Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / GlobalDataBindingHandler.cs / 1 / GlobalDataBindingHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using System.Diagnostics; using System.Reflection; using System.Web.UI; //using DataBinding = System.Web.UI.DataBinding; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] internal sealed class GlobalDataBindingHandler { public static readonly EventHandler Handler = new EventHandler(GlobalDataBindingHandler.OnDataBind); private static Hashtable dataBindingHandlerTable; ////// /// private GlobalDataBindingHandler() { } ////// /// private static Hashtable DataBindingHandlerTable { get { if (dataBindingHandlerTable == null) { dataBindingHandlerTable = new Hashtable(); } return dataBindingHandlerTable; } } ////// /// public static void OnDataBind(object sender, EventArgs e) { Debug.Assert(sender is Control, "DataBindings can only be present on Controls."); Control control = (Control)sender; // check if this control has any data-bindings IDataBindingsAccessor dataBindingsAccessor = (IDataBindingsAccessor)sender; if (dataBindingsAccessor.HasDataBindings == false) { return; } // check if the control type has an associated data-binding handler DataBindingHandlerAttribute handlerAttribute = (DataBindingHandlerAttribute)TypeDescriptor.GetAttributes(sender)[typeof(DataBindingHandlerAttribute)]; if ((handlerAttribute == null) || (handlerAttribute.HandlerTypeName.Length == 0)) { return; } // components in the designer/container do not get handled here; the // designer for that control handles it in its own special way ISite site = control.Site; IDesignerHost designerHost = null; if (site == null) { Page page = control.Page; if (page != null) { site = page.Site; } else { // When the designer is working on a UserControl instead of a Page Control parent = control.Parent; // We shouldn't have to walk up the parent chain a whole lot - maybe a couple // of levels on the average while ((site == null) && (parent != null)) { if (parent.Site != null) { site = parent.Site; } parent = parent.Parent; } } } if (site != null) { designerHost = (IDesignerHost)site.GetService(typeof(IDesignerHost)); } if (designerHost == null) { Debug.Fail("Did not get back an IDesignerHost"); return; } // non-top level components, such as controls within templates do not have designers // these are the only things that need the data-binding handler stuff IDesigner designer = designerHost.GetDesigner(control); if (designer != null) { return; } // get the handler and cache it the first time around DataBindingHandler dataBindingHandler = null; try { string handlerTypeName = handlerAttribute.HandlerTypeName; dataBindingHandler = (DataBindingHandler)DataBindingHandlerTable[handlerTypeName]; if (dataBindingHandler == null) { Type handlerType = Type.GetType(handlerTypeName); if (handlerType != null) { dataBindingHandler = (DataBindingHandler)Activator.CreateInstance(handlerType, BindingFlags.Instance | BindingFlags.Public | BindingFlags.CreateInstance, null, null, null); DataBindingHandlerTable[handlerTypeName] = dataBindingHandler; } } } catch (Exception ex) { Debug.Fail(ex.ToString()); return; } // finally delegate to it to handle this particular control if (dataBindingHandler != null) { dataBindingHandler.DataBindControl(designerHost, control); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
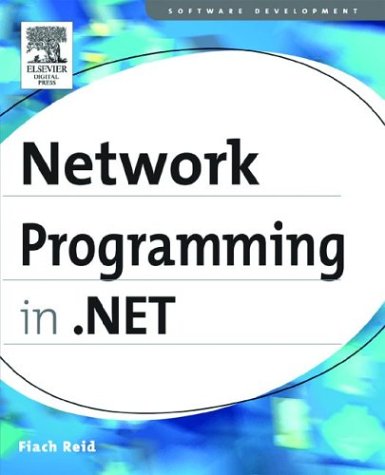
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSequenceWriter.cs
- Aggregates.cs
- BaseResourcesBuildProvider.cs
- WpfMemberInvoker.cs
- RepeaterItem.cs
- QuadraticBezierSegment.cs
- ImportDesigner.xaml.cs
- EntryWrittenEventArgs.cs
- ScrollViewer.cs
- PackagingUtilities.cs
- DashStyle.cs
- VisualStyleInformation.cs
- CodeMemberField.cs
- MatrixTransform3D.cs
- DocumentSequenceHighlightLayer.cs
- Odbc32.cs
- ClrProviderManifest.cs
- UnsafeNativeMethodsCLR.cs
- ToolStripActionList.cs
- UrlPath.cs
- LoginView.cs
- CaseStatement.cs
- LineSegment.cs
- AgileSafeNativeMemoryHandle.cs
- BooleanAnimationBase.cs
- PersonalizationDictionary.cs
- DomainConstraint.cs
- BitmapEffectvisualstate.cs
- PartialList.cs
- DomainConstraint.cs
- IsolatedStoragePermission.cs
- TemplateBindingExpression.cs
- OleDbTransaction.cs
- FixedDocument.cs
- DeploymentSectionCache.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- CacheVirtualItemsEvent.cs
- ContextToken.cs
- DataGridViewSelectedCellCollection.cs
- HtmlTableCellCollection.cs
- ADMembershipUser.cs
- ClientTargetSection.cs
- HtmlForm.cs
- StatementContext.cs
- ConfigurationPropertyCollection.cs
- AndMessageFilter.cs
- XmlUnspecifiedAttribute.cs
- FontSourceCollection.cs
- ContentFileHelper.cs
- EmbeddedMailObjectsCollection.cs
- CustomPopupPlacement.cs
- RedirectionProxy.cs
- PriorityRange.cs
- TargetException.cs
- AssemblySettingAttributes.cs
- DependencyObjectPropertyDescriptor.cs
- ProgressPage.cs
- ColorMatrix.cs
- BitmapEffectGeneralTransform.cs
- BitmapEffectDrawingContent.cs
- BadImageFormatException.cs
- Literal.cs
- ChooseAction.cs
- InkCanvasAutomationPeer.cs
- UIElementHelper.cs
- SqlParameterizer.cs
- SignatureTargetIdManager.cs
- VisualTreeHelper.cs
- TypedReference.cs
- processwaithandle.cs
- XmlSchemaSimpleContentRestriction.cs
- PageRequestManager.cs
- XmlName.cs
- ColumnReorderedEventArgs.cs
- XmlSchemaSequence.cs
- CacheChildrenQuery.cs
- PermissionListSet.cs
- ellipse.cs
- ChooseAction.cs
- ControlCollection.cs
- CodeParameterDeclarationExpression.cs
- SoapIncludeAttribute.cs
- EdmEntityTypeAttribute.cs
- NativeCppClassAttribute.cs
- OleDbCommandBuilder.cs
- Rights.cs
- BitmapEffectDrawing.cs
- FocusChangedEventArgs.cs
- EventlogProvider.cs
- wgx_commands.cs
- Transform.cs
- AsyncStreamReader.cs
- WebServicesInteroperability.cs
- SecurityElement.cs
- xsdvalidator.cs
- RecipientInfo.cs
- IntegerValidatorAttribute.cs
- CompositeControlDesigner.cs
- PolicyLevel.cs
- TypeForwardedToAttribute.cs