Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / Configuration / WasHttpModulesInstallComponent.cs / 1 / WasHttpModulesInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install.Configuration { using WebAdmin = Microsoft.Web.Administration; using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Globalization; using System.Text; using System.Web.Configuration; internal class WasHttpModulesInstallComponent : ServiceModelInstallComponent { IIS7ConfigurationLoader configLoader; string displayString; WasHttpModulesInstallComponent(IIS7ConfigurationLoader configLoader) { if (!IisHelper.ShouldInstallWas || !IisHelper.ShouldInstallApplicationHost) { throw new WasNotInstalledException(SR.GetString(SR.WasNotInstalled, SR.GetString(SR.HttpModulesComponentNameWAS))); } else if (!IisHelper.ShouldInstallIis) { throw new IisNotInstalledException(SR.GetString(SR.IisNotInstalled, SR.GetString(SR.HttpModulesComponentNameWAS))); } this.configLoader = configLoader; } internal override string DisplayName { get {return this.displayString; } } protected override string InstallActionMessage { get {return SR.GetString(SR.HttpModulesComponentInstall, ServiceModelInstallStrings.ServiceModel); } } internal override string[] InstalledVersions { get { ListinstalledVersions = new List (); WebAdmin.ConfigurationElement httpModule = this.GetHttpModuleFromCollection(); if(null != httpModule) { string moduleTypeVersion = String.Empty; if (null != httpModule.GetAttribute(ServiceModelInstallStrings.Type)) { moduleTypeVersion = InstallHelper.GetVersionStringFromTypeString( (string)httpModule.GetAttribute(ServiceModelInstallStrings.Type).Value); } string versionString = String.Format(CultureInfo.CurrentCulture, "{0}{1}{2}", (string)httpModule.GetAttribute(ServiceModelInstallStrings.Name).Value, ServiceModelInstallStrings.VersionStringSeparator, moduleTypeVersion); if (!installedVersions.Contains(versionString)) { installedVersions.Add(versionString); } } return installedVersions.ToArray(); } } internal override bool IsInstalled { get { return (null != this.GetHttpModuleFromCollection()); } } protected override string ReinstallActionMessage { get {return SR.GetString(SR.HttpModulesComponentReinstall, ServiceModelInstallStrings.ServiceModel); } } protected override string UninstallActionMessage { get {return SR.GetString(SR.HttpModulesComponentUninstall, ServiceModelInstallStrings.ServiceModel); } } internal static WasHttpModulesInstallComponent CreateNativeWasHttpModulesInstallComponent() { WasHttpModulesInstallComponent wasHttpModulesComponent = new WasHttpModulesInstallComponent(new IIS7ConfigurationLoader(new NativeConfigurationLoader())); wasHttpModulesComponent.displayString = SR.GetString(SR.HttpModulesComponentNameWAS); return wasHttpModulesComponent; } WebAdmin.ConfigurationElement GetHttpModuleFromCollection() { WebAdmin.ConfigurationElement httpModule = null; if (null != this.configLoader.HttpModulesSection) { WebAdmin.ConfigurationElementCollection modulesCollection = this.configLoader.HttpModulesSection.GetCollection(); // ModulesCollection does not have a unique key, thus need to enumerate collection rather than check for key foreach (WebAdmin.ConfigurationElement element in modulesCollection) { if (((string)element.GetAttribute(ServiceModelInstallStrings.Name).Value).Equals(ServiceModelInstallStrings.ServiceModel, StringComparison.OrdinalIgnoreCase)) { httpModule = element; } } } return httpModule; } internal override void Install(OutputLevel outputLevel) { if (!this.IsInstalled) { if (null != this.configLoader.HttpModulesSection) { WebAdmin.ConfigurationElementCollection modulesCollection = this.configLoader.HttpModulesSection.GetCollection(); WebAdmin.ConfigurationElement moduleAction = modulesCollection.CreateElement(); moduleAction.GetAttribute(ServiceModelInstallStrings.Name).Value = ServiceModelInstallStrings.ServiceModel; moduleAction.GetAttribute(ServiceModelInstallStrings.Type).Value = ServiceModelInstallStrings.HttpModulesType; moduleAction.GetAttribute(ServiceModelInstallStrings.PreCondition).Value = ServiceModelInstallStrings.WasHttpModulesPreCondition; modulesCollection.Add(moduleAction); configLoader.Save(); } else { throw new InvalidOperationException(SR.GetString(SR.IIS7ConfigurationSectionNotFound, this.configLoader.HttpModulesSectionPath)); } } else { EventLogger.LogWarning(SR.GetString(SR.HttpModulesComponentAlreadyExists, ServiceModelInstallStrings.ServiceModel), (OutputLevel.Verbose == outputLevel)); } } internal override void Uninstall(OutputLevel outputLevel) { if (this.IsInstalled) { WebAdmin.ConfigurationElementCollection modulesCollection = this.configLoader.HttpModulesSection.GetCollection(); // ModulesCollection does not have a unique key, thus need to enumerate collection rather than check for key // Also, Microsoft.Web.Administration.ConfigurationElementCollection.Remove(element) does not work here. It // translates (bug?) to RemoveAt(-1) which fails. Instead, get index manually and call RemoveAt(int) directly. for (int i = 0; i < modulesCollection.Count; i++) { WebAdmin.ConfigurationElement element = modulesCollection[i]; if (((string)element.GetAttribute(ServiceModelInstallStrings.Name).Value).Equals(ServiceModelInstallStrings.ServiceModel, StringComparison.OrdinalIgnoreCase)) { modulesCollection.RemoveAt(i); configLoader.Save(); break; } } } else { EventLogger.LogWarning(SR.GetString(SR.HttpModulesComponentNotInstalled, ServiceModelInstallStrings.ServiceModel), (OutputLevel.Verbose == outputLevel)); } } internal override InstallationState VerifyInstall() { InstallationState installState = InstallationState.Unknown; if (this.IsInstalled) { WebAdmin.ConfigurationElement httpModule = this.GetHttpModuleFromCollection(); if (null != httpModule.GetAttribute(ServiceModelInstallStrings.Type) && ((string)httpModule.GetAttribute(ServiceModelInstallStrings.Type).Value).Equals(ServiceModelInstallStrings.HttpModulesType, StringComparison.OrdinalIgnoreCase) && null != httpModule.GetAttribute(ServiceModelInstallStrings.PreCondition) && ((string)httpModule.GetAttribute(ServiceModelInstallStrings.PreCondition).Value).Equals(ServiceModelInstallStrings.WasHttpModulesPreCondition, StringComparison.OrdinalIgnoreCase)) { installState = InstallationState.InstalledDefaults; } else { installState = InstallationState.InstalledCustom; } } else { installState = InstallationState.NotInstalled; } return installState; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
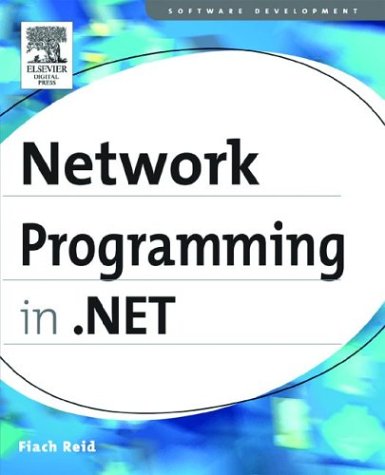
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArglessEventHandlerProxy.cs
- WrappedReader.cs
- AnnotationAdorner.cs
- ColorConverter.cs
- CodeBinaryOperatorExpression.cs
- MsmqPoisonMessageException.cs
- ContainerActivationHelper.cs
- SettingsProperty.cs
- ToolStripDropDownButton.cs
- SecurityTokenResolver.cs
- XmlDocument.cs
- WebPartZoneBase.cs
- DbParameterCollection.cs
- RegexWorker.cs
- PointAnimation.cs
- ManagedFilter.cs
- COM2IDispatchConverter.cs
- ReachDocumentSequenceSerializerAsync.cs
- ImageAttributes.cs
- SplayTreeNode.cs
- DES.cs
- ExpandableObjectConverter.cs
- NavigationWindow.cs
- QilInvokeLateBound.cs
- OuterProxyWrapper.cs
- MessageDecoder.cs
- MarginsConverter.cs
- ComplexType.cs
- ProgressiveCrcCalculatingStream.cs
- Vector3DAnimationUsingKeyFrames.cs
- CodeEventReferenceExpression.cs
- EmptyStringExpandableObjectConverter.cs
- HttpWebResponse.cs
- StrongNameIdentityPermission.cs
- PostBackOptions.cs
- CqlParser.cs
- SharedPerformanceCounter.cs
- OdbcConnectionPoolProviderInfo.cs
- ConfigurationConverterBase.cs
- ScriptManagerProxy.cs
- CollectionViewSource.cs
- DataRecordInfo.cs
- RoleServiceManager.cs
- ErrorStyle.cs
- IndexingContentUnit.cs
- DbDeleteCommandTree.cs
- UpDownEvent.cs
- ChildTable.cs
- PropertyKey.cs
- CodeExpressionRuleDeclaration.cs
- HttpRequestWrapper.cs
- TimeZone.cs
- XmlSubtreeReader.cs
- BrowserCapabilitiesFactory.cs
- ExpressionBuilder.cs
- ContextMenu.cs
- CultureInfo.cs
- TextServicesContext.cs
- Pair.cs
- PageAdapter.cs
- SQLStringStorage.cs
- SelectedPathEditor.cs
- ExpressionBuilderContext.cs
- TableLayoutPanelCellPosition.cs
- TransformerTypeCollection.cs
- LinearGradientBrush.cs
- PriorityBindingExpression.cs
- OAVariantLib.cs
- SetterBase.cs
- LambdaCompiler.Expressions.cs
- FileInfo.cs
- CustomAttribute.cs
- NetworkStream.cs
- securitycriticaldataformultiplegetandset.cs
- TabItemAutomationPeer.cs
- PermissionSet.cs
- KnownBoxes.cs
- PersistChildrenAttribute.cs
- UIElementHelper.cs
- CharacterBuffer.cs
- View.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- ControlCommandSet.cs
- RuntimeWrappedException.cs
- WebConfigurationFileMap.cs
- ResXResourceWriter.cs
- Pen.cs
- AlphabeticalEnumConverter.cs
- DataGridViewRowsRemovedEventArgs.cs
- FromRequest.cs
- ProcessModuleCollection.cs
- NativeRecognizer.cs
- RelatedPropertyManager.cs
- DesignOnlyAttribute.cs
- CalendarAutomationPeer.cs
- CodeStatementCollection.cs
- QueryResults.cs
- MethodBuilderInstantiation.cs
- DefaultValueTypeConverter.cs
- StylusPointPropertyInfo.cs