowserDialog.cs
- DocumentStream.cs
- TimeSpanMinutesConverter.cs
- DataPagerField.cs
- TraceSwitch.cs
- ContextMenu.cs
- FileAuthorizationModule.cs
- RoutedEvent.cs
- ToolStripDropDownClosingEventArgs.cs
- ApplicationDirectory.cs
- ExternalDataExchangeService.cs
- DateTimeValueSerializer.cs
- MouseEvent.cs
- recordstatefactory.cs
- PointKeyFrameCollection.cs
- TypeElement.cs
- Encoder.cs
- SoapEnumAttribute.cs
- DataRowComparer.cs
- StateBag.cs
- DateTimeFormat.cs
- LowerCaseStringConverter.cs
- BaseTreeIterator.cs
- GenericAuthenticationEventArgs.cs
- ScrollItemProviderWrapper.cs
- MissingManifestResourceException.cs
- ProxyHwnd.cs
- DesignerHierarchicalDataSourceView.cs
- GlobalDataBindingHandler.cs
- SqlDataSourceEnumerator.cs
- ObjectItemConventionAssemblyLoader.cs
- URLIdentityPermission.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- ConfigurationValidatorBase.cs
- IPHostEntry.cs
- DataGridViewCellValidatingEventArgs.cs
- ListInitExpression.cs
- XamlTypeMapper.cs
- ProtocolsConfigurationEntry.cs
- MouseButtonEventArgs.cs
- UpdatePanelControlTrigger.cs
- ServiceDefaults.cs
- DateTimeParse.cs
- CustomWebEventKey.cs
- DataBinding.cs
- TextViewDesigner.cs
- DeviceContext.cs
- NetMsmqSecurity.cs
- SelectionItemProviderWrapper.cs
- CorrelationQueryBehavior.cs
- CallbackException.cs
- DPTypeDescriptorContext.cs
- ManagementException.cs
- FixedTextSelectionProcessor.cs
- MessageAction.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- ActivityCollectionMarkupSerializer.cs
- TextBoxRenderer.cs
- FontUnit.cs
- Enumerable.cs
- TrustSection.cs
- BlockCollection.cs
- CodeTypeOfExpression.cs
- MimeTypeAttribute.cs
- Soap11ServerProtocol.cs
- LineGeometry.cs
- XmlILTrace.cs
- HMACSHA512.cs
- ExclusiveHandle.cs
- SqlRemoveConstantOrderBy.cs
- UserControlFileEditor.cs
- ObjectStorage.cs
- DiscoveryClientProtocol.cs
- BuildManagerHost.cs
- InfoCardAsymmetricCrypto.cs
- PathStreamGeometryContext.cs
- WebPartConnectionsEventArgs.cs
- DataGridViewRowPrePaintEventArgs.cs
- QilCloneVisitor.cs
- ArgumentNullException.cs
- Events.cs
- BitmapEffectRenderDataResource.cs
- SchemaNamespaceManager.cs