Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / HostedNamedPipeTransportManager.cs / 1 / HostedNamedPipeTransportManager.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Collections.Generic; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; class HostedNamedPipeTransportManager : NamedPipeTransportManager { bool settingsApplied; OnViaDelegate onViaCallback; SharedConnectionListener listener; ConnectionDemuxer connectionDemuxer; int queueId; Guid token; OnDuplicatedViaDelegate onDuplicatedViaCallback; bool demuxerCreated; public HostedNamedPipeTransportManager(BaseUriWithWildcard baseAddress) : base(baseAddress.BaseAddress) { this.HostNameComparisonMode = baseAddress.HostNameComparisonMode; this.onViaCallback = new OnViaDelegate(OnVia); this.onDuplicatedViaCallback = new OnDuplicatedViaDelegate(OnDuplicatedVia); } protected override bool IsCompatible(NamedPipeChannelListener channelListener) { if (channelListener.HostedVirtualPath == null) { return false; } return base.IsCompatible(channelListener); } internal void Start(int queueId, Guid token, MessageReceivedCallback messageReceivedCallback) { SetMessageReceivedCallback(messageReceivedCallback); OnOpenInternal(queueId, token); } internal override void OnOpen() { // This is intentionally empty. } internal void Stop() { lock (ThisLock) { if (listener != null) { listener.Stop(); // The listener will be closed by the demuxer. listener = null; } if (connectionDemuxer != null) { connectionDemuxer.Dispose(); } demuxerCreated = false; settingsApplied = false; } } void CreateConnectionDemuxer() { IConnectionListener connectionListener = new BufferedConnectionListener(listener, MaxOutputDelay, ConnectionBufferSize); if (DiagnosticUtility.ShouldUseActivity) { connectionListener = new TracingConnectionListener(connectionListener, this.ListenUri); } connectionDemuxer = new ConnectionDemuxer(connectionListener, MaxPendingAccepts, MaxPendingConnections, ChannelInitializationTimeout, IdleTimeout, MaxPooledConnections, OnGetTransportFactorySettings, OnGetSingletonMessageHandler, OnHandleServerSessionPreamble, OnDemuxerError); connectionDemuxer.StartDemuxing(onViaCallback); } void OnOpenInternal(int queueId, Guid token) { lock (ThisLock) { this.queueId = queueId; this.token = token; BaseUriWithWildcard path = new BaseUriWithWildcard(this.ListenUri, this.HostNameComparisonMode); listener = new SharedConnectionListener(path, queueId, token, this.onDuplicatedViaCallback); } } internal override void OnClose() { Stop(); base.OnClose(); } void OnVia(Uri address) { Debug.Print("HostedNamedPipeTransportManager.OnVia() address: " + address + " calling EnsureServiceAvailable()"); ServiceHostingEnvironment.EnsureServiceAvailable(address.LocalPath); } protected override void OnSelecting(NamedPipeChannelListener channelListener) { if (settingsApplied) { return; } lock (ThisLock) { if (settingsApplied) { // Use the setting for the first one. return; } this.ApplyListenerSettings(channelListener); settingsApplied = true; } } // This method is called only for the first via of the current proxy. void OnDuplicatedVia(Uri via, out int connectionBufferSize) { OnVia(via); if (!demuxerCreated) { lock (ThisLock) { if (listener == null) { // The listener has been stopped. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CommunicationObjectAbortedException( SR.GetString(SR.Sharing_ListenerProxyStopped))); } if (!demuxerCreated) { CreateConnectionDemuxer(); demuxerCreated = true; } } } connectionBufferSize = this.ConnectionBufferSize; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
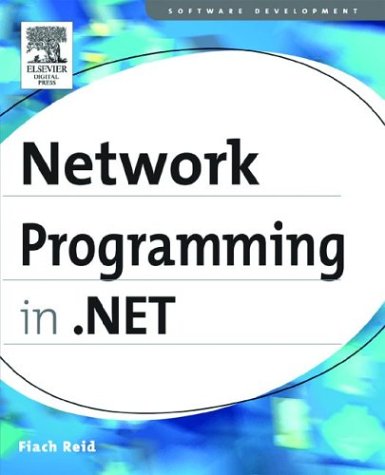
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonDialog.cs
- Interlocked.cs
- ProjectionPathSegment.cs
- StrongName.cs
- InstancePersistenceContext.cs
- AccessibilityHelperForVista.cs
- WorkflowCompensationBehavior.cs
- ColorAnimation.cs
- CookieProtection.cs
- InterleavedZipPartStream.cs
- DynamicValidatorEventArgs.cs
- GeometryHitTestResult.cs
- DiscoveryDocument.cs
- FrameworkContentElement.cs
- IISUnsafeMethods.cs
- X509CertificateChain.cs
- DirectoryLocalQuery.cs
- AnimationClock.cs
- WebRequestModuleElement.cs
- ResourceReferenceKeyNotFoundException.cs
- TextTreeExtractElementUndoUnit.cs
- SoapTypeAttribute.cs
- StringKeyFrameCollection.cs
- CompositeControl.cs
- CodeIterationStatement.cs
- DataGridViewButtonColumn.cs
- NameValuePair.cs
- Property.cs
- PageContent.cs
- SettingsAttributeDictionary.cs
- XhtmlTextWriter.cs
- UInt64Storage.cs
- SamlAuthenticationStatement.cs
- TimeSpanStorage.cs
- EncryptedKey.cs
- CellTreeNodeVisitors.cs
- Inline.cs
- Image.cs
- SingleAnimationUsingKeyFrames.cs
- HttpModuleActionCollection.cs
- ProxyWebPart.cs
- DataGridViewTextBoxEditingControl.cs
- ListChunk.cs
- TPLETWProvider.cs
- ToolStripDropDownClosedEventArgs.cs
- PrinterUnitConvert.cs
- AppliedDeviceFiltersDialog.cs
- ClientSponsor.cs
- SelectionService.cs
- RelationshipDetailsRow.cs
- UnionExpr.cs
- CryptoHandle.cs
- DesignTimeType.cs
- Grammar.cs
- MemoryPressure.cs
- DataObjectAttribute.cs
- ListDictionary.cs
- UnknownBitmapEncoder.cs
- Rijndael.cs
- GraphicsContext.cs
- ProfileSettings.cs
- WebReferenceOptions.cs
- ListControl.cs
- Lazy.cs
- WeakHashtable.cs
- KoreanLunisolarCalendar.cs
- CompiledIdentityConstraint.cs
- OlePropertyStructs.cs
- Rotation3DAnimation.cs
- WorkflowValidationFailedException.cs
- MimeXmlImporter.cs
- UnionExpr.cs
- WebPartMenuStyle.cs
- QueryOperationResponseOfT.cs
- StylusPointPropertyInfo.cs
- BitFlagsGenerator.cs
- ContentType.cs
- VerticalAlignConverter.cs
- DoWorkEventArgs.cs
- Atom10FeedFormatter.cs
- SqlCacheDependencySection.cs
- CodeSubDirectory.cs
- WMICapabilities.cs
- AnnotationHighlightLayer.cs
- BaseDataListActionList.cs
- CodeBlockBuilder.cs
- Padding.cs
- CheckableControlBaseAdapter.cs
- AsymmetricAlgorithm.cs
- ImageMap.cs
- ScrollPatternIdentifiers.cs
- Activator.cs
- TemplateBindingExtensionConverter.cs
- DependencyPropertyDescriptor.cs
- ExpressionBindings.cs
- DataGridViewCellValidatingEventArgs.cs
- FontStyles.cs
- MsmqEncryptionAlgorithm.cs
- RadialGradientBrush.cs
- ConfigurationSectionHelper.cs