Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / NamedPipeTransportBindingElement.cs / 1 / NamedPipeTransportBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.ServiceModel.Activation; using System.Collections.Generic; using System.Net.Security; using System.Security.Principal; using System.Runtime.Serialization; using System.Xml; public class NamedPipeTransportBindingElement : ConnectionOrientedTransportBindingElement { ListallowedUsers; NamedPipeConnectionPoolSettings connectionPoolSettings = new NamedPipeConnectionPoolSettings(); public NamedPipeTransportBindingElement() : base() { } protected NamedPipeTransportBindingElement(NamedPipeTransportBindingElement elementToBeCloned) : base(elementToBeCloned) { if (elementToBeCloned.allowedUsers != null) { this.allowedUsers = new List (elementToBeCloned.AllowedUsers.Count); foreach (SecurityIdentifier id in elementToBeCloned.allowedUsers) { this.allowedUsers.Add(id); } } this.connectionPoolSettings = elementToBeCloned.connectionPoolSettings.Clone(); } // Used by SMSvcHost (see Activation\SharingService.cs) internal List AllowedUsers { get { return this.allowedUsers; } set { this.allowedUsers = value; } } public NamedPipeConnectionPoolSettings ConnectionPoolSettings { get { return this.connectionPoolSettings; } } public override string Scheme { get { return "net.pipe"; } } internal override string WsdlTransportUri { get { return TransportPolicyConstants.NamedPipeTransportUri; } } public override BindingElement Clone() { return new NamedPipeTransportBindingElement(this); } public override IChannelFactory BuildChannelFactory (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if(!this.CanBuildChannelFactory (context)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.ChannelTypeNotSupported, typeof(TChannel))); } return (IChannelFactory )(object)new NamedPipeChannelFactory (this, context); } public override IChannelListener BuildChannelListener (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (!this.CanBuildChannelListener (context)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.ChannelTypeNotSupported, typeof(TChannel))); } NamedPipeChannelListener listener; if (typeof(TChannel) == typeof(IReplyChannel)) { listener = new NamedPipeReplyChannelListener(this, context); } else if (typeof(TChannel) == typeof(IDuplexSessionChannel)) { listener = new NamedPipeDuplexChannelListener(this, context); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.ChannelTypeNotSupported, typeof(TChannel))); } VirtualPathExtension.ApplyHostedContext(listener, context); return (IChannelListener )(object)listener; } public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(IBindingDeliveryCapabilities)) { return (T)(object)new BindingDeliveryCapabilitiesHelper(); } else { return base.GetProperty (context); } } internal override bool IsMatch(BindingElement b) { if (!base.IsMatch(b)) { return false; } NamedPipeTransportBindingElement namedPipe = b as NamedPipeTransportBindingElement; if (namedPipe == null) { return false; } if (!this.ConnectionPoolSettings.IsMatch(namedPipe.ConnectionPoolSettings)) { return false; } return true; } class BindingDeliveryCapabilitiesHelper : IBindingDeliveryCapabilities { internal BindingDeliveryCapabilitiesHelper() { } bool IBindingDeliveryCapabilities.AssuresOrderedDelivery { get { return true; } } bool IBindingDeliveryCapabilities.QueuedDelivery { get { return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
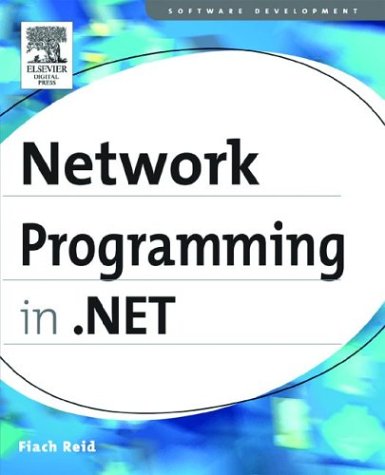
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentSequenceHighlightLayer.cs
- MappingException.cs
- ControlEvent.cs
- HtmlTitle.cs
- AnimationLayer.cs
- SchemaCollectionPreprocessor.cs
- ToolStripComboBox.cs
- RuntimeConfigLKG.cs
- Popup.cs
- GifBitmapEncoder.cs
- ToolStripSystemRenderer.cs
- DateTime.cs
- LayoutEngine.cs
- InstanceNormalEvent.cs
- ColorPalette.cs
- PseudoWebRequest.cs
- DefaultSettingsSection.cs
- SQLInt64Storage.cs
- Point4D.cs
- Matrix3DStack.cs
- CompilationUtil.cs
- DebuggerService.cs
- NativeMethods.cs
- IntPtr.cs
- ColorEditor.cs
- DataSetSchema.cs
- typedescriptorpermission.cs
- WeakEventManager.cs
- CounterSetInstanceCounterDataSet.cs
- LinqDataSourceValidationException.cs
- IteratorDescriptor.cs
- HandlerMappingMemo.cs
- RegularExpressionValidator.cs
- KnownColorTable.cs
- SystemThemeKey.cs
- Types.cs
- _ProxyRegBlob.cs
- Effect.cs
- CollectionChangeEventArgs.cs
- Track.cs
- SiteMapNodeItemEventArgs.cs
- ResetableIterator.cs
- ComponentCollection.cs
- TemplatedWizardStep.cs
- StructuredTypeInfo.cs
- BufferAllocator.cs
- StreamGeometry.cs
- DesignerRegion.cs
- TraceUtils.cs
- SqlTypesSchemaImporter.cs
- XPathMultyIterator.cs
- SamlAuthorizationDecisionStatement.cs
- XmlAnyElementAttributes.cs
- ProcessModule.cs
- Geometry.cs
- DataSourceControlBuilder.cs
- ProcessModelSection.cs
- Console.cs
- StructuralCache.cs
- JapaneseLunisolarCalendar.cs
- ApplicationInfo.cs
- MemberAccessException.cs
- WebColorConverter.cs
- ScrollEvent.cs
- BamlBinaryReader.cs
- Transform.cs
- PathData.cs
- XmlCodeExporter.cs
- HttpListenerException.cs
- CompoundFileDeflateTransform.cs
- EntityChangedParams.cs
- SvcMapFile.cs
- KnownIds.cs
- SafeViewOfFileHandle.cs
- EntityType.cs
- DataGridCellInfo.cs
- Mapping.cs
- SqlRowUpdatedEvent.cs
- SqlMethodAttribute.cs
- CollectionViewGroup.cs
- AssemblyNameProxy.cs
- DataSourceView.cs
- Emitter.cs
- XPathAxisIterator.cs
- RelationshipFixer.cs
- CompilerGlobalScopeAttribute.cs
- RpcCryptoRequest.cs
- IdleTimeoutMonitor.cs
- ZipIOExtraFieldPaddingElement.cs
- ComboBox.cs
- HtmlInputButton.cs
- BindableTemplateBuilder.cs
- WebEventCodes.cs
- ListViewEditEventArgs.cs
- WindowsStatusBar.cs
- Base64Decoder.cs
- ObjectCloneHelper.cs
- UserControl.cs
- RecognizerInfo.cs
- SQLGuidStorage.cs