Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputCheckBox.cs / 1 / HtmlInputCheckBox.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputCheckBox.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ DefaultEvent("ServerChange"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputCheckBox : HtmlInputControl, IPostBackDataHandler { private static readonly object EventServerChange = new object(); /* * Creates an intrinsic Html INPUT type=checkbox control. */ ////// The ///class defines the methods, /// properties, and events for the HtmlInputCheckBox control. This class allows /// programmatic access to the HTML <input type= /// checkbox> /// element on the server. /// /// public HtmlInputCheckBox() : base("checkbox") { } /* * Checked property. */ ///Initializes a new instance of a ///class. /// [ WebCategory("Default"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), TypeConverter(typeof(MinimizableAttributeTypeConverter)) ] public bool Checked { get { string s = Attributes["checked"]; return((s != null) ? (s.Equals("checked")) : false); } set { if (value) Attributes["checked"] = "checked"; else Attributes["checked"] = null; } } /* * Adds an event handler for the OnServerChange event. * value: New handler to install for this event. */ ///Gets or sets a value indicating whether the checkbox is /// currently selected. ////// [ WebCategory("Action"), WebSysDescription(SR.Control_OnServerCheckChanged) ] public event EventHandler ServerChange { add { Events.AddHandler(EventServerChange, value); } remove { Events.RemoveHandler(EventServerChange, value); } } /* * This method is invoked just prior to rendering. */ ///Occurs when ////// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && !Disabled) { Page.RegisterRequiresPostBack(this); Page.RegisterEnabledControl(this); } // if no change handler, no need to save posted property unless // we are disabled if (Events[EventServerChange] == null && !Disabled) { ViewState.SetItemDirty("checked",false); } } /* * Method used to raise the OnServerChange event. */ ////// protected virtual void OnServerChange(EventArgs e) { // invoke delegates AFTER binding EventHandler handler = (EventHandler)Events[EventServerChange]; if (handler != null) handler(this, e); } /* * Method of IPostBackDataHandler interface to process posted data. * Checkbox determines the posted Checked state. */ ///[To be supplied.] ///bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } /// protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string post = postCollection[postDataKey]; bool newValue = !String.IsNullOrEmpty(post); bool valueChanged = (newValue != Checked); Checked = newValue; if (newValue) { ValidateEvent(postDataKey); } return valueChanged; } protected override void RenderAttributes(HtmlTextWriter writer) { base.RenderAttributes(writer); if (Page != null) { Page.ClientScript.RegisterForEventValidation(RenderedNameAttribute); } } /* [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] * Method of IPostBackDataHandler interface which is invoked whenever * posted data for a control has changed. RadioButton fires an * OnServerChange event. */ /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } /// protected virtual void RaisePostDataChangedEvent() { OnServerChange(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputCheckBox.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ DefaultEvent("ServerChange"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputCheckBox : HtmlInputControl, IPostBackDataHandler { private static readonly object EventServerChange = new object(); /* * Creates an intrinsic Html INPUT type=checkbox control. */ ////// The ///class defines the methods, /// properties, and events for the HtmlInputCheckBox control. This class allows /// programmatic access to the HTML <input type= /// checkbox> /// element on the server. /// /// public HtmlInputCheckBox() : base("checkbox") { } /* * Checked property. */ ///Initializes a new instance of a ///class. /// [ WebCategory("Default"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), TypeConverter(typeof(MinimizableAttributeTypeConverter)) ] public bool Checked { get { string s = Attributes["checked"]; return((s != null) ? (s.Equals("checked")) : false); } set { if (value) Attributes["checked"] = "checked"; else Attributes["checked"] = null; } } /* * Adds an event handler for the OnServerChange event. * value: New handler to install for this event. */ ///Gets or sets a value indicating whether the checkbox is /// currently selected. ////// [ WebCategory("Action"), WebSysDescription(SR.Control_OnServerCheckChanged) ] public event EventHandler ServerChange { add { Events.AddHandler(EventServerChange, value); } remove { Events.RemoveHandler(EventServerChange, value); } } /* * This method is invoked just prior to rendering. */ ///Occurs when ////// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && !Disabled) { Page.RegisterRequiresPostBack(this); Page.RegisterEnabledControl(this); } // if no change handler, no need to save posted property unless // we are disabled if (Events[EventServerChange] == null && !Disabled) { ViewState.SetItemDirty("checked",false); } } /* * Method used to raise the OnServerChange event. */ ////// protected virtual void OnServerChange(EventArgs e) { // invoke delegates AFTER binding EventHandler handler = (EventHandler)Events[EventServerChange]; if (handler != null) handler(this, e); } /* * Method of IPostBackDataHandler interface to process posted data. * Checkbox determines the posted Checked state. */ ///[To be supplied.] ///bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } /// protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string post = postCollection[postDataKey]; bool newValue = !String.IsNullOrEmpty(post); bool valueChanged = (newValue != Checked); Checked = newValue; if (newValue) { ValidateEvent(postDataKey); } return valueChanged; } protected override void RenderAttributes(HtmlTextWriter writer) { base.RenderAttributes(writer); if (Page != null) { Page.ClientScript.RegisterForEventValidation(RenderedNameAttribute); } } /* [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] * Method of IPostBackDataHandler interface which is invoked whenever * posted data for a control has changed. RadioButton fires an * OnServerChange event. */ /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } /// protected virtual void RaisePostDataChangedEvent() { OnServerChange(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
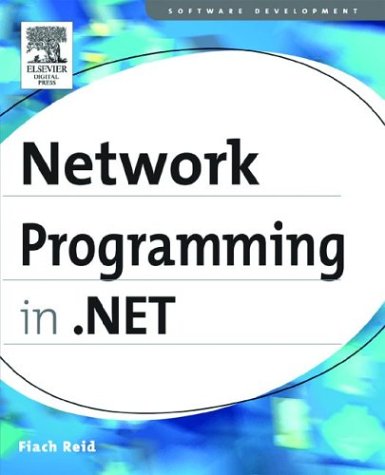
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextFormatter.cs
- NativeMethods.cs
- CompositeKey.cs
- EventMappingSettings.cs
- Button.cs
- ObjectView.cs
- UserInitiatedNavigationPermission.cs
- ProfileParameter.cs
- WebDisplayNameAttribute.cs
- BitmapCacheBrush.cs
- CellRelation.cs
- SyntaxCheck.cs
- AxHost.cs
- WindowsTitleBar.cs
- AstNode.cs
- Vector3DAnimation.cs
- PropertyRecord.cs
- IPGlobalProperties.cs
- SQLBytesStorage.cs
- CodeArgumentReferenceExpression.cs
- HttpClientProtocol.cs
- XPathNavigator.cs
- Application.cs
- XhtmlBasicImageAdapter.cs
- TTSEngineTypes.cs
- ProgressChangedEventArgs.cs
- TextContainerChangedEventArgs.cs
- DocumentApplicationDocumentViewer.cs
- DiscoveryClientRequestChannel.cs
- DataService.cs
- AssociationSetMetadata.cs
- OletxTransactionManager.cs
- METAHEADER.cs
- DataColumn.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- Configuration.cs
- DbConnectionPoolIdentity.cs
- FixedSOMLineRanges.cs
- cookieexception.cs
- WrapPanel.cs
- NavigationService.cs
- ObjectHandle.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- DelegatingTypeDescriptionProvider.cs
- DiscoveryEndpointValidator.cs
- BitmapDownload.cs
- CallbackHandler.cs
- MembershipSection.cs
- HttpWebResponse.cs
- RegionData.cs
- WindowsListViewItem.cs
- WebPartTransformerCollection.cs
- RoutingEndpointTrait.cs
- querybuilder.cs
- DependencyObject.cs
- BaseCollection.cs
- InvokePattern.cs
- MailWriter.cs
- BmpBitmapDecoder.cs
- ComponentEditorPage.cs
- AssemblyBuilder.cs
- XmlILModule.cs
- StringKeyFrameCollection.cs
- GenericRootAutomationPeer.cs
- QueryOperatorEnumerator.cs
- TemplatedMailWebEventProvider.cs
- MenuStrip.cs
- ChannelPool.cs
- PartEditor.cs
- UIElementPropertyUndoUnit.cs
- CngProperty.cs
- InternalEnumValidatorAttribute.cs
- OneOf.cs
- ImageMetadata.cs
- FixedStringLookup.cs
- TypeBuilderInstantiation.cs
- NumericUpDown.cs
- TableLayoutPanel.cs
- Trace.cs
- ExpressionCopier.cs
- InstanceDataCollectionCollection.cs
- ProvideValueServiceProvider.cs
- DataGridViewComponentPropertyGridSite.cs
- NetworkInformationPermission.cs
- PenContext.cs
- ArgumentOutOfRangeException.cs
- SqlDataAdapter.cs
- PageStatePersister.cs
- TextServicesContext.cs
- BitmapFrameEncode.cs
- ServiceContractGenerator.cs
- ProvideValueServiceProvider.cs
- SessionPageStatePersister.cs
- URL.cs
- DbConnectionPoolIdentity.cs
- DataServiceException.cs
- RefreshPropertiesAttribute.cs
- OpenTypeLayoutCache.cs
- JournalEntryListConverter.cs
- Predicate.cs