Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ListenerBinder.cs / 1 / ListenerBinder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; static class ListenerBinder { internal static IListenerBinder GetBinder(IChannelListener listener, MessageVersion messageVersion) { IChannelListenerinput = listener as IChannelListener ; if (input != null) return new InputListenerBinder(input, messageVersion); IChannelListener inputSession = listener as IChannelListener ; if (inputSession != null) return new InputSessionListenerBinder(inputSession, messageVersion); IChannelListener reply = listener as IChannelListener ; if (reply != null) return new ReplyListenerBinder(reply, messageVersion); IChannelListener replySession = listener as IChannelListener ; if (replySession != null) return new ReplySessionListenerBinder(replySession, messageVersion); IChannelListener duplex = listener as IChannelListener ; if (duplex != null) return new DuplexListenerBinder(duplex, messageVersion); IChannelListener duplexSession = listener as IChannelListener ; if (duplexSession != null) return new DuplexSessionListenerBinder(duplexSession, messageVersion); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.UnknownListenerType1, listener.Uri.AbsoluteUri))); } // ----------------------------------------------------------------------------------------------------------- // Listener Binders class DuplexListenerBinder : IListenerBinder { IRequestReplyCorrelator correlator; IChannelListener listener; MessageVersion messageVersion; internal DuplexListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.correlator = new RequestReplyCorrelator(); this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IDuplexChannel channel = this.listener.AcceptChannel(timeout); if (channel == null) return null; return new DuplexChannelBinder(channel, this.correlator, this.listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IDuplexChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new DuplexChannelBinder(channel, this.correlator, this.listener.Uri); } } class DuplexSessionListenerBinder : IListenerBinder { IRequestReplyCorrelator correlator; IChannelListener listener; MessageVersion messageVersion; internal DuplexSessionListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.correlator = new RequestReplyCorrelator(); this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IDuplexSessionChannel channel = this.listener.AcceptChannel(timeout); if (channel == null) return null; return new DuplexChannelBinder(channel, this.correlator, this.listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IDuplexSessionChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new DuplexChannelBinder(channel, this.correlator, this.listener.Uri); } } class InputListenerBinder : IListenerBinder { IChannelListener listener; MessageVersion messageVersion; internal InputListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IInputChannel channel = this.listener.AcceptChannel(timeout); if (channel == null) return null; return new InputChannelBinder(channel, this.listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IInputChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new InputChannelBinder(channel, this.listener.Uri); } } class InputSessionListenerBinder : IListenerBinder { IChannelListener listener; MessageVersion messageVersion; internal InputSessionListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IInputSessionChannel channel = this.listener.AcceptChannel(timeout); if (null == channel) return null; return new InputChannelBinder(channel, this.listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IInputSessionChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new InputChannelBinder(channel, this.listener.Uri); } } class ReplyListenerBinder : IListenerBinder { IChannelListener listener; MessageVersion messageVersion; internal ReplyListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IReplyChannel channel = this.listener.AcceptChannel(timeout); if (channel == null) return null; return new ReplyChannelBinder(channel, listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IReplyChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new ReplyChannelBinder(channel, listener.Uri); } } class ReplySessionListenerBinder : IListenerBinder { IChannelListener listener; MessageVersion messageVersion; internal ReplySessionListenerBinder(IChannelListener listener, MessageVersion messageVersion) { this.listener = listener; this.messageVersion = messageVersion; } public IChannelListener Listener { get { return this.listener; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public IChannelBinder Accept(TimeSpan timeout) { IReplySessionChannel channel = this.listener.AcceptChannel(timeout); if (channel == null) return null; return new ReplyChannelBinder(channel, listener.Uri); } public IAsyncResult BeginAccept(TimeSpan timeout, AsyncCallback callback, object state) { return this.listener.BeginAcceptChannel(timeout, callback, state); } public IChannelBinder EndAccept(IAsyncResult result) { IReplySessionChannel channel = this.listener.EndAcceptChannel(result); if (channel == null) return null; return new ReplyChannelBinder(channel, listener.Uri); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
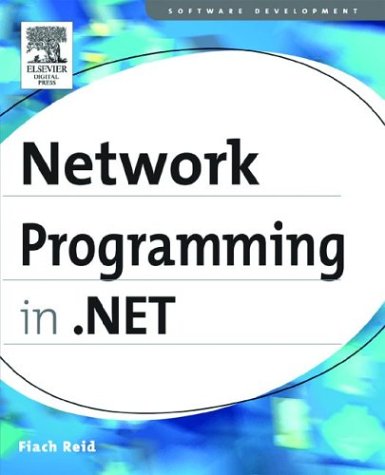
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Condition.cs
- TemplatedAdorner.cs
- SwitchElementsCollection.cs
- ObjectDataSourceMethodEventArgs.cs
- WebBrowserHelper.cs
- PreProcessInputEventArgs.cs
- XXXOnTypeBuilderInstantiation.cs
- MetadataHelper.cs
- XmlNamespaceMappingCollection.cs
- XmlAttributeCache.cs
- QilPatternVisitor.cs
- AbstractSvcMapFileLoader.cs
- SqlCacheDependencyDatabaseCollection.cs
- SystemWebSectionGroup.cs
- GridEntry.cs
- ZipPackagePart.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- categoryentry.cs
- ContainerActivationHelper.cs
- SQLInt16Storage.cs
- XamlSerializerUtil.cs
- PreloadedPackages.cs
- OutputCacheSettings.cs
- SqlMultiplexer.cs
- DataGridComboBoxColumn.cs
- CopyCodeAction.cs
- GuidConverter.cs
- OutgoingWebRequestContext.cs
- Scheduling.cs
- COM2EnumConverter.cs
- EntityDataSourceSelectingEventArgs.cs
- FormViewDeletedEventArgs.cs
- DES.cs
- XmlEntity.cs
- PropertyGridEditorPart.cs
- MetaType.cs
- TextEditorTyping.cs
- PasswordRecovery.cs
- MsmqIntegrationChannelFactory.cs
- WebPartMinimizeVerb.cs
- CompositeTypefaceMetrics.cs
- SectionVisual.cs
- HandledMouseEvent.cs
- FactoryGenerator.cs
- PerspectiveCamera.cs
- LineVisual.cs
- BasicCommandTreeVisitor.cs
- XmlSchemaAttribute.cs
- TextContainerChangedEventArgs.cs
- WsatAdminException.cs
- FullTextLine.cs
- XmlReader.cs
- InkCanvasInnerCanvas.cs
- ReadContentAsBinaryHelper.cs
- XmlSortKeyAccumulator.cs
- SecureEnvironment.cs
- EntityDataSourceWrapperCollection.cs
- DataGridHelper.cs
- FilteredDataSetHelper.cs
- HandlerBase.cs
- TokenBasedSet.cs
- BinaryWriter.cs
- RealProxy.cs
- ContextMenuAutomationPeer.cs
- Graphics.cs
- EntitySqlQueryCacheEntry.cs
- ConfigurationValidatorAttribute.cs
- TemplateInstanceAttribute.cs
- BaseDataList.cs
- ExtensibleClassFactory.cs
- DataGridToolTip.cs
- DataGridViewHitTestInfo.cs
- BindingGroup.cs
- RangeValidator.cs
- QuaternionValueSerializer.cs
- AuditLevel.cs
- TraceInternal.cs
- WebConfigurationHost.cs
- DataGridItemEventArgs.cs
- VirtualPathUtility.cs
- DataSourceProvider.cs
- DateTimeConstantAttribute.cs
- AncillaryOps.cs
- NotConverter.cs
- DataGridTablesFactory.cs
- JsonDeserializer.cs
- SmtpNetworkElement.cs
- UpDownBase.cs
- UInt64Converter.cs
- JsonObjectDataContract.cs
- AttributeCollection.cs
- ItemsControlAutomationPeer.cs
- ManipulationInertiaStartingEventArgs.cs
- EntityViewGenerationAttribute.cs
- WorkflowMessageEventArgs.cs
- SqlErrorCollection.cs
- UnauthorizedAccessException.cs
- StreamReader.cs
- KerberosSecurityTokenProvider.cs
- WmpBitmapDecoder.cs