Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / NetworkInformation / SystemIPAddressInformation.cs / 1 / SystemIPAddressInformation.cs
////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; //this is the main addressinformation class that contains the ipaddress //and other properties internal class SystemIPAddressInformation:IPAddressInformation{ IPAddress address; internal bool transient = false; internal bool dnsEligible = true; /* // Consider removing. internal SystemIPAddressInformation(){} */ internal SystemIPAddressInformation(IPAddress address) { this.address = address; if (address.AddressFamily == AddressFamily.InterNetwork) { //DngEligible is true except for 169.254.x.x addresses (the APIPA addresses ) dnsEligible = !((address.m_Address & 0x0000FEA9)>0); } } internal SystemIPAddressInformation(IpAdapterUnicastAddress adapterAddress,IPAddress address) { this.address = address; transient = (adapterAddress.flags & AdapterAddressFlags.Transient)>0; dnsEligible = (adapterAddress.flags & AdapterAddressFlags.DnsEligible)>0; } internal SystemIPAddressInformation(IpAdapterAddress adapterAddress,IPAddress address) { this.address = address; transient = (adapterAddress.flags & AdapterAddressFlags.Transient)>0; dnsEligible = (adapterAddress.flags & AdapterAddressFlags.DnsEligible)>0; } public override IPAddress Address{get {return address;}} /// /// Provides support for ip configuation information and statistics. /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (transient); } } ///This address can be used for DNS. public override bool IsDnsEligible{ get { return (dnsEligible); } } //helper method that marshals the addressinformation into the classes internal static IPAddressCollection ToAddressCollection(IntPtr ptr,IPVersion versionSupported) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. IPAddressCollection addressList = new IPAddressCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(ep.Address); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; //only add addresses that are the same type as what is supported by the interface //this fixes a bug in iphelper regarding dns addresses if (((family == AddressFamily.InterNetwork) && ((versionSupported & IPVersion.IPv4) > 0)) || ((family == AddressFamily.InterNetworkV6) && ((versionSupported & IPVersion.IPv6) > 0))) { sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(ep.Address); } } return addressList; } //helper method that marshals the addressinformation into the classes internal static IPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr,IPVersion versionSupported) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. IPAddressInformationCollection addressList = new IPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; //only add addresses that are the same type as what is supported by the interface //this fixes a bug in iphelper regarding dns addresses if (((family == AddressFamily.InterNetwork) && ((versionSupported & IPVersion.IPv4) > 0)) || ((family == AddressFamily.InterNetworkV6) && ((versionSupported & IPVersion.IPv6) > 0))) { sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemIPAddressInformation(addr,ep.Address)); } } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. ////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; //this is the main addressinformation class that contains the ipaddress //and other properties internal class SystemIPAddressInformation:IPAddressInformation{ IPAddress address; internal bool transient = false; internal bool dnsEligible = true; /* // Consider removing. internal SystemIPAddressInformation(){} */ internal SystemIPAddressInformation(IPAddress address) { this.address = address; if (address.AddressFamily == AddressFamily.InterNetwork) { //DngEligible is true except for 169.254.x.x addresses (the APIPA addresses ) dnsEligible = !((address.m_Address & 0x0000FEA9)>0); } } internal SystemIPAddressInformation(IpAdapterUnicastAddress adapterAddress,IPAddress address) { this.address = address; transient = (adapterAddress.flags & AdapterAddressFlags.Transient)>0; dnsEligible = (adapterAddress.flags & AdapterAddressFlags.DnsEligible)>0; } internal SystemIPAddressInformation(IpAdapterAddress adapterAddress,IPAddress address) { this.address = address; transient = (adapterAddress.flags & AdapterAddressFlags.Transient)>0; dnsEligible = (adapterAddress.flags & AdapterAddressFlags.DnsEligible)>0; } public override IPAddress Address{get {return address;}} /// /// Provides support for ip configuation information and statistics. /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (transient); } } ///This address can be used for DNS. public override bool IsDnsEligible{ get { return (dnsEligible); } } //helper method that marshals the addressinformation into the classes internal static IPAddressCollection ToAddressCollection(IntPtr ptr,IPVersion versionSupported) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. IPAddressCollection addressList = new IPAddressCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(ep.Address); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; //only add addresses that are the same type as what is supported by the interface //this fixes a bug in iphelper regarding dns addresses if (((family == AddressFamily.InterNetwork) && ((versionSupported & IPVersion.IPv4) > 0)) || ((family == AddressFamily.InterNetworkV6) && ((versionSupported & IPVersion.IPv6) > 0))) { sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(ep.Address); } } return addressList; } //helper method that marshals the addressinformation into the classes internal static IPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr,IPVersion versionSupported) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. IPAddressInformationCollection addressList = new IPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; //only add addresses that are the same type as what is supported by the interface //this fixes a bug in iphelper regarding dns addresses if (((family == AddressFamily.InterNetwork) && ((versionSupported & IPVersion.IPv4) > 0)) || ((family == AddressFamily.InterNetworkV6) && ((versionSupported & IPVersion.IPv6) > 0))) { sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemIPAddressInformation(addr,ep.Address)); } } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
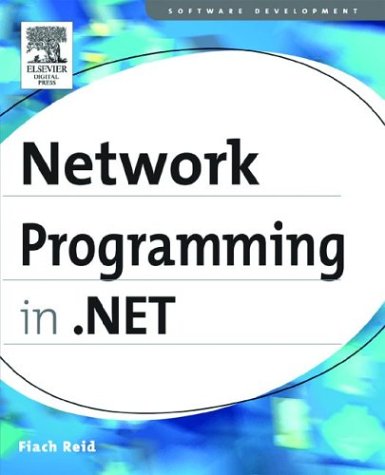
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestResizeEvent.cs
- ISAPIRuntime.cs
- XmlCollation.cs
- TableRow.cs
- XamlSerializationHelper.cs
- PartialTrustHelpers.cs
- ValidationError.cs
- ConfigViewGenerator.cs
- SafeNativeMethodsMilCoreApi.cs
- IDQuery.cs
- ReceiveMessageContent.cs
- ExplicitDiscriminatorMap.cs
- ButtonRenderer.cs
- DbProviderFactory.cs
- BindToObject.cs
- DbTransaction.cs
- LinqDataSourceEditData.cs
- CodeDomDesignerLoader.cs
- ExpressionNormalizer.cs
- InvokeWebService.cs
- NonVisualControlAttribute.cs
- XPathNavigatorReader.cs
- PassportAuthenticationEventArgs.cs
- SqlMultiplexer.cs
- WebEvents.cs
- DirectionalLight.cs
- MenuEventArgs.cs
- DirectoryNotFoundException.cs
- DeviceContext2.cs
- HwndKeyboardInputProvider.cs
- DataBoundControlActionList.cs
- PackagePartCollection.cs
- connectionpool.cs
- NonBatchDirectoryCompiler.cs
- Internal.cs
- SqlIdentifier.cs
- DPTypeDescriptorContext.cs
- GeneralTransform3DTo2DTo3D.cs
- ReadOnlyCollectionBase.cs
- RadioButtonFlatAdapter.cs
- ListViewGroupConverter.cs
- AnimationClockResource.cs
- PropertyToken.cs
- BitmapEffectRenderDataResource.cs
- BinarySecretSecurityToken.cs
- LazyTextWriterCreator.cs
- RayHitTestParameters.cs
- StateMachineWorkflowDesigner.cs
- OneOfScalarConst.cs
- Mutex.cs
- HitTestResult.cs
- BinaryConverter.cs
- SqlBuilder.cs
- Models.cs
- DispatchChannelSink.cs
- ConditionalWeakTable.cs
- PriorityBinding.cs
- MemberProjectionIndex.cs
- EventsTab.cs
- Camera.cs
- WorkflowInstanceContextProvider.cs
- ErrorLog.cs
- SystemTcpStatistics.cs
- ValuePattern.cs
- ProvidePropertyAttribute.cs
- XmlILAnnotation.cs
- XPathNavigator.cs
- MasterPageParser.cs
- EmptyEnumerable.cs
- DesignerForm.cs
- SystemIPv6InterfaceProperties.cs
- BaseValidator.cs
- Point3DCollectionValueSerializer.cs
- JsonEnumDataContract.cs
- DecoderFallback.cs
- RenderingBiasValidation.cs
- SqlServer2KCompatibilityCheck.cs
- RowBinding.cs
- RemoteWebConfigurationHostStream.cs
- DataSourceSelectArguments.cs
- ApplicationFileParser.cs
- DataRow.cs
- BindingMAnagerBase.cs
- InstanceLockQueryResult.cs
- EditCommandColumn.cs
- SchemaElementLookUpTableEnumerator.cs
- ConfigXmlText.cs
- XmlIlGenerator.cs
- GZipUtils.cs
- ExtractCollection.cs
- SqlAliaser.cs
- NameValuePair.cs
- GenericEnumerator.cs
- Site.cs
- OpCodes.cs
- WpfWebRequestHelper.cs
- SafePEFileHandle.cs
- DesignerCommandAdapter.cs
- ADMembershipUser.cs
- SqlBulkCopyColumnMappingCollection.cs