Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / Inline.cs / 1 / Inline.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
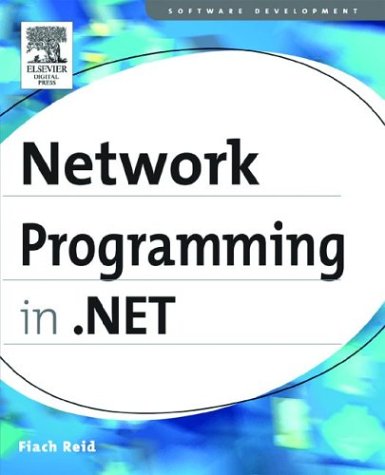
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrugalList.cs
- XmlLangPropertyAttribute.cs
- EncoderBestFitFallback.cs
- TraceSource.cs
- XPathEmptyIterator.cs
- DataPagerField.cs
- XmlDocumentFragment.cs
- DbProviderFactories.cs
- LeftCellWrapper.cs
- BinaryFormatter.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- MultiDataTrigger.cs
- AppearanceEditorPart.cs
- ChannelFactoryBase.cs
- ProxyManager.cs
- TableLayoutStyleCollection.cs
- DesignerCategoryAttribute.cs
- PseudoWebRequest.cs
- BrowserCapabilitiesFactoryBase.cs
- ColorKeyFrameCollection.cs
- InvalidPipelineStoreException.cs
- Preprocessor.cs
- BigIntegerStorage.cs
- KeyFrames.cs
- SoapObjectReader.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ZoneIdentityPermission.cs
- HostingEnvironmentWrapper.cs
- LinkArea.cs
- OrderedDictionary.cs
- ContextMenuStripActionList.cs
- InfiniteIntConverter.cs
- TableCellCollection.cs
- DataGridCell.cs
- PageThemeBuildProvider.cs
- SqlDataAdapter.cs
- StoreConnection.cs
- ProvidePropertyAttribute.cs
- AliasExpr.cs
- OutputWindow.cs
- StringAnimationBase.cs
- ValidationRuleCollection.cs
- LocalizationComments.cs
- HelpProvider.cs
- FactoryRecord.cs
- CopyCodeAction.cs
- Encoder.cs
- CqlErrorHelper.cs
- XmlSchemaGroupRef.cs
- Operators.cs
- HostProtectionPermission.cs
- CapabilitiesPattern.cs
- DateTimeStorage.cs
- PropertyItemInternal.cs
- GetPageCompletedEventArgs.cs
- SafeRightsManagementQueryHandle.cs
- ScriptIgnoreAttribute.cs
- Row.cs
- ConfigurationSectionCollection.cs
- ReflectionUtil.cs
- HtmlFormWrapper.cs
- InfoCardTrace.cs
- OleDbRowUpdatedEvent.cs
- SafeEventLogWriteHandle.cs
- SqlRetyper.cs
- SQLInt32Storage.cs
- EventListenerClientSide.cs
- ApplyTemplatesAction.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- PropertyTab.cs
- NameValueSectionHandler.cs
- Signature.cs
- TransferRequestHandler.cs
- CacheAxisQuery.cs
- WebPartTransformerCollection.cs
- OpenTypeLayout.cs
- ToolStrip.cs
- BaseParser.cs
- JulianCalendar.cs
- SqlUdtInfo.cs
- SrgsText.cs
- MailHeaderInfo.cs
- Renderer.cs
- SecurityKeyIdentifier.cs
- DoubleLinkList.cs
- _NestedSingleAsyncResult.cs
- AspCompat.cs
- MimePart.cs
- DataListItem.cs
- DateTimeConverter2.cs
- SelectionItemPattern.cs
- BuildProviderAppliesToAttribute.cs
- WebPartConnectVerb.cs
- XmlUtil.cs
- ParenExpr.cs
- XmlReaderSettings.cs
- URLString.cs
- Facet.cs
- HttpWebRequestElement.cs
- FixedSOMFixedBlock.cs