Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Security / Policy / URL.cs / 1 / URL.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Url.cs // // Url is an IIdentity representing url internet sites. // namespace System.Security.Policy { using System.IO; using System.Security.Util; using UrlIdentityPermission = System.Security.Permissions.UrlIdentityPermission; using System.Runtime.Serialization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Url : IIdentityPermissionFactory, IBuiltInEvidence { private URLString m_url; internal Url() { m_url = null; } internal Url( SerializationInfo info, StreamingContext context ) { m_url = new URLString( (String)info.GetValue( "Url", typeof( String ) ) ); } internal Url( String name, bool parsed ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name, parsed ); } public Url( String name ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name ); } public String Value { get { if (m_url == null) return null; return m_url.ToString(); } } internal URLString GetURLString() { return m_url; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new UrlIdentityPermission( m_url ); } public override bool Equals(Object o) { if (o == null) return false; if (o is Url) { Url url = (Url) o; if (this.m_url == null) { return url.m_url == null; } else if (url.m_url == null) { return false; } else { return this.m_url.Equals( url.m_url ); } } return false; } public override int GetHashCode() { if (this.m_url == null) return 0; else return this.m_url.GetHashCode(); } public Object Copy() { Url url = new Url(); url.m_url = this.m_url; return url; } internal SecurityElement ToXml() { SecurityElement root = new SecurityElement( "System.Security.Policy.Url" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Url" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_url != null) root.AddChild( new SecurityElement( "Url", m_url.ToString() ) ); return root; } public override String ToString() { return ToXml().ToString(); } ///int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position++] = BuiltInEvidenceHelper.idUrl; String value = this.Value; int length = value.Length; if (verbose) { BuiltInEvidenceHelper.CopyIntToCharArray(length, buffer, position); position += 2; } value.CopyTo( 0, buffer, position, length ); return length + position; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose ) { if (verbose) return this.Value.Length + 3; else return this.Value.Length + 1; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position) { int length = BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); position += 2; m_url = new URLString( new String(buffer, position, length )); return position + length; } #if false public void GetObjectData(SerializationInfo info, StreamingContext context) { String normalizedUrl = m_url.NormalizeUrl(); info.AddValue( "Url", normalizedUrl ); } #endif // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_url.NormalizeUrl(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Url.cs // // Url is an IIdentity representing url internet sites. // namespace System.Security.Policy { using System.IO; using System.Security.Util; using UrlIdentityPermission = System.Security.Permissions.UrlIdentityPermission; using System.Runtime.Serialization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Url : IIdentityPermissionFactory, IBuiltInEvidence { private URLString m_url; internal Url() { m_url = null; } internal Url( SerializationInfo info, StreamingContext context ) { m_url = new URLString( (String)info.GetValue( "Url", typeof( String ) ) ); } internal Url( String name, bool parsed ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name, parsed ); } public Url( String name ) { if (name == null) throw new ArgumentNullException( "name" ); m_url = new URLString( name ); } public String Value { get { if (m_url == null) return null; return m_url.ToString(); } } internal URLString GetURLString() { return m_url; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new UrlIdentityPermission( m_url ); } public override bool Equals(Object o) { if (o == null) return false; if (o is Url) { Url url = (Url) o; if (this.m_url == null) { return url.m_url == null; } else if (url.m_url == null) { return false; } else { return this.m_url.Equals( url.m_url ); } } return false; } public override int GetHashCode() { if (this.m_url == null) return 0; else return this.m_url.GetHashCode(); } public Object Copy() { Url url = new Url(); url.m_url = this.m_url; return url; } internal SecurityElement ToXml() { SecurityElement root = new SecurityElement( "System.Security.Policy.Url" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Url" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_url != null) root.AddChild( new SecurityElement( "Url", m_url.ToString() ) ); return root; } public override String ToString() { return ToXml().ToString(); } /// int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position++] = BuiltInEvidenceHelper.idUrl; String value = this.Value; int length = value.Length; if (verbose) { BuiltInEvidenceHelper.CopyIntToCharArray(length, buffer, position); position += 2; } value.CopyTo( 0, buffer, position, length ); return length + position; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose ) { if (verbose) return this.Value.Length + 3; else return this.Value.Length + 1; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position) { int length = BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); position += 2; m_url = new URLString( new String(buffer, position, length )); return position + length; } #if false public void GetObjectData(SerializationInfo info, StreamingContext context) { String normalizedUrl = m_url.NormalizeUrl(); info.AddValue( "Url", normalizedUrl ); } #endif // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_url.NormalizeUrl(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
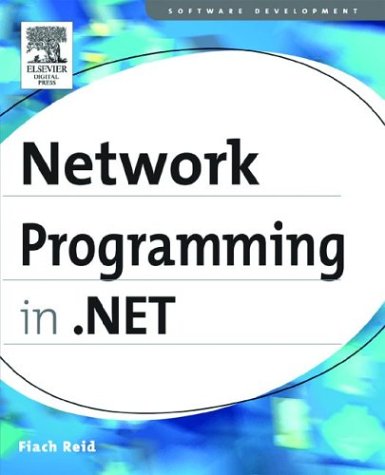
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlNodeAnnotations.cs
- LabelEditEvent.cs
- SafeBitVector32.cs
- Matrix.cs
- ZipIOFileItemStream.cs
- AlphabeticalEnumConverter.cs
- CachedTypeface.cs
- ReachSerializer.cs
- StorageSetMapping.cs
- _ConnectOverlappedAsyncResult.cs
- SelectionProviderWrapper.cs
- XmlReader.cs
- PointCollectionValueSerializer.cs
- DesignTimeParseData.cs
- SrgsGrammarCompiler.cs
- WebPageTraceListener.cs
- SimpleType.cs
- CellLabel.cs
- ComponentEvent.cs
- ServiceObjectContainer.cs
- WebMessageBodyStyleHelper.cs
- Triangle.cs
- ValidationRule.cs
- ScriptRef.cs
- BoundColumn.cs
- RSAOAEPKeyExchangeFormatter.cs
- HashHelper.cs
- LinkButton.cs
- ListViewUpdateEventArgs.cs
- BitmapDecoder.cs
- PageRouteHandler.cs
- ValidationHelper.cs
- UTF8Encoding.cs
- SurrogateEncoder.cs
- SystemIPInterfaceStatistics.cs
- SafeLibraryHandle.cs
- SoapHeader.cs
- PopupRootAutomationPeer.cs
- TemplateContent.cs
- XmlCharType.cs
- LocatorBase.cs
- ProcessModuleCollection.cs
- SqlDelegatedTransaction.cs
- DataGridViewCellCancelEventArgs.cs
- DataColumnChangeEvent.cs
- ViewEvent.cs
- AxHostDesigner.cs
- OverflowException.cs
- LicenseProviderAttribute.cs
- CheckableControlBaseAdapter.cs
- CharUnicodeInfo.cs
- SqlInternalConnectionSmi.cs
- ObservableDictionary.cs
- ApplyHostConfigurationBehavior.cs
- OracleException.cs
- SizeChangedInfo.cs
- SerTrace.cs
- WebRequestModuleElementCollection.cs
- ScrollableControl.cs
- PriorityItem.cs
- UrlPath.cs
- CompoundFileIOPermission.cs
- SynchronizationContext.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- ControlDesignerState.cs
- OwnerDrawPropertyBag.cs
- LinkButton.cs
- FilteredReadOnlyMetadataCollection.cs
- SelectionProcessor.cs
- StateDesigner.TransitionInfo.cs
- ELinqQueryState.cs
- HtmlPageAdapter.cs
- KeyValueConfigurationElement.cs
- PipeStream.cs
- XPathAxisIterator.cs
- CachedPathData.cs
- RemotingConfiguration.cs
- SingleResultAttribute.cs
- RootBrowserWindowAutomationPeer.cs
- DataGridCommandEventArgs.cs
- DataGridCommandEventArgs.cs
- SerializerWriterEventHandlers.cs
- BitStream.cs
- Identity.cs
- Lease.cs
- RijndaelManaged.cs
- Error.cs
- Scene3D.cs
- BinHexDecoder.cs
- CodeTypeReference.cs
- EditorPartCollection.cs
- SyntaxCheck.cs
- InstalledFontCollection.cs
- TextEditorSelection.cs
- InkCanvasFeedbackAdorner.cs
- Quad.cs
- DataConnectionHelper.cs
- XmlReader.cs
- DependencyPropertyValueSerializer.cs
- XmlQueryTypeFactory.cs