Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / StateMachines / Volatile.cs / 1 / Volatile.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the the implementation of the various states used by // the volatile TwoPhaseCommit participant state machine using System; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.StateMachines { //============================================================================= // VolatileRegistering // // A participant registered for volatile 2PC // We asked the TM to create a new subordinate enlistment //============================================================================= class VolatileRegistering : InactiveState { public VolatileRegistering (ProtocolState state) : base (state) {} public override void OnEvent(TmRegisterResponseEvent e) { ProcessTmRegisterResponse (e); if (e.Status == Status.Success) { e.StateMachine.ChangeState(state.States.VolatilePhaseZeroActive); } else { e.StateMachine.ChangeState(state.States.VolatileInitializationFailed); } } } //============================================================================== // VolatilePhaseZeroActive // // The TM created a volatile enlistment and success was sent to the participant // The participant requested phase zero //============================================================================= class VolatilePhaseZeroActive : ActiveState { public VolatilePhaseZeroActive (ProtocolState state) : base (state) {} // This is an unsolicited readonly public override void OnEvent(MsgReadOnlyEvent e) { e.StateMachine.ChangeState(state.States.VolatilePhaseZeroUnregistered); } public override void OnEvent(MsgAbortedEvent e) { state.TransactionManagerSend.Rollback (e.Participant); e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(TmPrePrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TwoPhaseCommitCoordinator.SendPrepare (participant); e.StateMachine.ChangeState(state.States.VolatilePrePreparing); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; state.TwoPhaseCommitCoordinator.SendRollback (participant); participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborting); } } //============================================================================== // VolatilePhaseZeroUnregistered // // The participant requested only phase zero // The participant sent an unsolicited readonly // This is an active state because we can still abort the transaction //============================================================================== class VolatilePhaseZeroUnregistered : ActiveState { public VolatilePhaseZeroUnregistered (ProtocolState state) : base (state) {} // Tolerate duplicate messages public override void OnEvent(MsgReadOnlyEvent e) { return; } // We need to be gentle with the TM until we can issue a readonly and escape public override void OnEvent(TmPrePrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.PrePrepared (participant); e.StateMachine.ChangeState(state.States.VolatilePhaseOneUnregistered); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborted); } } //============================================================================= // VolatilePhaseOneUnregistered // // The participant sent an unsolicited readonly // This is an active state because we can still abort the transaction //============================================================================== class VolatilePhaseOneUnregistered : ActiveState { public VolatilePhaseOneUnregistered (ProtocolState state) : base (state) { } // Tolerate duplicate messages public override void OnEvent(MsgReadOnlyEvent e) { return; } public override void OnEvent(TmPrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.ReadOnly (participant); e.StateMachine.ChangeState(state.States.VolatileInDoubt); } public override void OnEvent(TmSinglePhaseCommitEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Committed(participant); e.StateMachine.ChangeState(state.States.VolatileCommitted); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================= // VolatilePrePreparing // // The participant requested phase zero // The TM asked us to preprepare // We sent a preprepare to the participant //============================================================================= class VolatilePrePreparing : ActiveState { public VolatilePrePreparing (ProtocolState state) : base (state) {} public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); // Set send Prepare time for performance counter ((ParticipantEnlistment) stateMachine.Enlistment).LastMessageTime = State.QueryStartTime(); // Start up a Prepare timer stateMachine.StartTimer (TimerProfile.Preparing); } public override void Leave (StateMachine stateMachine) { base.Leave (stateMachine); ParticipantEnlistment participant = (ParticipantEnlistment) stateMachine.Enlistment; long elapsed = State.QueryStopTime() - participant.LastMessageTime; state.Perf.AverageParticipantPrepareResponseTimeBase.Increment(); state.Perf.AverageParticipantPrepareResponseTime.IncrementBy(elapsed); // Cancel the Prepare timer participant.Retries = 0; stateMachine.CancelTimer(); } public override void OnEvent(MsgReadOnlyEvent e) { state.TransactionManagerSend.PrePrepared (e.Participant); e.StateMachine.ChangeState(state.States.VolatilePhaseOneUnregistered); } // Prepared means that the volatile participant wants outcome public override void OnEvent(MsgPreparedEvent e) { state.TransactionManagerSend.PrePrepared (e.Participant); e.StateMachine.ChangeState(state.States.VolatilePrePrepared); } public override void OnEvent(MsgAbortedEvent e) { state.TransactionManagerSend.PrePrepareAborted(e.Participant); e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; state.TwoPhaseCommitCoordinator.SendRollback (participant); participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborting); } public override void OnEvent(TimerParticipantEvent e) { ParticipantEnlistment participant = e.Participant; participant.Retries ++; if (PrepareMessageRetryRecord.ShouldTrace) { PrepareMessageRetryRecord.Trace ( participant.EnlistmentId, participant.Enlistment.RemoteTransactionId, participant.Retries ); } state.Perf.PrepareRetryCountPerInterval.Increment(); // Send another pre-prepare message state.TwoPhaseCommitCoordinator.SendPrepare (participant); } } //============================================================================= // VolatilePrePrepared // // The participant requested phase zero // The participant responded prepared to our preprepare // This is an active state because we can still abort //============================================================================== class VolatilePrePrepared : ActiveState { public VolatilePrePrepared (ProtocolState state) : base (state) {} // Tolerate duplicate messages public override void OnEvent(MsgPreparedEvent e) { return; } // Respond prepared and await outcome public override void OnEvent(TmPrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Prepared (participant); e.StateMachine.ChangeState(state.States.VolatilePrepared); } // The participant really only wants outcome, so we unilaterally declare commit // The participant is volatile, so we do not worry about logging the outcome. public override void OnEvent(TmSinglePhaseCommitEvent e) { ParticipantEnlistment participant = e.Participant; state.TwoPhaseCommitCoordinator.SendCommit(participant); participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Committed(participant); e.StateMachine.ChangeState(state.States.VolatileCommitting); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; state.TwoPhaseCommitCoordinator.SendRollback (participant); participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborting); } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================= // VolatilePrepared // // The participant has responded Prepared to the PrePrepare // The participant is now awaiting the outcome of the transaction //============================================================================== class VolatilePrepared : DecidedState { public VolatilePrepared (ProtocolState state) : base (state) { } // Tolerate duplicate messages public override void OnEvent(MsgPreparedEvent e) { return; } public override void OnEvent(TmCommitEvent e) { ParticipantEnlistment participant = e.Participant; // Send the participant its outcome state.TwoPhaseCommitCoordinator.SendCommit (participant); // We short-circuit a committed response immediately and transition to finished. // We do this because the participant is volatile and we don't // want to be caught in failed-to-notify with a volatile participant participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Committed (participant); e.StateMachine.ChangeState(state.States.VolatileCommitting); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; state.TwoPhaseCommitCoordinator.SendRollback (participant); participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); e.StateMachine.ChangeState(state.States.VolatileAborting); } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================== // VolatileCommitting // // We sent the participant a Commit message // We'll remain in this state until we see one of the following: // - Committed message // - Send failure // - Fault //============================================================================= class VolatileCommitting : DecidedState { public VolatileCommitting (ProtocolState state) : base (state) {} public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); // Start up a volatile outcome timer stateMachine.StartTimer (TimerProfile.VolatileOutcomeAssurance); } public override void Leave (StateMachine stateMachine) { base.Leave (stateMachine); // Cancel the volatile outcome timer stateMachine.CancelTimer(); } // Remind the participant that we're committing public override void OnEvent(MsgPreparedEvent e) { state.TwoPhaseCommitCoordinator.SendCommit (e.Participant); } // This is our usual exit event public override void OnEvent(MsgCommittedEvent e) { e.StateMachine.ChangeState(state.States.VolatileCommitted); } public override void OnEvent(MsgParticipantFaultEvent e) { e.StateMachine.ChangeState(state.States.VolatileCommitted); } public override void OnEvent(MsgParticipantSendFailureEvent e) { e.StateMachine.ChangeState(state.States.VolatileCommitted); } public override void OnEvent(TimerParticipantEvent e) { if (e.Profile == TimerProfile.VolatileOutcomeAssurance) { ParticipantEnlistment participant = e.Participant; if (VolatileOutcomeTimeoutRecord.ShouldTrace) { VolatileOutcomeTimeoutRecord.Trace( participant.EnlistmentId, participant.Enlistment.RemoteTransactionId, TransactionOutcome.Committed, state.Config.VolatileOutcomePolicy.InitialDelay ); } // Send another commit and move on state.TwoPhaseCommitCoordinator.SendCommit (participant); e.StateMachine.ChangeState(state.States.VolatileCommitted); } } } //============================================================================== // VolatileAborting // // We sent the participant a Rollback message // We'll remain in this state until we see one of the following: // - Aborted message // - ReadOnly message // - Send failure // - Fault //============================================================================= class VolatileAborting : DecidedState { public VolatileAborting (ProtocolState state) : base (state) {} public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); // Start up a volatile outcome timer stateMachine.StartTimer (TimerProfile.VolatileOutcomeAssurance); } public override void Leave (StateMachine stateMachine) { base.Leave (stateMachine); // Cancel the volatile outcome timer stateMachine.CancelTimer(); } // The participant doesn't care about outcome public override void OnEvent(MsgReadOnlyEvent e) { e.StateMachine.ChangeState(state.States.VolatileAborted); } // Remind the participant that we're aborting public override void OnEvent(MsgPreparedEvent e) { state.TwoPhaseCommitCoordinator.SendRollback (e.Participant); } // This is our usual exit event public override void OnEvent(MsgAbortedEvent e) { e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(MsgParticipantFaultEvent e) { e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(MsgParticipantSendFailureEvent e) { e.StateMachine.ChangeState(state.States.VolatileAborted); } public override void OnEvent(TimerParticipantEvent e) { if (e.Profile == TimerProfile.VolatileOutcomeAssurance) { ParticipantEnlistment participant = e.Participant; if (VolatileOutcomeTimeoutRecord.ShouldTrace) { VolatileOutcomeTimeoutRecord.Trace( participant.EnlistmentId, participant.Enlistment.RemoteTransactionId, TransactionOutcome.Aborted, state.Config.VolatileOutcomePolicy.InitialDelay ); } // Send another rollback and move on state.TwoPhaseCommitCoordinator.SendRollback (participant); e.StateMachine.ChangeState(state.States.VolatileAborted); } } } //============================================================================= // VolatileCommitted // // We think we're done, and the transaction committed //============================================================================= class VolatileCommitted : TerminalState { public VolatileCommitted (ProtocolState state) : base (state) {} public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); if (ParticipantStateMachineFinishedRecord.ShouldTrace) { ParticipantStateMachineFinishedRecord.Trace( stateMachine.Enlistment.EnlistmentId, stateMachine.Enlistment.Enlistment.RemoteTransactionId, TransactionOutcome.Committed ); } } public override void OnEvent(MsgReadOnlyEvent e) { return; } public override void OnEvent(MsgPreparedEvent e) { state.TwoPhaseCommitCoordinator.SendCommit(e.ReplyTo); } public override void OnEvent(MsgCommittedEvent e) { return; } // The TM could issue a commit, and then a forget immediately afterwards public override void OnEvent(TmParticipantForgetEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.ForgetResponse(participant, Status.Success); } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================== // VolatileAborted // // We think we're done, and the transaction aborted //============================================================================= class VolatileAborted : TerminalState { public VolatileAborted(ProtocolState state) : base (state) {} public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); if (ParticipantStateMachineFinishedRecord.ShouldTrace) { ParticipantStateMachineFinishedRecord.Trace( stateMachine.Enlistment.EnlistmentId, stateMachine.Enlistment.Enlistment.RemoteTransactionId, TransactionOutcome.Aborted ); } } public override void OnEvent(MsgReadOnlyEvent e) { return; } public override void OnEvent(MsgPreparedEvent e) { state.TwoPhaseCommitCoordinator.SendRollback(e.ReplyTo); } public override void OnEvent(MsgAbortedEvent e) { return; } public override void OnEvent(TmPrePrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.PrePrepareAborted(participant); } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); } public override void OnEvent(TmPrepareEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); } public override void OnEvent(TmSinglePhaseCommitEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); } public override void OnEvent(TmRollbackResponseEvent e) { return; } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================== // VolatileInDoubt // // We think we're done, and we have no idea what happened to the transaction //============================================================================== class VolatileInDoubt : TerminalState { public VolatileInDoubt (ProtocolState state) : base (state) { } public override void Enter (StateMachine stateMachine) { base.Enter (stateMachine); if (ParticipantStateMachineFinishedRecord.ShouldTrace) { ParticipantStateMachineFinishedRecord.Trace( stateMachine.Enlistment.EnlistmentId, stateMachine.Enlistment.Enlistment.RemoteTransactionId, TransactionOutcome.InDoubt ); } } public override void OnEvent(MsgReadOnlyEvent e) { return; } public override void OnEvent(MsgCommittedEvent e) { return; } public override void OnEvent(MsgAbortedEvent e) { return; } public override void OnEvent(TmRollbackEvent e) { ParticipantEnlistment participant = e.Participant; participant.SetCallback(e.Callback, e.CallbackState); state.TransactionManagerSend.Aborted(participant); } public override void OnEvent(TimerParticipantEvent e) { return; } } //============================================================================= // VolatileInitializationFailed // // We think we're done because we couldn't create a subordinate enlistment //============================================================================== class VolatileInitializationFailed : TerminalState { public VolatileInitializationFailed(ProtocolState state) : base(state) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
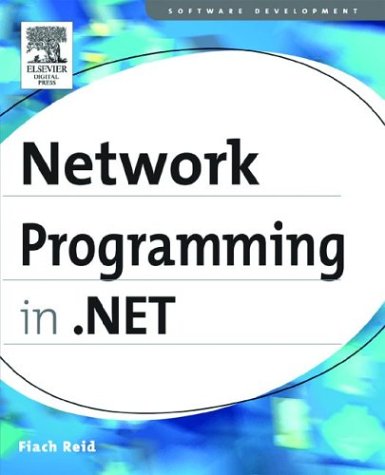
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuItemAutomationPeer.cs
- PolyLineSegment.cs
- HtmlEmptyTagControlBuilder.cs
- input.cs
- Color.cs
- WindowsEditBoxRange.cs
- GPPOINT.cs
- FrameworkElementFactoryMarkupObject.cs
- DataGridViewRowPrePaintEventArgs.cs
- AudioLevelUpdatedEventArgs.cs
- TextChange.cs
- UriSection.cs
- KerberosSecurityTokenAuthenticator.cs
- StringFunctions.cs
- ActivityDesignerResources.cs
- UIElement.cs
- NotificationContext.cs
- SecurityRuntime.cs
- ConfigurationPropertyCollection.cs
- CustomErrorCollection.cs
- OrderedDictionary.cs
- Subtree.cs
- Int64AnimationBase.cs
- HwndSourceKeyboardInputSite.cs
- BCLDebug.cs
- XPathPatternBuilder.cs
- WebServiceFault.cs
- SecurityTokenInclusionMode.cs
- RootProfilePropertySettingsCollection.cs
- SolidBrush.cs
- DataBindingExpressionBuilder.cs
- FileCodeGroup.cs
- ControlCollection.cs
- WebPart.cs
- TextSyndicationContentKindHelper.cs
- SoapHeader.cs
- EventHandlerService.cs
- CodeValidator.cs
- DllNotFoundException.cs
- SettingsProviderCollection.cs
- SiteMapNode.cs
- DependentList.cs
- MediaContext.cs
- WithParamAction.cs
- ActiveXContainer.cs
- FormatterConverter.cs
- SelectorItemAutomationPeer.cs
- LinearGradientBrush.cs
- BamlMapTable.cs
- ColorAnimationUsingKeyFrames.cs
- TextRange.cs
- DeferredTextReference.cs
- FactoryId.cs
- HiddenField.cs
- Stream.cs
- DataListItemEventArgs.cs
- BamlResourceSerializer.cs
- FileDialogPermission.cs
- RunWorkerCompletedEventArgs.cs
- PerfProviderCollection.cs
- Metadata.cs
- SecurityUniqueId.cs
- Flowchart.cs
- StructuralComparisons.cs
- ProfileService.cs
- MetabaseSettings.cs
- DropSource.cs
- DataBoundControlDesigner.cs
- SoapTypeAttribute.cs
- ThicknessConverter.cs
- EncoderNLS.cs
- DesignerCommandAdapter.cs
- Attributes.cs
- JpegBitmapEncoder.cs
- WinInetCache.cs
- BitConverter.cs
- MonthChangedEventArgs.cs
- ToolStrip.cs
- AppliedDeviceFiltersEditor.cs
- ReadWriteObjectLock.cs
- XmlPreloadedResolver.cs
- EventMap.cs
- IndicFontClient.cs
- StoreItemCollection.cs
- CodeDefaultValueExpression.cs
- WeakReference.cs
- X509Extension.cs
- MouseWheelEventArgs.cs
- ListSourceHelper.cs
- Span.cs
- AudioSignalProblemOccurredEventArgs.cs
- FaultException.cs
- safesecurityhelperavalon.cs
- TrackBar.cs
- SqlLiftWhereClauses.cs
- AutomationPropertyInfo.cs
- EqualityComparer.cs
- QilFactory.cs
- storagemappingitemcollection.viewdictionary.cs
- DataSet.cs