Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Policy / Site.cs / 1 / Site.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Site.cs // // Site is an IIdentity representing internet sites. // namespace System.Security.Policy { using System.Security.Util; using System.Globalization; using SiteIdentityPermission = System.Security.Permissions.SiteIdentityPermission; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Site : IIdentityPermissionFactory, IBuiltInEvidence { private SiteString m_name; internal Site() { m_name = null; } public Site(String name) { if (name == null) throw new ArgumentNullException("name"); m_name = new SiteString( name ); } internal Site( byte[] id, String name ) { m_name = ParseSiteFromUrl( name ); } public static Site CreateFromUrl( String url ) { Site site = new Site(); site.m_name = ParseSiteFromUrl( url ); return site; } private static SiteString ParseSiteFromUrl( String name ) { URLString urlString = new URLString( name ); if (String.Compare( urlString.Scheme, "file", StringComparison.OrdinalIgnoreCase) == 0) throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidSite" ) ); return new SiteString( new URLString( name ).Host ); } public String Name { get { if (m_name != null) return m_name.ToString(); else return null; } } internal SiteString GetSiteString() { return m_name; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new SiteIdentityPermission( Name ); } public override bool Equals(Object o) { if (o is Site) { Site s = (Site) o; if (Name == null) return (s.Name == null); return String.Compare( Name, s.Name, StringComparison.OrdinalIgnoreCase) == 0; } return false; } public override int GetHashCode() { String name = this.Name; if (name == null) return 0; else return name.GetHashCode(); } public Object Copy() { return new Site(this.Name); } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Site" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Site" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if(m_name != null) elem.AddChild( new SecurityElement( "Name", m_name.ToString() ) ); return elem; } ///int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position++] = BuiltInEvidenceHelper.idSite; String name = this.Name; int length = name.Length; if (verbose) { BuiltInEvidenceHelper.CopyIntToCharArray(length, buffer, position); position += 2; } name.CopyTo( 0, buffer, position, length ); return length + position; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose) { if (verbose) return this.Name.Length + 3; else return this.Name.Length + 1; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position) { int length = BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); position += 2; m_name = new SiteString( new String(buffer, position, length )); return position + length; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_name.ToString().ToUpper(CultureInfo.InvariantCulture); } } }
Link Menu
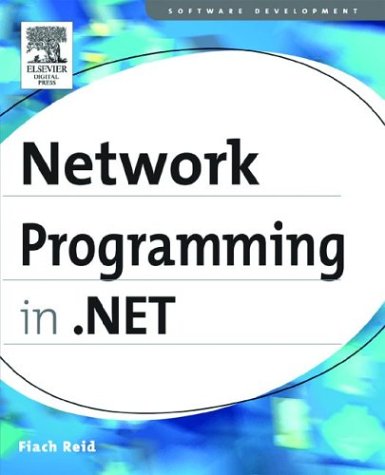
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Int32KeyFrameCollection.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- SmiGettersStream.cs
- IntSecurity.cs
- _WinHttpWebProxyDataBuilder.cs
- DocumentPage.cs
- SecurityBindingElementImporter.cs
- ReceiveContextCollection.cs
- XmlDataCollection.cs
- FunctionCommandText.cs
- PreviewPageInfo.cs
- BaseTypeViewSchema.cs
- CollectionViewProxy.cs
- Light.cs
- CodeTypeMemberCollection.cs
- GeneralTransformCollection.cs
- OdbcReferenceCollection.cs
- ImageAnimator.cs
- CategoryNameCollection.cs
- SafeNativeMethods.cs
- TreeNodeSelectionProcessor.cs
- HandlerBase.cs
- SmtpDigestAuthenticationModule.cs
- TextRangeAdaptor.cs
- MSAAEventDispatcher.cs
- TreeNodeBindingCollection.cs
- WebPartDescription.cs
- httpserverutility.cs
- SocketInformation.cs
- WebPartAddingEventArgs.cs
- _SSPIWrapper.cs
- DataGridViewMethods.cs
- TemplateParser.cs
- ServiceDeploymentInfo.cs
- EncryptedPackageFilter.cs
- ImpersonationContext.cs
- ProxyAssemblyNotLoadedException.cs
- DebugTraceHelper.cs
- ExtendedPropertyDescriptor.cs
- Selection.cs
- CodeTypeReferenceExpression.cs
- TTSVoice.cs
- PeerInvitationResponse.cs
- BaseUriWithWildcard.cs
- EmptyStringExpandableObjectConverter.cs
- Renderer.cs
- FontStretches.cs
- PropertyChangedEventArgs.cs
- EncoderNLS.cs
- CodeBinaryOperatorExpression.cs
- OdbcTransaction.cs
- URLMembershipCondition.cs
- SelectionPatternIdentifiers.cs
- Enum.cs
- MsmqIntegrationInputChannel.cs
- EllipseGeometry.cs
- HtmlMeta.cs
- GradientStop.cs
- WebReferenceOptions.cs
- MediaScriptCommandRoutedEventArgs.cs
- TabControl.cs
- HostProtectionPermission.cs
- ContextProperty.cs
- TableRow.cs
- ReceiveActivityDesigner.cs
- ContentPlaceHolder.cs
- PhysicalAddress.cs
- StringInfo.cs
- SoapTransportImporter.cs
- SqlStream.cs
- XmlTextReaderImpl.cs
- RubberbandSelector.cs
- CurrencyWrapper.cs
- validationstate.cs
- TypeSchema.cs
- DictionarySectionHandler.cs
- SocketAddress.cs
- BasicDesignerLoader.cs
- XamlTemplateSerializer.cs
- Stackframe.cs
- DataGridViewCellFormattingEventArgs.cs
- ArithmeticException.cs
- ButtonRenderer.cs
- ServiceInfo.cs
- PublishLicense.cs
- JournalEntryStack.cs
- SizeConverter.cs
- XPathDescendantIterator.cs
- WindowPattern.cs
- TableItemPattern.cs
- SelectedCellsCollection.cs
- UnionCodeGroup.cs
- JournalEntryStack.cs
- XmlSchemaComplexContentExtension.cs
- ToolStripStatusLabel.cs
- XmlSchemaComplexType.cs
- DataServiceQueryException.cs
- XPathAncestorQuery.cs
- DtdParser.cs
- xmlfixedPageInfo.cs