Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / HashUtility.cs / 1 / HashUtility.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Singleton utilty class for hashing data. // Remarks: // This class is currently global. Need to discuss a suitable hosting environment for it // ( per user is probably appropriate ) // internal static class HashUtility { // // Summary: // The String identifier of the alg we use. Do we need to abstract this choice somewhere // public const string HashAlgorithmName = "SHA256"; private static object s_lock = new Object(); private static SHA256Managed s_hasher = new SHA256Managed(); // // Summary: // Gets the BitLength of the current hashing algorithm // public static Int32 HashBitLength { get{ return s_hasher.HashSize; } } // // Summary: // Gets the Buffer Size of the current hashing algorithm // public static Int32 HashBufferLength { get { return HashBitLength / 8; } } // // Summary: // Hashes a given value, and write the value to the output buffer. // // Remarks: // To get the size required for the output buffer, use HashBufferLength // // Parameters: // data: the output data buffer // dataIndex: the index to place the hash in the output data buffer // dataToHash: the input data to hash // public static void SetHashValue( byte[] data, int dataIndex, byte[] dataToHash ) { if( null == dataToHash ) { throw IDT.ThrowHelperArgumentNull( "dataToHash" ); } SetHashValue( data, dataIndex, dataToHash, 0, dataToHash.Length ); } // // Summary: // Hashes a given value, and write the value to the output buffer. // // Remarks: // To get the size required for the output buffer, use HashBufferLength // // Parameters: // data: the output data buffer // dataIndex: the index to place the hash in the output data buffer // dataToHash: the input data to hash // dataToHashIndex: the index in the input to start hashing // dataToHashSize: the size of the data to hash. // public static void SetHashValue( byte[] data, int dataIndex, byte[] dataToHash, int dataToHashIndex, int dataToHashSize ) { if( null == data ) { throw IDT.ThrowHelperArgumentNull( "data" ); } if( dataIndex < 0 || dataIndex >= data.Length ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataIndex", dataIndex, SR.GetString( SR.StoreHashUtilityDataOutOfRange ) ) ); } if( null == dataToHash ) { throw IDT.ThrowHelperArgumentNull( "dataToHash" ); } if( dataToHashIndex < 0 || dataToHashIndex > dataToHash.Length ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataToHashIndex", dataToHashIndex, SR.GetString( SR.StoreHashUtilityDataToHashOutOfRange ) ) ); } if( dataToHashSize < 0 || dataToHashSize > ( dataToHash.Length - dataToHashIndex ) ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "dataToHashIndex", dataToHashIndex, SR.GetString( SR.StoreHashUtilityDataToHashOutOfRange ) ) ); } byte[] newBuffer = null; lock( s_lock ) { newBuffer = s_hasher.ComputeHash( dataToHash, dataToHashIndex, dataToHashSize ); } // // Copy this buffer to the output buffer // Array.Copy( newBuffer, 0, data, dataIndex, newBuffer.Length ); // // Clear this has, as we can't ensure it will // be collected immediatly. // Array.Clear( newBuffer, 0, newBuffer.Length ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
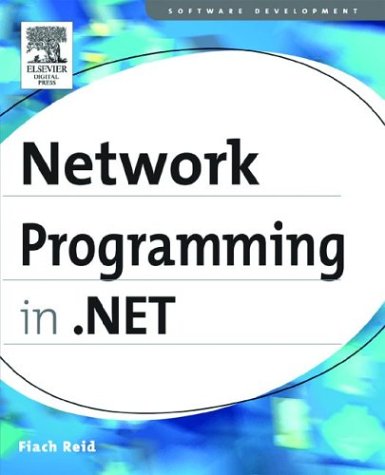
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewIntLinkedList.cs
- WpfKnownType.cs
- RenderOptions.cs
- NamespaceQuery.cs
- SessionEndingEventArgs.cs
- WindowVisualStateTracker.cs
- Rules.cs
- RelationshipEndCollection.cs
- DataGrid.cs
- _SSPIWrapper.cs
- StrokeNodeData.cs
- TraceSource.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- ManagedIStream.cs
- DefaultAsyncDataDispatcher.cs
- AssociatedControlConverter.cs
- ControllableStoryboardAction.cs
- VisualStateManager.cs
- SmiConnection.cs
- WindowsSpinner.cs
- EntityViewContainer.cs
- CqlQuery.cs
- SkipStoryboardToFill.cs
- SequenceRangeCollection.cs
- InheritanceUI.cs
- ListViewItemMouseHoverEvent.cs
- DynamicPropertyHolder.cs
- Win32.cs
- DataPagerFieldCollection.cs
- DbModificationCommandTree.cs
- QilPatternVisitor.cs
- DataGridTableCollection.cs
- Assert.cs
- SqlErrorCollection.cs
- BrowserCapabilitiesFactory35.cs
- DynamicMethod.cs
- HostingPreferredMapPath.cs
- ToolboxService.cs
- FtpCachePolicyElement.cs
- DataGridViewIntLinkedList.cs
- SecurityHelper.cs
- Internal.cs
- BinaryMethodMessage.cs
- StreamedWorkflowDefinitionContext.cs
- XmlWrappingReader.cs
- DPCustomTypeDescriptor.cs
- ServiceBusyException.cs
- ContentPosition.cs
- SqlGenericUtil.cs
- Odbc32.cs
- BrowserCapabilitiesCompiler.cs
- DrawingContextWalker.cs
- XmlSubtreeReader.cs
- ExtenderControl.cs
- ComplexType.cs
- OdbcConnectionPoolProviderInfo.cs
- SqlConnectionPoolGroupProviderInfo.cs
- StorageRoot.cs
- ItemAutomationPeer.cs
- ToolStripItemRenderEventArgs.cs
- SvcMapFile.cs
- SmtpMail.cs
- HtmlUtf8RawTextWriter.cs
- AttributeData.cs
- DateTimeStorage.cs
- JsonReaderDelegator.cs
- RoutedUICommand.cs
- AddressAlreadyInUseException.cs
- SignalGate.cs
- WizardPanelChangingEventArgs.cs
- QueryAsyncResult.cs
- NetSectionGroup.cs
- HttpListenerResponse.cs
- BulletChrome.cs
- SspiHelper.cs
- DocumentSequenceHighlightLayer.cs
- _LocalDataStoreMgr.cs
- COM2PropertyDescriptor.cs
- SqlLiftIndependentRowExpressions.cs
- SQLDecimal.cs
- XmlHierarchyData.cs
- Drawing.cs
- WhitespaceRuleReader.cs
- XmlQueryStaticData.cs
- CustomErrorsSectionWrapper.cs
- PngBitmapDecoder.cs
- ObjectStateFormatter.cs
- SplashScreen.cs
- WindowInteractionStateTracker.cs
- ToolStripSplitStackLayout.cs
- _AutoWebProxyScriptHelper.cs
- Style.cs
- ReadOnlyNameValueCollection.cs
- UnionCodeGroup.cs
- SQLDateTimeStorage.cs
- CmsInterop.cs
- StringValidatorAttribute.cs
- ImplicitInputBrush.cs
- TransformerTypeCollection.cs
- AttributeUsageAttribute.cs