Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / WhitespaceRuleReader.cs / 1 / WhitespaceRuleReader.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.IO; using System.Xml; using System.Xml.Schema; using System.Diagnostics; using MS.Internal.Xml; namespace System.Xml.Xsl.Runtime { ////// internal class WhitespaceRuleReader : XmlWrappingReader { private WhitespaceRuleLookup wsRules; private BitStack stkStrip; private bool shouldStrip, preserveAdjacent; private string val; private XmlCharType xmlCharType = XmlCharType.Instance; static public XmlReader CreateReader(XmlReader baseReader, WhitespaceRuleLookup wsRules) { if (wsRules == null) { return baseReader; // There is no rules to process } XmlReaderSettings readerSettings = baseReader.Settings; if (readerSettings != null) { if (readerSettings.IgnoreWhitespace) { return baseReader; // V2 XmlReader that strips all WS } } else { XmlTextReader txtReader = baseReader as XmlTextReader; if (txtReader != null && txtReader.WhitespaceHandling == WhitespaceHandling.None) { return baseReader; // V1 XmlTextReader that strips all WS } XmlTextReaderImpl txtReaderImpl = baseReader as XmlTextReaderImpl; if (txtReaderImpl != null && txtReaderImpl.WhitespaceHandling == WhitespaceHandling.None) { return baseReader; // XmlTextReaderImpl that strips all WS } } return new WhitespaceRuleReader(baseReader, wsRules); } private WhitespaceRuleReader(XmlReader baseReader, WhitespaceRuleLookup wsRules) : base(baseReader) { Debug.Assert(wsRules != null); this.val = null; this.stkStrip = new BitStack(); this.shouldStrip = false; this.preserveAdjacent = false; this.wsRules = wsRules; this.wsRules.Atomize(baseReader.NameTable); } ////// Override Value in order to possibly prepend extra whitespace. /// public override string Value { get { return (this.val == null) ? base.Value : this.val; } } ////// Override Read in order to search for strippable whitespace, to concatenate adjacent text nodes, and to /// resolve entities. /// public override bool Read() { XmlCharType xmlCharType = XmlCharType.Instance; string ws = null; // Clear text value this.val = null; while (base.Read()) { switch (base.NodeType) { case XmlNodeType.Element: // Push boolean indicating whether whitespace children of this element should be stripped if (!base.IsEmptyElement) { this.stkStrip.PushBit(this.shouldStrip); // Strip if rules say we should and we're not within the scope of xml:space="preserve" this.shouldStrip = wsRules.ShouldStripSpace(base.LocalName, base.NamespaceURI) && (base.XmlSpace != XmlSpace.Preserve); } break; case XmlNodeType.EndElement: // Restore parent shouldStrip setting this.shouldStrip = this.stkStrip.PopBit(); break; case XmlNodeType.Text: case XmlNodeType.CDATA: // If preserving adjacent text, don't perform any further checks if (this.preserveAdjacent) return true; if (this.shouldStrip) { // Reader may report whitespace as Text or CDATA if (xmlCharType.IsOnlyWhitespace(base.Value)) goto case XmlNodeType.Whitespace; // If whitespace was cached, then prepend it to text or CDATA value if (ws != null) this.val = string.Concat(ws, base.Value); // Preserve adjacent whitespace this.preserveAdjacent = true; return true; } break; case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: // If preserving adjacent text, don't perform any further checks if (this.preserveAdjacent) return true; if (this.shouldStrip) { // Save whitespace until it can be determined whether it will be stripped if (ws == null) ws = base.Value; else ws = string.Concat(ws, base.Value); // Read next event continue; } break; case XmlNodeType.EntityReference: reader.ResolveEntity(); break; case XmlNodeType.EndEntity: // Read next event continue; } // No longer preserve adjacent space this.preserveAdjacent = false; return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.IO; using System.Xml; using System.Xml.Schema; using System.Diagnostics; using MS.Internal.Xml; namespace System.Xml.Xsl.Runtime { ////// internal class WhitespaceRuleReader : XmlWrappingReader { private WhitespaceRuleLookup wsRules; private BitStack stkStrip; private bool shouldStrip, preserveAdjacent; private string val; private XmlCharType xmlCharType = XmlCharType.Instance; static public XmlReader CreateReader(XmlReader baseReader, WhitespaceRuleLookup wsRules) { if (wsRules == null) { return baseReader; // There is no rules to process } XmlReaderSettings readerSettings = baseReader.Settings; if (readerSettings != null) { if (readerSettings.IgnoreWhitespace) { return baseReader; // V2 XmlReader that strips all WS } } else { XmlTextReader txtReader = baseReader as XmlTextReader; if (txtReader != null && txtReader.WhitespaceHandling == WhitespaceHandling.None) { return baseReader; // V1 XmlTextReader that strips all WS } XmlTextReaderImpl txtReaderImpl = baseReader as XmlTextReaderImpl; if (txtReaderImpl != null && txtReaderImpl.WhitespaceHandling == WhitespaceHandling.None) { return baseReader; // XmlTextReaderImpl that strips all WS } } return new WhitespaceRuleReader(baseReader, wsRules); } private WhitespaceRuleReader(XmlReader baseReader, WhitespaceRuleLookup wsRules) : base(baseReader) { Debug.Assert(wsRules != null); this.val = null; this.stkStrip = new BitStack(); this.shouldStrip = false; this.preserveAdjacent = false; this.wsRules = wsRules; this.wsRules.Atomize(baseReader.NameTable); } ////// Override Value in order to possibly prepend extra whitespace. /// public override string Value { get { return (this.val == null) ? base.Value : this.val; } } ////// Override Read in order to search for strippable whitespace, to concatenate adjacent text nodes, and to /// resolve entities. /// public override bool Read() { XmlCharType xmlCharType = XmlCharType.Instance; string ws = null; // Clear text value this.val = null; while (base.Read()) { switch (base.NodeType) { case XmlNodeType.Element: // Push boolean indicating whether whitespace children of this element should be stripped if (!base.IsEmptyElement) { this.stkStrip.PushBit(this.shouldStrip); // Strip if rules say we should and we're not within the scope of xml:space="preserve" this.shouldStrip = wsRules.ShouldStripSpace(base.LocalName, base.NamespaceURI) && (base.XmlSpace != XmlSpace.Preserve); } break; case XmlNodeType.EndElement: // Restore parent shouldStrip setting this.shouldStrip = this.stkStrip.PopBit(); break; case XmlNodeType.Text: case XmlNodeType.CDATA: // If preserving adjacent text, don't perform any further checks if (this.preserveAdjacent) return true; if (this.shouldStrip) { // Reader may report whitespace as Text or CDATA if (xmlCharType.IsOnlyWhitespace(base.Value)) goto case XmlNodeType.Whitespace; // If whitespace was cached, then prepend it to text or CDATA value if (ws != null) this.val = string.Concat(ws, base.Value); // Preserve adjacent whitespace this.preserveAdjacent = true; return true; } break; case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: // If preserving adjacent text, don't perform any further checks if (this.preserveAdjacent) return true; if (this.shouldStrip) { // Save whitespace until it can be determined whether it will be stripped if (ws == null) ws = base.Value; else ws = string.Concat(ws, base.Value); // Read next event continue; } break; case XmlNodeType.EntityReference: reader.ResolveEntity(); break; case XmlNodeType.EndEntity: // Read next event continue; } // No longer preserve adjacent space this.preserveAdjacent = false; return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
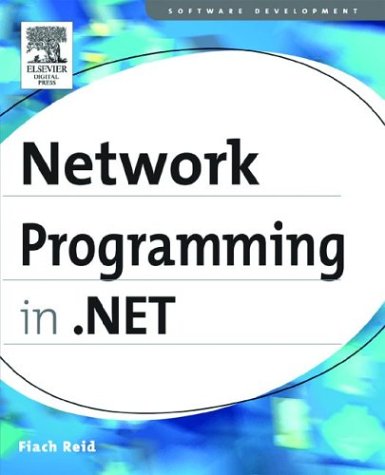
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleSheetComponentEditor.cs
- IndentTextWriter.cs
- CodeSubDirectory.cs
- ObjectNotFoundException.cs
- DataFieldCollectionEditor.cs
- PartialCachingAttribute.cs
- RtType.cs
- GetCardDetailsRequest.cs
- LocationUpdates.cs
- SafeLibraryHandle.cs
- Mutex.cs
- RelationshipWrapper.cs
- NativeMethods.cs
- UnknownBitmapDecoder.cs
- LookupNode.cs
- StorageRoot.cs
- ToolStripSplitButton.cs
- LabelTarget.cs
- ClientConvert.cs
- OrthographicCamera.cs
- UIElement3DAutomationPeer.cs
- GridLength.cs
- DataColumn.cs
- PropertyCondition.cs
- CodeIterationStatement.cs
- DesigntimeLicenseContextSerializer.cs
- DockingAttribute.cs
- StreamGeometryContext.cs
- ConfigsHelper.cs
- ToolStripPanelCell.cs
- TextWriter.cs
- IncrementalHitTester.cs
- DragDropManager.cs
- ApplicationCommands.cs
- InstanceContextManager.cs
- SmiContext.cs
- ImageKeyConverter.cs
- AdornerDecorator.cs
- TemplatedMailWebEventProvider.cs
- QueueProcessor.cs
- XmlNodeComparer.cs
- ProtocolsConfiguration.cs
- ConvertTextFrag.cs
- SHA1.cs
- XpsThumbnail.cs
- XmlNamedNodeMap.cs
- MsmqInputMessage.cs
- SR.cs
- SingleTagSectionHandler.cs
- DataColumnChangeEvent.cs
- Viewport2DVisual3D.cs
- Table.cs
- WhitespaceRule.cs
- TextModifierScope.cs
- SemanticTag.cs
- securitymgrsite.cs
- FormViewRow.cs
- StrongNameIdentityPermission.cs
- WorkflowExecutor.cs
- LayeredChannelListener.cs
- GPPOINTF.cs
- XmlEntity.cs
- ExtensionSimplifierMarkupObject.cs
- XmlSchemaObjectTable.cs
- XmlStreamNodeWriter.cs
- FilterableAttribute.cs
- DirectoryRedirect.cs
- TextPointer.cs
- EntityTypeEmitter.cs
- DigestTraceRecordHelper.cs
- WorkflowItemsPresenter.cs
- WorkflowExecutor.cs
- SecureEnvironment.cs
- ContainerParaClient.cs
- ConnectionProviderAttribute.cs
- PrivateFontCollection.cs
- TableCell.cs
- PartialList.cs
- ExpandSegment.cs
- SqlErrorCollection.cs
- Internal.cs
- ModuleBuilderData.cs
- DataBindingCollection.cs
- FrameworkElement.cs
- TypeLoadException.cs
- TrackingServices.cs
- TextElementAutomationPeer.cs
- WorkflowInstance.cs
- AdRotator.cs
- StyleSheet.cs
- TdsValueSetter.cs
- Vector3dCollection.cs
- XmlSchemas.cs
- XPathNodeInfoAtom.cs
- FormsAuthenticationCredentials.cs
- ObjectParameter.cs
- SiteMap.cs
- DataRowChangeEvent.cs
- PageClientProxyGenerator.cs
- CfgArc.cs