Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / CodeDOM / Compiler / IndentTextWriter.cs / 1 / IndentTextWriter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System.Diagnostics; using System; using System.IO; using System.Text; using System.Security.Permissions; using System.Globalization; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class IndentedTextWriter : TextWriter { private TextWriter writer; private int indentLevel; private bool tabsPending; private string tabString; ///Provides a text writer that can indent new lines by a tabString token. ////// public const string DefaultTabString = " "; ///[To be supplied.] ////// public IndentedTextWriter(TextWriter writer) : this(writer, DefaultTabString) { } ////// Initializes a new instance of ///using the specified /// text writer and default tab string. /// /// public IndentedTextWriter(TextWriter writer, string tabString): base(CultureInfo.InvariantCulture) { this.writer = writer; this.tabString = tabString; indentLevel = 0; tabsPending = false; } ////// Initializes a new instance of ///using the specified /// text writer and tab string. /// /// public override Encoding Encoding { get { return writer.Encoding; } } ///[To be supplied.] ////// public override string NewLine { get { return writer.NewLine; } set { writer.NewLine = value; } } ////// Gets or sets the new line character to use. /// ////// public int Indent { get { return indentLevel; } set { Debug.Assert(value >= 0, "Bogus Indent... probably caused by mismatched Indent++ and Indent--"); if (value < 0) { value = 0; } indentLevel = value; } } ////// Gets or sets the number of spaces to indent. /// ////// public TextWriter InnerWriter { get { return writer; } } internal string TabString { get { return tabString; } } ////// Gets or sets the TextWriter to use. /// ////// public override void Close() { writer.Close(); } ////// Closes the document being written to. /// ////// public override void Flush() { writer.Flush(); } ///[To be supplied.] ////// protected virtual void OutputTabs() { if (tabsPending) { for (int i=0; i < indentLevel; i++) { writer.Write(tabString); } tabsPending = false; } } ///[To be supplied.] ////// public override void Write(string s) { OutputTabs(); writer.Write(s); } ////// Writes a string /// to the text stream. /// ////// public override void Write(bool value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of a Boolean value to the text stream. /// ////// public override void Write(char value) { OutputTabs(); writer.Write(value); } ////// Writes a character to the text stream. /// ////// public override void Write(char[] buffer) { OutputTabs(); writer.Write(buffer); } ////// Writes a /// character array to the text stream. /// ////// public override void Write(char[] buffer, int index, int count) { OutputTabs(); writer.Write(buffer, index, count); } ////// Writes a subarray /// of characters to the text stream. /// ////// public override void Write(double value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of a Double to the text stream. /// ////// public override void Write(float value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of /// a Single to the text /// stream. /// ////// public override void Write(int value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an integer to the text stream. /// ////// public override void Write(long value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an 8-byte integer to the text stream. /// ////// public override void Write(object value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an object /// to the text stream. /// ////// public override void Write(string format, object arg0) { OutputTabs(); writer.Write(format, arg0); } ////// Writes out a formatted string, using the same semantics as specified. /// ////// public override void Write(string format, object arg0, object arg1) { OutputTabs(); writer.Write(format, arg0, arg1); } ////// Writes out a formatted string, /// using the same semantics as specified. /// ////// public override void Write(string format, params object[] arg) { OutputTabs(); writer.Write(format, arg); } ////// Writes out a formatted string, /// using the same semantics as specified. /// ////// public void WriteLineNoTabs(string s) { writer.WriteLine(s); } ////// Writes the specified /// string to a line without tabs. /// ////// public override void WriteLine(string s) { OutputTabs(); writer.WriteLine(s); tabsPending = true; } ////// Writes the specified string followed by /// a line terminator to the text stream. /// ////// public override void WriteLine() { OutputTabs(); writer.WriteLine(); tabsPending = true; } ////// Writes a line terminator. /// ////// public override void WriteLine(bool value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ////// Writes the text representation of a Boolean followed by a line terminator to /// the text stream. /// ////// public override void WriteLine(char value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(char[] buffer) { OutputTabs(); writer.WriteLine(buffer); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(char[] buffer, int index, int count) { OutputTabs(); writer.WriteLine(buffer, index, count); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(double value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(float value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(int value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(long value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(object value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, object arg0) { OutputTabs(); writer.WriteLine(format, arg0); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, object arg0, object arg1) { OutputTabs(); writer.WriteLine(format, arg0, arg1); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, params object[] arg) { OutputTabs(); writer.WriteLine(format, arg); tabsPending = true; } ///[To be supplied.] ////// [CLSCompliant(false)] public override void WriteLine(UInt32 value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } internal void InternalOutputTabs() { for (int i=0; i < indentLevel; i++) { writer.Write(tabString); } } } internal class Indentation { private IndentedTextWriter writer; private int indent; private string s; internal Indentation(IndentedTextWriter writer, int indent) { this.writer = writer; this.indent = indent; s = null; } internal string IndentationString { get { if ( s == null) { string tabString = writer.TabString; StringBuilder sb = new StringBuilder(indent * tabString.Length); for( int i = 0; i < indent; i++) { sb.Append(tabString); } s = sb.ToString(); } return s; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System.Diagnostics; using System; using System.IO; using System.Text; using System.Security.Permissions; using System.Globalization; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class IndentedTextWriter : TextWriter { private TextWriter writer; private int indentLevel; private bool tabsPending; private string tabString; ///Provides a text writer that can indent new lines by a tabString token. ////// public const string DefaultTabString = " "; ///[To be supplied.] ////// public IndentedTextWriter(TextWriter writer) : this(writer, DefaultTabString) { } ////// Initializes a new instance of ///using the specified /// text writer and default tab string. /// /// public IndentedTextWriter(TextWriter writer, string tabString): base(CultureInfo.InvariantCulture) { this.writer = writer; this.tabString = tabString; indentLevel = 0; tabsPending = false; } ////// Initializes a new instance of ///using the specified /// text writer and tab string. /// /// public override Encoding Encoding { get { return writer.Encoding; } } ///[To be supplied.] ////// public override string NewLine { get { return writer.NewLine; } set { writer.NewLine = value; } } ////// Gets or sets the new line character to use. /// ////// public int Indent { get { return indentLevel; } set { Debug.Assert(value >= 0, "Bogus Indent... probably caused by mismatched Indent++ and Indent--"); if (value < 0) { value = 0; } indentLevel = value; } } ////// Gets or sets the number of spaces to indent. /// ////// public TextWriter InnerWriter { get { return writer; } } internal string TabString { get { return tabString; } } ////// Gets or sets the TextWriter to use. /// ////// public override void Close() { writer.Close(); } ////// Closes the document being written to. /// ////// public override void Flush() { writer.Flush(); } ///[To be supplied.] ////// protected virtual void OutputTabs() { if (tabsPending) { for (int i=0; i < indentLevel; i++) { writer.Write(tabString); } tabsPending = false; } } ///[To be supplied.] ////// public override void Write(string s) { OutputTabs(); writer.Write(s); } ////// Writes a string /// to the text stream. /// ////// public override void Write(bool value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of a Boolean value to the text stream. /// ////// public override void Write(char value) { OutputTabs(); writer.Write(value); } ////// Writes a character to the text stream. /// ////// public override void Write(char[] buffer) { OutputTabs(); writer.Write(buffer); } ////// Writes a /// character array to the text stream. /// ////// public override void Write(char[] buffer, int index, int count) { OutputTabs(); writer.Write(buffer, index, count); } ////// Writes a subarray /// of characters to the text stream. /// ////// public override void Write(double value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of a Double to the text stream. /// ////// public override void Write(float value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of /// a Single to the text /// stream. /// ////// public override void Write(int value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an integer to the text stream. /// ////// public override void Write(long value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an 8-byte integer to the text stream. /// ////// public override void Write(object value) { OutputTabs(); writer.Write(value); } ////// Writes the text representation of an object /// to the text stream. /// ////// public override void Write(string format, object arg0) { OutputTabs(); writer.Write(format, arg0); } ////// Writes out a formatted string, using the same semantics as specified. /// ////// public override void Write(string format, object arg0, object arg1) { OutputTabs(); writer.Write(format, arg0, arg1); } ////// Writes out a formatted string, /// using the same semantics as specified. /// ////// public override void Write(string format, params object[] arg) { OutputTabs(); writer.Write(format, arg); } ////// Writes out a formatted string, /// using the same semantics as specified. /// ////// public void WriteLineNoTabs(string s) { writer.WriteLine(s); } ////// Writes the specified /// string to a line without tabs. /// ////// public override void WriteLine(string s) { OutputTabs(); writer.WriteLine(s); tabsPending = true; } ////// Writes the specified string followed by /// a line terminator to the text stream. /// ////// public override void WriteLine() { OutputTabs(); writer.WriteLine(); tabsPending = true; } ////// Writes a line terminator. /// ////// public override void WriteLine(bool value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ////// Writes the text representation of a Boolean followed by a line terminator to /// the text stream. /// ////// public override void WriteLine(char value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(char[] buffer) { OutputTabs(); writer.WriteLine(buffer); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(char[] buffer, int index, int count) { OutputTabs(); writer.WriteLine(buffer, index, count); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(double value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(float value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(int value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(long value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(object value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, object arg0) { OutputTabs(); writer.WriteLine(format, arg0); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, object arg0, object arg1) { OutputTabs(); writer.WriteLine(format, arg0, arg1); tabsPending = true; } ///[To be supplied.] ////// public override void WriteLine(string format, params object[] arg) { OutputTabs(); writer.WriteLine(format, arg); tabsPending = true; } ///[To be supplied.] ////// [CLSCompliant(false)] public override void WriteLine(UInt32 value) { OutputTabs(); writer.WriteLine(value); tabsPending = true; } internal void InternalOutputTabs() { for (int i=0; i < indentLevel; i++) { writer.Write(tabString); } } } internal class Indentation { private IndentedTextWriter writer; private int indent; private string s; internal Indentation(IndentedTextWriter writer, int indent) { this.writer = writer; this.indent = indent; s = null; } internal string IndentationString { get { if ( s == null) { string tabString = writer.TabString; StringBuilder sb = new StringBuilder(indent * tabString.Length); for( int i = 0; i < indent; i++) { sb.Append(tabString); } s = sb.ToString(); } return s; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
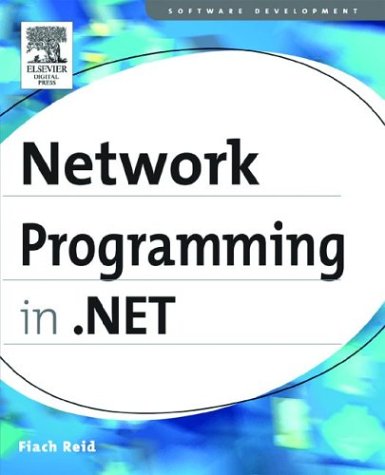
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableCellAutomationPeer.cs
- WinEventTracker.cs
- BitmapCache.cs
- PanelDesigner.cs
- DeviceContexts.cs
- XNodeNavigator.cs
- FrameworkContentElementAutomationPeer.cs
- DockPanel.cs
- TextServicesDisplayAttributePropertyRanges.cs
- CompiledRegexRunnerFactory.cs
- CompositeDataBoundControl.cs
- TextProperties.cs
- XDeferredAxisSource.cs
- AccessText.cs
- RelationshipConverter.cs
- UnsafeNetInfoNativeMethods.cs
- EpmSyndicationContentSerializer.cs
- SecureUICommand.cs
- GridViewRow.cs
- DataViewSetting.cs
- ParameterRefs.cs
- XmlNodeComparer.cs
- ParseHttpDate.cs
- HttpApplicationFactory.cs
- SqlDataSourceSelectingEventArgs.cs
- RadioButtonRenderer.cs
- ProfileServiceManager.cs
- TimeEnumHelper.cs
- ExtensionQuery.cs
- LogArchiveSnapshot.cs
- ListBindableAttribute.cs
- Helper.cs
- XslNumber.cs
- DataContext.cs
- MethodCallTranslator.cs
- AssemblyNameEqualityComparer.cs
- FieldDescriptor.cs
- HttpApplicationFactory.cs
- MailMessageEventArgs.cs
- UrlAuthorizationModule.cs
- IPipelineRuntime.cs
- OrderPreservingPipeliningMergeHelper.cs
- Debug.cs
- DiffuseMaterial.cs
- _NegotiateClient.cs
- OracleBFile.cs
- MatrixTransform.cs
- StackBuilderSink.cs
- OneWayBindingElement.cs
- HyperLinkField.cs
- OracleBoolean.cs
- ProjectedSlot.cs
- ReadOnlyActivityGlyph.cs
- UnmanagedMarshal.cs
- ProfileInfo.cs
- WinFormsSecurity.cs
- MemberAccessException.cs
- UserMapPath.cs
- GridViewAutomationPeer.cs
- UDPClient.cs
- WebPartActionVerb.cs
- ExpressionBuilderCollection.cs
- AnimationStorage.cs
- Nodes.cs
- DataBindingExpressionBuilder.cs
- SqlRewriteScalarSubqueries.cs
- Error.cs
- CompiledRegexRunnerFactory.cs
- SqlNotificationRequest.cs
- DurableServiceAttribute.cs
- ManualResetEvent.cs
- InkCanvasAutomationPeer.cs
- DrawingContextWalker.cs
- PackagePartCollection.cs
- PropertyContainer.cs
- PanelDesigner.cs
- EntityDataSourceQueryBuilder.cs
- IgnoreDeviceFilterElementCollection.cs
- FontResourceCache.cs
- JsonWriter.cs
- SafeFileMappingHandle.cs
- BridgeDataRecord.cs
- AuthenticationServiceManager.cs
- RouteParametersHelper.cs
- HashLookup.cs
- Baml2006ReaderContext.cs
- ZoneLinkButton.cs
- SafeLibraryHandle.cs
- UnsafeNativeMethodsCLR.cs
- EdgeProfileValidation.cs
- OutputCacheModule.cs
- GeneralTransform.cs
- CryptoApi.cs
- KeyPullup.cs
- WebServicesInteroperability.cs
- UIElement.cs
- VScrollProperties.cs
- TextFindEngine.cs
- DataControlButton.cs
- HostingEnvironmentSection.cs