Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / DebugTracing.cs / 1 / DebugTracing.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains debug tracing support for the WS-AT protocol using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.Threading; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.StateMachines; namespace Microsoft.Transactions.Wsat.Protocol { class DebugTracingEventSink : IIncomingEventSink { // // Generic trace methods // void TraceGenericEvent (SynchronizationEvent e) { DebugTrace.TxTrace (TraceLevel.Info, e.Enlistment.EnlistmentId, "{0}", e); } void TraceNotificationMessage (CompletionEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.Completion.ParticipantProxy) ); } void TraceNotificationMessage (CoordinatorEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.Coordinator.CoordinatorProxy) ); } void TraceNotificationMessage (VolatileCoordinatorEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.VolatileCoordinator.CoordinatorProxy) ); } void TraceNotificationMessage (ParticipantEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.Participant.ParticipantProxy) ); } void TraceTmEvent (ParticipantCallbackEvent e) { DebugTrace.TxTrace( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} for {1}", e, Ports.TryGetAddress(e.Participant.ParticipantProxy) ); } void TraceTmResponse (SynchronizationEvent e, Status status) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1}", e, status ); } void TraceTmEvent (CoordinatorCallbackEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} for {1}", e, Ports.TryGetAddress (e.Coordinator.CoordinatorProxy) ); } void TraceFault (MessageFault fault, SynchronizationEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1}: {2}", e, Library.GetFaultCodeName(fault), Library.GetFaultCodeReason(fault) ); } // // IIncomingEventSink implementation // public void OnEvent(MsgCreateTransactionEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0}", e ); } public void OnEvent(MsgEnlistTransactionEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0}{1}", e, e.Body.IssuedToken != null ? " with issued token" : string.Empty ); } public void OnEvent(TmCreateTransactionResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1}", e, e.Status ); } public void OnEvent(TmEnlistTransactionResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1}", e, e.Status ); } public void OnEvent(MsgRegisterCompletionEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} for {1} at {2}", e, ControlProtocol.Completion, Ports.TryGetAddress (e.Proxy) ); } public void OnEvent(MsgRegisterDurableResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.Coordinator.RegistrationProxy) ); } public void OnEvent(MsgRegisterVolatileResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} from {1}", e, Ports.TryGetAddress (e.VolatileCoordinator.Coordinator.RegistrationProxy) ); } public void OnEvent(MsgRegistrationCoordinatorFaultEvent e) { TraceFault (e.Fault, e); } public void OnEvent(MsgRegistrationCoordinatorSendFailureEvent e) { TraceGenericEvent (e); } public void OnEvent(TmRegisterResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1} for {2}", e, e.Status, e.Participant.ControlProtocol ); } public void OnEvent(TmSubordinateRegisterResponseEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} {1} for {2}", e, e.Status, e.Participant.ControlProtocol ); } public void OnEvent(TmEnlistPrePrepareEvent e) { TraceGenericEvent (e); } public void OnEvent(MsgCompletionCommitEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgCompletionRollbackEvent e) { TraceNotificationMessage (e); } public void OnEvent(TmCompletionCommitResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(TmCompletionRollbackResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(MsgVolatilePrepareEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgDurablePrepareEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgVolatileCommitEvent e) { TraceNotificationMessage(e); } public void OnEvent(MsgDurableCommitEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgVolatileRollbackEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgDurableRollbackEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgDurableCoordinatorFaultEvent e) { TraceFault (e.Fault, e); } public void OnEvent(MsgVolatileCoordinatorFaultEvent e) { TraceFault (e.Fault, e); } public void OnEvent(MsgDurableCoordinatorSendFailureEvent e) { TraceGenericEvent (e); } public void OnEvent(MsgVolatileCoordinatorSendFailureEvent e) { TraceGenericEvent (e); } public void OnEvent(MsgPreparedEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgAbortedEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgReadOnlyEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgCommittedEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgReplayEvent e) { TraceNotificationMessage (e); } public void OnEvent(MsgParticipantFaultEvent e) { TraceFault (e.Fault, e); } public void OnEvent(MsgParticipantSendFailureEvent e) { TraceGenericEvent (e); } public void OnEvent(TmPrePrepareResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(TmPrepareResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(TmCommitResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(TmRollbackResponseEvent e) { TraceTmResponse (e, e.Status); } public void OnEvent(TmPrePrepareEvent e) { TraceTmEvent (e); } public void OnEvent(TmPrepareEvent e) { TraceTmEvent (e); } public void OnEvent(TmSinglePhaseCommitEvent e) { TraceTmEvent(e); } public void OnEvent(TmCommitEvent e) { TraceTmEvent (e); } public void OnEvent(TmRollbackEvent e) { TraceTmEvent (e); } public void OnEvent(TmParticipantForgetEvent e) { TraceTmEvent (e); } public void OnEvent(TmRejoinEvent e) { TraceTmEvent (e); } public void OnEvent(TmReplayEvent e) { TraceTmEvent (e); } public void OnEvent(TmCoordinatorForgetEvent e) { TraceTmEvent (e); } public void OnEvent(TmAsyncRollbackEvent e) { TraceGenericEvent (e); } public void OnEvent(TimerCoordinatorEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} for coordinator at {1}", e, Ports.TryGetAddress (e.Coordinator.CoordinatorProxy) ); } public void OnEvent(TimerParticipantEvent e) { DebugTrace.TxTrace ( TraceLevel.Info, e.Enlistment.EnlistmentId, "{0} for {1} participant at {2}", e, e.Participant.ControlProtocol, Ports.TryGetAddress (e.Participant.ParticipantProxy) ); } public void OnEvent(InternalEnlistSubordinateTransactionEvent e) { TraceGenericEvent(e); } public void OnEvent(TransactionContextEnlistTransactionEvent e) { TraceGenericEvent(e); } public void OnEvent(TransactionContextCreatedEvent e) { TraceGenericEvent(e); } public void OnEvent(TransactionContextTransactionDoneEvent e) { TraceGenericEvent(e); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
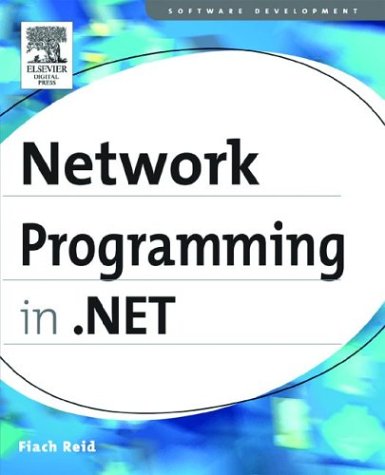
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuotedPrintableStream.cs
- IteratorFilter.cs
- InkCanvasInnerCanvas.cs
- SpecularMaterial.cs
- ObjectItemAttributeAssemblyLoader.cs
- SHA1.cs
- CompoundFileIOPermission.cs
- OracleDataAdapter.cs
- ColorMap.cs
- ObjectHandle.cs
- SerializationSectionGroup.cs
- UnsafeNativeMethodsTablet.cs
- EnumUnknown.cs
- StorageMappingFragment.cs
- RoutingExtension.cs
- Ops.cs
- WebPartVerbsEventArgs.cs
- ToolStripHighContrastRenderer.cs
- ClientSettingsSection.cs
- DataGridItem.cs
- IDictionary.cs
- controlskin.cs
- UnsafeNativeMethodsPenimc.cs
- HandlerWithFactory.cs
- Utils.cs
- SelectionProcessor.cs
- WeakEventManager.cs
- WpfGeneratedKnownProperties.cs
- AttributeCallbackBuilder.cs
- CacheSection.cs
- ParameterCollection.cs
- GeneralTransform3DGroup.cs
- CodeTypeOfExpression.cs
- OleStrCAMarshaler.cs
- FontStretch.cs
- NullableLongSumAggregationOperator.cs
- NullableDoubleAverageAggregationOperator.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- ChangeBlockUndoRecord.cs
- CollectionViewSource.cs
- WorkflowInstanceExtensionProvider.cs
- ContainerSelectorBehavior.cs
- Panel.cs
- Row.cs
- DockPatternIdentifiers.cs
- NetworkInterface.cs
- DnsEndPoint.cs
- VariableExpressionConverter.cs
- WebPartCloseVerb.cs
- MiniModule.cs
- StrokeNodeData.cs
- InstanceDataCollectionCollection.cs
- FileDialog.cs
- PingOptions.cs
- DeflateInput.cs
- ListControl.cs
- ParsedRoute.cs
- ToolboxControl.cs
- ManifestResourceInfo.cs
- MetadataAssemblyHelper.cs
- InvokePattern.cs
- ForceCopyBuildProvider.cs
- OptimizedTemplateContent.cs
- WebServiceEnumData.cs
- FilterUserControlBase.cs
- OLEDB_Util.cs
- DBConnectionString.cs
- Scheduler.cs
- XmlSchemaAnnotation.cs
- NameTable.cs
- FixedTextContainer.cs
- CodeTypeMemberCollection.cs
- Token.cs
- BindValidationContext.cs
- Glyph.cs
- Ops.cs
- Schema.cs
- SafeNativeMemoryHandle.cs
- NotConverter.cs
- XmlEntity.cs
- CodeFieldReferenceExpression.cs
- InvalidDataException.cs
- PathFigureCollection.cs
- DictionarySectionHandler.cs
- SystemIcmpV4Statistics.cs
- HwndKeyboardInputProvider.cs
- OperatingSystem.cs
- TdsParserStateObject.cs
- ToolStripMenuItem.cs
- HtmlControl.cs
- PersonalizationProvider.cs
- MetabaseReader.cs
- JavascriptCallbackBehaviorAttribute.cs
- IsolationInterop.cs
- NativeMethods.cs
- UIPermission.cs
- serverconfig.cs
- AssemblyCollection.cs
- DocumentSchemaValidator.cs
- CustomValidator.cs